How to Troubleshoot Write Failures in Java Memory Mapped Files
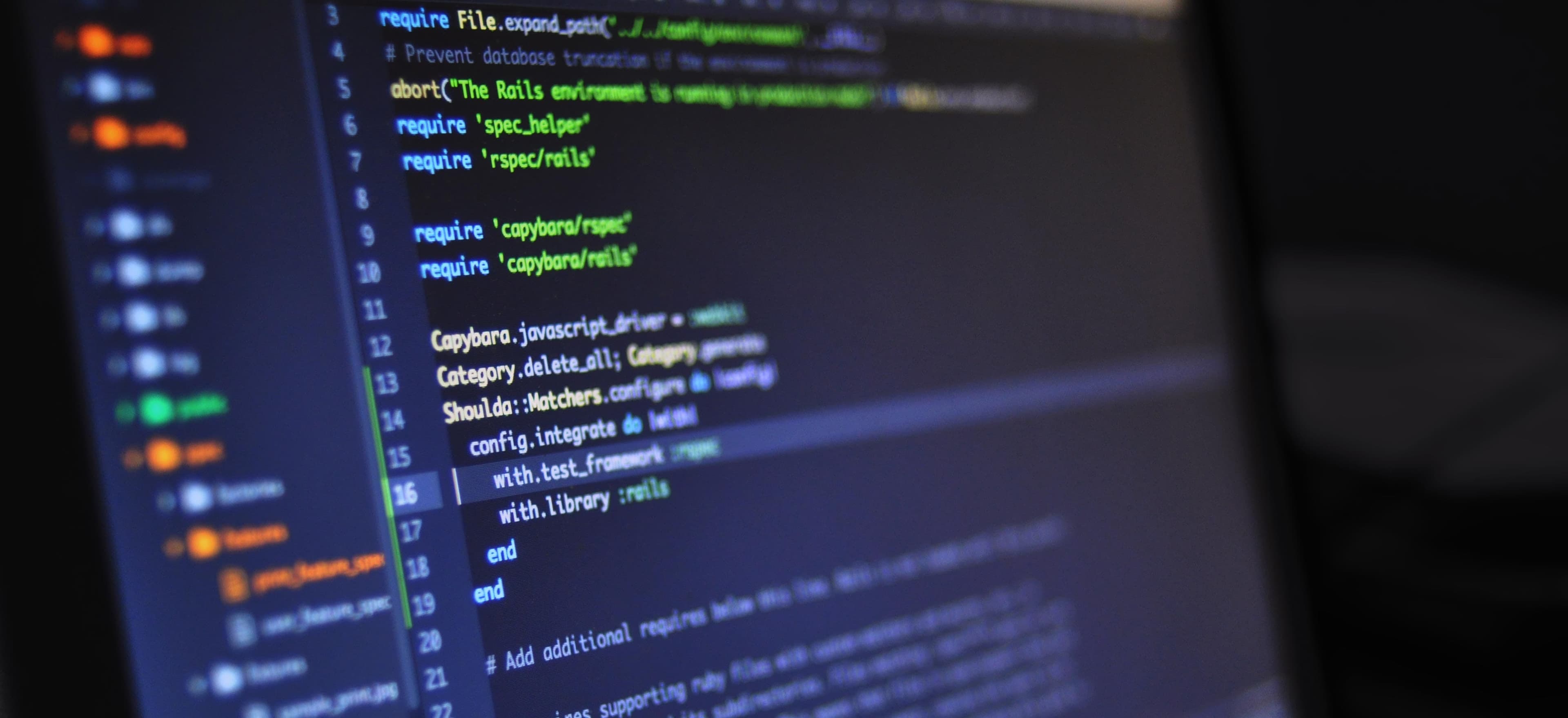
- Published on
How to Troubleshoot Write Failures in Java Memory Mapped Files
Memory-mapped files in Java offer a powerful way to read and write to files using the operating system's virtual memory capabilities. They can enhance performance and enable more efficient file I/O operations. However, as with all powerful tools, they can present significant challenges when something goes wrong, especially with write operations. In this blog post, we will explore troubleshooting techniques for write failures in Java memory-mapped files.
What are Memory Mapped Files?
Memory-mapped files allow a file to be mapped into the memory space of a Java application. This approach bypasses conventional I/O methods, enabling applications to interact with files through memory accesses. The java.nio
package provides the functionality to implement memory-mapped files effectively.
Example Code - Creating a Memory-Mapped File
import java.io.RandomAccessFile;
import java.nio.MappedByteBuffer;
import java.nio.channels.FileChannel;
public class MemoryMappedFileExample {
private static final String FILE_PATH = "example.txt";
public static void main(String[] args) {
try (RandomAccessFile memoryMappedFile = new RandomAccessFile(FILE_PATH, "rw")) {
FileChannel fileChannel = memoryMappedFile.getChannel();
MappedByteBuffer mappedBuffer =
fileChannel.map(FileChannel.MapMode.READ_WRITE, 0, fileChannel.size());
// Writing data to the mapped buffer
String data = "Hello, Memory Mapped Files!";
mappedBuffer.put(data.getBytes());
// Ensure data is written to file
mappedBuffer.force();
System.out.println("Data written successfully.");
} catch (Exception e) {
e.printStackTrace();
}
}
}
Understanding the Code
- File Access: We start by opening a
RandomAccessFile
in read/write mode to allow both reading from and writing to the underlying file. - Mapping to Buffer: Using
FileChannel
and themap
method, we create aMappedByteBuffer
that gives us the ability to read and write data directly. - Writing to Buffer: Data is written to the mapped buffer using the
put
method. - Forcing Updates: Call
force()
to ensure that the changes in the buffer are synchronized with the underlying file.
Hopefully, the above code demonstrates a straightforward method to create and write to a memory-mapped file. However, write failures can occur, and knowing how to troubleshoot these issues is essential.
Common Causes of Write Failures
1. Insufficient Disk Space
One of the first things to check is the available disk space. If the file system does not have adequate space, write operations will fail. Always ensure that there is enough space on the disk where the memory-mapped file resides.
2. File Permissions
Java may fail to write to a memory-mapped file if the file does not have the correct permissions. Ensure the application has write permissions on the file and the directory where the file is located.
3. Buffer Size Limitations
If you attempt to write more data to the mapped buffer than it can handle, an exception will be thrown. The size of the buffer must be managed carefully.
4. Garbage Collection
Java's garbage collector can interfere with memory-mapped file data if the mapped buffer is not referenced. If your application does not maintain a strong reference to the MappedByteBuffer
, the garbage collector may reclaim it, leading to write failures.
5. Operating System Resource Limits
Certain operating systems impose limits on the number of memory-mapped files that can be open at the same time. Frequently, issues may arise if the limit is reached. This is more common in older systems.
6. Concurrency Issues
When multiple threads attempt to write to the same memory-mapped file simultaneously, data corruption or failures can happen. Utilizing synchronization methods becomes crucial in a multi-threaded context.
Troubleshooting Steps
To effectively troubleshoot write failures in Java memory-mapped files, follow these steps:
Step 1: Logging
Incorporate extensive logging within your application to capture high-level errors and warnings related to I/O operations. Consider logging the specific exceptions caught during write operations.
try {
mappedBuffer.put(data.getBytes());
} catch (IOException e) {
System.err.println("I/O Error while writing to the buffer: " + e.getMessage());
}
Step 2: Check Disk Space and Permissions
Make sure to verify that the underlying disk has enough space. You can programmatically check the disk space, and also use command-line tools or system properties to verify file permissions.
Step 3: Validate Buffer Size
After mapping the file, ensure that the data being written does not exceed the mapped buffer size:
if (mappedBuffer.remaining() < data.length()) {
System.err.println("Insufficient buffer space for writing data.");
}
Step 4: Maintain References
Keep a strong reference to the MappedByteBuffer
throughout its lifecycle. This will prevent the garbage collector from deallocating the buffer.
Step 5: Monitor System Limits
Be aware of your operating system's resource limits on mapped files. Monitor open files and adjust your application accordingly.
Step 6: Synchronize Access in Multithreading
When using multi-threading, ensure shared access to the memory-mapped file is managed properly using synchronization. You may use synchronized
blocks or ReentrantLock
to control access:
synchronized (mappedBuffer) {
mappedBuffer.put(data.getBytes());
}
Example of a Thread-Safe Memory-Mapped File Writer
Here's an example of how you might implement a thread-safe writer for memory-mapped files:
import java.io.RandomAccessFile;
import java.nio.MappedByteBuffer;
import java.nio.channels.FileChannel;
import java.util.concurrent.locks.ReentrantLock;
public class ThreadSafeMemoryMappedFileWriter {
private static final String FILE_PATH = "example.txt";
private final ReentrantLock lock = new ReentrantLock();
private MappedByteBuffer mappedBuffer;
public ThreadSafeMemoryMappedFileWriter() throws Exception {
RandomAccessFile memoryMappedFile = new RandomAccessFile(FILE_PATH, "rw");
FileChannel fileChannel = memoryMappedFile.getChannel();
mappedBuffer = fileChannel.map(FileChannel.MapMode.READ_WRITE, 0, fileChannel.size());
}
public void writeData(String data) {
lock.lock();
try {
if (mappedBuffer.remaining() >= data.length()) {
mappedBuffer.put(data.getBytes());
mappedBuffer.force(); // Ensure data is flushed to the file
} else {
System.err.println("Not enough space in mapped buffer to write data.");
}
} finally {
lock.unlock();
}
}
}
Why This Matters
By using a locking mechanism, you ensure that only one thread can write to the mapped buffer at a time. This minimizes the risk of data corruption and enhances the overall reliability of your memory-mapped file operations.
To Wrap Things Up
Memory-mapped files present an efficient way to handle file I/O in Java, but they come with their own set of challenges. By understanding potential write failures and applying effective troubleshooting steps, you can streamline your application and enhance its reliability.
For further reading, you might find these articles useful:
- Java NIO: A Quick Overview
- Multi-threading in Java
Incorporate these strategies into your workflow, and troubleshooting write failures in Java memory-mapped files will become a manageable task rather than a daunting challenge. Happy coding!
Checkout our other articles