Why Changing HashMap Implementation Can Break Your Code
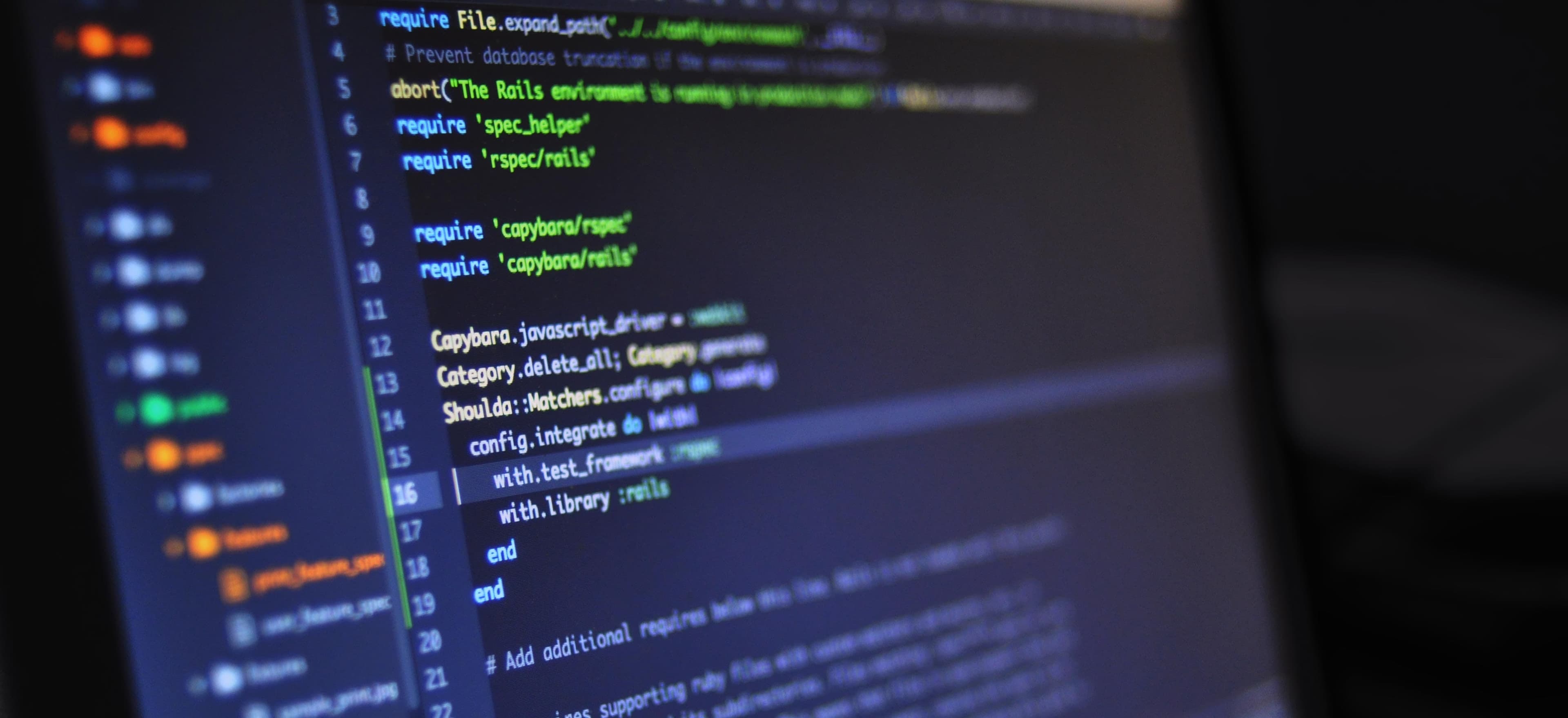
- Published on
Why Changing HashMap Implementation Can Break Your Code
In Java, the HashMap is one of the most widely used data structures. It provides a way to store key-value pairs in an efficient manner. However, changes to the underlying implementation of HashMap can cause unexpected issues in your code. In this blog post, we will explore the intricacies of HashMap, what happens when you change its implementation, and best practices to safeguard your code.
Understanding HashMap
A HashMap is a part of the Java Collections Framework and implements the Map interface. It stores data in key-value pairs, allowing quick retrieval based on keys. The core concepts of HashMap include:
-
Hashing: It uses a hash function to compute an index (hash code) into an array of buckets or slots, from which the desired value can be found.
-
Collisions: When two keys hash to the same index, this is called a collision. Java manages collisions using a technique called chaining or, in later versions, a balanced tree structure.
Because of its efficiency in searching, adding, and removing key-value pairs, HashMaps are often chosen for large datasets. However, that's where potential pitfalls lie – particularly in how different implementations can lead to issues in code that relies on specific behaviors.
Why Changing the Implementation Matters
In Java 8, the implementation of HashMap underwent a significant change. Previously, if a bucket (a place where key-value pairs are stored) became too crowded (with a threshold defined by load factors), it would handle collisions using linked lists. However, in an effort to improve performance, the Java 8 version introduced a new structure—balanced trees, specifically Red-Black trees — when the number of elements in a bucket exceeds a certain limit.
Performance Considerations
This implementation change offers benefits but could also lead to performance regressions in specific scenarios. For example, if your application heavily relies on the performance characteristics of a linked list but has shifted to a tree structure, the lookup time could increase, leading to unexpected slowdowns.
This difference is crucial for developers to understand. To tackle this, always benchmark your application after any library or framework updates.
Example of HashMap Basics
To illustrate the basic usage of HashMap and how changing its behavior can affect your code, consider the following code snippet:
import java.util.HashMap;
public class HashMapExample {
public static void main(String[] args) {
HashMap<String, Integer> map = new HashMap<>();
// Inserting data
map.put("Alice", 30);
map.put("Bob", 25);
// Retrieving data
Integer ageAlice = map.get("Alice");
System.out.println("Alice's age: " + ageAlice);
// Handling missing keys
Integer ageCharlie = map.get("Charlie"); // returns null
System.out.println("Charlie's age: " + ageCharlie);
}
}
Commentary on the Code
-
Insertion and Retrieval: In this basic example, we create a HashMap, add two entries, and retrieve one. This encapsulates the fundamental duties of a HashMap.
-
Handling Null Values: Notice how retrieving a non-existent key returns
null
. This can be alarming if you have logic that doesn't account for null values.
When you switch from Java 7 to Java 8 or higher, any heavy use of HashMap could be impacted tremendously based on the underlying collision resolution strategy that changes its behavior at runtime and affects performance.
Best Practices
1. Test Your Code After Updates
Always write unit tests and integration tests. Ensure that these tests encompass a variety of scenarios, including edge cases, to ascertain that your code behaves correctly with the new HashMap implementation.
2. Avoid Assumptions
Assumptions about the order of elements or their retrieval times can lead to bugs. For example, before Java 8, the order of keys retrieved from a HashMap could be considered fairly stable, but this is not guaranteed. If a HashMap is converted internally to a tree structure, key retrieval can incur different performance costs.
3. Understand the Load Factor
Java HashMaps utilize a load factor, which conditionally determines when the map should be resized. By default, the load factor is set to 0.75, balancing space and performance. Unequivocally understanding this can prevent unanticipated performance issues when a resize happens.
HashMap<String, Integer> map = new HashMap<>(16, 0.75f);
Updating these parameters appropriately can lead to optimized performance for specific use cases.
4. Usage of SynchronizedMap
If your application is multithreaded, consider using Collections.synchronizedMap()
around your HashMap to avoid concurrent modification exceptions and ensure thread safety.
Map<String, Integer> synchronizedMap = Collections.synchronizedMap(new HashMap<>());
With the right threading model and design, issues become less likely, although modifying data in concurrent scenarios should still be handled appropriately.
Performance Testing
To fully understand the effects of implementation changes and to gauge performance impact, consider running benchmarks. Libraries like JMH can help you compare performance in various scenarios.
Closing Remarks
While the HashMap is a powerful and versatile data structure, changes in its implementation can perplex developers and introduce bugs. Being aware of these pitfalls can help you write resilient and maintainable code. Always test after updates, avoid assumptions about ordering and performance, and consider best practices in your coding standards.
For further reading about the internals of Java HashMap, you can refer to the official documentation on Oracle's Java SE Documentation and explore advanced performance implications on Baeldung.
By following these guidelines, you'll safeguard your applications against unwanted surprises—all thanks to a deeper understanding of how HashMap works under the hood. Happy coding!
Checkout our other articles