Struggling with Bigtable Pagination in Java? Here's the Fix!
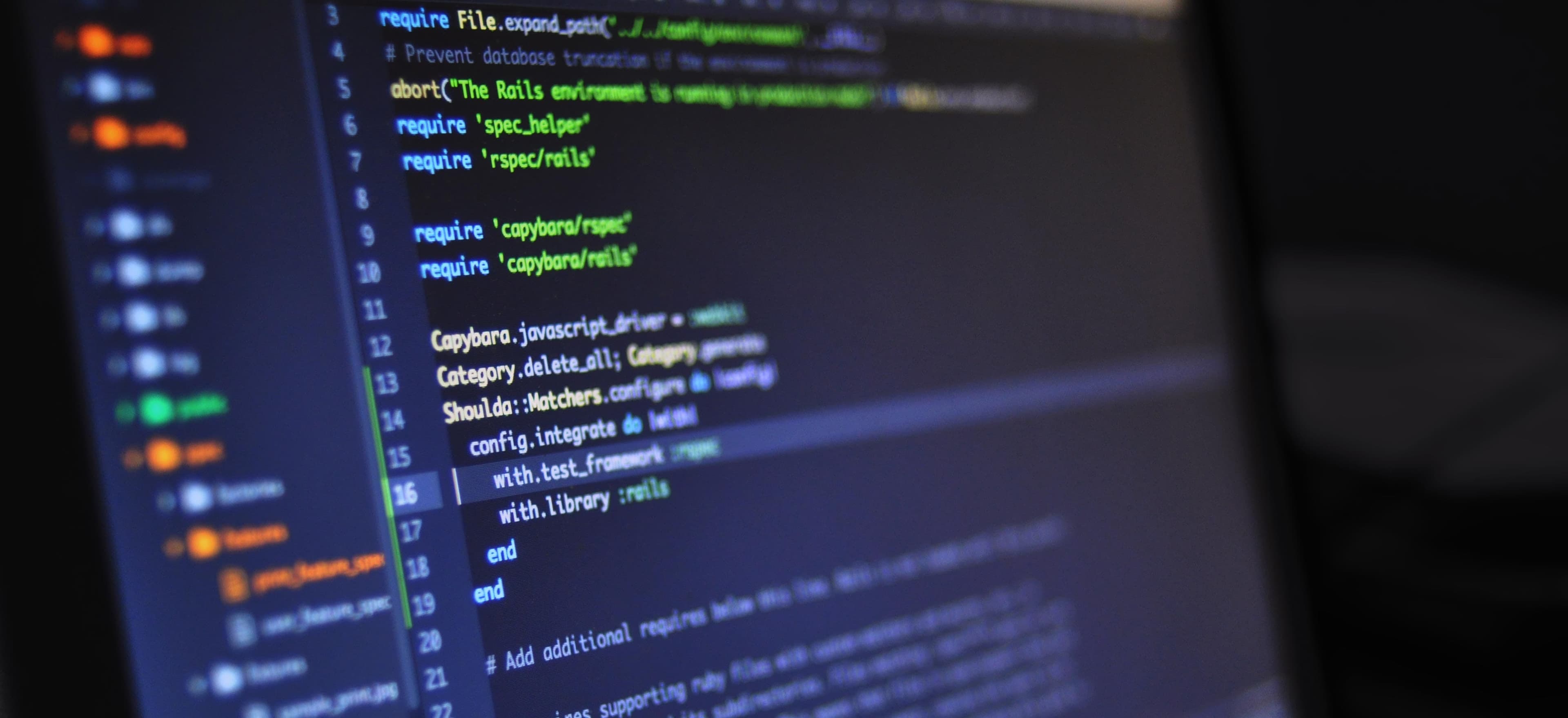
- Published on
Struggling with Bigtable Pagination in Java? Here's the Fix!
When it comes to managing large datasets in Google Cloud, Bigtable stands as a powerful, scalable solution designed for high-performance applications. However, as developers wade through extensive rows of data, they often encounter the challenge of pagination. Pagination is essential for user experience, allowing users to navigate through massive datasets seamlessly. In this post, we’ll explore the intricacies of pagination in Bigtable using Java and illustrate an effective solution to make the process manageable.
Understanding Bigtable and its Pagination
Google Bigtable is a NoSQL database designed to handle vast amounts of structured data across many machines. While its setup is powerful, navigating through the rows and columns can become cumbersome without a well-implemented pagination strategy.
What is Pagination?
Pagination is the process of dividing a dataset into smaller, manageable segments (or pages). When dealing with Bigtable, pagination typically implies limiting the number of rows returned in a single query to prevent overwhelming customers and servers alike. However, implementing pagination in Bigtable presents certain challenges due to its underlying architecture.
Challenges of Pagination in Bigtable
-
Lack of Built-in Pagination Support: Unlike traditional SQL databases which offer features like
LIMIT
andOFFSET
, Bigtable requires custom implementations for pagination. -
Performance Concerns: Fetching the entire dataset, and then filtering it in memory, can lead to performance bottlenecks.
-
Row Key Design: The design of your row keys can greatly influence the efficiency and ease of pagination.
Key Concepts of Pagination in Bigtable
Before diving into the code, let’s clarify some fundamental concepts essential for implementing pagination effectively.
-
Row Limit: The maximum number of rows you want to fetch per page.
-
Start Row Key: The key from which you want to start fetching rows.
-
Continuity: For a smooth user experience, it's pivotal to maintain continuity between consecutive pages.
Implementing Pagination in Bigtable
Let’s jump into the practical side of things. Below, we will demonstrate a basic Java method to implement pagination with Google Cloud Bigtable. First, make sure you have the necessary dependencies in your pom.xml
file:
<dependency>
<groupId>com.google.cloud</groupId>
<artifactId>google-cloud-bigtable-client</artifactId>
<version>1.29.0</version>
</dependency>
Sample Code for Bigtable Pagination
Let us write a method that fetches data in a paginated manner. This method accepts parameters for tableId
, rowLimit
, and an optional startRowKey
.
import com.google.cloud.bigtable.data.v2.BigtableDataClient;
import com.google.cloud.bigtable.data.v2.models.RowMutation;
import com.google.cloud.bigtable.data.v2.models.Row;
import com.google.cloud.bigtable.data.v2.models.RowFilter;
import java.util.List;
import java.util.ArrayList;
public class BigtablePagination {
public List<Row> fetchPaginatedRows(String projectId, String instanceId, String tableId, int rowLimit, String startRowKey) {
List<Row> rows = new ArrayList<>();
try (BigtableDataClient dataClient = BigtableDataClient.create(projectId, instanceId)) {
// Create a ReadOptions object to specify pagination.
ReadRowsRequest request = ReadRowsRequest.newBuilder()
.setTableId(tableId)
.setLimit(rowLimit)
.setRowPrefix(startRowKey)
.build();
// Fetch the rows from Bigtable.
for (Row row : dataClient.readRows(request).getRows()) {
rows.add(row);
}
} catch (Exception e) {
System.err.println("Error fetching rows: " + e.getMessage());
}
return rows;
}
}
Breakdown of the Code
-
Creating the Data Client: We utilize
BigtableDataClient
to connect to our Bigtable instance. -
Building the Request: The
ReadRowsRequest
facilitates the retrieval of data where we define the table ID, row limit, and optional start row key for pagination. -
Iterating Through Rows: The retrieved results are added to a list, enabling efficient access and further processing.
Why Use RowPrefix and Pagination?
Using the row prefix enhances the query by allowing you to specify the starting point for your data extraction. You can initialize startRowKey
to the last key from the previous page, ensuring seamless transitions between pages.
Handling Edge Cases in Pagination
While the sample code lays a solid foundation for pagination, it’s essential to address potential edge cases:
Empty Responses
Handling situations where no rows are returned is vital:
if (rows.isEmpty()) {
System.out.println("No rows found for the given pagination criteria.");
}
Limitations of Start Row Key
Ensure that when you set the startRowKey
, it aligns with existing keys to avoid confusion in pagination.
Performance Enhancements
Utilize caching strategies for frequently accessed data, as it can improve read performance significantly.
Testing Your Pagination Code
Before deploying, thorough testing is paramount. To validate the functionality of your pagination code, consider the following strategies:
-
Unit Tests: Create concise unit tests to confirm the expected results from the pagination logic.
-
Performance Testing: Simulate large datasets to evaluate how your pagination performs under load.
-
User Simulation: Emulate user behavior to ensure that the pagination experience is seamless and intuitive.
Closing Remarks
Paginating data in Google Bigtable using Java may seem daunting, but with the right approach, it can be manageable. The key lies in understanding your dataset’s structure, implementing strategic code for data retrieval, and addressing potential challenges upfront. If you find yourself struggling with Bigtable pagination, leverage the sample code provided, adapt it as needed, and enhance your application's performance and user experience.
For further enhancement on this topic, feel free to explore the Google Cloud Bigtable Documentation and stay updated with new best practices!
By mastering pagination in Bigtable, you not only enhance the application’s usability but also bolster its performance in managing extensive datasets. Happy coding!