Why You Should Avoid Overusing the Builder Pattern
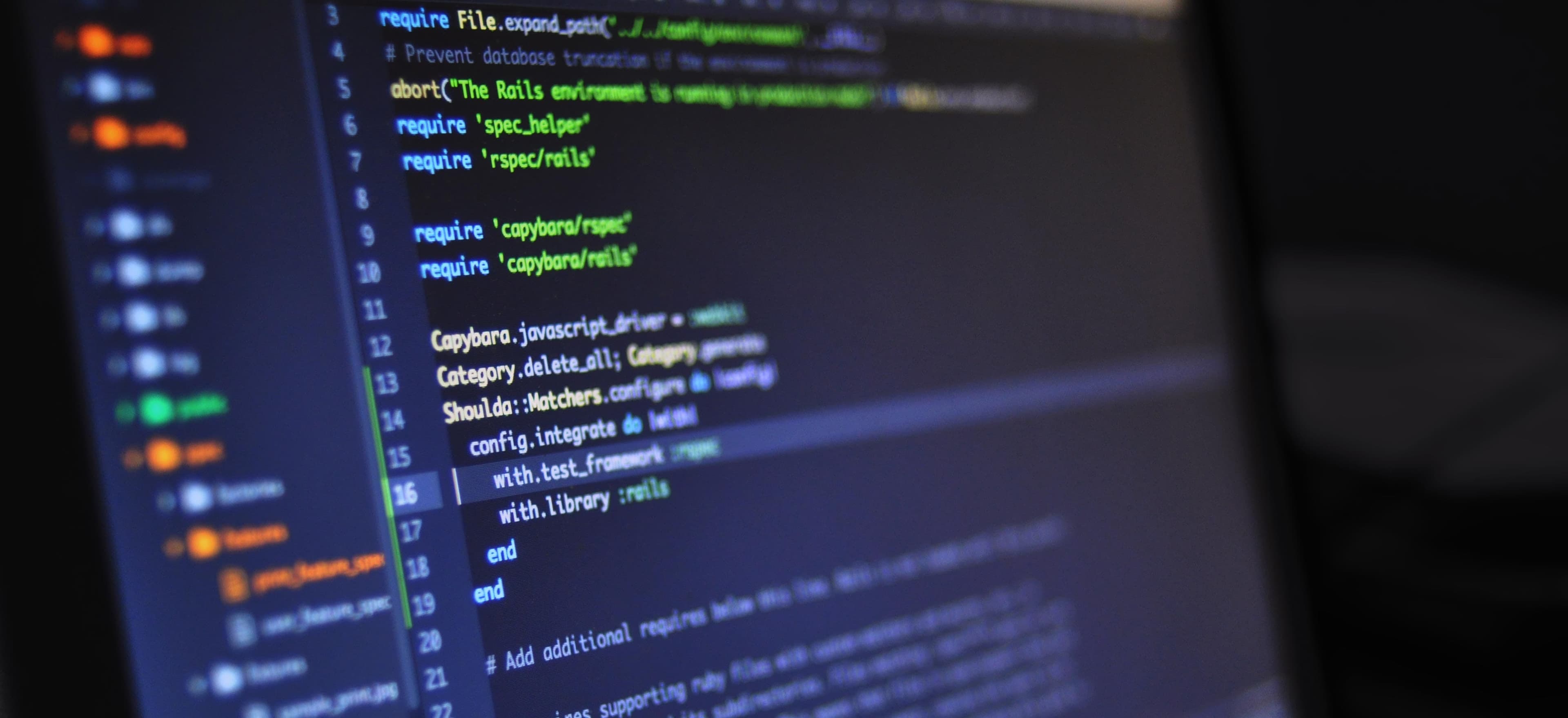
- Published on
Why You Should Avoid Overusing the Builder Pattern
The Builder Pattern is a popular design pattern in software engineering that aids in constructing complex objects step by step. It separates the construction of a complex object from its representation, allowing the same construction process to create different representations. While this pattern offers many advantages, such as enhanced readability and maintainability, it can also lead to over-engineering if misapplied. In this post, we will delve deeper into the Builder Pattern, examine its pros and cons, and ultimately discuss why one should avoid its overuse.
Understanding the Builder Pattern
Before we get into the negative aspects of overusing the Builder Pattern, it’s essential to grasp what it is. In Java, the Builder Pattern can be implemented as follows:
Example Code
public class Car {
private final String make;
private final String model;
private final int year;
private final String color;
private Car(CarBuilder builder) {
this.make = builder.make;
this.model = builder.model;
this.year = builder.year;
this.color = builder.color;
}
public static class CarBuilder {
private String make;
private String model;
private int year;
private String color;
public CarBuilder setMake(String make) {
this.make = make;
return this;
}
public CarBuilder setModel(String model) {
this.model = model;
return this;
}
public CarBuilder setYear(int year) {
this.year = year;
return this;
}
public CarBuilder setColor(String color) {
this.color = color;
return this;
}
public Car build() {
return new Car(this);
}
}
}
Commentary
In this example, we define a Car
class and a nested CarBuilder
class. The builder facilitates the object creation by providing methods to set each attribute. You can initiate building a car like this:
Car myCar = new Car.CarBuilder()
.setMake("Toyota")
.setModel("Corolla")
.setYear(2021)
.setColor("Blue")
.build();
This code is remarkably readable and accommodates optional parameters well. However, it’s crucial to be judicious when applying the Builder Pattern.
Pros of the Builder Pattern
-
Enhanced Readability: The pattern improves code readability. It makes your object construction logic clear by chaining method calls.
-
Immutable Objects: The Builder Pattern can help create immutable objects, making your code safer and more reliable.
-
Complex Object Creation: It excels at creating complex objects with many required and optional parameters. By separating the construction logic, you mitigate errors that may arise from constructors with multiple parameters.
-
Fluent Interface: The resulting code resembles natural language, improving overall understanding and maintenance.
Cons of the Builder Pattern
Conversely, overusing this pattern presents various drawbacks:
-
Over-Engineering: Not every object requires a Builder. For simple objects, using a builder can complicate the code unnecessarily. Using a constructor or a factory method might suffice.
-
Increased Boilerplate Code: Implementing a Builder for each class can lead to significant overhead in boilerplate code. This additional code can result in a higher maintenance burden.
-
Reduced Performance: Builders can introduce additional object creation and allocation overhead, which may impact performance, especially in critical code paths.
-
Inconsistent Use: Using the Builder Pattern inconsistently across your codebase can lead to confusion. A newcomer might find it challenging to identify when to expect a Builder and when not to.
-
Tight Coupling with Builder: The main class may become tightly coupled with its Builder. While this can be beneficial, it might inadvertently limit flexibility for future iterations or subclassing.
Best Practices for Implementing the Builder Pattern
While it is essential to be cautious about overusing the Builder Pattern, it’s equally important to implement it correctly when required. Here are some best practices:
-
Use When Necessary: Only apply the Builder Pattern for complex objects with many optional parameters or when immutability is critical.
-
Limit the Scope: If the number of parameters grows too large, consider if the class is trying to do too much. You may want to break the class apart into smaller, related classes.
-
Maintain Consistent Naming: Name your builder methods clearly to make it intuitive for users of the builder.
-
Document Your API: Provide documentation for the Builder Pattern in your code comments or external documentation. This will help future developers understand when and why to use the Builder.
-
Consider Alternatives: Think about using factory methods or static factory functions as alternatives for less complex cases. They may reduce boilerplate code while still allowing for clarity.
Code Example of a Simplified Factory Method
Instead of relying on a Builder in a simple case, consider the following static factory method approach:
public class Data {
private final String name;
private Data(String name) {
this.name = name;
}
public static Data of(String name) {
return new Data(name);
}
}
Commentary
In this case, the code is straightforward, concise, and does not introduce the additional complexity that a Builder would add. Simplicity often leads to easier maintenance and better code comprehension.
A Final Look
The Builder Pattern can be a powerful tool in your design toolkit, especially when dealing with complex object construction. However, its misuse can lead to unnecessarily complicated codebases and maintainability issues.
By implementing the Builder Pattern judiciously—reserved for complex scenarios—and considering alternatives for simpler cases, you can strike the right balance and create clean, maintainable, and efficient code. For a deeper understanding of Java design patterns, you might find the Refactoring Guru resource valuable.
Whether you choose to employ the Builder Pattern or opt for simpler alternatives, the paramount objective should always be clarity, maintainability, and a clean codebase. Remember, less is often more in software design.
Checkout our other articles