Mastering Cassandra: Demystifying Primary Key Design
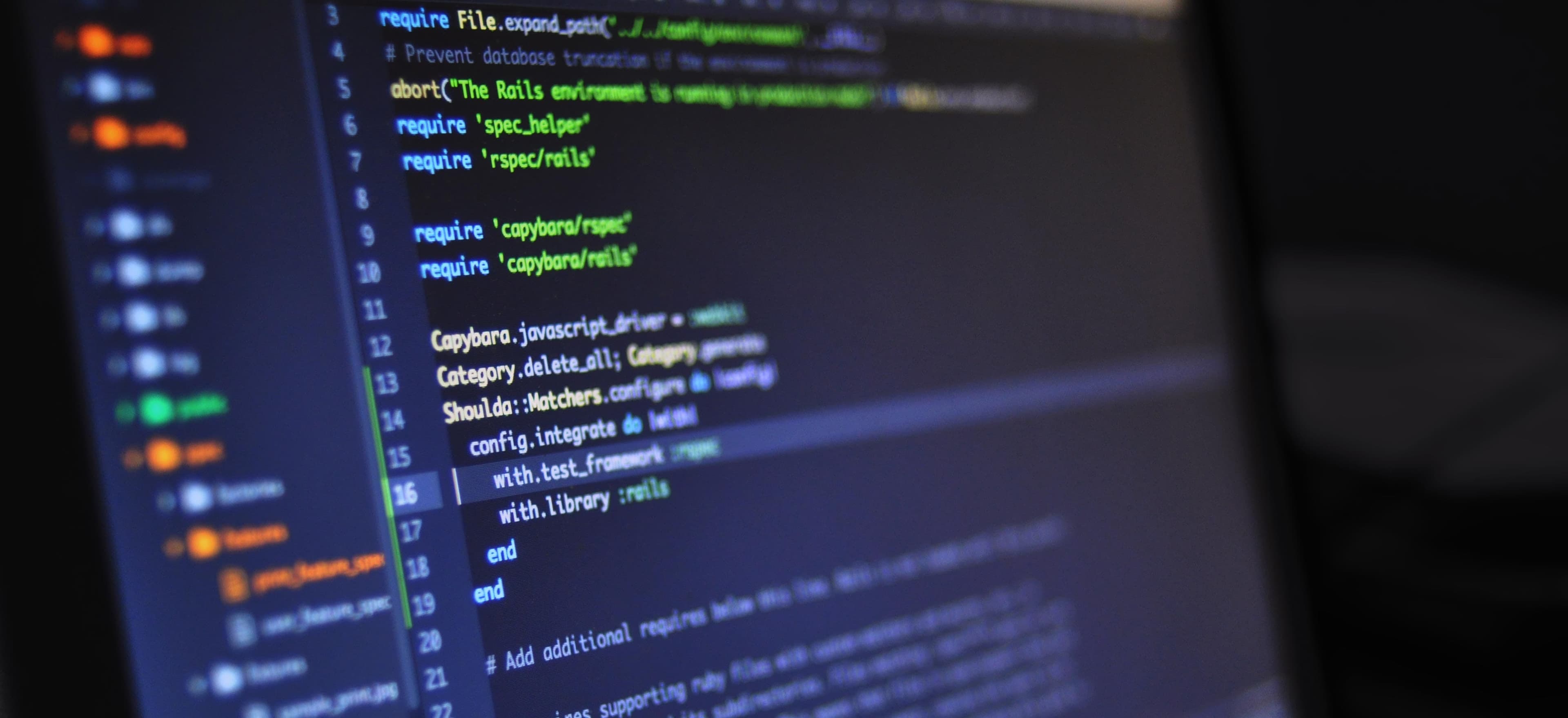
- Published on
Mastering Cassandra: Demystifying Primary Key Design
Apache Cassandra is a powerful distributed NoSQL database known for its high availability and scalability. When designing applications that leverage Cassandra, one of the most critical decisions you'll make is how to define your primary keys. A well-thought-out primary key design can significantly impact your application's performance, data retrieval efficiency, and overall architecture.
In this blog post, we will explore the intricacies of primary key design in Cassandra, discuss primary key composition, and provide effective strategies to ensure optimal database performance.
Understanding Primary Keys in Cassandra
In relational databases, primary keys are straightforward identifiers for records. However, in Cassandra, the concept of primary keys encompasses both a partition key and clustering columns.
Partition Key
The partition key determines which node stores your data. A well-distributed partition key ensures balanced data across the cluster and aids in efficient data retrieval.
Clustering Columns
Clustering columns, on the other hand, determine how data is ordered within a partition. They allow the retrieval of rows in a specific sequence, optimizing query performance significantly.
The Anatomy of a Primary Key
A primary key in Cassandra consists of two parts:
- Partition Key: Defines the partition of the data.
- Clustering Columns: Defines the sort order of the data within that partition.
Example:
CREATE TABLE user_activity (
user_id UUID,
activity_time TIMESTAMP,
activity_type TEXT,
PRIMARY KEY (user_id, activity_time)
);
In this example:
user_id
is the partition key.activity_time
is the clustering column.
Why This Primary Key Design?
Choosing user_id
as the partition key ensures all activities related to a specific user are stored in one partition. Adding activity_time
as a clustering column allows activities to be retrieved in chronological order.
Best Practices for Primary Key Design
Designing a primary key requires strategic thinking. Here are some best practices to follow:
1. Choose a Good Partition Key
A good partition key should ensure an even distribution of data across your nodes. The following factors can help you choose your partition key:
- Cardinality: A partition key should have high cardinality. For instance, using an
entity_id
oruser_id
makes sense if you have a large number of unique users. - Usage Patterns: Consider how you plan to query your data. If you regularly fetch data by a particular attribute, it’s wise to use that attribute as a partition key.
2. Avoid Hot Partitions
Hot partitions occur when one partition contains significantly more data than others. This can lead to performance bottlenecks. A diverse partition key, combined with high cardinality, can mitigate this risk.
3. Optimize Clustering Columns
Use clustering columns to refine your data retrieval.
Example:
CREATE TABLE blog_posts (
author_id UUID,
post_date TIMESTAMP,
post_id UUID,
content TEXT,
PRIMARY KEY (author_id, post_date, post_id)
);
In this table:
author_id
is the partition key.post_date
is the first clustering column, followed bypost_id
for unique ordering within each date.
This design allows retrieving all posts by an author efficiently and facilitates chronological sorting.
4. Think Ahead
It's essential to anticipate your system’s evolving needs. A primary key design that works today might not hold up in the future. Always design with future growth in mind. Consider query patterns that could emerge as usage grows.
5. Keep It Simple
While complex key designs might seem appealing, they can lead to complications. Keeping keys as simple as possible will save you from headaches later.
Query-driven Design
Cassandra is designed for fast writes and reads, but you need to consider your query patterns at the outset. The primary key design should be query-driven, focusing on anticipated use cases.
Example of Query-Driven Design
Suppose you need to support queries that filter on multiple attributes efficiently:
CREATE TABLE event_logs (
event_type TEXT,
user_id UUID,
timestamp TIMESTAMP,
event_data TEXT,
PRIMARY KEY ((event_type), user_id, timestamp)
);
Here, you have a composite primary key:
- The partition key is
event_type
, which allows separating different event categories efficiently. user_id
andtimestamp
as clustering columns allow sorting logs for each event type by user and time.
With this design, you can quickly retrieve event logs for a specific user type or time range.
Handling All Queries
Cassandra does not support joins like traditional relational databases. However, you can design your keys to ensure that you accommodate multiple access patterns without the need for complex relationships.
Example of Supporting Various Access Patterns
Consider a retail application:
CREATE TABLE order_history (
user_id UUID,
order_id UUID,
product_id UUID,
order_date TIMESTAMP,
quantity INT,
PRIMARY KEY ((user_id), order_date, order_id)
);
In this case:
- You can retrieve all orders for a user and sort them by the order date efficiently.
- This structure allows you to query orders in reverse chronological order simply by reversing the
order_date
clustering column.
Performance Considerations
Performance tuning in Cassandra heavily relies on your primary key design. Here are some aspects to consider:
- Read and Write Efficiency: Your read and write operations should be efficient based on your key structure. Avoid unnecessary complexity.
- Consistency: Depending on read consistency settings, poorly chosen keys can affect your data availability and consistency.
To Wrap Things Up
Mastering primary key design in Cassandra is akin to constructing a strong foundation. A deep understanding of how partition keys and clustering columns function together will enable you to build a database architecture that performs robustly under load while providing the fast access that users expect.
Being mindful of your data distribution, query patterns, and potential future needs will not only enhance performance but also set you up for success as your application expands.
For more on Cassandra architecture and design principles, you might find these resources helpful:
- Apache Cassandra Documentation
- Cassandra Data Modeling Best Practices
By following this guide, you're on your way to mastering Cassandra and unlocking its full potential. Happy coding!
Checkout our other articles