Simplifying Jersey Tests: How to Mock SecurityContext
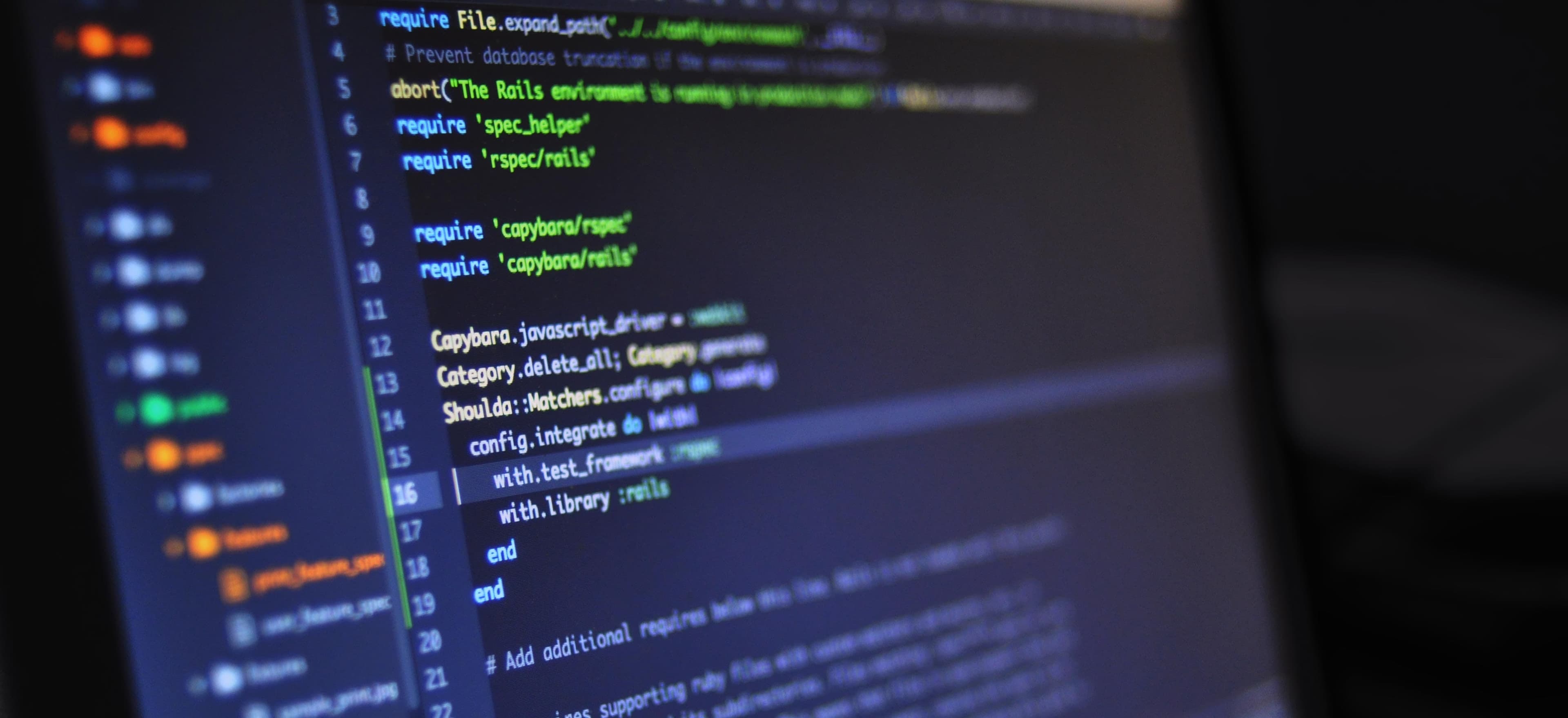
- Published on
Simplifying Jersey Tests: How to Mock SecurityContext
When developing RESTful web services with Jersey, a common requirement is to ensure that your application handles security contexts properly. Testing security in a Jersey application can often be cumbersome, especially when it comes to mocking the SecurityContext
. In this blog post, we will explore how to simplify your tests by effectively mocking the SecurityContext
in Jersey.
What is Jersey?
Jersey is a popular framework for developing RESTful web services in Java. It's built on top of the JAX-RS (Java API for RESTful Web Services) specification. With Jersey, you can easily create cohesive and scalable APIs with rich features like content negotiation and single-page applications.
Why Mock SecurityContext?
The SecurityContext
interface provides security-related information about the request and the current user. When testing, mocking the SecurityContext
helps mimic various user roles and permissions without needing an actual security framework. This approach allows for more controlled and reliable tests:
- Modularity: Tests can focus on business logic rather than security implementation.
- Performance: Mocking avoids the overhead associated with actual security checks.
- Variety: You can easily simulate different security scenarios.
Setting Up Your Jersey Testing Environment
Before diving into mocking, ensure you have the required libraries in your project. You will need Jersey dependencies and a testing framework, like JUnit. If you are using Maven, your pom.xml
might look like this:
<dependencies>
<!-- Jersey Core -->
<dependency>
<groupId>org.glassfish.jersey.containers</groupId>
<artifactId>jersey-container-servlet-core</artifactId>
<version>2.35</version>
</dependency>
<!-- JUnit for Testing -->
<dependency>
<groupId>junit</groupId>
<artifactId>junit</artifactId>
<version>4.13.2</version>
<scope>test</scope>
</dependency>
<!-- Mockito for Mocking -->
<dependency>
<groupId>org.mockito</groupId>
<artifactId>mockito-core</artifactId>
<version>3.11.2</version>
<scope>test</scope>
</dependency>
</dependencies>
Mocking the SecurityContext with Mockito
We choose Mockito as it simplifies the mocking process in Java applications significantly. Here is a simple way to mock the SecurityContext
.
Example Security Resource
Suppose you have a simple resource that returns user information based on the security context.
import javax.ws.rs.GET;
import javax.ws.rs.Path;
import javax.ws.rs.core.Context;
import javax.ws.rs.core.SecurityContext;
import javax.ws.rs.core.Response;
@Path("/user")
public class UserResource {
@GET
public Response getCurrentUser(@Context SecurityContext securityContext) {
String userName = securityContext.getUserPrincipal().getName();
return Response.ok("Current User: " + userName).build();
}
}
Writing the Test
Now, let's write a test that mocks the SecurityContext
.
import static org.mockito.Mockito.*;
import static org.junit.Assert.*;
import javax.ws.rs.core.Response;
import javax.ws.rs.core.SecurityContext;
import org.glassfish.jersey.server.ResourceConfig;
import org.glassfish.jersey.test.JerseyTest;
import org.junit.Test;
public class UserResourceTest extends JerseyTest {
@Override
protected Application configure() {
return new ResourceConfig(UserResource.class);
}
@Test
public void testGetCurrentUser() {
// Create a mock SecurityContext
SecurityContext securityContext = mock(SecurityContext.class);
Principal principal = mock(Principal.class);
// Stub the method to return a specific value when called
when(principal.getName()).thenReturn("testUser");
when(securityContext.getUserPrincipal()).thenReturn(principal);
// Activate the mocked SecurityContext
requestContext.setSecurityContext(securityContext);
// Make the API call
final Response response = target("/user").request().get();
// Validate the response
assertEquals(200, response.getStatus());
assertEquals("Current User: testUser", response.readEntity(String.class));
}
}
Code Breakdown
-
Mocking
SecurityContext
: We utilize Mockito to create a mockSecurityContext
and a mockPrincipal
. This allows us to simulate different user scenarios without external dependencies. -
Stubbing Behavior: Using
when...thenReturn
, we define the behavior of the mocked objects. Here, we specify what the principal will return when requested. -
Injecting the Mock: For the test to recognize the mocked
SecurityContext
, we set it up through the Jersey test environment. -
Assertions: We verify that our method behaves correctly by asserting the response status and checking the returned user information.
Additional Considerations
Testing Different Roles
You can extend the above mocking setup to test various user roles:
when(securityContext.isUserInRole("ADMIN")).thenReturn(true);
This enables you to test aspects of your business logic that are contingent upon user roles.
Using Annotations with Mocking
If you prefer annotations over manual mock setup, Mockito provides ways to manage this:
@RunWith(MockitoJUnitRunner.class)
public class UserResourceTest {
@Mock
SecurityContext securityContext;
...
}
The Closing Argument
Mocking the SecurityContext
in Jersey tests allows developers to simplify their testing strategy. This not only promotes better test coverage but also enhances modularity and facilitates different security scenarios. By using tools like Mockito, it becomes even easier to simulate various user interactions seamlessly.
With the examples provided, you should now have a strong foundation for implementing and testing security contexts in your Jersey applications. For a more extensive guide on Jersey, consider visiting the Jersey Documentation.
Happy coding! If you have any further questions or suggestions, feel free to share them in the comments below!
Checkout our other articles