Common Pitfalls When Writing Maven Plugins and How to Avoid Them
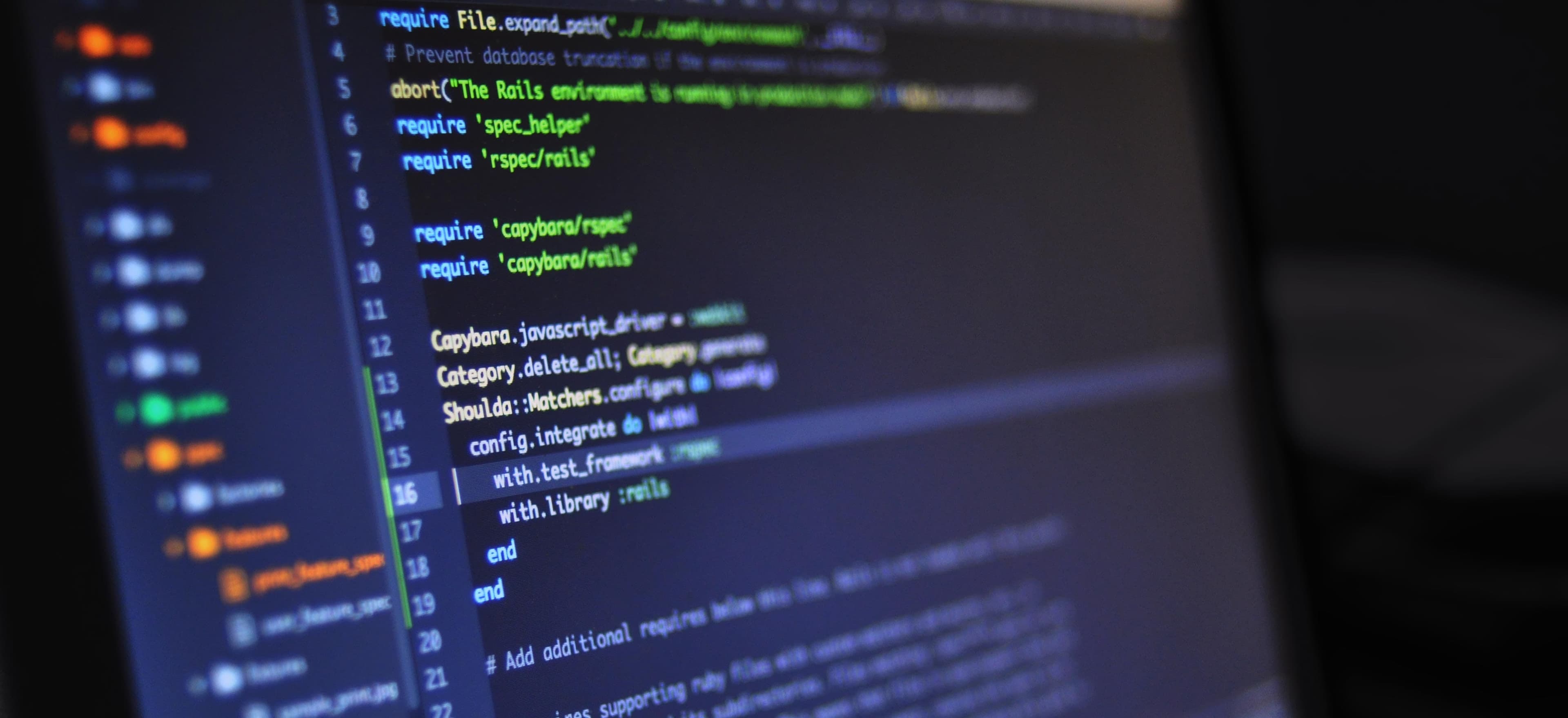
- Published on
Common Pitfalls When Writing Maven Plugins and How to Avoid Them
Maven is a powerful build automation tool used primarily for Java projects. One of its strengths lies in its extensibility through plugins. However, writing a Maven plugin can be a challenging endeavor. In this post, we'll delve into common pitfalls associated with developing Maven plugins and how to sidestep these issues effectively.
What Are Maven Plugins?
Maven plugins are reusable pieces of software that provide functionalities to build, test, and deploy Java applications. They can automate a range of tasks, from compiling code to generating documentation. The official documentation can be a valuable resource for anyone looking to delve deeper.
While creating a Maven plugin, it's crucial to understand both the architecture and the lifecycle of Maven itself. So let's examine some common pitfalls to avoid when developing your own Maven plugin.
1. Ignoring the Maven Life Cycle
The Importance of the Lifecycle
Maven operates on a carefully structured lifecycle that defines the phases of building and managing a project. Ignoring these phases can lead to unpredictable behavior and ultimately cause your plugin to fail unexpectedly.
Solution
Familiarize yourself with the Maven lifecycle phases, such as validate
, compile
, test
, package
, install
, and deploy
.
For instance, if you want your plugin to perform operations during the compile phase, it should be bound to the compile
lifecycle phase:
<plugin>
<groupId>com.example</groupId>
<artifactId>my-plugin</artifactId>
<version>1.0-SNAPSHOT</version>
<executions>
<execution>
<goals>
<goal>myGoal</goal>
</goals>
<phase>compile</phase> <!-- Binding to the compile phase -->
</execution>
</executions>
</plugin>
By correctly associating your plugin with the appropriate lifecycle phase, you can ensure that it operates as intended, maintaining the flow of the build process.
2. Not Handling Plugin Dependencies Properly
The Dependency Dilemma
Every plugin may have its own dependencies, which may lead to version conflicts or runtime failures if not managed correctly.
Solution
Define your dependencies in the plugin.xml
and ensure you use the specified versions. Always prefer using scope
attributes effectively to avoid unwanted inclusion in projects:
<dependencies>
<dependency>
<groupId>org.apache.commons</groupId>
<artifactId>commons-lang3</artifactId>
<version>3.12.0</version>
<scope>compile</scope> <!-- Defines when this dependency is needed -->
</dependency>
</dependencies>
For more information on handling dependencies, check out Maven’s dependency management.
3. Poorly Implemented Logging
Logging Isn't Optional
Logging is essential for debugging and providing users with feedback. Inadequate or missing logs can leave users of your plugin in the dark.
Solution
Maven plugins should utilize the SLF4J logging framework. When logging, ensure to include various levels (e.g., debug, info, warn, error) to give users granular control over what they want to see.
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
public class MyMojo extends AbstractMojo {
private static final Logger log = LoggerFactory.getLogger(MyMojo.class);
public void execute() {
log.info("Starting the plugin execution...");
// Main logic here
log.debug("This is a debug message to help with troubleshooting.");
}
}
With clear logs, users can easily trace the plugin's behavior, making your plugin more user-friendly and maintainable.
4. Not Validating Input Parameters
Input Matters
Users often provide parameters that your plugin will use. Neglecting to validate these inputs can result in runtime errors or unintended behavior.
Solution
Validate inputs as soon as your plugin starts executing. If a user provides incorrect input, throw a clear exception:
@Parameter(property = "inputParam", required = true)
private String inputParam;
public void execute() throws MojoExecutionException {
if (inputParam == null || inputParam.isEmpty()) {
throw new MojoExecutionException("inputParam is required and cannot be empty.");
}
// Proceed with logic
}
Having robust input validation leads to fewer runtime errors and provides a better user experience.
5. Neglecting Unit Tests
The Testing Trap
All too often, plugin developers skip writing unit tests due to time constraints. However, this leads to an unreliable product.
Solution
Maven provides excellent support for unit testing through frameworks like JUnit. Here’s a simple testing approach:
import static org.junit.Assert.*;
import org.junit.Test;
public class MyMojoTest {
@Test
public void testExecute() {
MyMojo mojo = new MyMojo();
mojo.setInputParam("test");
try {
mojo.execute(); // Assuming execute would set a status
assertEquals("expectedResult", mojo.getResult());
} catch (MojoExecutionException e) {
fail("Execution failed: " + e.getMessage());
}
}
}
By integrating unit tests into your workflow, you’ll capture bugs early, saving time and resources in the long run.
6. Lacking Comprehensive Documentation
Documentation Matters
Documentation is often an afterthought for developers, but it is critical for any tool. Users need to understand how to integrate and use your plugin effectively.
Solution
Create comprehensive documentation, including installation instructions, usage examples, and explanations of parameters. Given that plugins are often reused across multiple projects, detailed documentation can significantly enhance user experience.
Consider maintaining a wiki or a GitHub page for your plugin. Use Markdown for formatting to make it easier for users to read and comprehend.
Final Considerations
Developing a Maven plugin can be a rewarding, yet challenging experience. By avoiding the common pitfalls outlined in this post, you can create plugins that are both functional and user-friendly. From understanding the Maven lifecycle to implementing robust input validation and testing, each step you take contributes to the overall quality of your plugin.
Remember, taking the time to write unit tests, create thorough documentation, and manage dependencies effectively will pay off dramatically in the long run. Happy coding!
For further in-depth reading on this topic, consider checking the Maven Plugin Development Guide for additional insights and strategies.