Struggling with HATEOAS Implementation in Spring? Here’s Help!
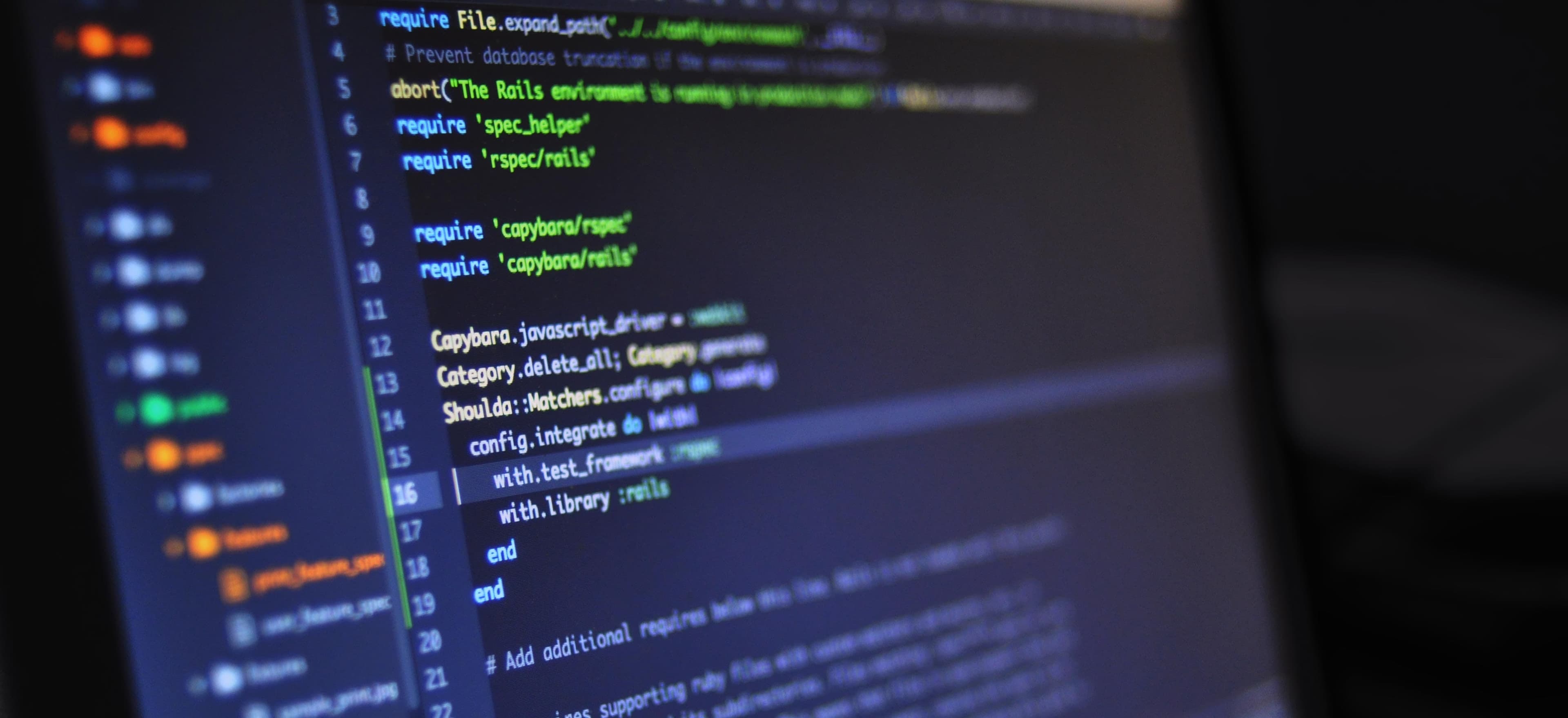
- Published on
Struggling with HATEOAS Implementation in Spring? Here’s Help!
In the world of REST APIs, HATEOAS (Hypermedia as the Engine of Application State) is a standout concept, enabling dynamic interaction between clients and servers. If you are working with Spring Framework and are struggling to implement HATEOAS, you are not alone. This guide will walk you through the principles of HATEOAS, its advantages, and a step-by-step implementation in a Spring application.
What is HATEOAS?
HATEOAS is a constraint of the REST application architecture that allows clients to interact with a web service entirely through hypermedia. In simpler terms, this means that the client can navigate the API by following links provided in the responses rather than needing to hardcode the endpoints.
Key Benefits of HATEOAS:
- Decoupling: It decouples the client from the server by minimizing the need for the client to know full, static URLs.
- Dynamic Discovery: Clients can discover how to interact with the application dynamically.
- Maintainability: Changes to endpoint structures don't necessarily break clients since they rely on hypermedia links.
Getting Started with HATEOAS in Spring
-
Setting Up Your Spring Boot Application
Before implementing HATEOAS, you need a Spring Boot application. You can create a new project using Spring Initializr with the necessary dependencies:
- Spring Web
- Spring HATEOAS
Here’s how your
pom.xml
might look:<dependencies> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-web</artifactId> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-hateoas</artifactId> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-data-jpa</artifactId> </dependency> <dependency> <groupId>org.h2database</groupId> <artifactId>h2</artifactId> <scope>runtime</scope> </dependency> </dependencies>
-
Creating Your Model Class
Let's assume you are building an application to manage books. Here's how you might create a simple
Book
entity:import javax.persistence.Entity; import javax.persistence.GeneratedValue; import javax.persistence.GenerationType; import javax.persistence.Id; @Entity public class Book { @Id @GeneratedValue(strategy = GenerationType.IDENTITY) private Long id; private String title; private String author; // Constructors, getters, and setters }
In this model, we’ve defined a
Book
with attributesid
,title
, andauthor
.
3. Implementing a Repository Interface
You’ll need a repository interface to handle CRUD operations:
import org.springframework.data.jpa.repository.JpaRepository;
public interface BookRepository extends JpaRepository<Book, Long> {
}
The BookRepository
interface extends JpaRepository
, giving you access to methods like save
, findAll
, and more without the need to write boilerplate code.
-
Creating a REST Controller
This is where HATEOAS comes into play. The controller handles client requests and serves the appropriate responses containing links to related resources.
import org.springframework.hateoas.Link; import org.springframework.hateoas.server.mvc.WebMvcLinkBuilder; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.hateoas.EntityModel; import org.springframework.web.bind.annotation.*; import java.util.List; import java.util.stream.Collectors; @RestController @RequestMapping("/books") public class BookController { @Autowired private BookRepository bookRepository; @GetMapping public List<EntityModel<Book>> all() { return bookRepository.findAll().stream() .map(this::toEntityModel) .collect(Collectors.toList()); } @GetMapping("/{id}") public EntityModel<Book> one(@PathVariable Long id) { Book book = bookRepository.findById(id) .orElseThrow(() -> new ResourceNotFoundException(id)); return toEntityModel(book); } private EntityModel<Book> toEntityModel(Book book) { EntityModel<Book> resource = EntityModel.of(book); Link selfLink = WebMvcLinkBuilder.linkTo(WebMvcLinkBuilder.methodOn(BookController.class).one(book.getId())).withSelfRel(); resource.add(selfLink); return resource; } } class ResourceNotFoundException extends RuntimeException { ResourceNotFoundException(Long id) { super("Could not find book " + id); } }
In this example, we have defined two endpoints:
GET /books
: This retrieves a list of all books and converts each to anEntityModel
, which includes hypermedia links.GET /books/{id}
: This retrieves a specific book by its ID.
Detailed Explanation
- The
EntityModel<Book>
represents the resources, including links to related resources. - The
toEntityModel
private method creates these models and adds a self-link for the book resource. - Using
WebMvcLinkBuilder
, we create a self-referencing link that conforms to the HATEOAS principles.
-
Testing Your API
With your REST controller in place, you can test your API using tools like Postman or CURL. When you access
GET /books
, your response should look something like this:[ { "content": { "id": 1, "title": "1984", "author": "George Orwell" }, "_links": { "self": { "href": "http://localhost:8080/books/1" } } } ]
This clearly shows the representation of the book along with a hypermedia link to access it directly.
6. Additional HATEOAS links
As your application grows, consider adding more links for related resources. For instance, if you have an Author
entity, you might want to provide additional links to the author’s details.
Benefits of a HATEOAS-Compliant API
- Auto-discovery: Clients can programmatically navigate through your API without needing to know the specifics.
- Clearer API shape: Dependencies and relationships among resources are clear via links.
- Easier client-side code: The client can dynamically discover related resources without tightly coupling with the server.
Bringing It All Together
Implementing HATEOAS in a Spring Boot application is not as daunting as it seems. By following the outlined steps, you're on your way to creating a more flexible, maintainable, and user-friendly REST API.
For further reading, consult the official Spring HATEOAS documentation and explore additional patterns and examples of how to enhance your API’s functionality.
With HATEOAS, your API can evolve without needing to overhaul client implementations — it’s the future of RESTful services!
Happy coding!