Stop Relying on DI Frameworks: They’re Hindering Your Code
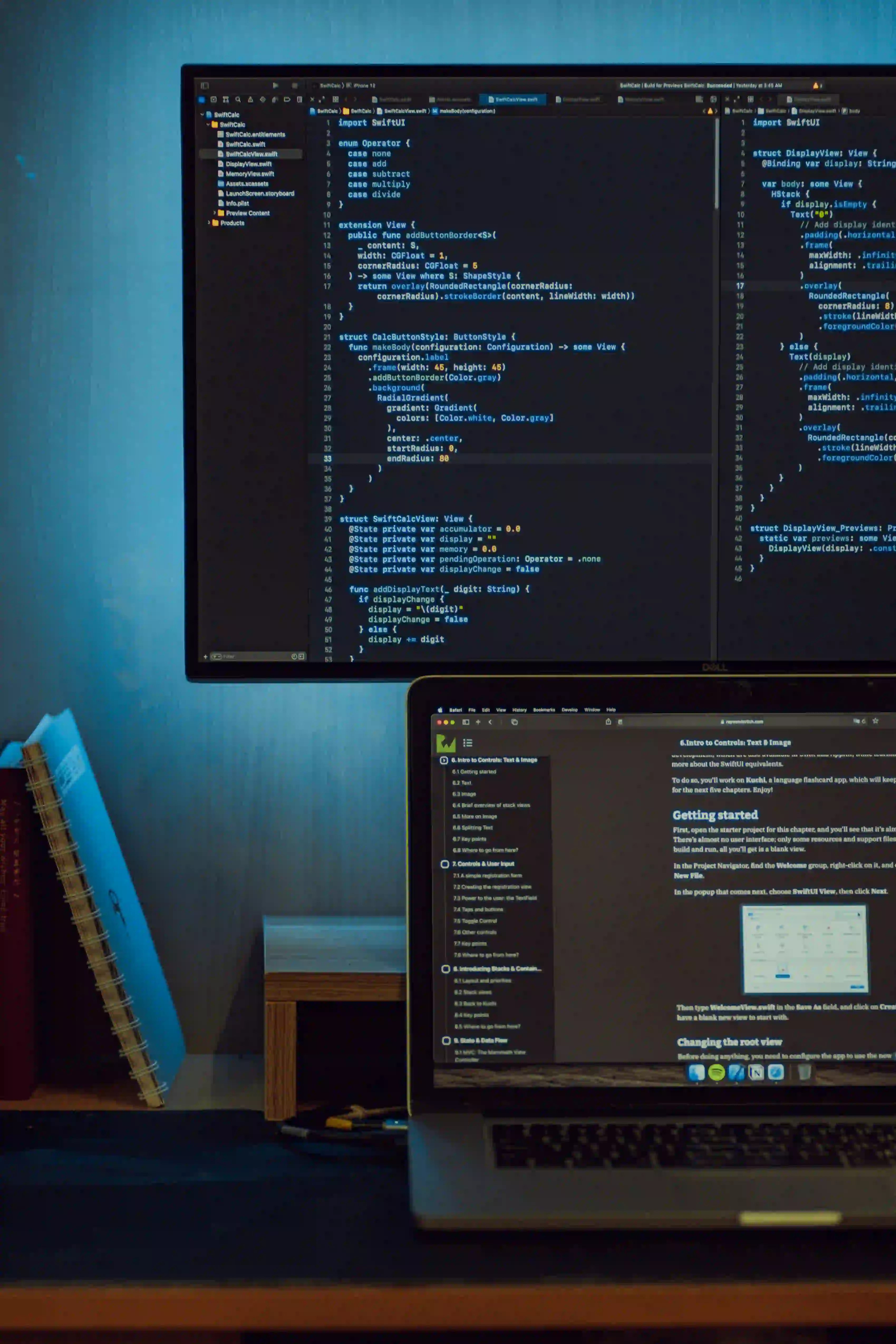
Stop Relying on DI Frameworks: They’re Hindering Your Code
Dependency Injection (DI) frameworks have become ubiquitous in Java development. While they provide a structured and often elegant way to handle dependencies, relying on them heavily can add a layer of complexity that may hinder your code's maintainability and understanding. In this blog post, we’ll delve into the reasons why you might reconsider your dependency injection framework usage and explore alternatives that can simplify your code structure.
Understanding Dependency Injection Frameworks
First, let’s clarify what we mean by Dependency Injection (DI). DI is a design pattern that allows a class to receive its dependencies from external sources rather than creating them internally. This approach encourages loose coupling and enhances code testability. Popular DI frameworks include Spring, Google Guice, and PicoContainer.
public class DatabaseService {
private final DatabaseConnection connection;
// Constructor injection
public DatabaseService(DatabaseConnection connection) {
this.connection = connection;
}
public void executeQuery(String query) {
connection.run(query);
}
}
In this example, the DatabaseService
class receives a DatabaseConnection
instance through its constructor. At first glance, this pattern promotes separation of concerns and makes it easier to test the service in isolation.
The Downsides of Heavy DI Usage
Increased Complexity
While DI frameworks do provide some conveniences, they often come with a steep learning curve and additional boilerplate code. For example, setting up a Spring application can involve lengthy XML configurations or complex annotations, leading to code that is hard to follow.
- Boilerplate Code: Boilerplate can clutter your codebase.
- Learning Curve: New developers face challenges getting accustomed to the intricacies of the framework.
Hidden Dependencies
When you abstract dependencies via a DI framework, it can become difficult to ascertain what dependencies a particular class requires just by looking at the code. For instance:
@Component
public class UserService {
@Autowired private UserRepository userRepository;
public User getUser(Long id) {
return userRepository.findById(id);
}
}
Here, the UserService
class conceals its reliance on UserRepository
using the @Autowired
annotation. This makes it harder for developers to ascertain what’s required for UserService
to function correctly.
Performance Overheads
DI frameworks often manage object lifecycle, proxying, and other advanced features. While they do optimize many operations, they can introduce unnecessary overhead, especially for larger applications where optimized performance is crucial.
- Startup Time: Application startup may become slower.
- Memory Usage: Some frameworks can lead to increased memory consumption.
Code Alternatives to DI Frameworks
While DI frameworks serve a purpose, there are simpler and often more efficient alternatives to manage dependencies in Java code.
Manual Dependency Injection
Manual DI simply involves creating and passing dependencies explicitly, giving you complete control. This approach can keep your code straightforward.
public class OrderService {
private final PaymentService paymentService;
// Manual dependency management
public OrderService() {
this.paymentService = new PaymentService();
}
public void processOrder(Order order) {
paymentService.process(order);
}
}
Advantages of Manual DI
- Explicitness: All dependencies are clear and presented upfront.
- Easy Testing: You can simply replace actual implementations with mocks or stubs.
Factory Pattern
The Factory Pattern can also provide a structured way of managing dependencies without relying on DI frameworks. A factory class can take care of the object creation logic, decoupling the instantiations from business logic.
public class ServiceFactory {
public static OrderService createOrderService() {
PaymentService paymentService = new PaymentService();
return new OrderService(paymentService);
}
}
// Usage
OrderService orderService = ServiceFactory.createOrderService();
This method retains the benefits of Dependency Injection while avoiding the complexities that frameworks introduce.
Service Locator Pattern
The Service Locator Pattern is another way to eliminate the need for DI frameworks while still allowing centralized management of dependencies. This pattern can help manage class dependencies effectively while reducing the number of coupling instances.
public class ServiceLocator {
private static final Map<Class<?>, Object> services = new HashMap<>();
public static <T> void registerService(Class<T> clazz, T service) {
services.put(clazz, service);
}
public static <T> T getService(Class<T> clazz) {
return clazz.cast(services.get(clazz));
}
}
// Registering a service
ServiceLocator.registerService(PaymentService.class, new PaymentService());
// Retrieving the service
PaymentService paymentService = ServiceLocator.getService(PaymentService.class);
Performance Benefits
- Reduced Overhead: No need for complex configuration.
- Explicit Management: Clear visibility of dependencies.
Final Considerations: Making Informed Choices
While DI frameworks can simplify some aspects of Java application development, they aren’t without their drawbacks. Relying heavily on these frameworks can lead to complexity, hidden dependencies, and performance issues. It’s essential to consider alternative methods for dependency management that maintain simplicity and enhance code clarity.
Whether you opt for manual DI, the Factory Pattern, or the Service Locator Pattern, the key is to ensure your code remains maintainable, testable, and easy for new team members to understand.
For further reading on Java design patterns, consider visiting Java Design Patterns or check out Effective Java for best practices in Java programming.
Armed with this knowledge, you can move forward confidently, making decisions that enhance your code quality and development efficiency. Happy coding!