Mastering Semantic File Merging in Java: Common Pitfalls
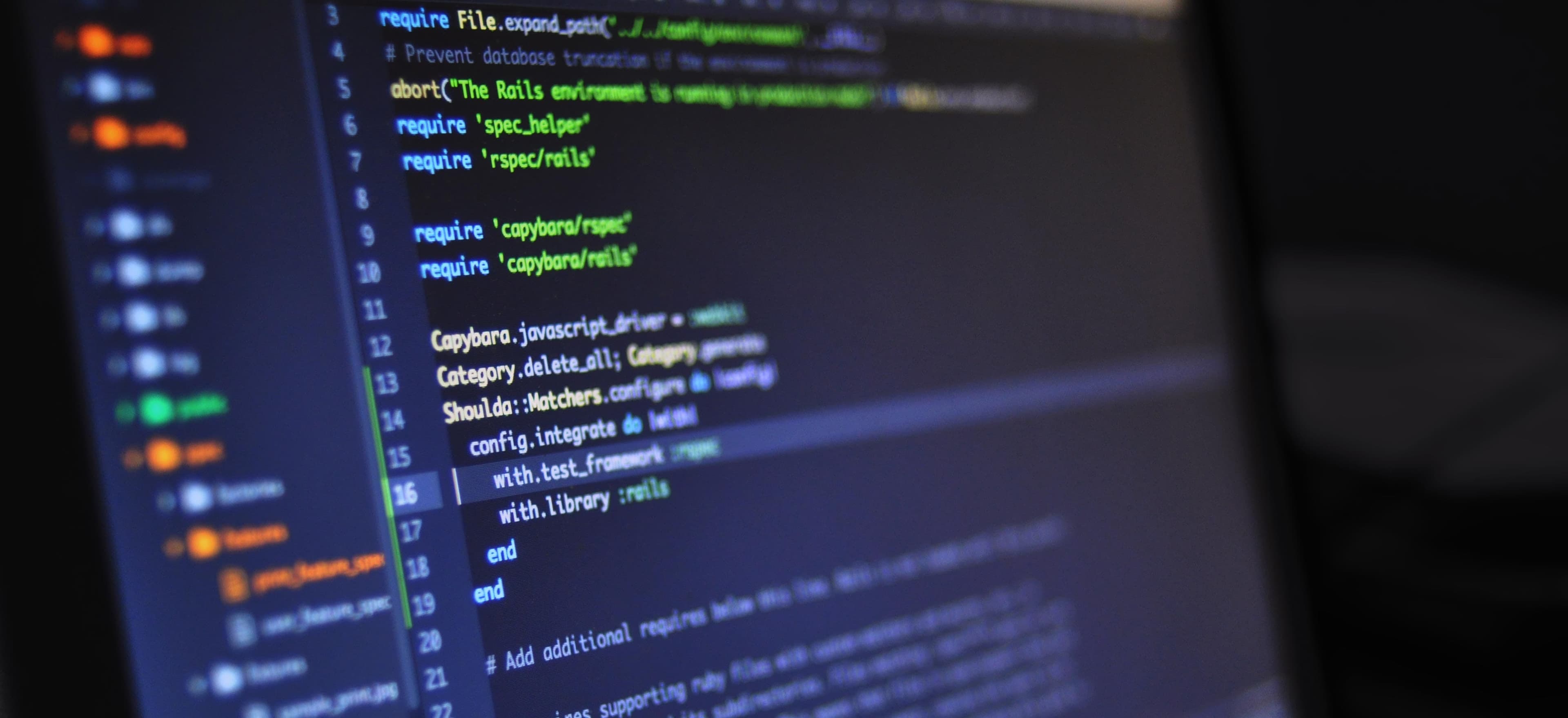
- Published on
Mastering Semantic File Merging in Java: Common Pitfalls
In the realm of software development, data management often presents intricate challenges. One such challenge is file merging, particularly when it comes to ensuring the merged configuration remains semantically correct. In this blog post, we will delve deep into the process of semantic file merging in Java, while highlighting common pitfalls developers face.
Understanding Semantic Merging
Semantic merging goes beyond simply concatenating files. It involves understanding the content, ensuring logical cohesion, and maintaining context and relationships within the data. For example, merging configuration files for an application requires that keys and values not only combine but also form a coherent overall structure.
Why Use Java for Semantic Merging?
Java provides a robust framework and tools for handling various file formats, including XML, JSON, and properties files. This ability to manage and merge different configurations makes it a popular choice for developers working on large-scale applications.
Key Libraries for File Merging in Java
- Jackson: For processing JSON content.
- Apache Commons Configuration: For merging properties files.
- JDOM: For handling XML files.
Setup and Dependencies
Before we begin exploring file merging, ensure you have the following libraries added to your project:
<dependency>
<groupId>com.fasterxml.jackson.core</groupId>
<artifactId>jackson-databind</artifactId>
<version>2.13.0</version>
</dependency>
<dependency>
<groupId>org.apache.commons</groupId>
<artifactId>commons-configuration2</artifactId>
<version>2.7</version>
</dependency>
<dependency>
<groupId>org.jdom</groupId>
<artifactId>jdom2</artifactId>
<version>2.0.6</version>
</dependency>
Common Pitfalls in Semantic File Merging
-
Ignoring Data Types
One common issue developers encounter is overlooking the data types of the values being merged. For instance, merging a numeric value with a string without proper conversion can lead to runtime errors.
// Example: Merging a string and an integer without conversion String value = "100"; int sum = 50 + Integer.parseInt(value); // Correct
-
Conflicting Keys
Merging files with conflicting keys can lead to unexpected behavior. For example, if two configuration files define the same key but with different values, determining which value to keep becomes a primary concern.
// Example: Handling conflicts by keeping the first value Map<String, String> config1 = new HashMap<>(); config1.put("timeout", "30"); Map<String, String> config2 = new HashMap<>(); config2.put("timeout", "60"); // Use a map to merge Map<String, String> mergedConfig = new HashMap<>(config1); mergedConfig.putAll(config2); // This keeps the value from config1
-
Loss of Hierarchical Structure
When merging hierarchical data formats like JSON or XML, preserving the structure is crucial. Flattening these formats without retaining their hierarchy can lead to data misrepresentation.
// Merging JSON objects while preserving hierarchy ObjectMapper mapper = new ObjectMapper(); JsonNode json1 = mapper.readTree(new File("file1.json")); JsonNode json2 = mapper.readTree(new File("file2.json")); JsonNode merged = JsonNodeMerger.merge(json1, json2); // Custom method to merge hierarchically
-
Neglecting Encoding Issues
Encoding problems can arise, especially when merging files from various sources. Always ensure that the encoding is consistent across all files to avoid data loss or corruption.
// Reading files with UTF-8 encoding BufferedReader reader = new BufferedReader(new InputStreamReader(new FileInputStream("file.txt"), StandardCharsets.UTF_8));
-
Not Validating Final Output
After merging files, validating the final output is essential to ensure it adheres to the required formats and logical constraints. Use schema validation for JSON and XML to automate the process.
// Example: Validate XML against a Schema SchemaFactory factory = SchemaFactory.newInstance(XMLConstants.W3C_XML_SCHEMA_NS_URI); Schema schema = factory.newSchema(new File("schema.xsd")); Validator validator = schema.newValidator(); validator.validate(new StreamSource(new File("merged.xml"))); // Throws exception if invalid
Implementing a Simple File Merger
To put together all that we've discussed, let’s create a simple file merger that handles JSON merging with conflict resolution based on the latest file.
import com.fasterxml.jackson.databind.JsonNode;
import com.fasterxml.jackson.databind.ObjectMapper;
import java.io.File;
import java.io.IOException;
import java.util.Iterator;
public class JsonFileMerger {
private final ObjectMapper mapper;
public JsonFileMerger() {
this.mapper = new ObjectMapper();
}
public JsonNode mergeFiles(File file1, File file2) throws IOException {
JsonNode json1 = mapper.readTree(file1);
JsonNode json2 = mapper.readTree(file2);
for (Iterator<String> it = json2.fieldNames(); it.hasNext(); ) {
String fieldName = it.next();
json1 = mergeNode(json1, fieldName, json2.get(fieldName));
}
return json1;
}
private JsonNode mergeNode(JsonNode original, String fieldName, JsonNode newValue) {
// Simple merging logic
if (original.has(fieldName)) {
return newValue; // Overwrite with new value
}
return original; // Keep existing value
}
public static void main(String[] args) {
JsonFileMerger merger = new JsonFileMerger();
try {
JsonNode result = merger.mergeFiles(new File("config1.json"), new File("config2.json"));
System.out.println(result.toPrettyString());
} catch (IOException e) {
e.printStackTrace();
}
}
}
Wrapping Up
In conclusion, semantic file merging in Java is a nuanced process. By being aware of common pitfalls such as data type mismatches, conflicting keys, loss of hierarchical structure, encoding issues, and failing to validate the final output, you can greatly enhance the reliability and correctness of your merges.
Armed with the right knowledge and tools, you can tackle your file merging tasks with confidence. Explore additional resources on JSON handling with Jackson and Apache Commons Configuration to deepen your understanding.
Happy merging!