Handling CORS Issues in Spring REST with AJAX Calls
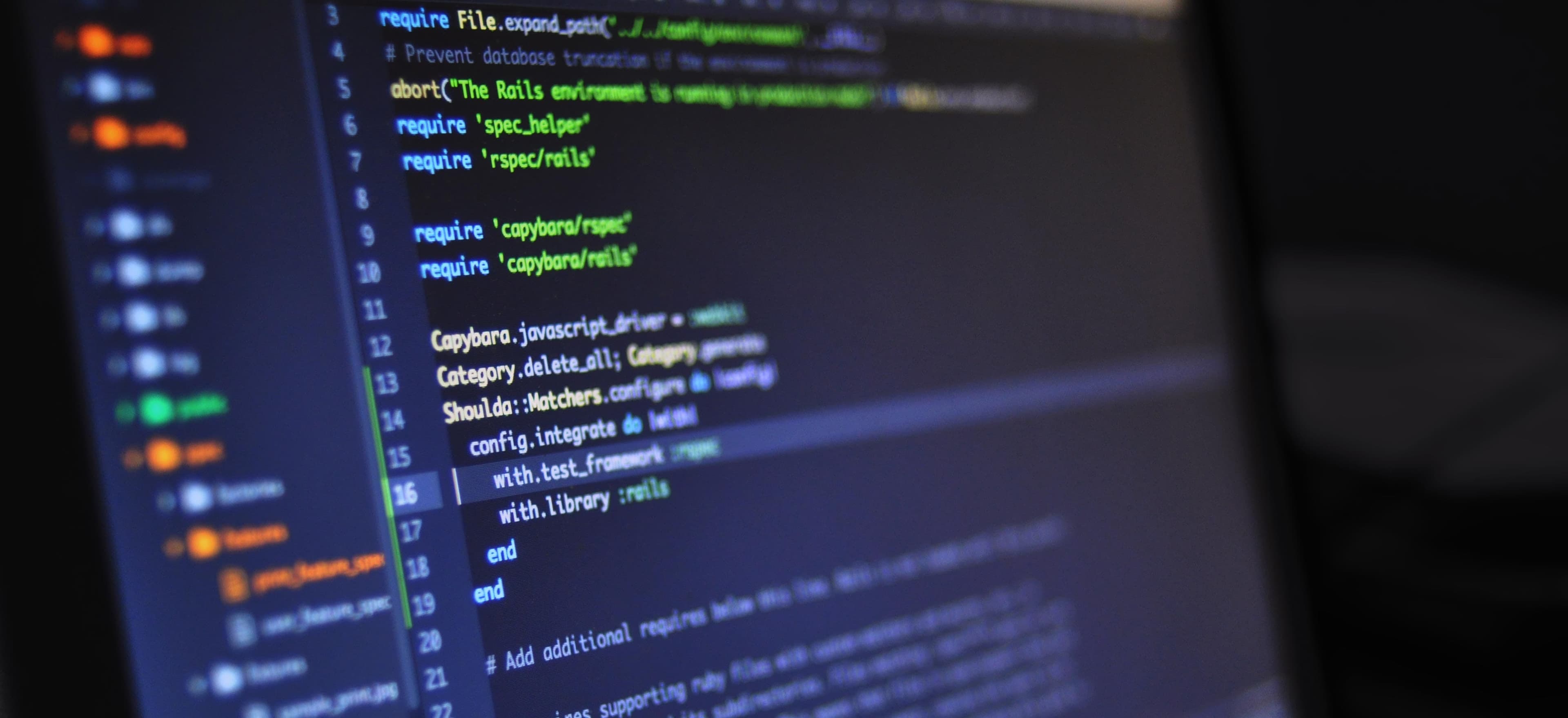
- Published on
Handling CORS Issues in Spring REST with AJAX Calls
Cross-Origin Resource Sharing (CORS) is a security feature implemented by browsers to prevent malicious sites from making unauthorized requests to another domain. Understanding how to manage CORS effectively is crucial, especially when developing web applications that utilize RESTful services. This blog post will walk you through handling CORS issues in Spring REST APIs while making AJAX calls.
What is CORS?
CORS is a protocol that enables restricted resources on a web page to be requested from another domain outside the domain from which the first resource was served. Browsers implement CORS so that they can modify the behavior of requests from one domain to another.
For example, if your front-end application is hosted at http://localhost:3000
and your backend API is at http://localhost:8080
, your web application would face CORS restrictions when trying to access the APIs because they are on different origins.
Identifying CORS Issues
Common signs of CORS issues include:
-
Error messages in the browser console. For example:
Access to XMLHttpRequest at 'http://localhost:8080/api/resource' from origin 'http://localhost:3000' has been blocked by CORS policy.
-
The inability to make successful AJAX calls to your Spring REST API.
Setting Up CORS in a Spring Boot Application
Spring Boot makes handling CORS configurations straightforward. Here’s how you can do it.
Step 1: Adding Dependencies
Ensure that you have the appropriate dependencies in your pom.xml
. Typically, you would include Spring Web Starter:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
Step 2: Global CORS Configuration
For applications that require access across many endpoints, global CORS configuration can be implemented. This is useful if you are exposing numerous APIs.
Create a configuration class, WebConfig
, for CORS setup:
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.web.servlet.config.annotation.CorsRegistry;
import org.springframework.web.servlet.config.annotation.EnableWebMvc;
import org.springframework.web.servlet.config.annotation.WebMvcConfigurer;
@Configuration
@EnableWebMvc
public class WebConfig implements WebMvcConfigurer {
@Override
public void addCorsMappings(CorsRegistry registry) {
registry.addMapping("/**") // All endpoints
.allowedOrigins("http://localhost:3000") // Front-end URLs
.allowedMethods("GET", "POST", "PUT", "DELETE")
.allowCredentials(true); // Needed for cookies or authentication
}
}
Step 3: Controller-Level CORS Configuration
In some cases, CORS settings may only be necessary for specific endpoints. Here's how you can handle it at the controller level:
import org.springframework.web.bind.annotation.CrossOrigin;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RestController;
@RestController
@CrossOrigin(origins = "http://localhost:3000") // Allowed origin
public class MyController {
@GetMapping("/api/resource")
public String getResource() {
return "CORS worked!";
}
}
Why Use CORS?
- Security: CORS is designed to prevent malicious behavior by allowing only certain domains to access resources.
- Maintainability: By setting CORS correctly, you can easily manage access policies. You won’t have to alter your frontend code, thereby improving maintainability.
For more information on CORS, feel free to refer to Mozilla's CORS documentation.
Making AJAX Calls
Now that CORS is configured, let us move on to how you can make AJAX calls from your front-end application to the Spring REST API.
AJAX Call Example
Here’s a simple jQuery-based AJAX call to your Spring application:
$.ajax({
url: "http://localhost:8080/api/resource",
type: "GET",
success: function(data) {
console.log("Response from API: ", data);
},
error: function(xhr) {
console.error("Error occurred: ", xhr.status, xhr.statusText);
}
});
Code Explanation
- $.ajax: This is the jQuery method for making an AJAX request.
- url: This is the endpoint of your Spring REST API.
- type: Specifies the request type (GET, POST, etc.).
- success: This function is called upon successfully making the request.
- error: This function logs any error that occurs during the request.
Using Fetch API
If you opt for a more modern approach, you can utilize the Fetch API:
fetch("http://localhost:8080/api/resource", {
method: "GET"
})
.then(response => {
if (!response.ok) {
throw new Error("Network response was not ok.");
}
return response.json();
})
.then(data => {
console.log("Response from API: ", data);
})
.catch(error => {
console.error("Error occurred: ", error);
});
Code Explanation
- fetch: A native JavaScript method to make network requests.
- method: Specifies the type of request.
- response.ok: Checks if the response was successful.
- catch: Handles network errors or issues that could arise during the fetch process.
Final Thoughts
Handling CORS issues is essential for permitting safe cross-origin requests, particularly within web applications. In Spring REST applications, configuring CORS can be accomplished globally or on a case-by-case basis, providing flexibility and security.
By implementing these configurations, you can allow your AJAX calls from various origins smoothly. If you still encounter difficulties, double-check your configurations and ensure that your front-end application’s origin is correctly specified.
For a complete guide on setting up Spring Boot with REST APIs, feel free to refer to Spring's official documentation.
Now go ahead and implement these configurations, and you'll be on your way to a seamless development experience!
Checkout our other articles