Mastering Command Pattern: Solving Complex User Actions
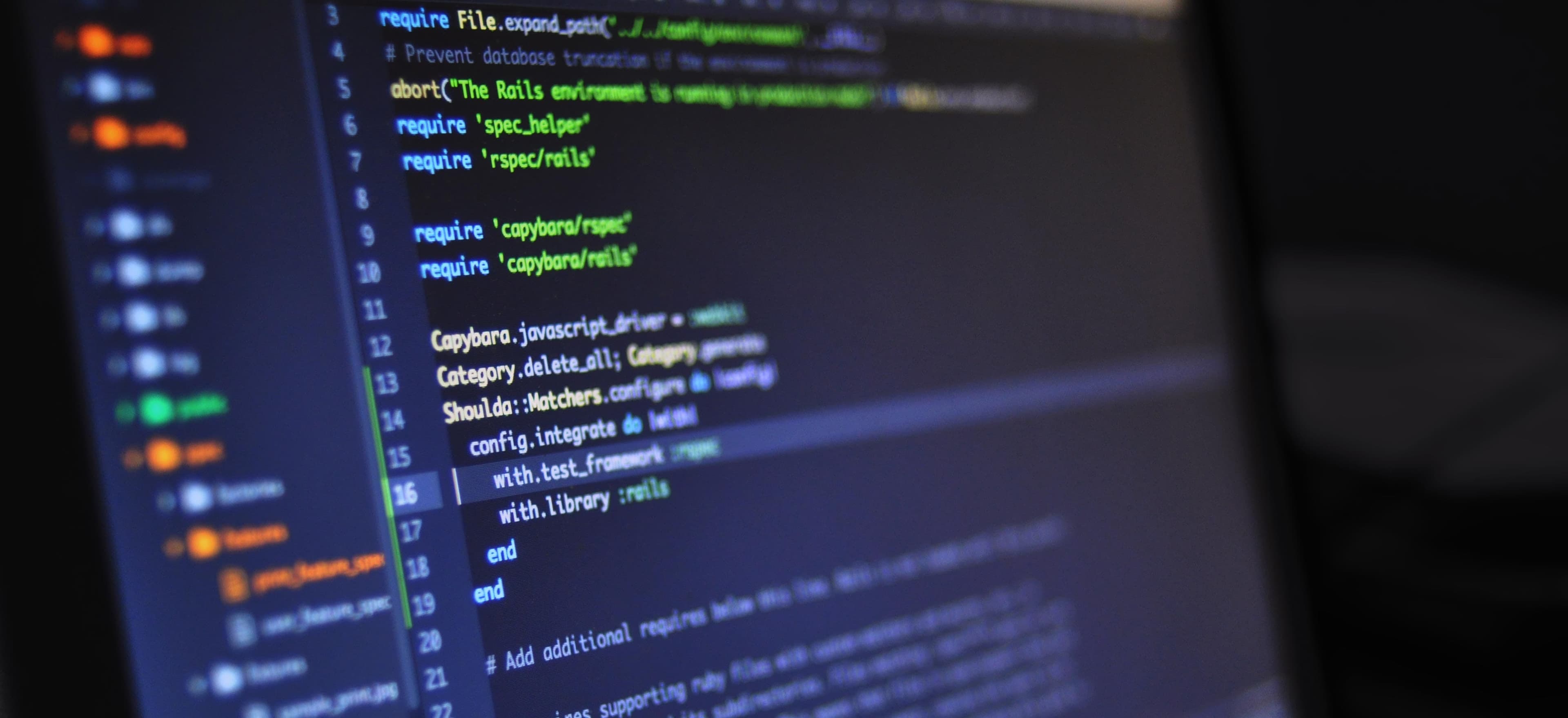
- Published on
Mastering the Command Pattern: Solving Complex User Actions in Java
In the realm of software design, patterns are essential tools that help developers manage complexity. The Command Pattern is one such pattern that shines when controlling user actions in a seamless and organized manner. This post will delve into the intricacies of the Command Pattern, providing clear explanations, concise code snippets, and practical applications.
What is the Command Pattern?
The Command Pattern is a behavioral design pattern that encapsulates a request as an object. This allows you to parameterize clients with queues, requests, or operations, and delay the execution of a command.
Key Components of the Command Pattern:
- Command Interface: Defines a method for executing operations.
- Concrete Command: Implements the Command interface and defines the binding between a receiver and an action.
- Receiver: The object that knows how to perform the operations.
- Invoker: Asks the command to execute the request.
- Client: Creates a Concrete Command and associates it with a receiver.
Why Use the Command Pattern?
The Command Pattern offers multiple benefits that simplify code and enhance functionality:
- Decoupling: The invoker and receiver are decoupled, leading to less interdependency in your code.
- Undo/Redo Functionality: You can easily implement undo/redo operations by keeping a history of commands.
- Parameterization of Operations: Commands can be stored, passed around, and passed to other functions as first-class objects.
- Support for Queuing: It allows operations to be queued, making it simple to handle complex user actions that require management.
Implementation of Command Pattern in Java
Let's illustrate how the Command Pattern can be implemented in Java using a simple example: a text editor where a user can execute commands like typing and deleting.
Step 1: Define the Command Interface
The first step is to create a Command interface that will define a single method, execute
.
public interface Command {
void execute();
}
Step 2: Create Concrete Commands
Next, we’ll create two concrete commands: TypeCommand
and DeleteCommand
. Each will interact with a TextEditor
receiver.
// Receiver
public class TextEditor {
private StringBuilder content = new StringBuilder();
public void type(String text) {
content.append(text);
System.out.println("Typed: " + text);
}
public void delete(int length) {
if (length > content.length()) {
length = content.length();
}
String removed = content.substring(content.length() - length);
content.delete(content.length() - length, content.length());
System.out.println("Deleted: " + removed);
}
public String getContent() {
return content.toString();
}
}
// Concrete Command for typing
public class TypeCommand implements Command {
private TextEditor editor;
private String text;
public TypeCommand(TextEditor editor, String text) {
this.editor = editor;
this.text = text;
}
@Override
public void execute() {
editor.type(text);
}
}
// Concrete Command for deleting
public class DeleteCommand implements Command {
private TextEditor editor;
private int length;
public DeleteCommand(TextEditor editor, int length) {
this.editor = editor;
this.length = length;
}
@Override
public void execute() {
editor.delete(length);
}
}
Step 3: Create the Invoker
The Invoker
is responsible for invoking commands. It can keep a history of commands for potential undo operations.
import java.util.ArrayList;
import java.util.List;
public class CommandInvoker {
private List<Command> commandHistory = new ArrayList<>();
public void executeCommand(Command command) {
command.execute();
commandHistory.add(command);
}
public void showContent(TextEditor editor) {
System.out.println("Current Content: " + editor.getContent());
}
}
Step 4: Use the Command Pattern
Finally, we will create a client that will use the Command
, Invoker
, and Receiver
.
public class Client {
public static void main(String[] args) {
TextEditor editor = new TextEditor();
CommandInvoker invoker = new CommandInvoker();
Command typeHello = new TypeCommand(editor, "Hello");
Command typeWorld = new TypeCommand(editor, " World");
Command deleteCommand = new DeleteCommand(editor, 6);
invoker.executeCommand(typeHello);
invoker.showContent(editor); // Outputs: "Current Content: Hello"
invoker.executeCommand(typeWorld);
invoker.showContent(editor); // Outputs: "Current Content: Hello World"
invoker.executeCommand(deleteCommand);
invoker.showContent(editor); // Outputs: "Current Content: Hello"
}
}
Explanation of the Code
In the client code:
- TextEditor is instantiated to manage our text content.
- CommandInvoker is used to execute commands and show the current content.
- Two types of commands (
TypeCommand
andDeleteCommand
) are created to type text and delete text, respectively. - We invoke commands to observe the changes in the
TextEditor
.
Benefits in Action
This implementation provides a clear structure. If you want to add an undo feature, you can simply modify CommandInvoker
to store a history of commands to reverse operations. It also facilitates testing since commands can be tested independently.
Real-World Applications of the Command Pattern
The Command Pattern is widely used in various applications:
- GUI Toolkits: For button clicks and menu item selections, where different actions are performed based on the command.
- Remote Controls: Where commands can be sent to various devices, allowing for a clean and intuitive interaction model.
- Transactional Systems: To execute systems that require a clear commit/rollback model.
My Closing Thoughts on the Matter
Mastering the Command Pattern can significantly simplify the management of user actions in your Java applications. Through its encapsulation of commands, it promotes cleaner, more maintainable code, while providing powerful features such as undo and queuing. Whether you're building a text editor, a remote control system, or a dynamic GUI toolkit, the Command Pattern can be an invaluable part of your design toolkit.
For further reading on design patterns in Java, check out Refactoring Guru or the official Java documentation.
By understanding and applying the Command Pattern, you can enhance your programming skills and build more robust, user-friendly applications. Happy coding!
Checkout our other articles