Overcoming Challenges in Testing Virtual Time with Reactor Core
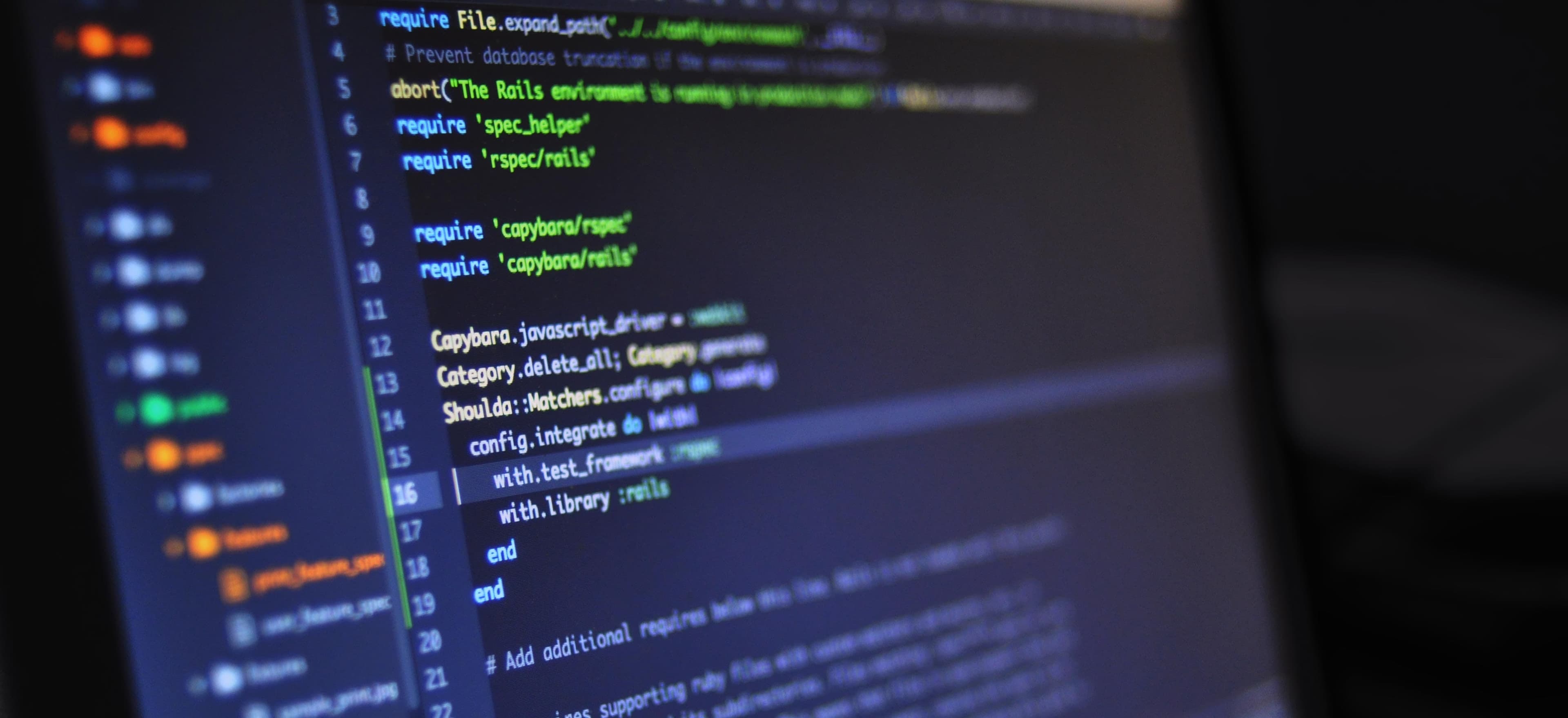
- Published on
Overcoming Challenges in Testing Virtual Time with Reactor Core
Reactive programming offers distinctive advantages for building asynchronous applications. With frameworks like Reactor Core, developers can handle events and data streams with unparalleled flexibility. However, working with time in a reactive world poses significant challenges, particularly when testing. In this post, we will delve into the complexities of virtual time in Reactor Core, discuss methodologies for effective testing, and provide practical examples to enhance your understanding of this crucial topic.
Understanding Reactor Core
Reactor Core is a foundational framework for building reactive applications on the JVM. It is part of the broader Project Reactor, which supports reactive programming through a set of powerful abstractions. The core building blocks of Reactor Core are:
- Flux: A reactive sequence that can emit zero or more items.
- Mono: A reactive sequence that can emit zero or one item.
These abstractions help manage asynchronous data flows. However, the management of time—especially in a unit-testing context—requires a nuanced understanding of Reactor's scheduling and timers.
The Challenge of Virtual Time
In reactive programming, virtual time refers to the ability to manage and manipulate time without relying on real-world clock ticks. The reactor framework provides an elegant solution for this through its Schedulers
and virtual time
features. However, testing with virtual time introduces complexities, especially when integrating with asynchronous flows.
Issues You May Encounter
- Non-deterministic Behavior: Asynchronous programming can lead to unpredictable outcomes, making it difficult to assert conditions in tests.
- Time Dependencies: When using timers and schedules, your tests may pass or fail based on the execution timing, leading to flaky tests.
- Mocking Time: Creating an environment where you can control the passage of time is non-trivial.
Strategies for Testing with Virtual Time
To overcome these challenges, there are several strategies you can employ while testing with Reactor Core:
1. Use VirtualTimeScheduler
Reactor provides a VirtualTimeScheduler
, which allows you to simulate the passage of time. By controlling the virtual clock, you can conduct your tests in a deterministic environment.
Code Snippet Example
import reactor.core.publisher.Flux;
import reactor.core.scheduler.VirtualTimeScheduler;
import java.time.Duration;
public class VirtualTimeExample {
public static void main(String[] args) {
VirtualTimeScheduler.getOrSet();
Flux<Long> flux = Flux.interval(Duration.ofSeconds(1))
.take(5);
// Simulating time passage
VirtualTimeScheduler.get().advanceTimeBy(Duration.ofSeconds(5));
flux.doOnNext(System.out::println)
.blockLast(); // ensure we wait for completion
}
}
Why This Works
In the above code, we first set up the VirtualTimeScheduler
. We create a Flux
that emits events every second. By advancing the virtual time by five seconds, we force the flux to emit all its values instantaneously, allowing us to test its output without waiting in real-time.
2. Proper Scheduling
When designing your reactive streams, ensure that you use appropriate scheduling to isolate your tests. This ensures that your tests are focused on the logic and less on timing.
Additional Code Example
import reactor.core.publisher.Flux;
import reactor.core.scheduler.Schedulers;
public class SchedulerExample {
public static void main(String[] args) {
Flux.range(1, 5)
.subscribeOn(Schedulers.parallel())
.map(i -> i * 2)
.subscribe(System.out::println);
// Create a sleep for main thread to ensure flush happens
try {
Thread.sleep(1000);
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
}
}
}
Why This Works
In this example, we utilize Schedulers.parallel()
to run the flux processing on a separate thread. This design allows us to simulate more realistic asynchronous behavior while keeping our tests isolated.
3. Utilize TestSchedulers
Reactor provides TestScheduler
, specifically designed for testing time-based operations. It offers methods to simulate time passing in a more granular and test-friendly manner.
Code Snippet Example
import reactor.core.publisher.Mono;
import reactor.test.scheduler.TestScheduler;
import java.time.Duration;
public class TestSchedulerExample {
public static void main(String[] args) {
TestScheduler testScheduler = new TestScheduler();
Mono<String> delayedMono = Mono.delay(Duration.ofSeconds(3), testScheduler)
.map(aLong -> "Hello, Reactor!");
testScheduler.advanceTimeBy(Duration.ofSeconds(3));
delayedMono.doOnNext(System.out::println).subscribe();
}
}
Why This Works
Here, TestScheduler
allows you to advance time programmatically. We schedule a delay for three seconds, and our test can successfully print the output once we simulate the time passage. This clarity in timing significantly reduces nondeterministic results.
4. Always Assert Outcomes
In testing, particularly when working with virtual time, it is crucial to always assert your outcomes meticulously. Use libraries like Reactor Test to provide structure and ease in assertions.
Lessons Learned
Testing with virtual time in Reactor Core might seem daunting initially. However, by leveraging tools like VirtualTimeScheduler
, TestScheduler
, and proper design patterns, you can effectively create a reliable testing strategy that accounts for time-based operations. This enables you to focus on developing robust applications without the overhead of flakiness in your tests.
For more advanced techniques and details on how to maximize Reactor Core's capabilities, consider checking out the Project Reactor documentation.
Happy coding!
Checkout our other articles