Common Pitfalls in Unit Testing and How to Avoid Them
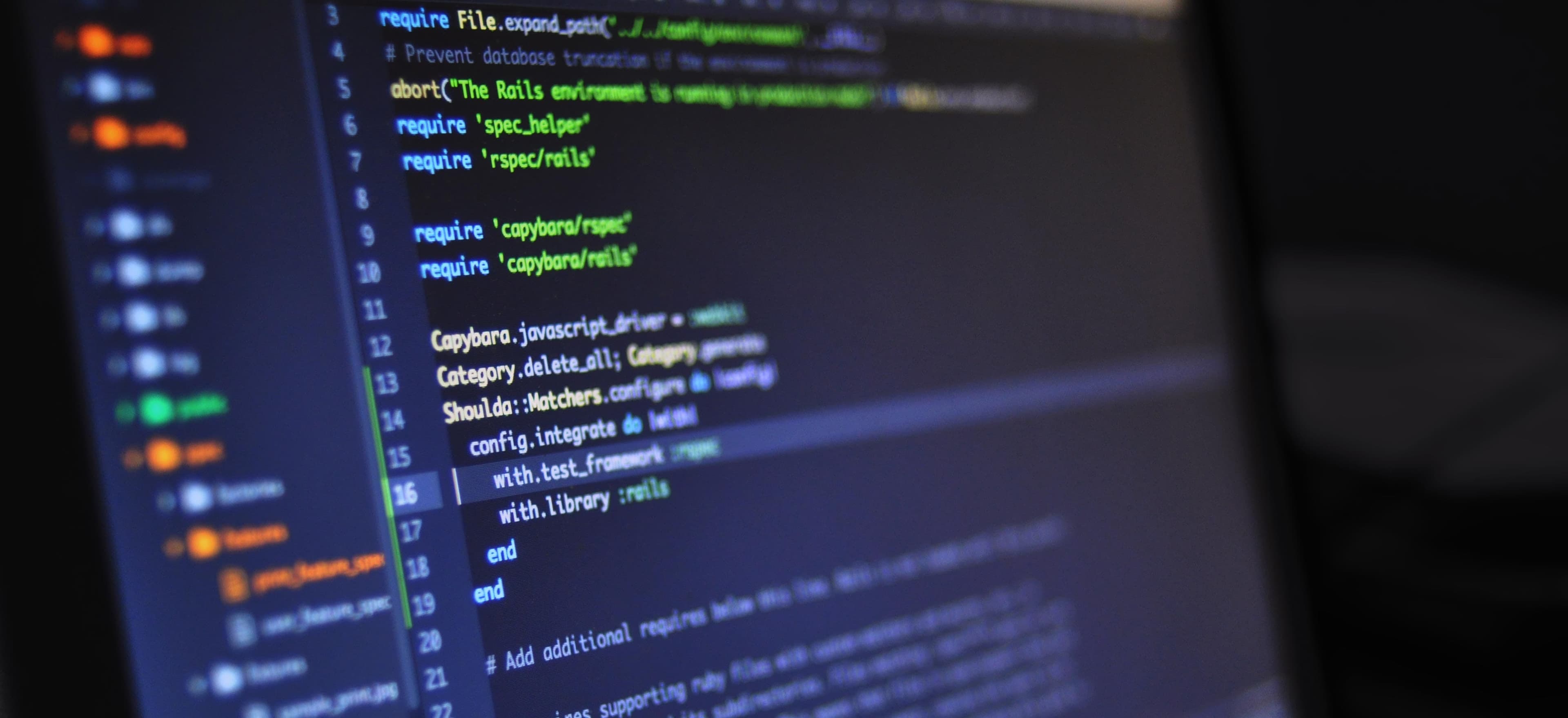
- Published on
Common Pitfalls in Unit Testing and How to Avoid Them
Unit testing is an essential practice in software development. It ensures the reliability of individual components and helps catch bugs early. However, there are several pitfalls that developers often encounter during the process. In this blog post, we will explore these common pitfalls and provide practical tips on how to avoid them. We'll also include some Java code snippets to illustrate best practices in unit testing.
Understanding Unit Testing
Before diving into the pitfalls, let’s briefly define what unit testing is. Unit tests are automated tests written and executed by software developers to ensure that a section of an application (the "unit") behaves as expected. Typically, these units consist of individual functions or classes in a programming language like Java.
Effective unit testing not only identifies bugs but also enhances code quality, improves design, and facilitates easier refactoring. However, the effectiveness of unit tests can be compromised by common mistakes.
Pitfall 1: Failing to Isolate Units
Why It Matters
One of the fundamental principles of unit testing is isolation. If your tests depend on external systems or states (like databases, APIs, etc.), they can become flaky and unreliable.
How to Avoid It
Use mocking frameworks to isolate units. In Java, you could use libraries like Mockito or PowerMock. Mocking allows you to simulate the behavior of complex objects, ensuring your tests focus only on the unit under examination.
Example Code:
import static org.mockito.Mockito.*;
public class UserServiceTest {
@Test
public void testGetUser() {
// Mock the UserRepository dependency
UserRepository mockRepo = mock(UserRepository.class);
// Set the behavior of the mock
when(mockRepo.findById(1)).thenReturn(new User("Alice"));
// Inject the mock into the service
UserService userService = new UserService(mockRepo);
// Act
User user = userService.getUser(1);
// Assert
assertEquals("Alice", user.getName());
}
}
In this example, UserRepository
is mocked, allowing us to test UserService
in isolation. This focuses the test on the behavior of UserService
, rather than the intricacies of UserRepository
.
Pitfall 2: Writing Tests with Poor Coverage
Why It Matters
High test coverage does not equate to good tests. Tests can be written that cover many lines of code without verifying the correct behavior of the application.
How to Avoid It
Adopt best practices for writing meaningful tests. Instead of just aiming for high coverage, focus on writing tests that validate critical business logic.
Example Code:
public class MathUtils {
public int divide(int a, int b) {
return a / b;
}
}
public class MathUtilsTest {
@Test(expected = ArithmeticException.class)
public void testDivideByZero() {
MathUtils utils = new MathUtils();
// Optimize critical edge case
utils.divide(10, 0);
}
}
Here, we are testing an essential edge case—division by zero. Rather than writing generic tests, we target specific, crucial scenarios to ensure robust functionality.
Pitfall 3: Lack of Consistency in Tests
Why It Matters
Inconsistent tests lead to confusion and can make debugging difficult. When unit tests fluctuate in their setup methods or naming conventions, the codebase becomes unmanageable.
How to Avoid It
Follow a consistent testing structure across your test classes. Use descriptive naming conventions for your test methods, and prepare utilities or base classes for common setup tasks.
Example Code:
public class CalculatorTest {
private Calculator calculator;
@Before
public void setUp() {
calculator = new Calculator();
}
@Test
public void testAddition() {
assertEquals(5, calculator.add(2, 3));
}
@Test
public void testSubtraction() {
assertEquals(1, calculator.subtract(3, 2));
}
}
In this example, the setUp
method ensures that the Calculator
instance is prepared before each test. Using clear and descriptive method names helps other developers to intuitively understand the purpose of each test.
Pitfall 4: Ignoring Test Failures
Why It Matters
Test failures are not just a nuisance—they signal underlying issues in your code. Ignoring them can lead to bugs creeping into your production environment.
How to Avoid It
Treat test failures seriously. Investigate failures immediately, and update your tests or production code accordingly. Implement Continuous Integration (CI) tools to automate testing and receive alerts about any broken tests immediately.
Setting Up CI
Popular CI tools like Jenkins, Travis CI, or GitHub Actions can seamlessly integrate testing into your workflow. Here's a brief overview of using GitHub Actions for Java:
- Create a
.github/workflows.yml
file in your repository. - Configure the workflow to run tests on Java build events:
name: Java CI
on:
push:
branches: [ main ]
pull_request:
branches: [ main ]
jobs:
build:
runs-on: ubuntu-latest
steps:
- name: Checkout repository
uses: actions/checkout@v2
- name: Set up JDK
uses: actions/setup-java@v1
with:
java-version: '11'
- name: Run tests
run: ./gradlew test
Pitfall 5: Not Reviewing Tests
Why It Matters
Just as you wouldn’t neglect code reviews, you shouldn’t overlook test reviews. Poorly written tests can lead to unreliable assertions and ultimately affect product quality.
How to Avoid It
Integrate test reviews into your regular code review process. Encourage team members to scrutinize tests just as they would critical logic in your production code.
Closing Remarks
Unit testing is a vital part of the software development lifecycle. However, common pitfalls can impede the effectiveness of your tests. By following the practices outlined in this article, you can improve the quality, reliability, and efficiency of your unit testing efforts.
For further reading on unit testing best practices, you might find Martin Fowler's article and JUnit documentation helpful. Adopting a disciplined approach to unit testing will pay off by producing robust, maintainable software, thus making development smoother and less error-prone. Happy testing!