Why Java Serialization is Misunderstood and Maligned
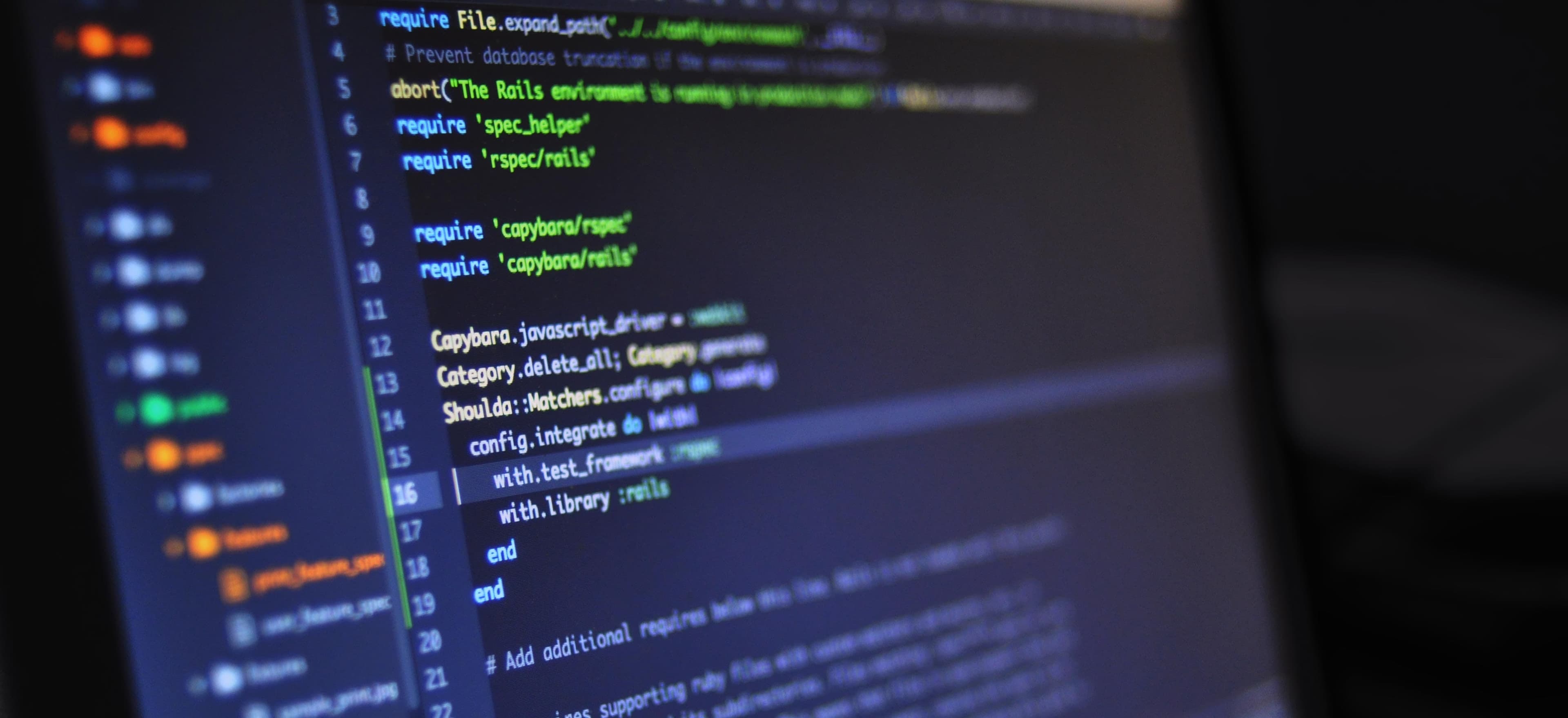
- Published on
Why Java Serialization is Misunderstood and Maligned
Java Serialization is a powerful feature capable of transforming an object into a byte stream. Yet, despite its utility, it is often misunderstood and maligned for several reasons. In this post, we will delve into the intricacies of Java Serialization, unveil why it's perceived negatively, and provide guidance on how to harness its power effectively.
What is Java Serialization?
At a fundamental level, Java Serialization is the process of converting an object into a format that can be easily stored or transmitted, typically a byte stream. This serialized representation can later be deserialized back into its original form. This functionality is particularly useful in scenarios involving data persistence, such as saving the state of a Java object into a file, or in distributed systems where objects need to be transmitted over a network.
Here's a simple illustration to demonstrate how Java Serialization works:
import java.io.*;
// A simple class to be serialized
class User implements Serializable {
private String name;
private int age;
// Constructor
public User(String name, int age) {
this.name = name;
this.age = age;
}
@Override
public String toString() {
return "User{name='" + name + "', age=" + age + '}';
}
}
public class SerializationDemo {
public static void main(String[] args) {
User user = new User("Alice", 30);
// Serialization: converting the User object into a byte stream
try (ObjectOutputStream oos = new ObjectOutputStream(new FileOutputStream("user.ser"))) {
oos.writeObject(user);
System.out.println("User has been serialized: " + user);
} catch (IOException e) {
e.printStackTrace();
}
}
}
In the code above, we define a simple User
class, implement the Serializable
interface, and serialize an instance of that class into a file named "user.ser". The Serializable
interface signals to the Java runtime that the object can be serialized.
Now, let's examine the deserialization process:
import java.io.*;
public class DeserializationDemo {
public static void main(String[] args) {
User user = null;
// Deserialization: converting the byte stream back into a User object
try (ObjectInputStream ois = new ObjectInputStream(new FileInputStream("user.ser"))) {
user = (User) ois.readObject();
System.out.println("User has been deserialized: " + user);
} catch (IOException | ClassNotFoundException e) {
e.printStackTrace();
}
}
}
This snippet demonstrates how to read the serialized object back from the file. The deserialization process reinstates the object to its original state, allowing you to work with it as if it was never transformed.
The Misunderstanding of Java Serialization
Despite the clear advantages, several issues make Java Serialization often misunderstood:
1. Security Concerns
Java Serialization is inherently risky. When an application deserializes data, it can inadvertently allow the execution of malicious code if the source of that data isn't trustworthy. Attackers might deploy serialized objects to execute harmful actions within an application.
2. Versioning Problems
One of the leading problems with Java Serialization is related to versioning. When class structures change—like adding new fields or modifying existing ones—previously serialized objects can become incompatible. This can lead to InvalidClassException
, burdening developers with managing serialized class versions meticulously.
3. Performance Overhead
Serialization can introduce significant performance overhead, especially when dealing with large objects or collections of objects. Java's default serialization mechanism can be slow and not as space-efficient as other binary serialization libraries available in the ecosystem.
4. Lack of Control
The built-in serialization mechanism in Java provides minimal control over how fields are serialized. By default, all fields, regardless of visibility or relevance to the application's state, are serialized. This could lead to unintended information leaks or excessive data retention.
Why Java Serialization is Maligned
Now that we've laid the groundwork understanding Java Serialization, let's discuss the negative perceptions and faults attributed to it:
-
Security Risks: Java developers often prioritize security. The risks of deserialization attacks loom large, deterring the use of native serialization.
-
Complexity in Maintenance: Maintaining classes that may evolve over time requires careful handling of transient fields, serialVersionUID, and overall management of the serialization protocol.
-
Alternatives: Many developers prefer faster and safer alternatives for data serialization. Libraries such as Kryo and Protocol Buffers provide simpler APIs and additional features without many drawbacks of Java's native serialization.
Best Practices for Java Serialization
Even with its downsides, there are ways to leverage Java Serialization effectively. Here are some best practices:
Use serialVersionUID
Always declare a serialVersionUID
in your Serializable classes. This unique identifier ensures that during deserialization, the class's compatibility is verified and prevents InvalidClassException
.
private static final long serialVersionUID = 1L;
Mark Fields as Transient
Use the transient
keyword for fields that do not need to be serialized. This reduces the risk of unnecessary data leaks and saves on serialization costs.
private transient String password; // Do not serialize password
Implement ReadResolve and WriteReplace
To control how objects are serialized and deserialized, you can implement the writeReplace
and readResolve
methods. This pattern allows for an analog or a different representation of the serialized object.
private Object writeReplace() {
return new User(name, age + 1); // For instance, incrementing age during serialization
}
The Last Word
Java Serialization, while misunderstood and maligned, plays an essential role in Java applications. The key is understanding its strengths and weaknesses and leveraging best practices to mitigate risks. By being aware of its pitfalls—including security, versioning, and performance—you can better determine when to use it, how to implement it, and what alternatives might be suitable.
In conclusion, embrace Java Serialization with caution, but don't shy away from it. In the right scenarios, it can be a valuable tool in your Java programming toolkit.
For further reading on serialization in Java, check out the Java Documentation on Serialization. If you're looking for robust alternatives, considering Kryo might be the right move for your project.
Happy coding!