Maximize Performance: C3PO Connection Pooling in Spring
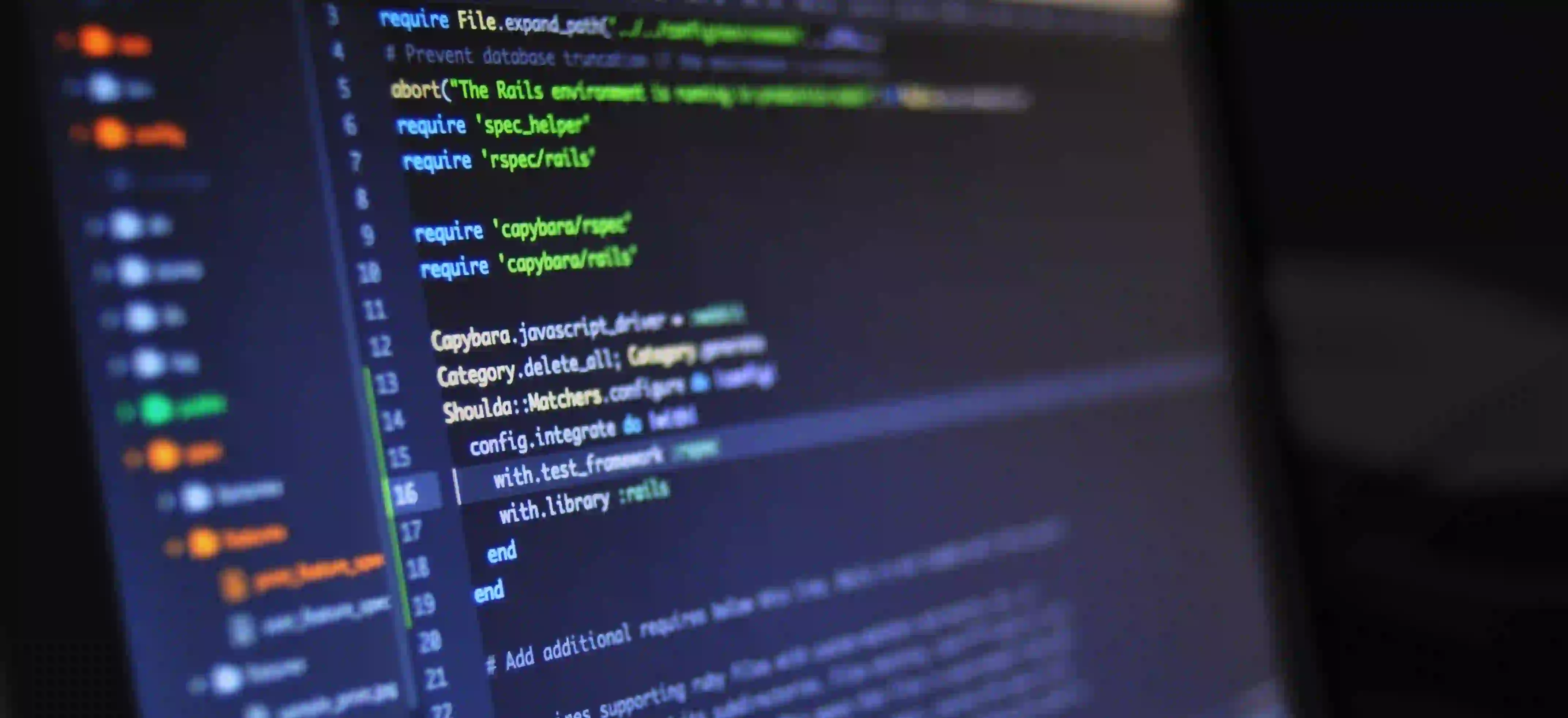
Maximize Performance: C3PO Connection Pooling in Spring
Connection pooling is an essential technique in modern application development for enhancing database interactions. When working with Java applications, specifically in Spring, utilizing a robust connection pool like C3PO can dramatically boost performance and resource management. This post will delve into why connection pooling is important, how C3PO fits into the Spring ecosystem, and provide practical examples with code snippets to illustrate best practices.
Why Connection Pooling?
Every time a Java application interacts with a database, it typically establishes a connection. This process, which involves network latency and authentication, can be time-consuming and resource-intensive. Here are the primary benefits of connection pooling:
- Resource Efficiency: Connection pools keep a cache of database connections, reducing the overhead associated with creating and destroying connections.
- Performance: With a pool of ready-to-use connections, applications can handle multiple requests quickly.
- Flexibility: Proper configuration allows for dynamic adjustment to varying workloads.
- Scalability: A well-tuned pool can handle increased load with minimal performance impacts.
What is C3PO?
C3PO is an open-source library that provides the functionality for connection pooling in Java Database Connectivity (JDBC). It seamlessly integrates with various Java application frameworks, including Spring. C3PO is a popular choice due to its:
- Ease of configuration: Simple XML and Java configuration options.
- Features: Supports automatic connection testing, the ability to handle multiple databases, and extensive logging capabilities.
Setting Up C3PO in Your Spring Application
To maximize performance using C3PO, we need to integrate it with a Spring-based application. This section will cover Maven dependency inclusion, configuration, and sample code snippets.
Step 1: Maven Dependency
Add the C3PO dependency to your pom.xml
. This will ensure that your project can leverage the C3PO connection pool.
<dependency>
<groupId>com.mchange</groupId>
<artifactId>c3p0</artifactId>
<version>0.9.5.5</version>
</dependency>
Step 2: Configuring C3PO in Spring
Next, we need to set up C3PO in our Spring configuration file. Below is a basic example of how to configure it in a Java-based configuration class.
import com.mchange.v2.c3p0.ComboPooledDataSource;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.jdbc.datasource.DataSourceTransactionManager;
import org.springframework.orm.jpa.JpaTransactionManager;
import org.springframework.orm.jpa.LocalContainerEntityManagerFactoryBean;
import javax.sql.DataSource;
import java.beans.PropertyVetoException;
@Configuration
public class DataSourceConfig {
@Bean
public DataSource dataSource() throws PropertyVetoException {
ComboPooledDataSource dataSource = new ComboPooledDataSource();
dataSource.setDriverClass("com.mysql.cj.jdbc.Driver"); // MySQL driver class
dataSource.setJdbcUrl("jdbc:mysql://localhost:3306/mydb");
dataSource.setUser("username");
dataSource.setPassword("password");
// Connection pool properties
dataSource.setMinPoolSize(5);
dataSource.setMaxPoolSize(20);
dataSource.setAcquireIncrement(5);
dataSource.setMaxIdleTime(300);
return dataSource;
}
@Bean
public JpaTransactionManager transactionManager() {
return new JpaTransactionManager();
}
}
Explanation of Configuration Properties
- Driver Class: This is the fully qualified name of the JDBC driver for the respective database.
- JdbcURL: The connection string that contains the host and database name.
- User & Password: Credentials to access the database.
- Pool Size Parameters: Adjustments can be made based on the expected load. For example, setting
setMaxPoolSize(20)
allows for a maximum of 20 connections in the pool.
For more details on C3PO properties, check the C3P0 Documentation.
Step 3: Using C3PO in Your Repository
Once C3PO is set up, you can simply inject the DataSource
into your repository beans. Below is an example of using the DataSource
to get a connection:
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.jdbc.core.JdbcTemplate;
import org.springframework.stereotype.Repository;
import javax.sql.DataSource;
@Repository
public class ExampleRepository {
private final JdbcTemplate jdbcTemplate;
@Autowired
public ExampleRepository(DataSource dataSource) {
this.jdbcTemplate = new JdbcTemplate(dataSource);
}
public void createDataEntry(String value) {
String sql = "INSERT INTO example_table (value) VALUES (?)";
jdbcTemplate.update(sql, value);
}
}
In this snippet, the JdbcTemplate
simplifies interaction with the database, allowing you to execute SQL statements easily.
Performance Tuning C3PO
Once you have C3PO configured, it's crucial to optimize its settings to fit your application. Below are some essential parameters to consider for performance tuning:
Connection Testing
It is vital to ensure that connections are valid before they are handed out to the application. This can be achieved by setting up connection testing properties.
dataSource.setPreferredTestQuery("SELECT 1"); // Test query
dataSource.setTestConnectionOnCheckout(true);
dataSource.setTestConnectionOnCheckin(true);
Connection Timeout
Avoid hanging connections by setting a timeout value. This prevents the application from waiting indefinitely for a connection.
dataSource.setCheckoutTimeout(30000); // 30 seconds
Handling Connection Leaks
Setting limits on idle connections can prevent resource exhaustion due to improper connection handling.
dataSource.setIdleConnectionTestPeriod(300); // in seconds
dataSource.setMaxIdleTime(600); // in seconds
Monitoring and Troubleshooting
Monitoring connection pools in a production environment is vital. C3PO can log detailed information that helps in troubleshooting. You can enable logging by setting properties in your C3PO configuration.
dataSource.setLogStatementsEnabled(true);
dataSource.setDebugUnreturnedConnectionStackTraces(true);
Ensure your log framework (like Log4j or SLF4J) is properly configured, so you can capture relevant information about the pool's behavior.
The Last Word
C3PO connection pooling in a Spring application is an effective way to maximize performance and optimize resource usage. By reducing the overhead of establishing connections and providing a robust infrastructure for managing them, developers can greatly enhance application responsiveness and scalability.
From configuration to performance tuning, understanding the principles behind C3PO will empower you to make your Java applications more efficient and responsive. For further references and documentation, you can explore the Spring Documentation or the C3P0 GitHub Page.
Implement these best practices, and watch your application’s performance soar!
Feel free to share your thoughts or questions in the comments below! Happy coding!