Solving Common Issues with Spring 4.2.2 Event Listeners
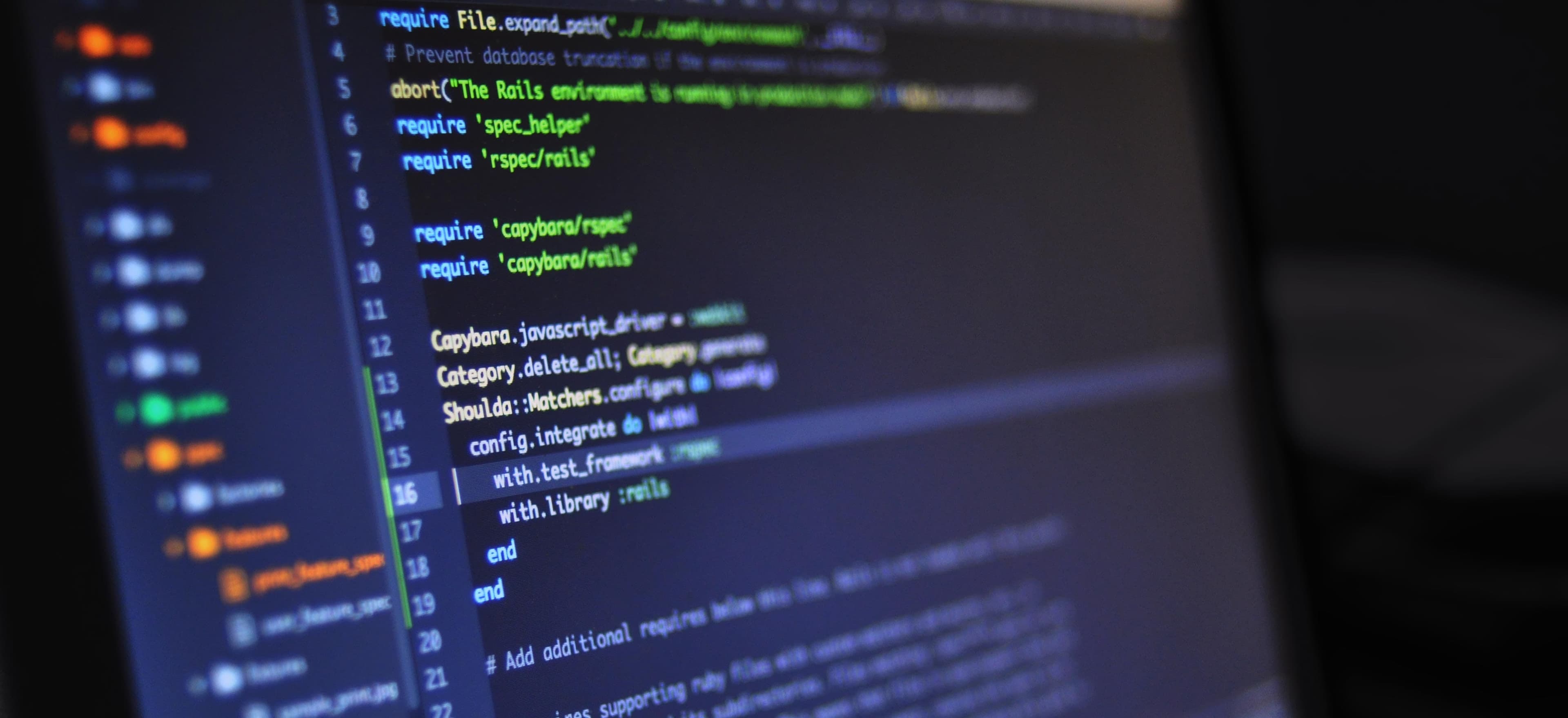
- Published on
Solving Common Issues with Spring 4.2.2 Event Listeners
In the world of enterprise applications, event-driven architecture has gained prominence due to its robustness and ability to decouple components. Spring, a popular Java framework, provides an extensive event handling mechanism that simplifies this approach. In this blog, we will explore the intricacies of Spring 4.2.2 event listeners, addressing common issues developers encounter and offering solutions.
Understanding Spring's Event Publishing
Before diving into common issues and solutions, let's clarify how event publishing works in Spring. Spring's event model relies on a publishing mechanism where events are sent and received through listeners.
Key Concepts
- ApplicationEvent: The base class for all application events in Spring.
- ApplicationListener: An interface that must be implemented to define a listener.
- ApplicationEventPublisher: A core interface used to publish events.
Basic Example
To understand how to set up an event listener, consider this basic structure:
import org.springframework.context.ApplicationEvent;
import org.springframework.context.ApplicationListener;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.context.event.EventListener;
@Configuration
public class AppConfig {
@Bean
public EventPublisher eventPublisher() {
return new EventPublisher();
}
}
This configuration initializes the necessary beans. Now let’s define an event and a listener.
Defining an Event
public class CustomEvent extends ApplicationEvent {
private String message;
public CustomEvent(Object source, String message) {
super(source);
this.message = message;
}
public String getMessage() {
return message;
}
}
In this snippet, CustomEvent
extends ApplicationEvent
and holds a specific message.
Creating a Listener
public class CustomEventListener implements ApplicationListener<CustomEvent> {
@Override
public void onApplicationEvent(CustomEvent event) {
System.out.println("Event received: " + event.getMessage());
}
}
Here, the CustomEventListener
implements ApplicationListener
and overrides the onApplicationEvent
method, providing a response to the event it listens to.
Publishing Events
To publish an event, we can do so with the ApplicationEventPublisher
:
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.context.ApplicationEventPublisher;
public class EventPublisher {
@Autowired
private ApplicationEventPublisher publisher;
public void publish(String message) {
CustomEvent event = new CustomEvent(this, message);
publisher.publishEvent(event);
}
}
This simple method lets you send events from anywhere in your Spring application.
Common Issues
While using Spring's event listener model, developers often encounter several common issues. Let’s discuss these problems and the remedies that can be applied.
Issue 1: Events Not Received
Problem: You publish an event, but the listeners do not seem to respond.
Solution
-
Check Listener Registration: Ensure listeners are correctly registered in your Spring context.
@Bean public CustomEventListener customEventListener() { return new CustomEventListener(); }
-
Spring Container: Verify that your event publisher and listener are within the same Spring application context.
-
Context Hierarchy: If you're using multiple contexts, ensure your event publisher can see the listener.
Issue 2: Event Handling Order
Problem: Events are being handled in an unexpected order.
Solution
You can control the order of event listeners by implementing the Ordered
interface, where lower values have higher priority:
import org.springframework.core.Ordered;
public class FirstListener implements ApplicationListener<CustomEvent>, Ordered {
@Override
public void onApplicationEvent(CustomEvent event) {
System.out.println("First Listener: " + event.getMessage());
}
@Override
public int getOrder() {
return 1;
}
}
public class SecondListener implements ApplicationListener<CustomEvent>, Ordered {
@Override
public void onApplicationEvent(CustomEvent event) {
System.out.println("Second Listener: " + event.getMessage());
}
@Override
public int getOrder() {
return 2;
}
}
Here, FirstListener
will always execute before SecondListener
.
Issue 3: Asynchronous Execution
Problem: Your listener is blocking the event publishing thread.
Solution
For non-blocking behavior, you can make your event listener asynchronous using the @Async
annotation:
import org.springframework.scheduling.annotation.Async;
import org.springframework.stereotype.Component;
@Component
public class AsyncEventListener {
@Async
@EventListener
public void handleAsyncEvent(CustomEvent event) {
// simulate processing
System.out.println("Async Event received: " + event.getMessage());
}
}
Make sure to enable asynchronous support in your configuration:
@EnableAsync
@Configuration
public class AppConfig {
// other beans
}
Issue 4: Memory Leaks
Problem: Long-lived listeners can inadvertently create memory leaks.
Solution
A good rule of thumb is to avoid overly long-lived listeners in your application. Prefer stateless listeners, or manage listener lifecycles effectively through context scoping.
Issue 5: Event Type Confusion
Problem: Multiple events might be handled in a single listener leading to type confusion.
Solution
Consider creating specific listeners for different event types or implement generic event handling logic with type checks.
import org.springframework.context.event.EventListener;
public class GenericEventListener {
@EventListener
public void handleEvent(ApplicationEvent event) {
if (event instanceof CustomEvent) {
System.out.println("Handle CustomEvent");
} else {
// handle other events
}
}
}
The Closing Argument
Spring 4.2.2 event listeners provide a powerful mechanism to decouple components and improve the structure of your application. However, with great power comes great responsibility. Understanding common pitfalls ensures you leverage the framework effectively.
For further reading, explore the Spring Framework Documentation or check out the community discussions on Stack Overflow.
By experimenting and applying the solutions presented in this blog, you can build responsive, efficient applications that fully employ the capabilities of Spring's event-driven architecture. Happy coding!
Checkout our other articles