Common JDBC Pitfalls and How to Avoid Them
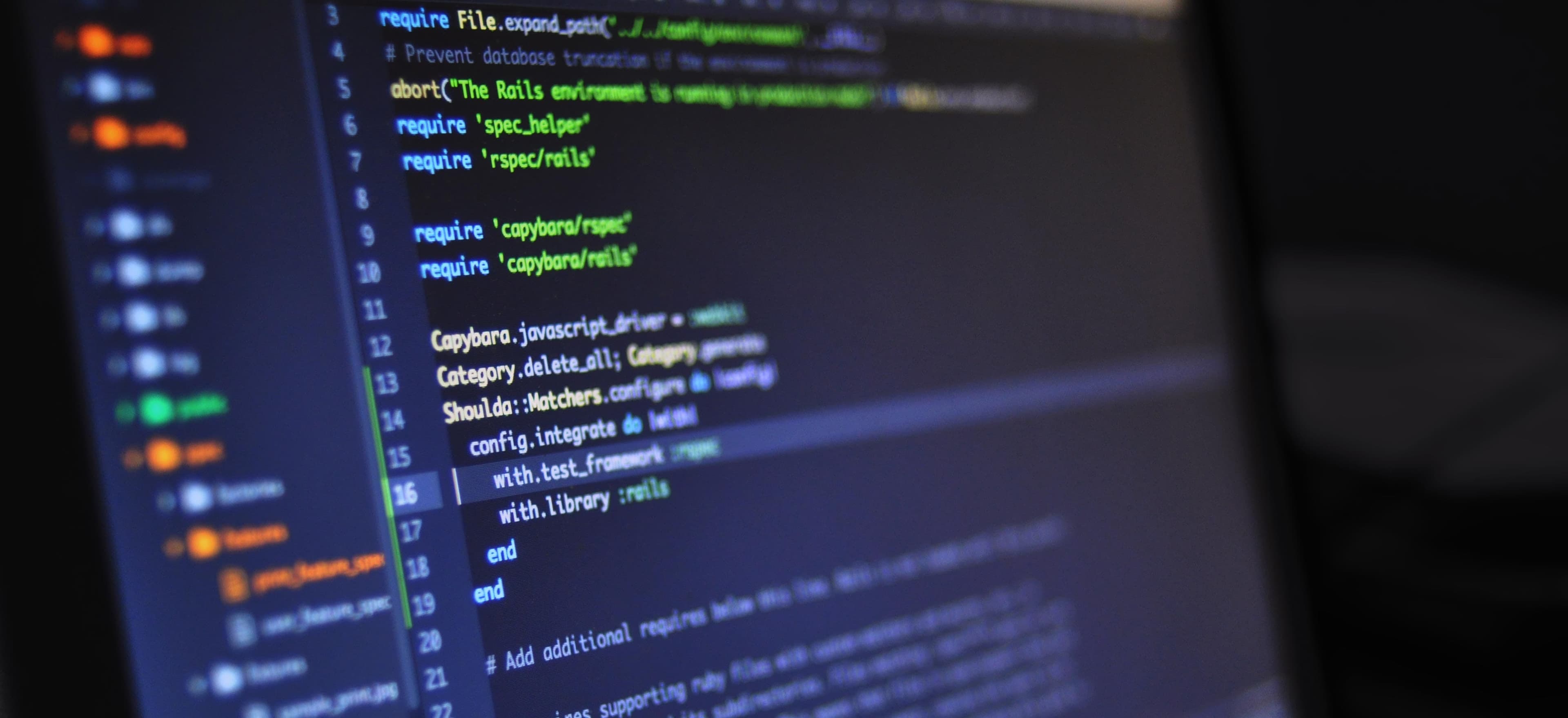
- Published on
Common JDBC Pitfalls and How to Avoid Them
Java Database Connectivity (JDBC) is one of the most fundamental APIs in Java, enabling developers to connect to relational databases from Java applications. Despite its importance, there are several common pitfalls developers encounter when using JDBC. In this blog post, we will discuss these common issues and provide effective strategies to avoid them.
Table of Contents
- Understanding JDBC
- Common Pitfalls
- Lack of Resource Management
- SQL Injection Vulnerabilities
- Not Handling Exceptions Properly
- Inefficient Use of Batch Updates
- Best Practices for JDBC
- Conclusion
Understanding JDBC
JDBC serves as a bridge between Java applications and a wide array of relational databases, such as MySQL, PostgreSQL, Oracle, and others. It provides the ability to execute SQL commands and retrieve results in a structured form that Java can use easily.
The basic steps for using JDBC include:
- Load the JDBC driver.
- Establish a connection to the database.
- Create a statement or prepared statement.
- Execute the statement.
- Process the results.
- Close the connection and statements.
Here’s a simple JDBC connection example:
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.SQLException;
public class JdbcExample {
public static void main(String[] args) {
Connection connection = null;
try {
// Load JDBC Driver
Class.forName("com.mysql.cj.jdbc.Driver");
// Establish the connection
connection = DriverManager.getConnection("jdbc:mysql://localhost:3306/mydatabase", "user", "password");
System.out.println("Connection established successfully!");
} catch (ClassNotFoundException e) {
System.err.println("JDBC Driver not found.");
e.printStackTrace();
} catch (SQLException e) {
System.err.println("Connection failed.");
e.printStackTrace();
} finally {
// Ensure that the connection is closed
if (connection != null) {
try {
connection.close();
} catch (SQLException e) {
e.printStackTrace();
}
}
}
}
}
In this snippet, we demonstrate how to establish a JDBC connection. The JdbcExample
class loads the JDBC driver and attempts to connect to a MySQL database, ensuring that resources are managed adequately.
Common Pitfalls
Despite its relative simplicity, many pitfalls can occur when using JDBC. Let's explore some of the most prevalent issues.
1. Lack of Resource Management
One of the primary pitfalls developers often fall into is neglecting resource management. Failing to close database connections, statements, and result sets can lead to memory leaks and performance bottlenecks.
Solution: Always Use Try-With-Resources
Java’s try-with-resources statement automatically closes resources when they are no longer needed, reducing the risk of leaks.
try (
Connection connection = DriverManager.getConnection("jdbc:mysql://localhost:3306/mydatabase", "user", "password");
PreparedStatement preparedStatement = connection.prepareStatement("SELECT * FROM users WHERE id = ?");
) {
preparedStatement.setInt(1, 1);
ResultSet resultSet = preparedStatement.executeQuery();
while (resultSet.next()) {
System.out.println("User: " + resultSet.getString("name"));
}
} catch (SQLException e) {
e.printStackTrace();
}
In this example, both the Connection
and PreparedStatement
will be automatically closed, minimizing resource leaks.
2. SQL Injection Vulnerabilities
SQL Injection is a serious security vulnerability where an attacker can manipulate SQL queries to gain unauthorized access to sensitive data.
Solution: Use Prepared Statements
Prepared statements prevent SQL injection by separating SQL logic from data, ensuring user inputs do not alter SQL structure.
String userInput = "1 OR 1=1"; // Malicious input
String query = "SELECT * FROM users WHERE id = ?";
try (Connection connection = DriverManager.getConnection("jdbc:mysql://localhost:3306/mydatabase", "user", "password");
PreparedStatement preparedStatement = connection.prepareStatement(query)) {
preparedStatement.setInt(1, Integer.parseInt(userInput));
ResultSet resultSet = preparedStatement.executeQuery();
// Process resultSet
}
Using prepared statements ensures that the user input is treated as data only and cannot compromise SQL logic.
3. Not Handling Exceptions Properly
Another common pitfall is improperly handling exceptions. Ignoring or merely logging exceptions can result in silent failures and make debugging difficult.
Solution: Proper Exception Management
Always provide meaningful logging or error handling. This helps identify problems early and gracefully recover from issues.
try {
// Database operations
} catch (SQLException e) {
System.err.println("Database access error: " + e.getMessage());
// Additional logging or recovery logic here
}
Using appropriate logging frameworks such as SLF4J or Log4j can enhance this process, capturing more detailed error messages.
4. Inefficient Use of Batch Updates
Batch updates can significantly boost performance when inserting, updating, or deleting multiple records. However, failing to leverage them can lead to inefficient database interactions.
Solution: Use Batch Processing
Here's an example of how to implement batch updates effectively:
try (Connection connection = DriverManager.getConnection("jdbc:mysql://localhost:3306/mydatabase", "user", "password");
PreparedStatement preparedStatement = connection.prepareStatement("INSERT INTO users (name, email) VALUES (?, ?)")) {
for (int i = 1; i <= 1000; i++) {
preparedStatement.setString(1, "User" + i);
preparedStatement.setString(2, "user" + i + "@example.com");
preparedStatement.addBatch(); // Add to the batch
// Execute the batch in batchSize intervals
if (i % 100 == 0) {
preparedStatement.executeBatch();
}
}
preparedStatement.executeBatch(); // Execute remaining commands
} catch (SQLException e) {
e.printStackTrace();
}
In this batch processing example, we perform efficient bulk inserts divided into manageable sets, enhancing performance drastically over single inserts.
Best Practices for JDBC
-
Use Connection Pooling: Avoid creating a new connection for every database operation. Use a connection pool (like HikariCP or Apache DBCP) to manage connections efficiently.
-
Implement Logging: Utilize a logging framework to track database interactions. This practice aids in debugging and monitoring database performance.
-
Validate User Input: Always validate and sanitize user inputs before passing them to a SQL query, even when using prepared statements.
-
Limit Database Privileges: Follow the principle of least privilege for database accounts, restricting access to only what is necessary.
-
Optimize Queries: Use indexing and analyze query performance, employing tools like
EXPLAIN
to refine your SQL commands.
For additional context on improving JDBC performance, consider visiting Oracle's JDBC Performance Guidelines.
My Closing Thoughts on the Matter
JDBC is a powerful API that facilitates smooth interactions with relational databases. However, it is essential to be aware of the common pitfalls associated with its use. By employing proper resource management, utilizing prepared statements, handling exceptions correctly, and leveraging batch processing, you can enhance your JDBC practices while ensuring security and performance.
Remember that proper coding habits lay the foundation for efficient database interactions. Keep refining your techniques and stay updated with the advancements in the JDBC landscape to write robust Java applications that engage users in a meaningful way.
If you found this blog post helpful, be sure to share it with your colleagues and enhance your JDBC knowledge. For further reading, you may find the official JDBC documentation to be particularly useful.
Happy coding!