Java EE 6 Events: Why Developers Seek Lightweight Alternatives
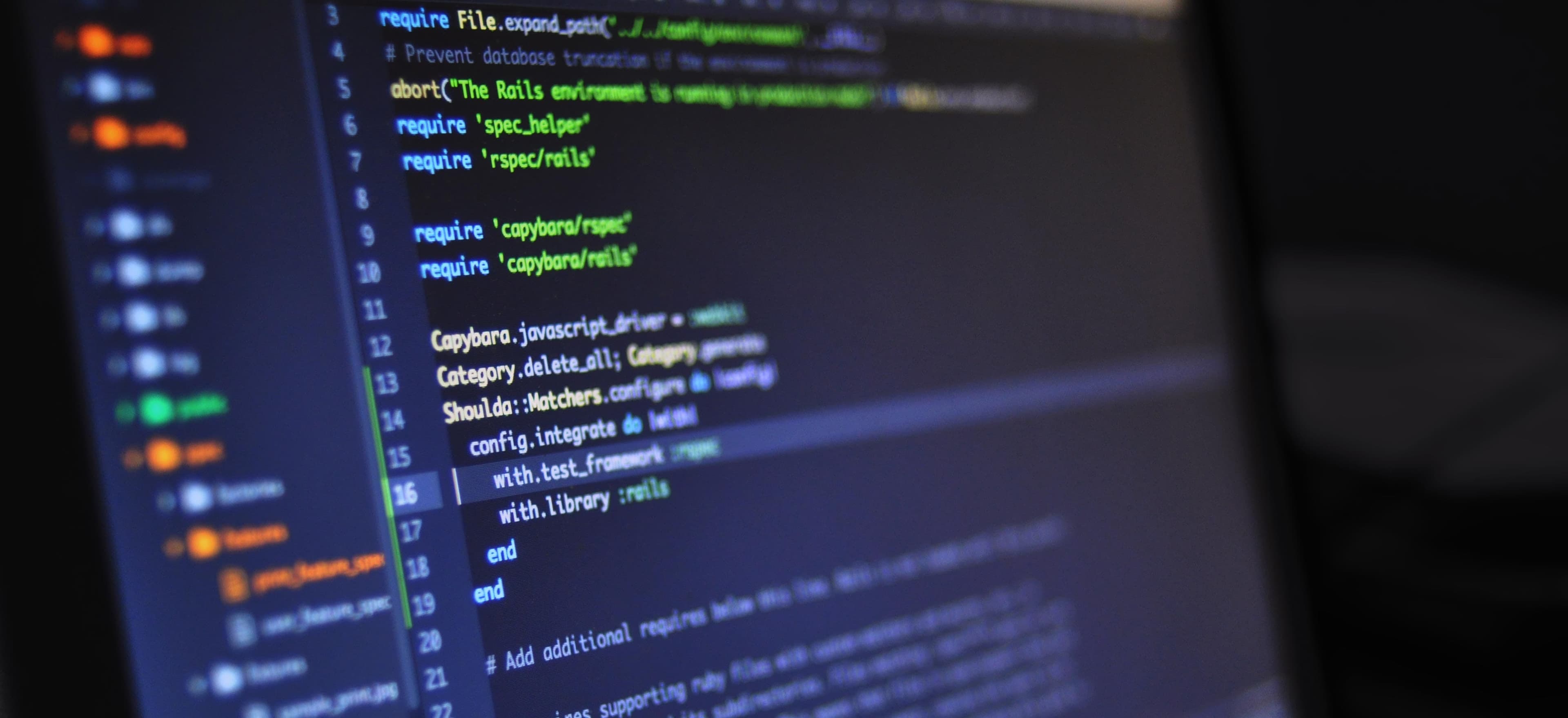
- Published on
Java EE 6 Events: Why Developers Seek Lightweight Alternatives
Java EE 6 introduced additional capabilities to the Java platform, allowing developers to build robust enterprise applications quickly. However, with the rise of modern frameworks and simpler architectures, many developers are seeking lightweight alternatives to Java EE, especially when it comes to the event-driven programming model.
In this blog post, we will explore the Java EE 6 events system, why it's beneficial, and the reasons developers are inclined to explore alternatives. We will also provide code snippets and guidance on how you can implement an event-driven architecture using lightweight frameworks.
Understanding Java EE 6 Events
Java EE 6 provides an event-driven programming model that enables developers to create a responsive and modular architecture. This model revolves around the Event Context and Event Observers.
The Event Context
The event context holds all the information pertaining to the event that has occurred. In Java EE 6, you can leverage the Event
interface to enable your CDI (Contexts and Dependency Injection) beans to fire and listen for events.
Here is a simple code snippet illustrating how to define an event:
import javax.enterprise.event.Event;
import javax.enterprise.event.Observes;
import javax.inject.Inject;
public class EventProducer {
@Inject
private Event<MyEvent> myEvent;
public void triggerEvent(Integer someValue) {
MyEvent event = new MyEvent(someValue);
myEvent.fire(event); // Fires the event
}
}
public class MyEvent {
private Integer value;
public MyEvent(Integer value) {
this.value = value;
}
// Getter and Setter
}
Event Observers
In order to react to these events, you would declare a method as an observer using the @Observes
annotation.
public class EventObserver {
public void onMyEvent(@Observes MyEvent event) {
System.out.println("Event received: " + event.getValue());
}
}
In this example, when triggerEvent
is called from EventProducer
, the onMyEvent
method in EventObserver
is triggered. This entire process allows for a clean separation of concerns.
Why Java EE Events Are Powerful
The event mechanism in Java EE allows for:
-
Decoupling: Your components can interact without being tightly coupled. They can communicate through events, which makes unit testing easier.
-
Flexibility: You can have multiple observers reacting to a single event. This flexibility supports various application use cases.
-
Asynchronous Processing: Events can support asynchronous processing, improving the application's responsiveness.
Limitations of Java EE 6 Events
Despite these advantages, many developers have turned to lightweight alternatives. Here are some compelling reasons for this shift:
Complexity and Overhead
Java EE, while powerful, comes with significant complexity and overhead. Many developers feel overwhelmed by the richness of the Java EE stack, especially for simpler applications. The event system can be particularly verbose and complicated for small projects.
Performance Concerns
Although the event-driven model is beneficial, it can introduce latency, particularly if there are too many observers or if they are performing complex operations. Lightweight alternatives often focus on performance, allowing developers to create applications that are faster and more responsive without the Java EE overhead.
Learning Curve
For new developers, Java EE's extensive API documentation and steep learning curve can be intimidating. Popular lightweight frameworks such as Spring Boot or Micronaut offer much shallower learning paths focusing on ease of use.
Exploring Lightweight Alternatives
Spring Boot
One of the most popular alternatives to Java EE is Spring Boot. It simplifies the development of Java applications by reducing configuration overhead and providing a wide array of functionalities out of the box.
How does Spring Boot handle events? With the use of @EventListener
, you can easily create and listen for events:
import org.springframework.context.ApplicationEventPublisher;
import org.springframework.context.event.EventListener;
import org.springframework.stereotype.Component;
public class ApplicationEventPublisherExample {
private final ApplicationEventPublisher publisher;
public ApplicationEventPublisherExample(ApplicationEventPublisher publisher) {
this.publisher = publisher;
}
public void publish() {
MyCustomEvent event = new MyCustomEvent(this);
publisher.publishEvent(event); // Fires the event
}
}
@Component
public class MyCustomEventListener {
@EventListener
public void handleMyCustomEvent(MyCustomEvent event) {
System.out.println("Handling event: " + event);
}
}
In Spring Boot, everything happens in a more streamlined manner. Setup is generally less verbose, with powerful annotations that simplify your code.
Micronaut
Micronaut is another lightweight framework that excels in building microservices. It has built-in support for AOP (Aspect-Oriented Programming), dependency injection, and event-driven architectures, combining simplicity and performance.
Here's how you can create an event listener in Micronaut:
import io.micronaut.context.event.ApplicationEventListener;
import io.micronaut.runtime.event.ApplicationStartedEvent;
import jakarta.inject.Singleton;
@Singleton
public class StartupListener implements ApplicationEventListener<ApplicationStartedEvent> {
@Override
public void onApplicationEvent(ApplicationStartedEvent event) {
System.out.println("Application Started!");
}
}
In Micronaut, you can easily listen for application events with improved performance and without the complexity of Java EE.
Making the Transition
When considering moving from Java EE to lightweight alternatives, it’s essential to evaluate your application's requirements. Here’s a brief checklist to guide your transition:
-
Identify Critical Features: Make sure that the new framework supports all the critical features you need.
-
Assess Learning Curves: Determine if your team is prepared to adapt to the new framework or if additional training will be needed.
-
Build a Proof of Concept: Before fully committing, create a small proof of concept to ensure the framework meets your needs.
-
Review Community and Documentation: A strong community and clear, thorough documentation can make a significant difference in the development process.
Key Takeaways
Java EE 6 events provide developers with powerful abstractions and modular design principles. However, the shift toward lightweight alternatives reflects broader trends in software development, emphasizing simplicity, performance, and maintainability.
Whether you choose to stick with Java EE or explore frameworks like Spring Boot or Micronaut, understanding the full capabilities and limitations of these technologies will empower you to make informed decisions when building your applications.
For further reading on Spring Boot, check out the official Spring Boot Documentation, and for insights into Micronaut, visit Micronaut's Official Site.
By keeping this discussion relevant and engaging, we hope to motivate both new and experienced Java developers to consider alternatives that can simplify their coding experience while maintaining performance and scalability.
Feel free to ask questions or share your experiences with these frameworks in the comments below!
Checkout our other articles