Why Java Updates Are Crucial for Your Development Success
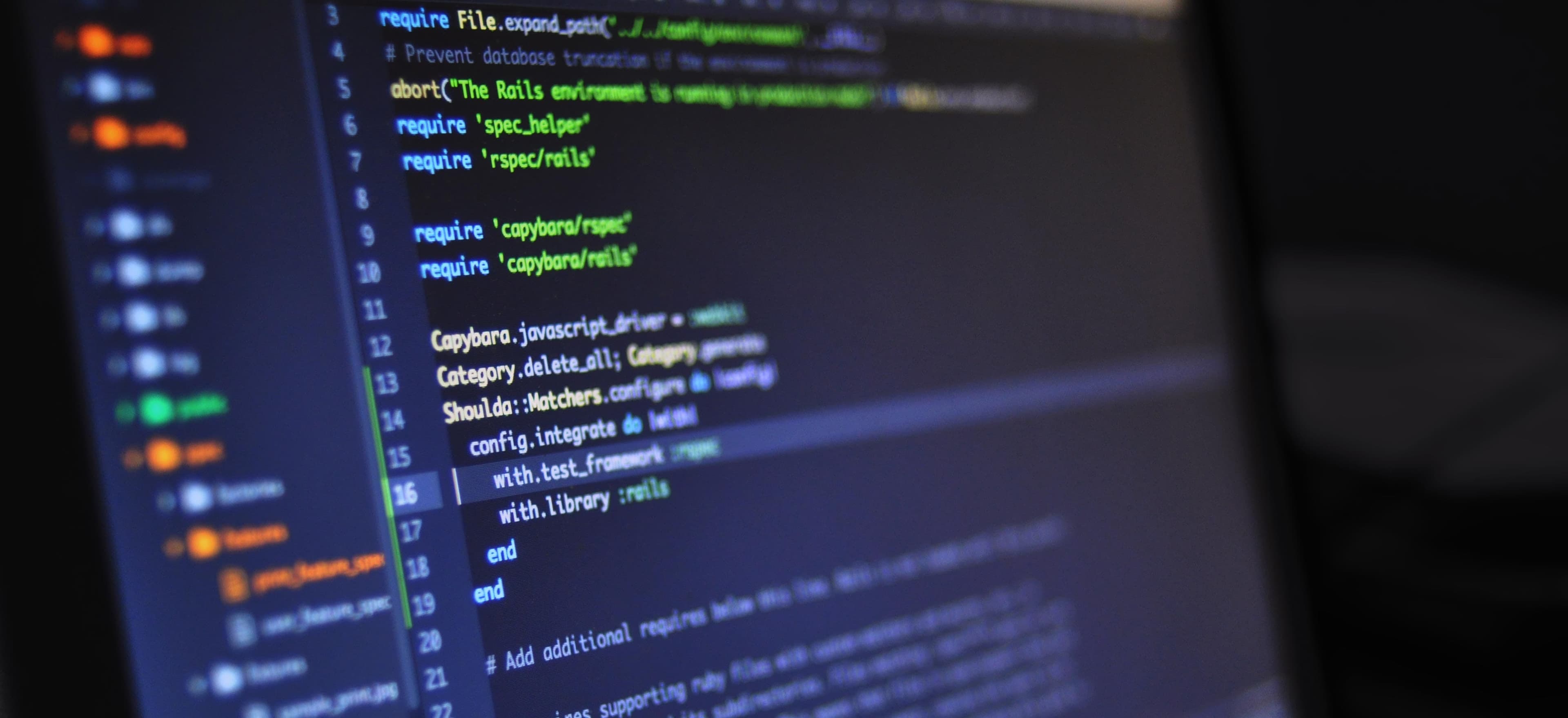
- Published on
Why Java Updates Are Crucial for Your Development Success
Java has been a staple in the world of programming since its inception in 1995. With its robust architecture, platform independence, and rich ecosystem, Java is the go-to language for many developers and enterprises. However, to stay relevant and competitive in the ever-evolving tech landscape, it is vital to keep up with Java updates. In this blog post, we will delve into the significance of these updates, exploring their benefits, and demonstrating some key features through practical examples.
The Importance of Java Updates
1. Security Enhancements
One of the most compelling reasons to keep your Java environment updated is to maintain security. Cybersecurity threats are continuously evolving, and so are the updates that aim to patch vulnerabilities. Outdated versions of Java may expose your applications to risks, making them susceptible to attacks.
Example:
public class SecurityExample {
// Use SecureRandom for better security
public static void main(String[] args) {
java.security.SecureRandom random = new java.security.SecureRandom();
byte[] bytes = new byte[20];
random.nextBytes(bytes);
System.out.println("Secure Random Bytes: " + java.util.Base64.getEncoder().encodeToString(bytes));
}
}
In this example, we are using SecureRandom
instead of Random
. The SecureRandom
class was introduced to generate cryptographically strong random numbers, ensuring higher security for sensitive data handling.
2. Performance Improvements
Java updates often come with performance enhancements. These refinements can lead to optimized memory management, faster execution times, and overall better efficiency of Java applications. Ignoring updates could mean running your applications using outdated performance standards.
Example:
public class PerformanceExample {
// Using streams introduced in Java 8 for better performance
public static void main(String[] args) {
java.util.List<String> names = java.util.Arrays.asList("Alice", "Bob", "Charlie", "David");
// Use of streams for filtering
names.stream()
.filter(name -> name.startsWith("A"))
.forEach(System.out::println);
}
}
The Stream API introduced in Java 8 allows for more expressive and efficient data manipulation. This leads to clearer code and can help in optimizing performance by leveraging parallel processing where applicable.
3. Enhanced Features and APIs
Each Java update introduces new features and APIs that can simplify coding practices and reduce development time. From adding new language constructs to enhancing existing libraries, these updates provide developers with powerful tools at their disposal.
Example of New API:
import java.time.LocalDate;
public class DateTimeExample {
public static void main(String[] args) {
// Using the new Date-Time API introduced in Java 8
LocalDate today = LocalDate.now();
System.out.println("Today's date is: " + today);
}
}
The Java Date-Time API, introduced in Java 8, has greatly simplified date and time manipulations. Unlike the older java.util.Date
and java.util.Calendar
, the newer API allows for a more intuitive and error-free date/time handling.
4. Community Support and Compatibility
Staying updated with the latest Java versions ensures compatibility with newer frameworks, libraries, and tools. The Java community is vibrant and continuously evolving. Keeping in pace with the community means benefiting from collective knowledge and support.
For instance, many frameworks, such as Spring and JavaFX, regularly update their versions and may drop support for earlier versions of Java. Hence, if you want to leverage the latest features of these frameworks or receive community support, updating Java is essential.
5. Improved Developer Productivity
Java updates often include tools aimed at making developers' lives easier. New features in integrated development environments (IDEs), better debugging tools, and simplified syntax can all boost productivity.
Example:
public class LambdaExample {
public static void main(String[] args) {
// Using lambda expressions introduced in Java 8
java.util.List<String> names = java.util.ArrayList.of("Alice", "Bob", "Charlie", "David");
// ForEach with lambda for cleaner syntax
names.forEach(name -> System.out.println("Name: " + name));
}
}
In this snippet, lambda expressions simplify the iteration process significantly. They allow developers to write clearer and more concise code, thus increasing productivity.
Key Takeaways
As we’ve explored throughout this post, Java updates bring a multitude of benefits, ranging from security enhancements to increased developer productivity. Neglecting these updates can lead to vulnerabilities, reduced performance, and incompatibility with modern tools and frameworks.
Ensuring that you keep your Java environment updated not only helps you leverage new features but also improves your code quality and the overall success of your development projects. Embrace the changes that come with each update, as they ultimately empower developers to create better, safer, and more efficient applications.
Keep Learning and Adapting
Staying informed about Java updates is crucial for career growth. Follow blogs, participate in community forums, and engage with other developers to exchange knowledge and stay ahead.
For more information on the latest updates, you can visit the official Java website for detailed release notes and resources.
Happy coding!