Overcoming COBOL to Java Migration Challenges
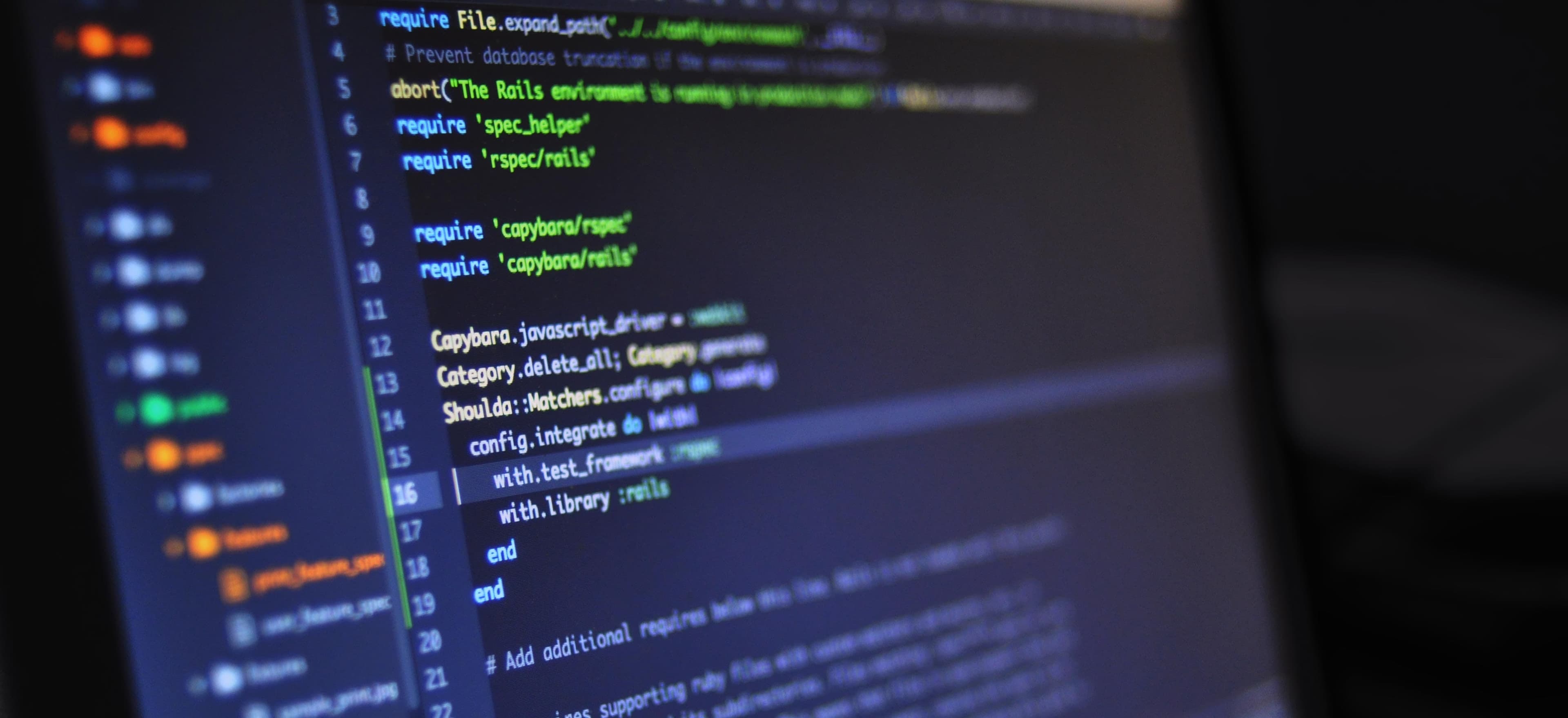
- Published on
Overcoming COBOL to Java Migration Challenges
In today's technology-driven world, many enterprises are looking at migrating their legacy systems, especially those built on COBOL, to modern programming languages like Java. While this transition can bring numerous benefits including improved performance, easier maintenance, and better integration with modern technologies, it does come with its own set of challenges. In this blog post, we will explore these challenges and provide strategies to effectively overcome them.
Understanding the COBOL Legacy
COBOL (Common Business-Oriented Language) has been prevalent since the 1960s, primarily used for business, finance, and administrative systems. While it has stood the test of time and continues to operate critical systems in many organizations, its structure can lead to complications during migration.
Why Migrate from COBOL to Java?
-
Modernization Needs: Legacy systems often lack compatibility with modern web services and cloud technologies. Transitioning to Java allows organizations to leverage new features and frameworks.
-
Talent Acquisition: Finding COBOL programmers is becoming increasingly difficult. Java, being one of the most popular programming languages, provides a wider pool of talent.
-
Cost Efficiency: Maintaining COBOL systems can be expensive. Java's extensive libraries and community support reduce development time and costs.
Key Challenges in COBOL to Java Migration
1. Code Complexity and Legacy Handcrafted Logic
COBOL applications mirror business logic that has evolved over decades. This complexity can be daunting when migrating to Java.
Solution: Break down the application into smaller, manageable modules. Create a detailed documentation of the existing COBOL logic. A collaborative approach with business analysts and domain experts will ensure no critical business rules are overlooked.
2. Data Structure Differences
COBOL uses a specific way to define data structures that might not have a direct equivalent in Java.
Solution: Extract mapping strategies that align COBOL data types with Java classes. For instance, COBOL's PIC
clauses can be mapped to Java's native types:
01 account-balance PIC 9(9)V99.
Java Equivalent:
private BigDecimal accountBalance; // BigDecimal handles precision better than float/double
Choosing BigDecimal
in Java is crucial for financial applications due to its high precision.
3. File Handling and Database Connectivity
COBOL relies heavily on sequential file processing and proprietary databases that necessitate a robust approach in Java.
Solution: Utilize Java's JDBC API for database connectivity. First, ensure that all data is migrated to a format compliant with modern databases (like MySQL or PostgreSQL).
Example Code Snippet:
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.ResultSet;
import java.sql.Statement;
public class DatabaseConnection {
public static void main(String[] args) {
String url = "jdbc:mysql://localhost:3306/mydatabase";
String user = "root";
String password = "password";
try (Connection conn = DriverManager.getConnection(url, user, password);
Statement stmt = conn.createStatement();
ResultSet rs = stmt.executeQuery("SELECT * FROM accounts")) {
while (rs.next()) {
System.out.println("Account ID: " + rs.getInt("id"));
System.out.println("Account Balance: " + rs.getBigDecimal("balance"));
}
} catch (Exception e) {
e.printStackTrace();
}
}
}
In this code snippet, a connection to a MySQL database is established. It is vital to replace legacy file interactions with efficient database queries.
4. Testing and Validation
Testing legacy COBOL systems can be problematic. Validating that the new system produces the same results is integral.
Solution: Implement a robust testing strategy that includes:
- Unit tests for individual components
- Integration tests to verify end-to-end functionality
- Regression tests to ensure that existing functionalities remain unaffected
Using Java testing frameworks like JUnit can facilitate this process:
import org.junit.Assert;
import org.junit.Test;
public class AccountTest {
@Test
public void testCalculateInterest() {
Account account = new Account();
account.setBalance(new BigDecimal("1000.00"));
BigDecimal interest = account.calculateInterest();
Assert.assertEquals(new BigDecimal("50.00"), interest); // assuming 5% interest rate
}
}
This snippet illustrates how to verify that the migrated function performs correctly. Implementing unit tests ensures the new system's reliability.
5. Change Management and Staff Training
Resistance to change is a natural human instinct. Employees accustomed to COBOL may find it challenging to adapt to Java.
Solution: Create comprehensive training programs for staff, highlighting Java’s advantages and new processes. Encourage dual-learning where COBOL and Java developers can collaborate.
Get support from platforms like Codecademy or Coursera, which offer Java courses tailored for varying levels.
In Conclusion, Here is What Matters: Navigating the Transition
Transitioning from COBOL to Java is not just a technical undertaking; it's a vital business decision that carries risk and opportunity. By breaking down the challenges methodically and approaching the migration as a collaborative project, businesses can drive modernization efforts successfully.
As with any transformational journey, maintaining a focus on understanding the legacy systems in-depth and adjusting both processes and personel's mindsets to the new technology is crucial. By fostering an environment of learning and adaptability, organizations not only overcome the challenges of migration but can also emerge more agile and prepared for future innovations.
Remember, every legacy is built on decades of knowledge and experiences. Respect that legacy as you propel your systems into the modern era. If you found this post helpful, consider subscribing to our blog for more insights on programming, technology trends, and best practices in software development!