Managing Flyway Migrations in Kubernetes: Common Pitfalls
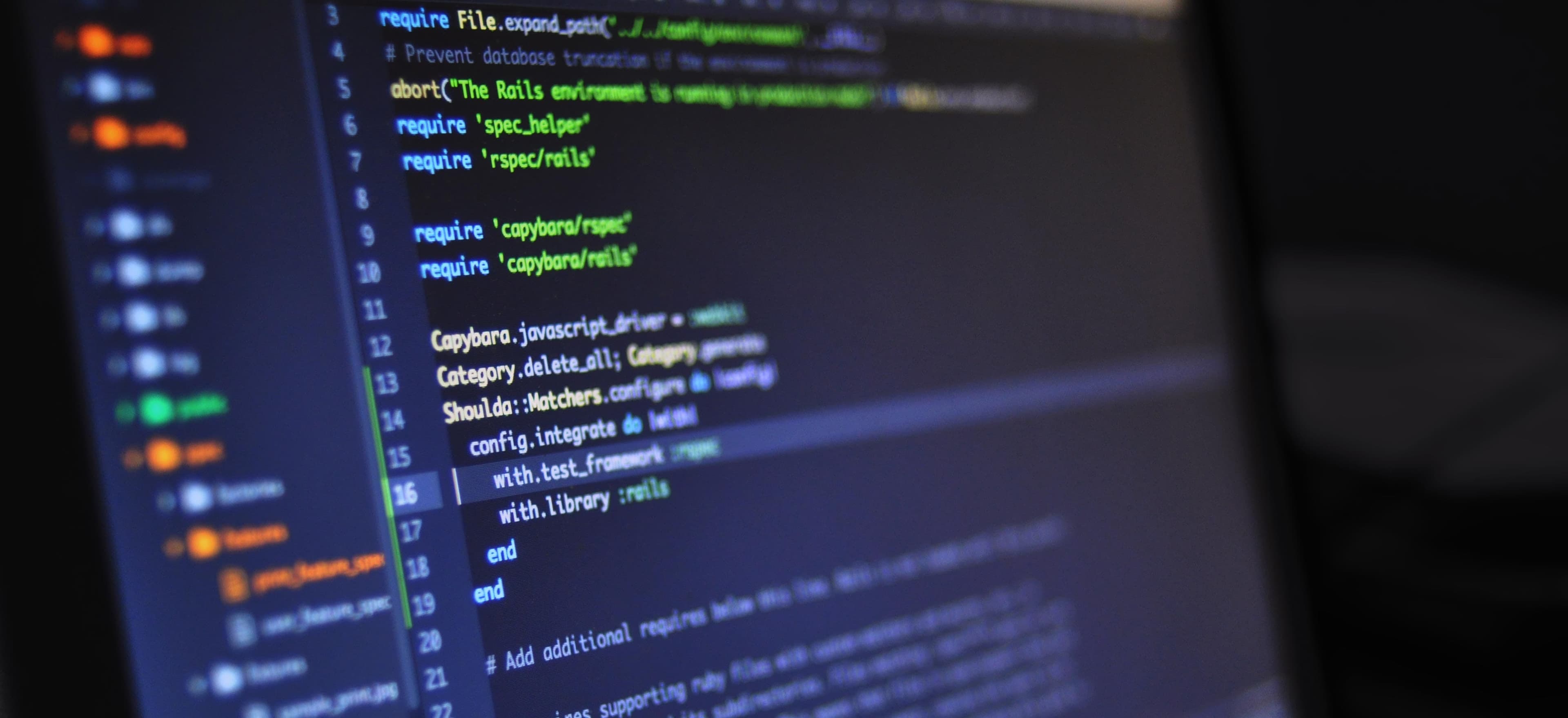
- Published on
Managing Flyway Migrations in Kubernetes: Common Pitfalls
In continuous integration/continuous deployment (CI/CD) environments, managing database migrations becomes critical, especially when you're working with container orchestration platforms like Kubernetes. One popular tool for database migrations in Java projects is Flyway. While Flyway simplifies the process of managing schema versions through SQL scripts, deploying these migrations in a Kubernetes environment can lead to several challenges.
In this post, we’ll discuss common pitfalls when managing Flyway migrations in Kubernetes and explore strategies for effectively orchestrating those migrations.
Understanding Flyway Migrations
What is Flyway?
Flyway is an open-source database migration tool that enables developers to version control their database changes. It uses plain SQL scripts or Java code to perform migrations, which means developers can apply changes easily and rollback when necessary. The Flyway core concept revolves around versioned migration files that are applied in sequential order.
For a comprehensive overview of Flyway, check out Flyway's official documentation.
How Flyway Works
Flyway operates by performing tasks such as:
- Checking the current database version.
- Applying any pending migrations to bring the database to the latest version.
- Tracking applied migrations to ensure they are not reapplied.
Integration with Java
When using Flyway in a Java application, you'd typically configure it in your application.properties
or application.yml
file. Here’s an example of a basic Flyway configuration in a Spring Boot application:
spring:
flyway:
enabled: true
url: jdbc:postgresql://localhost:5432/mydb
user: myuser
password: mypassword
locations: classpath:db/migration
Common Pitfalls in Managing Flyway Migrations in Kubernetes
When deploying Flyway migrations in a Kubernetes environment, it is vital to be aware of common pitfalls that can lead to issues. Let's take a look at some of the prominent challenges.
1. Coordinate Migrations with Application Deployments
One of the common pitfalls in a Kubernetes environment is executing Flyway migrations concurrently with application deployments. If you’re not careful, this can lead to race conditions where the application tries to access a database that has not yet been properly migrated.
Solution
Implement a migration job in Kubernetes that completes before the application deployment begins. This can be achieved through a Kubernetes Job resource. Here’s an exemplary configuration:
apiVersion: batch/v1
kind: Job
metadata:
name: flyway-migration
namespace: my-namespace
spec:
template:
spec:
containers:
- name: flyway
image: flyway/flyway:latest
env:
- name: FLYWAY_URL
value: jdbc:postgresql://my-database:5432/mydb
- name: FLYWAY_USER
value: myuser
- name: FLYWAY_PASSWORD
value: mypassword
- name: FLYWAY_LOCATIONS
value: classpath:db/migration
command: ["flyway", "migrate"]
restartPolicy: OnFailure
This Job ensures that migrations are applied before your application starts, eliminating race conditions.
2. Environment-Specific Configuration
You may run into situations where your Flyway configurations differ significantly between environments (e.g., development, staging, and production). Hardcoding different settings can lead to confusion and errors.
Solution
Make use of Kubernetes Secrets or ConfigMaps to manage your Flyway configurations. You can define environment-specific database connection strings using these resources. Here's an example of a ConfigMap:
apiVersion: v1
kind: ConfigMap
metadata:
name: flyway-config
data:
FLYWAY_URL: jdbc:postgresql://my-database:5432/mydb
FLYWAY_USER: myuser
FLYWAY_PASSWORD: mypassword
Then you can reference this ConfigMap in your Kubernetes Job as follows:
env:
- name: FLYWAY_URL
valueFrom:
configMapKeyRef:
name: flyway-config
key: FLYWAY_URL
3. Monitoring and Logging
Often overlooked, monitoring and logging migrations can help you troubleshoot issues. If something goes wrong during a migration, you need to have logs available for debugging.
Solution
Integrate logging within your migration pods. Make sure the container’s logs are visible, typically through the standard output of Kubernetes. Use a centralized logging solution like ELK (Elasticsearch, Logstash, Kibana) stack or Prometheus with Grafana for more robust monitoring.
4. Handling Rollbacks
Handling rollbacks in Flyway can be tricky, especially since Flyway does not support automatic rollbacks from applied migrations.
Solution
Plan your migrations carefully to create backward-compatible changes. Additionally, you can write rollback scripts that can be manually invoked in case of errors.
5. Managing Flyway Versioning and Scripts
Keeping track of multiple migration scripts can become complex, especially if multiple teams are modifying the database structure frequently.
Solution
Establish clear guidelines for versioning your migration scripts. Use semantic versioning (e.g., V1__Initial.sql
, V1_1__AddColumn.sql
), and ensure scripts are well-documented for clarity.
Closing Remarks
Managing Flyway migrations in Kubernetes has its challenges, but understanding these common pitfalls can help streamline the process. By making use of Kubernetes Jobs for migrations, utilizing ConfigMaps and Secrets for configuration management, ensuring thorough logging, and carefully planning for rollbacks and script versioning, you can effectively manage your database migrations in a Kubernetes environment.
As you deploy your applications, a well-structured migration strategy is paramount to achieving a successful deployment and maintaining database integrity.
If you want to delve deeper into database management practices, consider reading about database schema management best practices for a broader view.
With careful planning and strategy, you can overcome the hurdles of Flyway migrations and harness the full potential of your Kubernetes deployments.
Feel free to explore the Flyway documentation further and adapt the techniques mentioned in this post to fit your project's needs. Happy coding!