Overcoming Configuration Challenges with Spring Cloud Sidecar
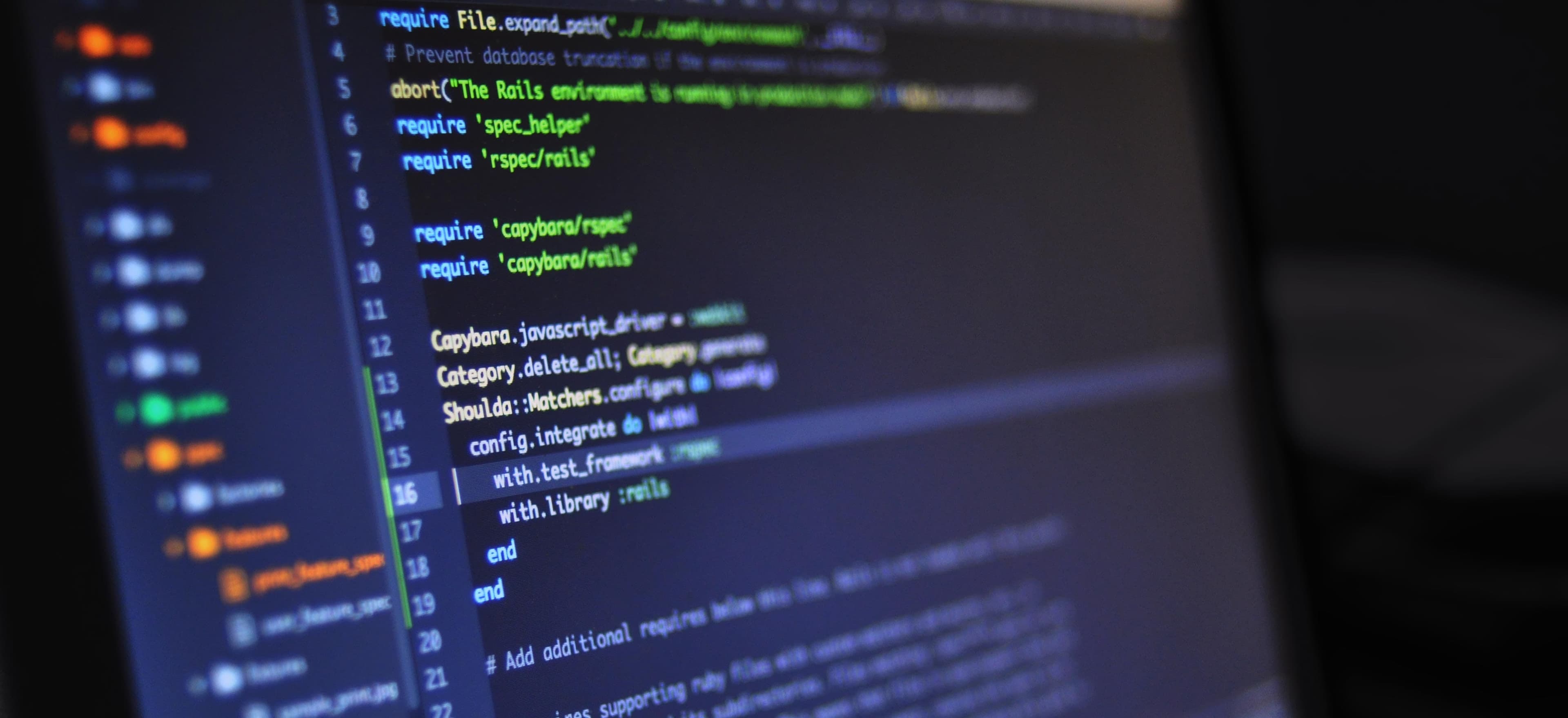
- Published on
Overcoming Configuration Challenges with Spring Cloud Sidecar
In the modern microservices architecture, managing configurations becomes a monumental challenge. Spring Cloud provides a solution with its Sidecar component, facilitating communication between the various microservices seamlessly. In this blog post, we will delve into the configuration challenges faced in microservices and how Spring Cloud Sidecar can help you navigate these hurdles.
Understanding Microservices and Configuration Challenges
Microservices architecture allows you to develop, deploy, and scale your applications independently. However, this autonomy comes with unique configuration challenges, such as:
- Service Discovery: Each service needs to locate other services dynamically as they scale.
- Centralized Configuration: Storing configuration properties in a centralized location is crucial for managing multiple services effectively.
- Load Balancing and Fault Tolerance: Distributing traffic among service instances while maintaining high availability is a primary consideration.
To address these challenges, Spring Cloud offers tools like Eureka for service discovery, Config Server for centralized configuration, and Ribbon for load balancing, among others. However, relying on individual components can be cumbersome.
Enter Spring Cloud Sidecar
Spring Cloud Sidecar complements these services by enabling non-Java applications to benefit from the Spring Cloud ecosystem. It's particularly useful for integrating legacy systems with modern architectures seamlessly.
Setting Up Spring Cloud Sidecar
Dependencies for Your Project
To start using Spring Cloud Sidecar, you must include the necessary dependencies in your Maven pom.xml
or Gradle build.gradle
. This way, your application can leverage the full capabilities of Spring Boot along with Sidecar components.
Maven Example
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-sidecar</artifactId>
</dependency>
Gradle Example
implementation 'org.springframework.cloud:spring-cloud-starter-sidecar'
Configuration
Once your dependencies are in place, the next step is to configure the application. The Sidecar service essentially acts as a proxy that sits alongside your microservice, providing functionality such as health checks, routing, and communication facilitation.
Sample Configuration
Here is an example of how you can set up your application properties for Sidecar:
server:
port: 8080
spring:
application:
name: my-sidecar-service
spring-cloud:
sidecar:
service-id: my-legacy-service
prefix: http://localhost:8081
In this configuration, service-id
is the identifier for your legacy service, while prefix
provides the URL that the Sidecar will proxy traffic through.
Key Features of Spring Cloud Sidecar
- Health Checks: It can monitor the health of your service endpoints automatically.
- Routing: Sidecar routes requests to the corresponding service, effectively abstracting service discovery.
- Client-side load balancing: Built-in capabilities facilitate distributing loads among multiple service instances.
These features are critical for maintaining an efficient, fault-tolerant microservices architecture while interfacing with legacy systems.
Implementing Sidecar with Code
Let’s dive deeper with an example that demonstrates how Spring Cloud Sidecar manages communication with a legacy system.
Sample Code
Here’s a minimal code example illustrating the setup:
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.cloud.netflix.sidecar.EnableSidecar;
@SpringBootApplication
@EnableSidecar
public class SidecarApplication {
public static void main(String[] args) {
SpringApplication.run(SidecarApplication.class, args);
}
}
Explanation
- @SpringBootApplication: This annotation indicates that it's a Spring Boot application.
- @EnableSidecar: This annotation is a crucial component that enables Sidecar functionality.
In the above example, when the application starts, it initializes the Sidecar, which listens for requests and forwards them to the legacy services.
Troubleshooting Common Configuration Issues
Despite the utility of Spring Cloud Sidecar, configurations can sometimes falter. Here are some common issues and how to tackle them:
1. Service Not Found
If you encounter errors indicating that your service cannot be found, check:
- Is the service you are trying to reach registered correctly with Eureka?
- Are the URLs in the configuration files formatted correctly?
2. Health Checks Failure
If health checks are failing, examine:
- Are your services healthy and responsive?
- Is the specified health check endpoint correctly configured?
Integrating with Other Spring Cloud Components
Spring Cloud Sidecar doesn't exist in a vacuum. It’s essential to integrate it with other components for an even richer functionality. For instance, integrating with Spring Cloud Config can centralize your configuration management.
Sample Code for Integration
Here’s an example configuration that showcases integration with Spring Cloud Config:
spring:
application:
name: my-sidecar-service
cloud:
config:
uri: http://localhost:8888 # Config Server URI
Benefits of This Integration
Harnessing the power of Spring Cloud Config alongside Spring Cloud Sidecar allows for:
- Centralized Management: All configuration properties for your microservices can be updated in one place.
- Version Control: Configuration can be versioned, ensuring that changes can be audited and rolled back more effectively.
For a deep dive into Spring Cloud Config, check the official documentation.
Wrapping Up
Spring Cloud Sidecar is a powerful tool for managing configuration challenges in microservices architectures. By acting as an intermediary between legacy systems and modern services, it allows for seamless integration and communication while enhancing resilience and performance.
By following the steps outlined in this post, you can set up Spring Cloud Sidecar, tackle configuration issues, and leverage it effectively alongside other Spring Cloud components.
Ensure to explore Spring Cloud’s features further to fully grasp the benefits it brings to microservices architecture.
Next Steps
- Experiment with your own microservices setup utilizing Spring Cloud Sidecar.
- Monitor and analyze your system's performance as you refine and enhance your architectural decisions.
With this knowledge at your fingertips, you are well on your way to effectively overcoming configuration challenges in your microservices landscape. Happy coding!