Overcoming Common Challenges in Apache Lucene 4.3 Configuration
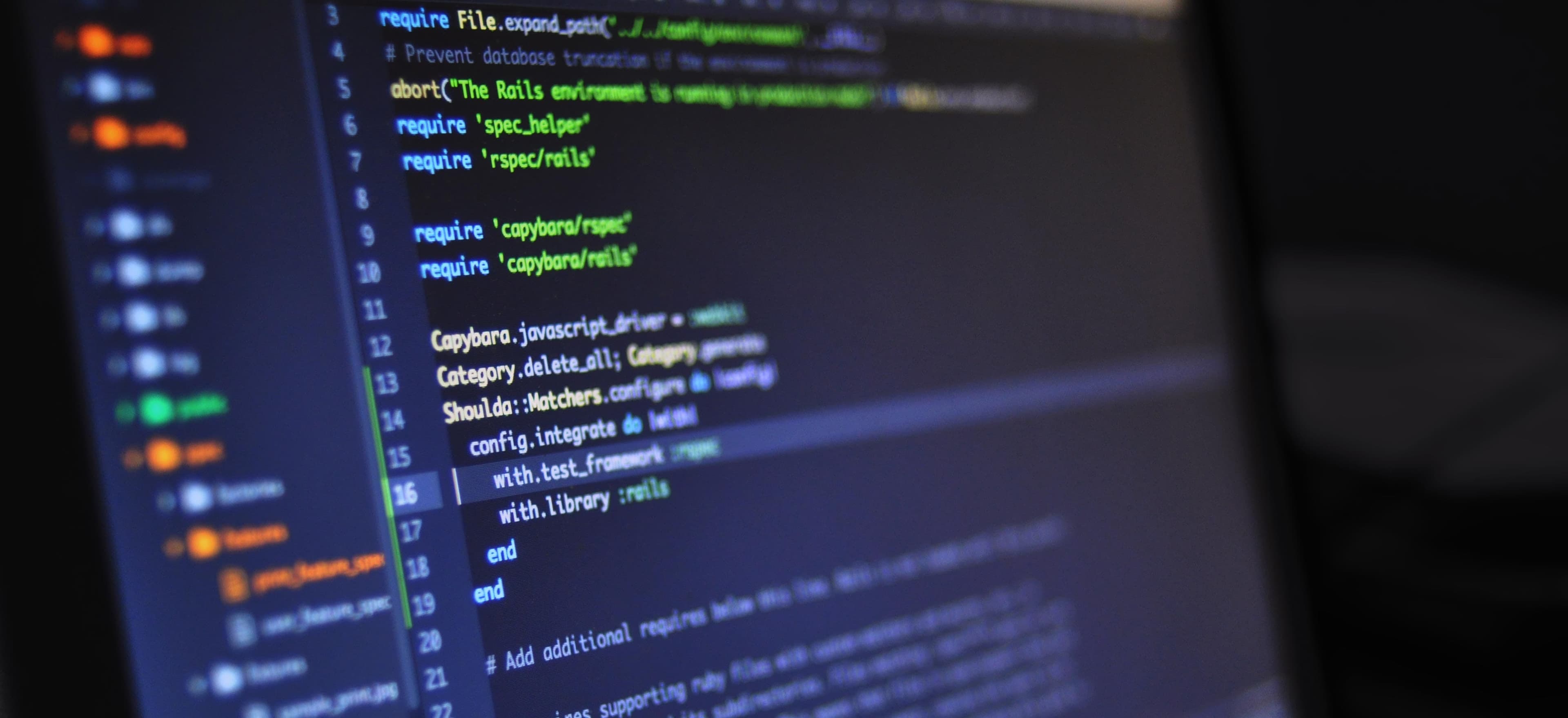
- Published on
Overcoming Common Challenges in Apache Lucene 4.3 Configuration
Apache Lucene is a powerful, high-performance search engine library written in Java. It provides a framework for indexing and searching text efficiently, which makes it popular for applications that require full-text search capabilities. However, configuring Lucene can be a daunting task, especially for beginners. In this blog post, we will address common challenges faced during the configuration of Apache Lucene 4.3, offering practical solutions and best practices.
Understanding Apache Lucene
Before diving into specific configuration challenges, it’s essential to understand Apache Lucene and how it works. At its core, Lucene allows you to create a searchable index from your documents, enhancing performance and retrieval speed. This involves several stages, including document parsing, tokenization, indexing, and searching.
To get started, make sure to include Lucene in your project. If using Maven, you can add Lucene 4.3 to your pom.xml
:
<dependency>
<groupId>org.apache.lucene</groupId>
<artifactId>lucene-core</artifactId>
<version>4.3.0</version>
</dependency>
Common Challenges and Solutions
1. Dependency Issues
Many newcomers to Lucene may face challenges with dependencies. Given that Lucene depends on multiple libraries, it’s crucial to ensure all required dependencies are correctly configured and compatible.
Solution: Use a build tool like Maven or Gradle to handle dependencies automatically. Maven simplifies dependency management with its pom.xml
. For Gradle, include the dependencies in your build.gradle
file:
dependencies {
implementation 'org.apache.lucene:lucene-core:4.3.0'
}
This automatically resolves dependencies, mitigating potential issues with mismatched versions.
Why? Automated dependency management ensures all components work together harmoniously, letting you focus on implementing functionality rather than troubleshooting conflicts.
2. Understanding Indexing Process
The indexing process can be ambiguous for beginners. Properly indexing your documents is crucial to leverage the full power of Lucene. The indexing process includes adding documents to your index, which involves creating a Document
object.
Example: Creating and Indexing a Document
import org.apache.lucene.document.Document;
import org.apache.lucene.document.Field;
import org.apache.lucene.document.FieldType;
import org.apache.lucene.index.IndexWriter;
import org.apache.lucene.index.IndexWriterConfig;
public void indexDocument(IndexWriter writer, String title, String content) throws IOException {
Document doc = new Document();
Field titleField = new Field("title", title, Field.Store.YES, Field.Index.ANALYZED);
Field contentField = new Field("content", content, Field.Store.YES, Field.Index.ANALYZED);
doc.add(titleField);
doc.add(contentField);
writer.addDocument(doc);
}
Why? In this example, the title
and content
fields are being indexed with specific configurations. The Field.Store.YES
indicates that these fields should be stored and retrievable, while Field.Index.ANALYZED
means the text will be tokenized for better search.
3. Handling Analyzers
Analyzers play a crucial role in how text is processed in Lucene. Choosing the right analyzer can significantly impact search results and performance. Lucene offers various built-in analyzers, such as StandardAnalyzer
, WhitespaceAnalyzer
, and KeywordAnalyzer
.
Solution: Start with StandardAnalyzer
for general-purpose text. It tokenizes and normalizes text efficiently, accommodating a wide range of use cases.
import org.apache.lucene.analysis.standard.StandardAnalyzer;
StandardAnalyzer analyzer = new StandardAnalyzer();
Why? Using the StandardAnalyzer
simplifies the process, allowing for effective tokenization without the need for extensive configuration. As you become more familiar with Lucene's functionality, you can explore custom analyzers tailored to your specific needs.
4. Query Syntax and Parsing
Lucene’s search capabilities are powerful, but they can be complex when it comes to query syntax. The challenge often lies in crafting effective queries to utilize the index.
Solution: Use the QueryParser
to create queries from user input:
import org.apache.lucene.queryparser.classic.QueryParser;
import org.apache.lucene.search.Query;
QueryParser parser = new QueryParser("content", analyzer);
Query query = parser.parse("your search text");
Why? The QueryParser
streamlines query creation and helps prevent syntax-related issues by interpreting the input intelligently before searching the index. Additionally, it allows for complex queries like Boolean queries.
5. Pagination and Sorting Results
When dealing with large datasets, effectively paginating and sorting search results is vital. Early stages of development may overlook this aspect, leading to performance bottlenecks.
Solution: Use TopDocs
and ScoreDoc
for pagination:
import org.apache.lucene.search.IndexSearcher;
import org.apache.lucene.search.TopDocs;
TopDocs results = searcher.search(query, 10);
By specifying the number of results
returned, you avoid overwhelming users with too much information at once.
Why? This promotes a better user experience, making it easier for users to digest results. You can then implement paging logic to allow navigation through larger datasets.
6. Performance Tuning
Improving index performance is crucial, especially for large datasets. Among the common performance pitfalls is the choice of RAMDirectory
versus FSDirectory
for storage.
Solution: For smaller datasets or development environments, RAMDirectory
offers speed. However, for production systems handling large volumes of data, FSDirectory
via a file path is usually the better choice.
import org.apache.lucene.store.FSDirectory;
FSDirectory fsDirectory = FSDirectory.open(Paths.get("/path/to/index"));
Why? FSDirectory
provides durability and allows for more extensive data storage beyond memory constraints.
Closing the Chapter
Configuring Apache Lucene 4.3 can be challenging, but by understanding common issues and employing strategic solutions, you can streamline your development process. From handling dependencies to managing analyzers and queries, every aspect plays a crucial role in leveraging Lucene’s capabilities.
If you are interested in further exploring Lucene’s functionalities, consider checking out the official Apache Lucene Documentation for deeper insights. Additionally, for advanced use cases, you can refer to Lucene in Action, which covers strategies for scaling search application deployments.
To make the most out of Apache Lucene, keep learning and iterating on your setup. The more you understand its capabilities, the better you can tailor it for your projects. Happy coding!