Avoiding Common Dependency Injection Pitfalls in Spring
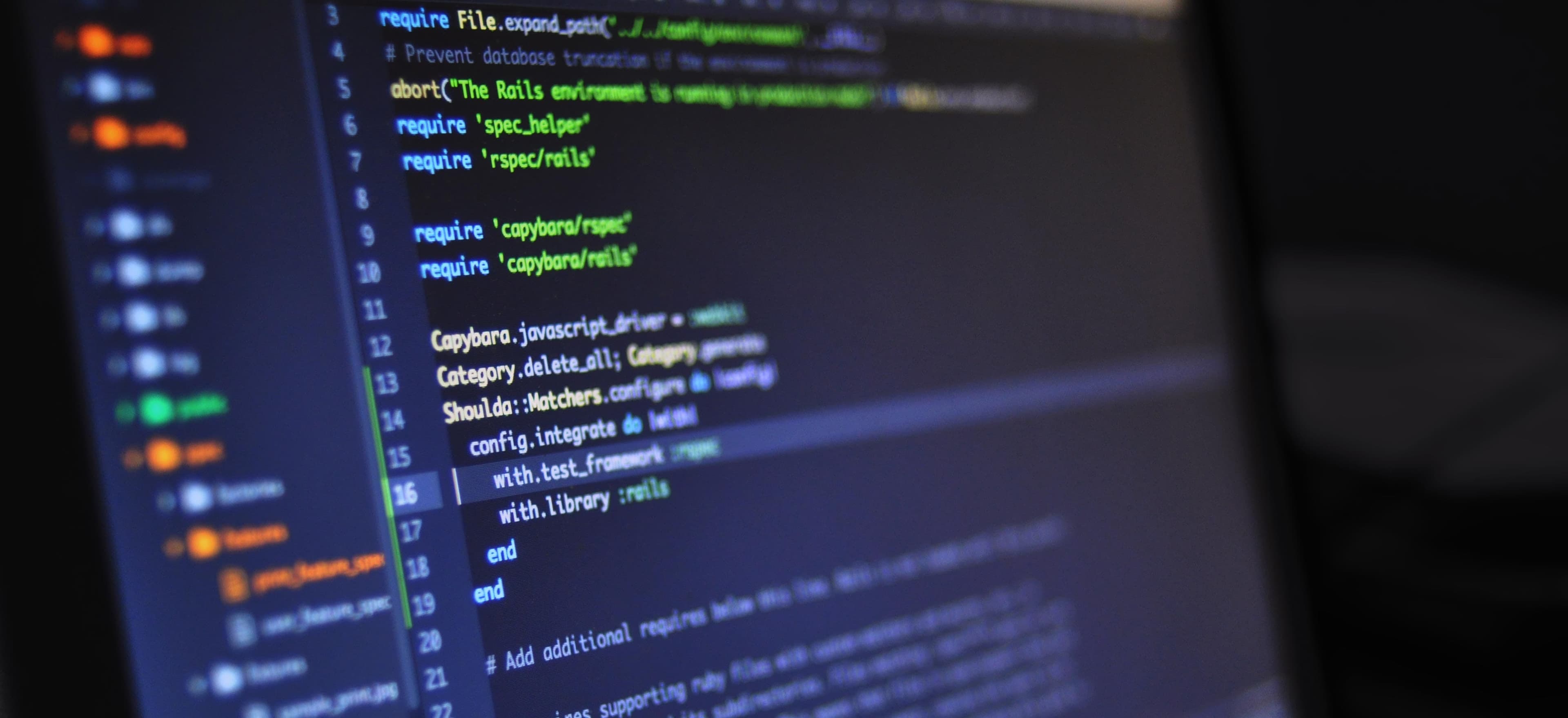
- Published on
Avoiding Common Dependency Injection Pitfalls in Spring
Dependency Injection (DI) is a core principle of the Spring Framework that greatly enhances software maintainability and scalability. However, it comes with its share of challenges. In this blog post, we will explore common pitfalls associated with DI in Spring, provide you with best practices to avoid these issues, and present illustrative code snippets to reinforce our discussion.
Understanding Dependency Injection
Before we dive into typical pitfalls, it's essential to have a firm grasp on what Dependency Injection is and why it's significant in the Spring ecosystem. At its core, DI allows you to inject dependencies into a class rather than having the class create them itself. This leads to higher modularity and easier testing.
Consider the following simple interface and implementation:
public interface GreetingService {
String greet(String name);
}
public class GreetingServiceImpl implements GreetingService {
@Override
public String greet(String name) {
return "Hello, " + name + "!";
}
}
These two classes demonstrate a straightforward dependency structure. Next, we will use DI in a Spring application by integrating this service into a controller.
Common Pitfall #1: Overusing @Autowired
One of the most common pitfalls in Spring's DI is the over-reliance on the @Autowired
annotation. While @Autowired
facilitates automatic injection of dependencies, its indiscriminate use can lead to several problems.
The Problem
Excessive reliance on @Autowired
for field injection can make code harder to test and understand:
@Component
public class UserController {
@Autowired
private GreetingService greetingService;
public String helloUser(String name) {
return greetingService.greet(name);
}
}
The Solution
Constructor injection is often a more sustainable approach. It provides better encapsulation and enables thorough testing:
@Component
public class UserController {
private final GreetingService greetingService;
@Autowired
public UserController(GreetingService greetingService) {
this.greetingService = greetingService;
}
public String helloUser(String name) {
return greetingService.greet(name);
}
}
Why Use Constructor Injection?
- Immutability: The injected service cannot change after the controller is constructed.
- Easier Testing: You can easily substitute mock or stub services when unit testing, leading to better test coverage.
- Clear Dependencies: The constructor clearly lists the required dependencies, making the class easier to understand.
Common Pitfall #2: Creating Cyclic Dependencies
Cyclic dependencies occur when two or more beans depend on each other. This often results in a BeanCurrentlyInCreationException
.
The Problem
Let's say we have two components, ServiceA
and ServiceB
, that reference each other:
@Component
public class ServiceA {
private final ServiceB serviceB;
@Autowired
public ServiceA(ServiceB serviceB) {
this.serviceB = serviceB;
}
}
@Component
public class ServiceB {
private final ServiceA serviceA;
@Autowired
public ServiceB(ServiceA serviceA) {
this.serviceA = serviceA;
}
}
The Solution
To avoid this problem, consider redesigning your architecture to eliminate cyclic dependencies, possibly by introducing an intermediary class or breaking the dependency:
@Component
public class ServiceA {
private final CommonService commonService;
@Autowired
public ServiceA(CommonService commonService) {
this.commonService = commonService;
}
}
@Component
public class ServiceB {
private final CommonService commonService;
@Autowired
public ServiceB(CommonService commonService) {
this.commonService = commonService;
}
}
@Component
public class CommonService {
// Common logic for both services
}
Why Avoid Cyclic Dependencies?
- Simplicity: Your code will be easier to maintain and understand.
- Cleaner Architecture: Clear boundaries between services allow for better separation of concerns.
Common Pitfall #3: Ignoring Scoped Beans
Another common issue developers face is misunderstanding bean scopes in Spring. Each Spring bean has a scope, which defines its lifecycle.
The Problem
If you mistakenly declare a service as a singleton when it should be prototype (e.g., when it maintains mutable state), you might encounter issues:
@Component
@Scope("singleton") // not ideal for a stateful service!
public class StatefulService {
private String state;
public void setState(String state) {
this.state = state;
}
public String getState() {
return state;
}
}
The Solution
In situations where your service has mutable state, ensure you define it as a prototype:
@Component
@Scope("prototype")
public class StatefulService {
private String state;
public void setState(String state) {
this.state = state;
}
public String getState() {
return state;
}
}
Why Is Bean Scope Important?
- Lifecycle Management: Understanding bean scopes allows you to manage the lifecycle of your components better.
- Thread Safety: Properly defining scopes can prevent issues with concurrency, especially in multi-threaded web applications.
Final Considerations
By avoiding these common dependency injection pitfalls, you can enhance the quality and maintainability of your Spring applications. Employing constructor injection, steering clear of cyclic dependencies, and carefully managing bean scopes will result in a more robust application.
For further reading on Spring Dependency Injection, check out Spring Framework Documentation and Spring's Dependency Injection Guide.
In summary, understanding and mastering Dependency Injection in Spring can serve as the foundation for building highly efficient and modular applications. Adopting best practices in DI not only simplifies your code but also fosters better collaboration and development speed in your teams.