Mastering Primitive Streams in Java 8: Common Pitfalls
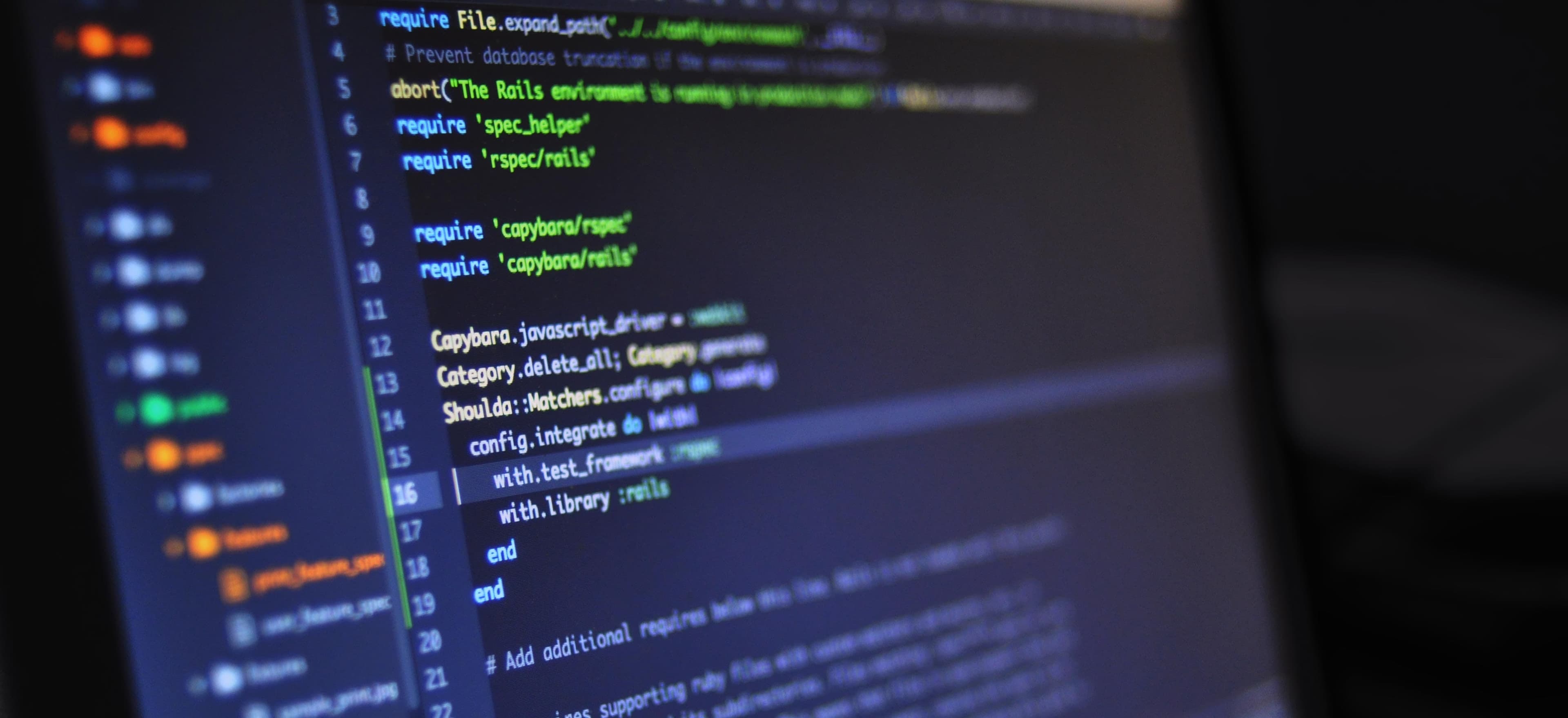
- Published on
Mastering Primitive Streams in Java 8: Common Pitfalls
Java 8 introduced a plethora of features that revolutionized the way developers handle data manipulation. One of the highlights is the Stream API, which provides a high-level abstraction for processing sequences of elements. Among different stream types, primitive streams cater specifically to the three primitive data types: int, long, and double. While they efficiently handle large datasets, they come with their own set of common pitfalls that can lead to ineffective programming practices.
In this blog post, we will explore primitive streams in Java 8, their advantages, and the common pitfalls that developers encounter. We will also provide illustrative code snippets to clarify these concepts.
What are Primitive Streams?
Primitive streams in Java come in three flavors:
IntStream
for handlingint
data typesLongStream
forlong
data typesDoubleStream
fordouble
data types
These streams are optimized for operations that involve primitives, eliminating the overhead of boxing and unboxing associated with their corresponding wrapper classes.
Example of Creating a Primitive Stream
The following is a simple example of creating an IntStream
:
import java.util.stream.IntStream;
public class PrimitiveStreamExample {
public static void main(String[] args) {
IntStream intStream = IntStream.range(1, 10);
// Print the squares of the numbers in the stream
intStream.map(num -> num * num)
.forEach(System.out::println);
}
}
In this example, IntStream.range(1, 10)
creates a stream of integers from 1 to 9 (the ending value is exclusive). The map
operation square each number, and forEach
outputs the results.
Benefits of Using Primitive Streams
Using primitive streams can significantly boost performance, especially when processing large datasets. Here are some reasons:
- Reduced Memory Overhead: Primitive streams avoid the boxing associated with object streams, leading to reduced memory usage.
- Enhanced Performance: Operations on primitive types are generally faster than their boxed counterparts.
- Simplicity: The API provides a fluent interface that simplifies data processing.
Common Pitfalls with Primitive Streams
While primitive streams offer numerous benefits, they can also introduce challenges if not used appropriately. Here are some common pitfalls developers should avoid:
1. Confusing Primitive and Object Streams
One of the most significant pitfalls is mixing primitive streams with object streams. This often leads to unexpected behaviors.
Example
import java.util.Arrays;
import java.util.List;
import java.util.stream.Collectors;
public class MixedStreamExample {
public static void main(String[] args) {
List<Integer> integers = Arrays.asList(1, 2, 3, 4);
// This will cause boxing and may lead to performance issues
List<Integer> squared = integers.stream()
.map(num -> num * num)
.collect(Collectors.toList());
// This works with IntStream, avoiding boxing
List<Integer> squaredPrimitive = integers.stream()
.mapToInt(num -> num * num)
.boxed()
.collect(Collectors.toList());
}
}
In this example, the first approach creates unnecessary boxes of integers, while the second approach leverages mapToInt
, which processes integers directly.
2. Ignoring Stream Pipeline Short-Circuiting
Primitive streams support short-circuiting operations like findFirst
and anyMatch
. Failing to utilize these can lead to inefficiencies.
Example
import java.util.stream.IntStream;
public class StreamShortCircuitExample {
public static void main(String[] args) {
boolean exists = IntStream.range(1, 100)
.anyMatch(num -> num % 50 == 0); // Short-circuits
System.out.println("Exists: " + exists);
}
}
Using anyMatch
returns immediately once it finds a matching element, making it more efficient than processing all elements.
3. Overlooking Empty Streams
Creating operations on an empty stream can lead to unexpected results. For example, attempting to perform reduction operations will return defaults without any indication of error.
Example
import java.util.OptionalDouble;
import java.util.stream.DoubleStream;
public class EmptyStreamExample {
public static void main(String[] args) {
OptionalDouble average = DoubleStream.empty().average(); // Returns an empty Optional
if (average.isPresent()) {
System.out.println("Average: " + average.getAsDouble());
} else {
System.out.println("No elements in the stream.");
}
}
}
Always check for presence using methods like isPresent()
when working with optional return values.
4. Unnecessary Boxing and Unboxing
Completing operations on a boxed primitive (like Integer
or Double
) can lead to inefficiencies due to repeated boxing and unboxing during processing.
Example
import java.util.Arrays;
import java.util.List;
public class BoxingUnboxingExample {
public static void main(String[] args) {
List<Integer> nums = Arrays.asList(1, 2, 3, 4);
// Inefficient: creates boxes for each number
int sum = nums.stream()
.mapToInt(Integer::intValue)
.sum(); // Minimal boxing here, but can still be avoided
System.out.println("Sum: " + sum);
}
}
In this example, even though mapToInt
reduces boxing, the use of a boxed list at the start may still introduce some overhead. Instead, working with primitive arrays when possible can improve efficiency.
5. Failing to Optimize Performance
While streams can be highly optimized for working with large datasets, accidental usage patterns can drastically degrade performance.
Example
import java.util.stream.LongStream;
public class PerformanceExample {
public static void main(String[] args) {
long total = LongStream.range(1, 1_000_000) // Performance tip: Use parallel streams carefully
.parallel()
.filter(num -> num % 2 == 0)
.count();
System.out.println("Total even numbers: " + total);
}
}
Using parallel streams indiscriminately can lead to performance degradation when the task is not CPU-intensive, or when there are frequent context switches.
Wrapping Up
Mastering primitive streams in Java 8 can enhance performance and improve how data is processed in your applications. However, it’s essential to be aware of the potential pitfalls that can limit the effectiveness of your code. By understanding the intricacies of primitive streams and avoiding common mistakes, you can write cleaner, more efficient Java code.
For more details, check the official Java documentation on the Stream API.
Feel free to share your thoughts, experiences, or questions regarding primitive streams in Java 8 in the comments section below! Happy coding!