Writing Code for Maintainability: Avoiding Developer Frustration
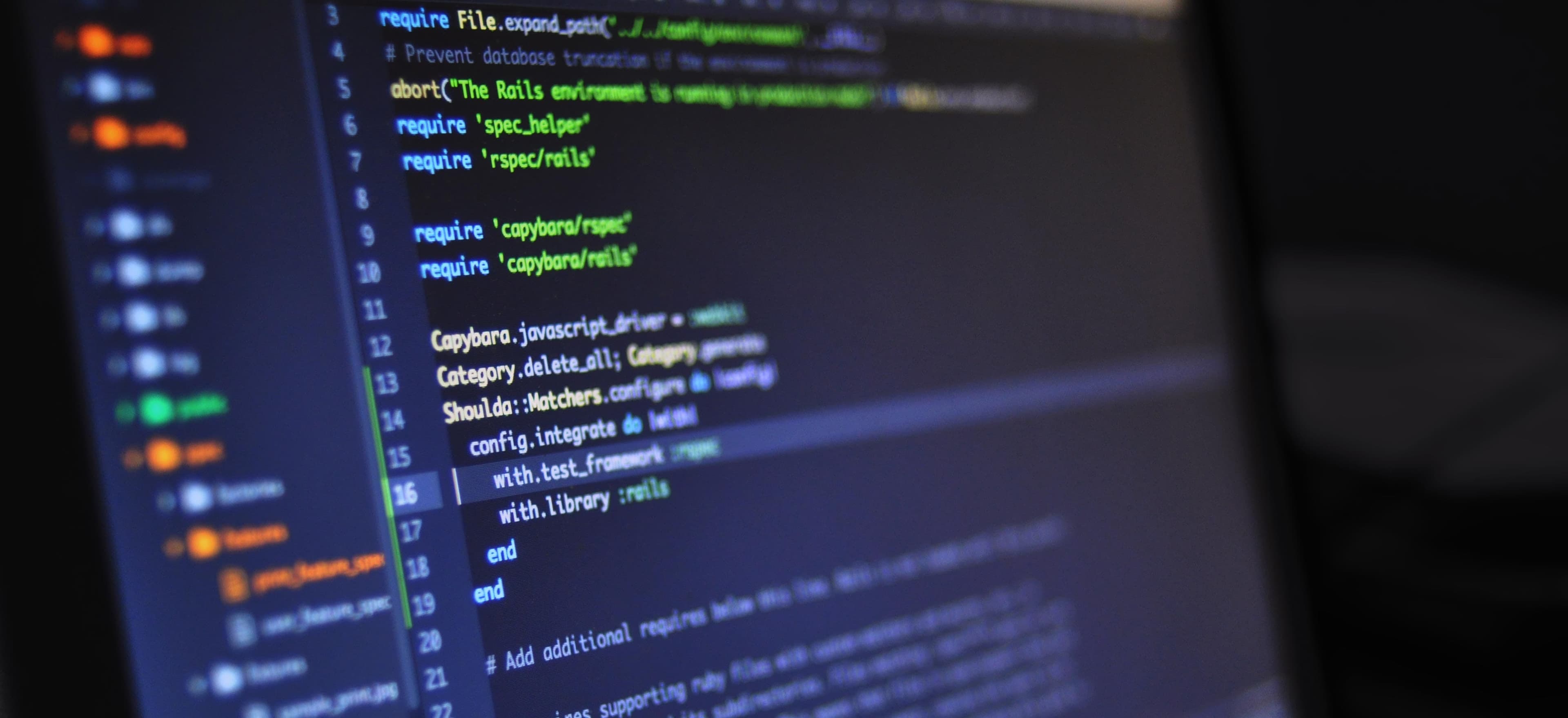
- Published on
Writing Code for Maintainability: Avoiding Developer Frustration
In today's fast-paced software development landscape, maintainability is often the Unsung Hero of successful projects. As developers, we spend a significant portion of our time revisiting and modifying existing code. If that code is poorly written, misunderstood, or overly complex, it can lead to frustration, bugs, and even project failure. This blog post will dive deep into the principles of writing maintainable Java code while providing clear examples and actionable insights.
What is Maintainability?
Maintainability refers to the ease with which code can be updated, modified, tested, and fixed. It encompasses several attributes:
- Readability: How easily can someone else (or you, after some time) read the code?
- Modularity: The degree to which code components can be separated and recombined.
- Testability: How easily can the code be tested?
- Scalability: The ability of the code to adapt to increased loads or new requirements without major rewrites.
The Importance of Maintainability
Here are a few reasons why maintainability should be at the forefront of your coding practices:
- Time is Money: Reduce the time taken to read, understand, and fix code.
- Collaboration is Key: Teams grow and change; maintainable code makes onboarding newcomers easier.
- Business Value: Longer-lasting software reduces operational costs and increases value delivery.
Principles of Maintainable Code
1. Naming Conventions
Naming conventions are vital in establishing clarity. When variables, methods, and classes are named appropriately, the intent of the code becomes obvious.
Example
// Poor naming
int a = 5;
// Good naming
int numberOfUsers = 5;
Why: The second example clearly communicates what the variable represents, making it easier to understand the purpose of the code at a glance.
2. DRY Principle (Don't Repeat Yourself)
While it might seem easier to copy and paste code during development, this leads to increased effort during maintenance.
Example
// Bad: Duplicate Code
public void calculateAreaOfCircle(float radius) {
System.out.println(3.14 * radius * radius);
}
public void calculateAreaOfRectangle(float width, float height) {
System.out.println(width * height);
}
// Good: Reusing Code
public void calculateAreaOfCircle(float radius) {
System.out.println(calculateAreaOfCircle(radius));
}
public double calculateAreaOfCircle(float radius) {
return 3.14 * radius * radius;
}
public double calculateAreaOfRectangle(float width, float height) {
return width * height;
}
Why: The good example abstracts the area calculation into methods that can be reused, meaning any changes can be done in one place.
3. Commenting Wisely
Code comments are incredibly useful when done right, but excessive or vague comments can be counterproductive. Strive for clarity in your code first and use comments to explain complex behavior.
Example
// Bad: Unhelpful comment
// This function does something
public void processUserData() {
// Process User Data
}
// Good: Meaningful comments
/**
* Processes user data and saves it to the database.
*
* @param userData The user data to be processed and saved.
*/
public void processUserData(String userData) {
// Convert userData to the required format
}
Why: The second example offers clear, informative context that assists any developer reviewing the code without requiring them to figure out what "something" means.
4. Modular Programming
Breaking your code into modules makes it easier to test and maintain. By encapsulating functionality, you create components that can be reused and altered independently.
Example
// Monolithic code
public class UserService {
public void registerUser(String userData) {
// Validation
if (userData == null) {
throw new IllegalArgumentException("User data can't be null");
}
// Logic to register user
// Logic to send confirmation email
}
}
// Modular code
public class UserService {
private final UserValidator userValidator;
private final EmailService emailService;
public UserService(UserValidator userValidator, EmailService emailService) {
this.userValidator = userValidator;
this.emailService = emailService;
}
public void registerUser(String userData) {
userValidator.validate(userData);
// Logic to register user
emailService.sendConfirmationEmail();
}
}
Why: The modular approach increases clarity and tests efficiency; you can test UserValidator
and EmailService
independently from the UserService
.
5. Consistent Indentation and Formatting
Consistency in code formatting promotes readability. Adopting a common code style reduces cognitive load on developers.
Tools to Use
- Google Java Style Guide: A widely accepted standard.
- CheckStyle: A development tool to help programmers write Java code that adheres to a coding standard.
6. Use of Design Patterns
Familiarizing yourself with design patterns can help you address common problems with reliable solutions. Be mindful, though, to adopt them where applicable, not just for the sake of it.
Example: Singleton Pattern
public class ConfigurationManager {
private static ConfigurationManager instance;
private ConfigurationManager() {
// Initialization code
}
public static ConfigurationManager getInstance() {
if (instance == null) {
instance = new ConfigurationManager();
}
return instance;
}
}
Why: This pattern ensures that only one instance of ConfigurationManager
exists, simplifying configuration management and improving maintainability.
Testing for Maintainability
Testing is integral to maintainability. With adequate unit tests, developers can make changes with confidence.
- Unit Testing: Write tests for individual units of code.
- Integration Testing: Assess how different modules interact.
- Automated Testing Tools: Leverage frameworks like JUnit or TestNG.
Sample Unit Test with JUnit
import static org.junit.jupiter.api.Assertions.assertEquals;
public class UserServiceTest {
private UserService userService;
@BeforeEach
public void setUp() {
userService = new UserService(new UserValidator(), new EmailService());
}
@Test
public void testRegisterUser() {
String userData = "validUserData";
userService.registerUser(userData);
// Add assertions to verify expected behavior
// assertEquals(expectedValue, actualValue);
}
}
Why: Unit tests facilitate rapid feedback on changes, fostering a culture of continuous improvement.
Closing the Chapter
In software development, maintainability is not just a nice-to-have; it's an essential quality that can drastically reduce both the time and frustration associated with managing code. By adopting best practices, including meaningful naming conventions, modular programming, proper commenting, and thorough testing, you can create a foundation upon which teams can build high-quality software.
Further Reading and Resources
- Clean Code: A Handbook of Agile Software Craftsmanship
- Design Patterns: Elements of Reusable Object-Oriented Software
- JUnit 5 User Guide
With these principles in mind, you can contribute to a culture of maintainability in your development environment, ensuring fewer headaches for you and your team in the long run. Happy coding!