Speeding Up Your Android Test Automation Process
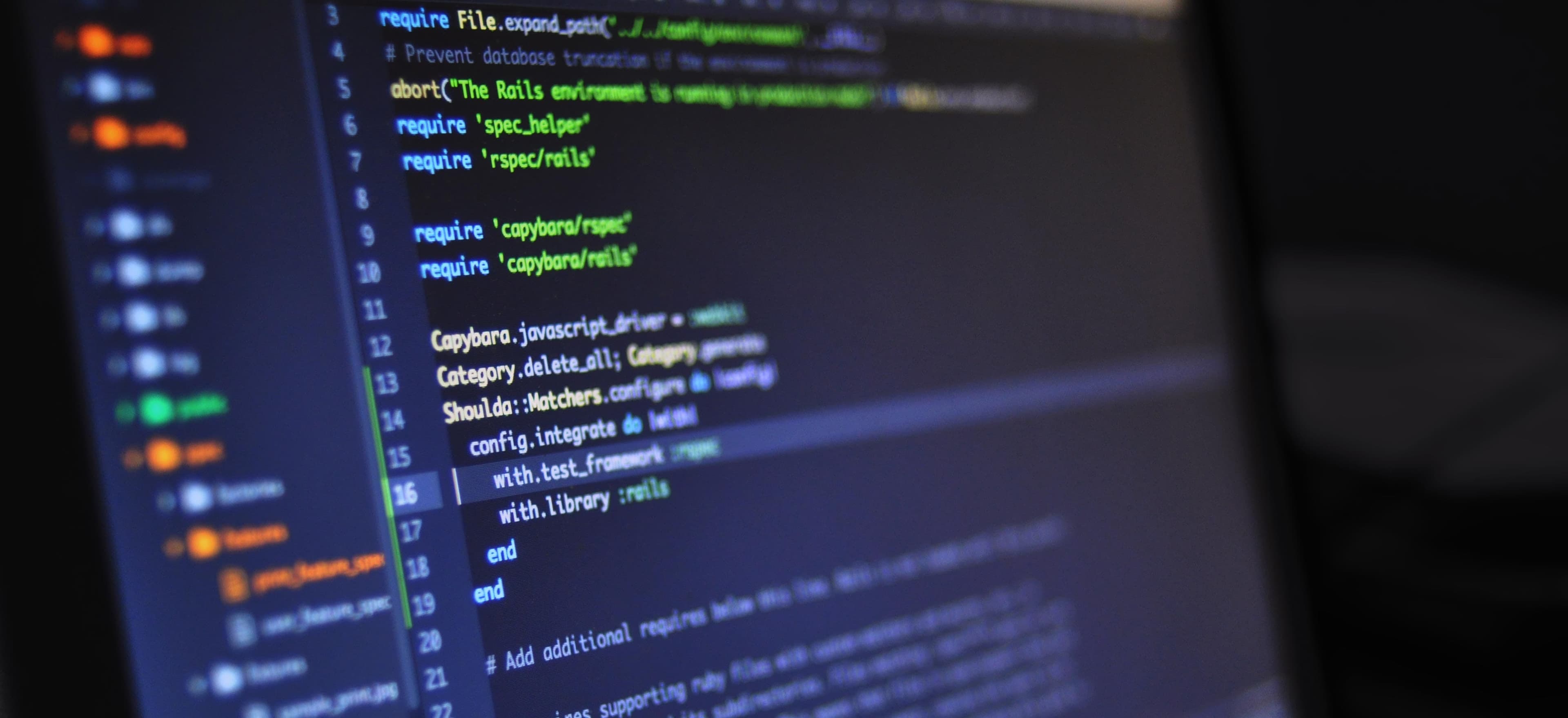
- Published on
Speeding Up Your Android Test Automation Process
As the Android ecosystem evolves, so does the necessity for efficient test automation. Android test automation can speed up development cycles, improve software quality, and enhance team productivity. However, one of the primary challenges that teams encounter involves optimizing this testing process. In this blog post, we will explore various methods to speed up your Android test automation process, including strategies, tools, and code examples you'll find invaluable.
Why Test Automation Matters
Before delving into how to optimize the automation process, it's crucial to understand why automating tests is essential. Here’s a brief rundown:
- Speed: Automated tests can be run significantly faster compared to manual testing.
- Consistency: Automation ensures that each test is performed identically, reducing variability.
- Repeatability: Automated tests can run as many times as necessary, facilitating regression testing.
- Resource Efficiency: Allows the QA team to focus on critical areas while reducing time spent on mundane tasks.
If you're looking for additional resources for Android testing, consider checking out Android Testing Codelabs.
Setting Up for Speed
The right setup lays the foundation for a faster automation process. Here’s how you can get started.
Choose the Right Tools
Select testing frameworks that offer seamless implementation. Some of the more popular tools include:
- Espresso: For UI testing.
- Robolectric: For unit testing.
- Mockito: For mocking.
- UI Automator: For cross-app functional UI testing.
Using these tools can simplify setup and speed up test execution.
Define Test Strategies
Having a robust test strategy is paramount. You should include:
- Unit Tests: Test individual components and functions.
- Integration Tests: Test interaction among components.
- UI Tests: Validate user interfaces.
Combining these tests effectively will diminish redundant checks and enhance speed.
Code Example: Espresso Simplified
Let’s dive into a simple Espresso test case.
import android.support.test.rule.ActivityTestRule;
import android.support.test.ext.junit.runners.AndroidJUnit4;
import org.junit.Rule;
import org.junit.Test;
import org.junit.runner.RunWith;
import static android.support.test.espresso.Espresso.onView;
import static android.support.test.espresso.action.ViewActions.click;
import static android.support.test.espresso.matcher.ViewMatchers.withId;
@RunWith(AndroidJUnit4.class)
public class ExampleTest {
@Rule
public ActivityTestRule<MainActivity> activityRule = new ActivityTestRule<>(MainActivity.class);
@Test
public void testButtonClick() {
// This test checks if the button is clickable and performs its action.
onView(withId(R.id.my_button)).perform(click());
// Verify the expected result here...
}
}
Why This Code Matters: The test focused on a single user interaction - clicking a button - in isolation, which allows for quicker execution and faster feedback on functionality.
Parallel Testing
Another method to expedite your test execution is through parallel testing. Running multiple tests simultaneously can save time, especially in larger test suites.
Here’s a high-level example using Gradle to enable parallel execution:
Update Gradle Configurations
In gradle.properties
, add:
android.useAndroidX=true
android.enableJetifier=true
org.gradle.parallel=true
By enabling parallel execution, tests that can be conducted independently will run at the same time, reducing overall execution time.
Continuous Integration / Continuous Delivery (CI/CD)
Incorporate CI/CD tools such as Jenkins or GitHub Actions to automate your testing process. This allows for the automatic running of tests with every code change, catching issues early in the cycle.
Example Jenkins Pipeline
Here’s a basic Jenkins pipeline setup for running your tests:
pipeline {
agent any
stages {
stage('Build') {
steps {
sh './gradlew build'
}
}
stage('Test') {
steps {
sh './gradlew test'
}
}
stage('UI Testing') {
steps {
sh './gradlew connectedAndroidTest'
}
}
}
}
Why CI/CD?: Implementing CI/CD ensures that your tests are consistently run against the latest codebase, catching bugs before they make it to production.
Mocking and Stubbing
Mocking external dependencies is another effective way to speed up testing. This isolates your test cases and ensures that they run without network calls or external API responses.
Example with Mockito
Assuming you want to mock a network call in a ViewModel class:
import org.junit.Test;
import org.mockito.Mockito;
public class UserViewModelTest {
@Test
public void fetchUser_DataIsFetched() {
UserRepository mockRepo = Mockito.mock(UserRepository.class);
UserViewModel userViewModel = new UserViewModel(mockRepo);
Mockito.when(mockRepo.fetchUser()).thenReturn(new User("name", "email"));
userViewModel.fetchUser();
// Assert that user data matches expectations...
}
}
Why Mocking is Important: This test does not depend on real data, making it fast and reliable.
Optimize Test Organization
An organized test suite can make a big difference. Group related tests, use appropriate naming conventions, and implement tagging to selectively run tests based on priorities or categories.
- Test groupings can minimize the tests run during specific CI/CD stages, enhancing speed.
- Use annotations provided by testing frameworks to categorize and selectively execute tests.
Wrapping Up
In an ever-evolving technological landscape, speeding up your Android test automation process is not just an option but a necessity. By following the strategies outlined in this article, you can optimize test execution, improve accuracy, and sustain quality without going through a cumbersome manual testing process.
In summary, consider these strategies:
- Choose the right tools.
- Define testing strategies.
- Leverage parallel testing and CI/CD tools.
- Use mocking and stubbing for isolated tests.
- Keep your test suite organized and efficient.
To keep enhancing your Android testing skills, explore Android Testing Documentation for more comprehensive guides and tutorials.
By implementing these techniques in your Android testing practice, you can build a robust testing framework that not only saves time but also increases reliability at every stage of your development. Happy coding!