Common MVVM Pitfalls in ZK Framework: Update View Issues
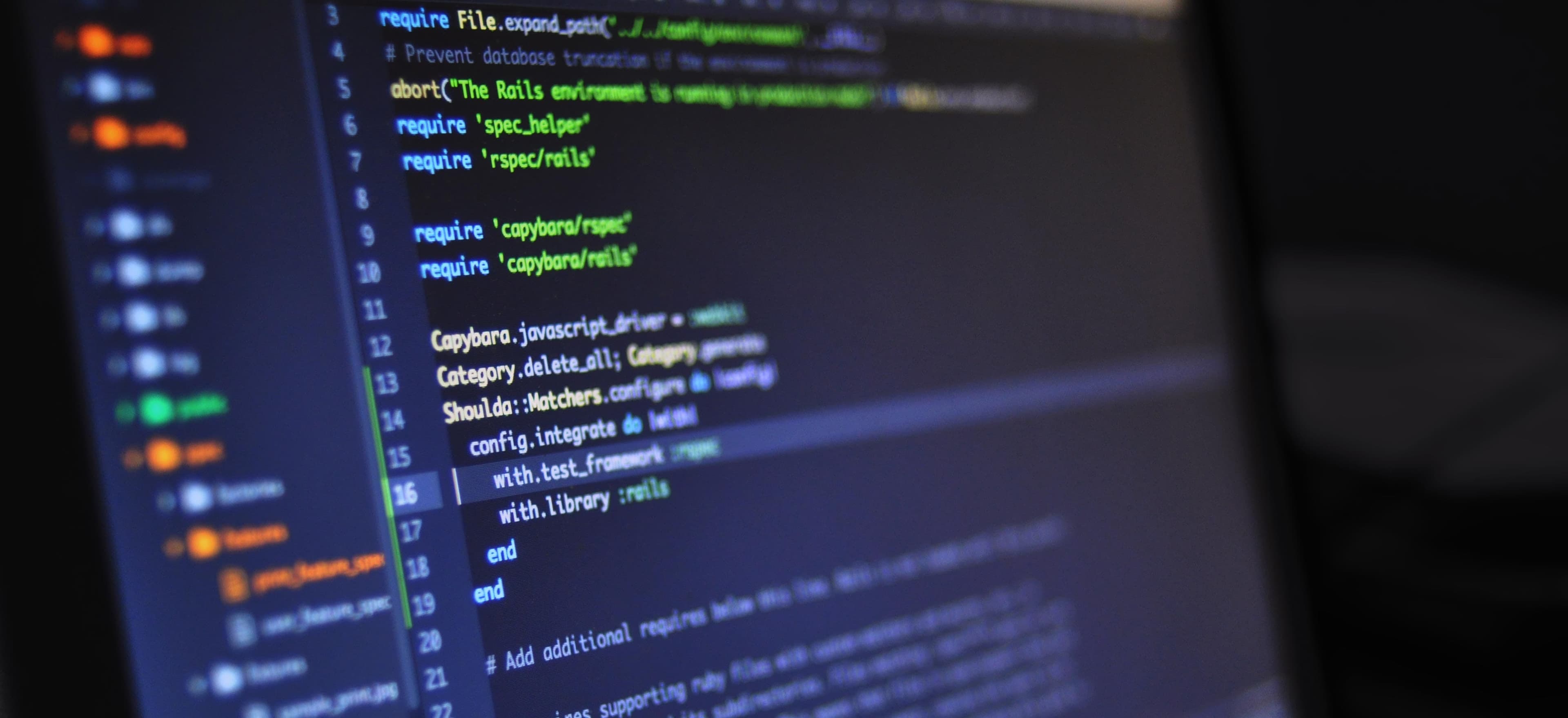
- Published on
Common MVVM Pitfalls in ZK Framework: Update View Issues
The Model-View-ViewModel (MVVM) design pattern is widely adopted in modern web development, offering a clean separation of concerns between the user interface and business logic. In the ZK framework, a popular Java framework for building rich web applications, implementing the MVVM pattern can enhance the maintainability and scalability of your projects. However, like any design pattern, it presents its challenges, especially when it comes to updating views. This article will explore common MVVM pitfalls in ZK, particularly focusing on view update issues, why they occur, and how to resolve them.
Understanding MVVM in ZK Framework
ZK is a Java-based framework that enables developers to create rich web applications easily. It incorporates the MVVM pattern which consists of three components:
- Model: Represents the application's data and business logic.
- View: The user interface, usually defined in XML.
- ViewModel: Acts as a mediator between the Model and View, containing properties and commands for data binding.
Basic Structure of an MVVM Application in ZK
Before diving deeper into the pitfalls, let's get an understanding of a simple MVVM architecture in ZK.
<window apply="org.zkoss.bind.BindComposer" viewModel="@id('vm') @init('com.example.MyViewModel')">
<label value="@{vm.message}" />
<button label="Update" onClick="@command('updateMessage')" />
</window>
In this example, a label
is bound to a property of the ViewModel, and clicking the button triggers a command in the ViewModel. The separation of concerns here allows for a clean architecture, but it requires careful handling of updates.
Common Pitfalls in View Updates
1. Failure to Notify Changes
One of the most common pitfalls in MVVM with the ZK framework is failing to notify the view of changes made in the ViewModel. ZK provides data binding capabilities, but if the properties in the ViewModel are not observable, the UI will not update.
Example
public class MyViewModel {
private String message;
public String getMessage() {
return message;
}
public void setMessage(String message) {
this.message = message;
// Notify the view of the change
Clients.clearBusy();
}
@Command
public void updateMessage() {
this.setMessage("Hello, ZK Framework!");
}
}
Solution
Use @NotifyChange
to automatically update the view when a property changes.
@NotifyChange("message")
public void setMessage(String message) {
this.message = message;
}
2. Binding Issues
Binding issues often arise due to mismatched names or paths. When ZK cannot find the property specified in the binding expression, it results in a silent failure.
Example
<label value="@{vm.nonExistentProperty}" />
Solution
Double-check the property names in both the ViewModel and the XML. Maintain consistency in naming conventions.
3. Asynchronous Updates
ZK allows for asynchronous updates via commands or events. However, if you're not careful about managing state, you could end up with a UI that displays stale data.
Example
Imagine performing a command that takes time to complete:
@Command
public void longRunningProcess() {
// Simulate a long-running process
Thread.sleep(5000);
this.setMessage("Process Completed");
}
Solution
Instead of blocking the UI thread, use a separate thread and update the ViewModel upon completion.
@Command
public void longRunningProcess() {
new Thread(() -> {
try {
Thread.sleep(5000);
} catch (InterruptedException e) {
// handle exception
}
this.setMessage("Process Completed");
}).start();
}
4. Missing Bindings in Views
Another potential issue can arise when components are not properly bound to their respective properties in the ViewModel. This often occurs when a developer forgets to define bindings in the XML or uses the wrong syntax.
Example
<input textbox="@{vm.inputValue}" />
If inputValue
has not been defined in the ViewModel, this will lead to no updates being reflected in the view.
Solution
Always ensure that properties are defined in the ViewModel and are correctly referenced in the binding expressions.
5. Incorrect Event Handling
ZK provides a robust event handling mechanism. If events are not handled correctly or if the method signatures do not match, updates may not be triggered.
Example
@Command
public void saveData() {
// logic to save data
}
If you reference @command('saveData')
but the method is not defined correctly, it will not work as expected.
Solution
Double-check the method signatures and ensure they match what you have declared in the XML.
Best Practices to Avoid These Pitfalls
-
Use @NotifyChange: Always use the
@NotifyChange
annotation to ensure the UI stays updated with changes. -
Validate Bindings: Regularly validate your bindings in the XML and ensure they correspond to properties in your ViewModel.
-
Debugging: Use tools like ZK’s built-in debug mode to trace updates and watch for missed bindings or changes.
-
Concurrency Handling: Always manage asynchronous operations with UI updates in mind to avoid blocking threads.
-
Testing: Regularly test your application to ensure that the separated concerns maintain their integrity and respond as expected. You can utilize testing frameworks like JUnit to simulate and validate your ViewModel logic.
Lessons Learned
Working with the MVVM pattern in the ZK framework enhances the development workflow but also introduces challenges that can derail updates to the view. By understanding common pitfalls such as notification failures, binding issues, and improper event handling, you can establish best practices that will help you maintain a robust application.
If you're diving deeper into ZK and MVVM, consider exploring the ZK Documentation for comprehensive guidance and tips. Adopting these best practices will lead to smoother application development and a better user experience.
Happy coding, and make the most out of the ZK framework!
Checkout our other articles