Choosing the Right Java Testing Framework: Key Challenges
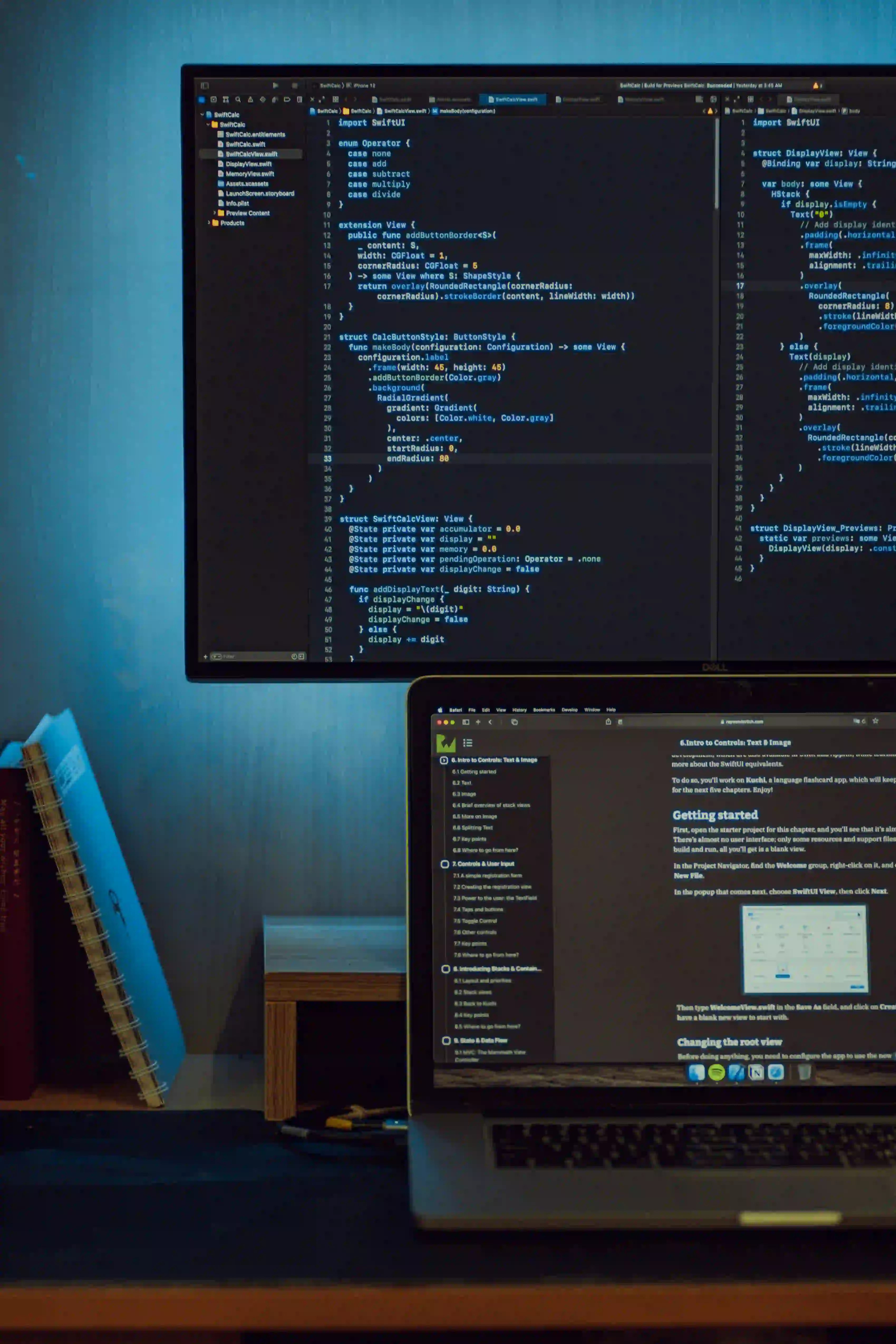
Choosing the Right Java Testing Framework: Key Challenges
In the fast-evolving world of software development, testing remains a crucial step to ensure quality and reliability. For Java developers, choosing the right testing framework can be daunting, given the plethora of options available. In this article, we’ll explore the primary challenges developers face when selecting a Java testing framework and provide insights to make an informed decision.
Why Testing Frameworks Matter
Testing frameworks provide the structure and tools needed to automate tests, ensuring that your code behaves as expected. They facilitate consistency, enhance collaboration, and ultimately help maintain code quality. The right framework can:
- Facilitate writing and organizing tests.
- Provide tools for assertions and coverage reporting.
- Support continuous integration and delivery.
So, how do we navigate through the maze of options? Let's dive into the key challenges.
Challenge 1: Variety of Testing Frameworks
Java offers various testing frameworks, each with unique features. Some of the notable frameworks include:
- JUnit: A well-established framework that is widely used in the Java community. JUnit is known for its simplicity and strong integration with most IDEs.
- TestNG: A versatile framework inspired by JUnit but designed to accommodate more complex testing requirements, such as dependency testing.
- Mockito: Primarily a mocking framework that integrates seamlessly with JUnit and TestNG.
Choosing one among many can be overwhelming. Understanding your project requirements is essential in this selection process.
Decision Factors
-
Nature of the Project: For simple projects, JUnit might suffice. For larger projects requiring extensive testing features, TestNG may be more appropriate.
-
Team Familiarity: If your team is more experienced with a particular framework, it may make sense to utilize that knowledge.
Challenge 2: Integration Capabilities
Integration with build tools and CI/CD pipelines significantly impacts how easily you can use a testing framework. A seamless integration process can save both time and effort.
Key Questions to Consider
- Does the framework integrate well with popular build tools like Maven or Gradle?
- Can it easily be integrated into CI tools such as Jenkins or GitLab CI?
For example, with JUnit, adding it to a Maven project is as simple as including the following dependency in your pom.xml
:
<dependency>
<groupId>junit</groupId>
<artifactId>junit</artifactId>
<version>4.13.2</version>
<scope>test</scope>
</dependency>
This seamless setup is why JUnit tends to be a go-to choice for many developers.
Challenge 3: Community Support and Documentation
No matter how powerful a framework is, it becomes impractical without robust community support and quality documentation. A well-supported framework can accelerate the learning curve and provide assistance when issues arise.
What to Look For:
- Active Community: Check for active forums, GitHub repositories, and user-contributed tutorials.
- Quality Documentation: Good documentation is vital. It should be clear, extensive, and provide examples.
For instance, the JUnit documentation serves as a benchmark for quality and comprehensiveness.
Challenge 4: Testing Type Requirements
Different projects have different testing needs. This could range from unit testing, integration testing, to acceptance testing.
Understanding Testing Types
- Unit Testing: Testing individual pieces of code in isolation. JUnit serves as a primary framework for unit testing.
- Integration Testing: Testing interactions between components. Here, TestNG can shine due to its advanced features for setting up tests with dependencies.
- Mocking: Mocking is especially crucial when testing components that depend on external systems. Frameworks like Mockito are invaluable in such scenarios.
How to Approach
Decide the type of testing that composes the majority of your project and align your framework choices accordingly.
Challenge 5: Performance and Speed
Time is always of the essence in software development. The speed at which tests run can affect development cycles significantly.
Considerations
- Framework Overhead: Evaluate the overhead introduced by the framework. Libraries like JUnit are lightweight and designed for quick execution.
- Parallel Testing: Frameworks like TestNG allow executing tests in parallel, which can significantly speed up the testing process in larger codebases.
Example
Consider the following TestNG configuration for parallel test execution.
<suite name="ParallelSuite" parallel="methods" thread-count="5">
<test name="Test1">
<classes>
<class name="com.example.TestClass1"/>
</classes>
</test>
<test name="Test2">
<classes>
<class name="com.example.TestClass2"/>
</classes>
</test>
</suite>
This would allow both tests to run simultaneously, thereby enhancing test execution speed.
Challenge 6: Evolving Project Needs
Software projects undergo continuous evolution. A testing framework that suits your needs today may not be adequate in the coming months or years.
Flexibility and Scalability
- Adaptability: Choose a framework that adapts easily to changing requirements.
- Extensibility: A framework should allow adding or integrating tools easily as your project grows.
Closing Remarks: Making the Right Choice
Choosing the right Java testing framework involves navigating through a labyrinth of options constrained by project requirements, team expertise, and your testing goals. There is no one-size-fits-all solution, but by understanding the key challenges outlined in this article, you'll be better prepared to make an informed decision.
As you evaluate frameworks, consider conducting small pilot tests to gauge usability and effectiveness relative to your project. Lastly, always keep an eye on community support and ongoing developments because the landscape of software testing continues to evolve.
For further reading on testing practices, check out Martin Fowler’s work on Testing.
By understanding these challenges and assessing frameworks according to your unique context, you can ensure your choice not only meets current needs but is also flexible enough for the future.
Happy testing!