Navigating Deprecated Features in Java EE 7
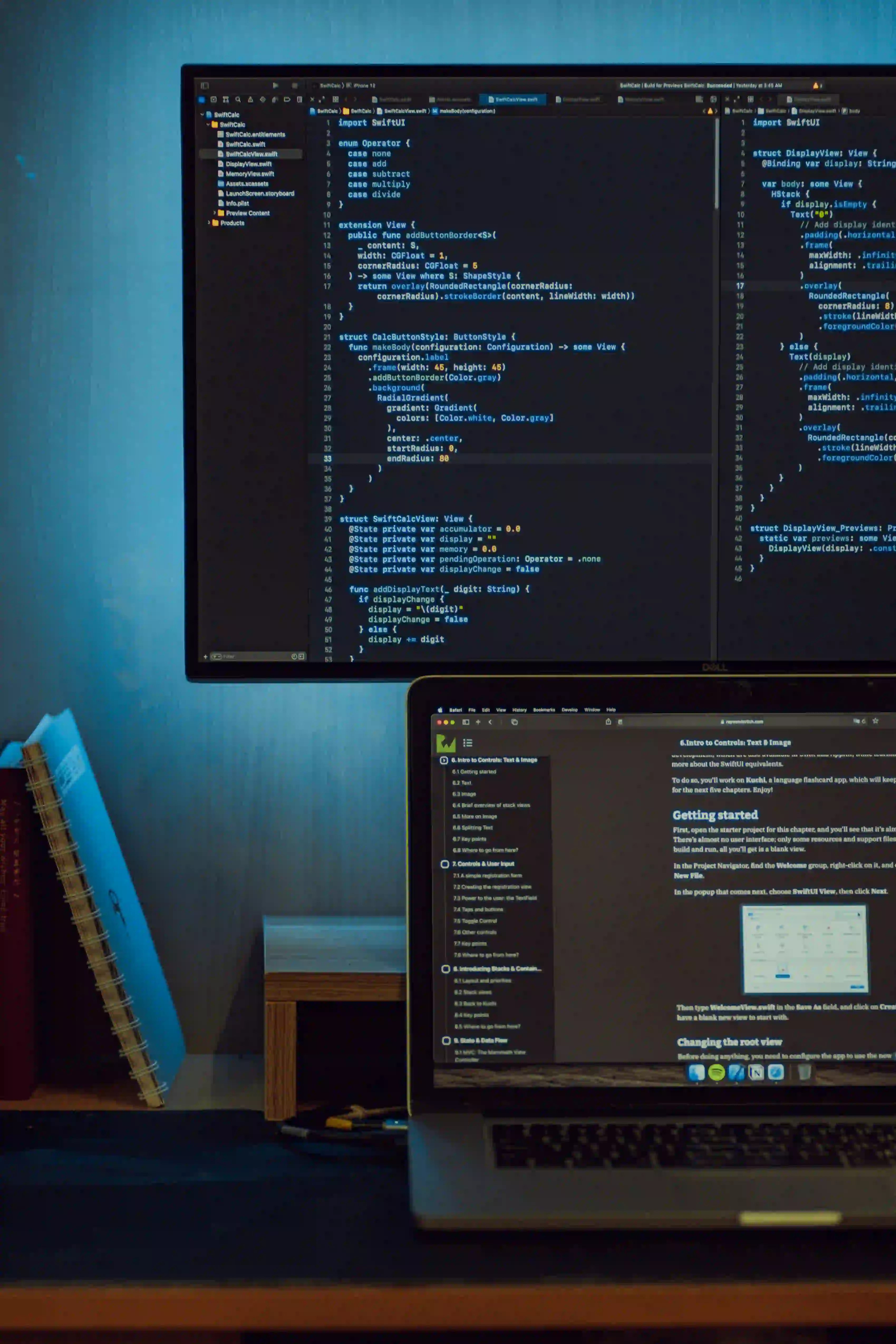
Navigating Deprecated Features in Java EE 7
Java EE (Enterprise Edition) 7 has introduced a plethora of advancements that modernized enterprise development. However, as with any evolving technology, some features have reached the end of their lifecycle. In this blog post, we will explore some of the key deprecated features in Java EE 7, the reasons behind their deprecation, and how to effectively navigate and replace them.
Understanding Deprecation in Java EE
What is Deprecation?
Deprecation is a process where old features or APIs are marked for future removal. The goal is to signal that developers should avoid using them in new applications. This is often due to a variety of reasons, such as:
- Improvements in technology: Newer APIs provide better performance or more features.
- Security vulnerabilities: Older methods may have undiscovered vulnerabilities that are not feasible to patch.
- Confusion among developers: Some APIs can lead to ambiguous code or excessive complexity.
Why Should You Care?
Ignoring deprecated features can lead to:
- Code that is hard to maintain: As deprecated features are likely to be removed in future Java releases, continuing to use them can result in legacy code that is difficult to upgrade.
- Security risks: Deprecated features are often less secure than their newer counterparts, making your application more vulnerable.
- Lack of community support: Using obsolete features can limit the help available from the developer community.
Key Deprecated Features in Java EE 7
Let's dive into some of the notable deprecated features and what you can use in their place.
1. EJB 2.x Stateless Session Beans
In Java EE 7, the EJB 2.x Stateless Session Beans are deprecated. The shift towards EJB 3.x introduced a more streamlined way to declare beans using annotations instead of XML.
Why is it Deprecated?
- Simplicity: EJB 3.x allows developers to write less code while increasing functionality.
- Annotation-based Configuration: This reduces the need for verbose XML configurations, making the development process more intuitive.
Replacement Code
If you are currently using EJB 2.x, you might have code that looks like this:
import javax.ejb.Stateless;
@Stateless
public class MyStatelessBean {
public void myMethod() {
// business logic here
}
}
Here, you can easily transition to the simpler EJB 3.x style:
import javax.ejb.Stateless;
@Stateless
public class MyStatelessBean {
public void myMethod() {
// business logic here
}
}
Notice the same concept applied differently. You no longer need to specify your beans in XML, thus garlanding productivity.
2. JAX-RPC
Java API for XML-based RPC (JAX-RPC) is a protocol used for web services development. In Java EE 7, JAX-RPC has been deprecated in favor of JAX-WS (Java API for XML Web Services).
Why is it Deprecated?
JAX-RPC was often cumbersome and verbose, which made it hard to work with compared to its successor. JAX-WS provides a more efficient and less error-prone way to call web services.
Replacement Code
If your code relies on JAX-RPC, you may see something like this:
import javax.xml.rpc.Stub;
import my.package.MyService;
MyService service = new MyServiceLocator();
Stub stub = (Stub) service.getMyServicePort();
stub.setUsername("user");
stub.setPassword("pass");
You can replace this with JAX-WS in a much cleaner manner:
import javax.xml.ws.Service;
import my.package.MyService;
MyService service = new MyService();
MyServicePort port = service.getMyServicePort();
port.doSomething("param");
This conversion is much more straightforward and less prone to errors.
3. Java EE 6’s Web Services API (JAX-WS and JAX-RS)
With Java EE 7, we see the introduction of new annotations and features for both JAX-WS and JAX-RS. Many features from the previous versions were marked as deprecated.
Why is They are Deprecated?
- Annotations: New, simplified annotations enhance readability.
- Enhanced Support: New versions provide better support for RESTful services, making it easier for modern web development paradigms.
Example
Instead of using older JAX-RS methods, switching to the newer way gives you this clarity:
import javax.ws.rs.GET;
import javax.ws.rs.Path;
import javax.ws.rs.Produces;
@Path("/example")
public class ExampleEndpoint {
@GET
@Produces("application/json")
public Response getExample() {
return Response.ok(new ExampleResponse()).build();
}
}
This code snippet clearly defines the REST endpoint without the clutter of older conventions.
Best Practices for Migrating from Deprecated Features
1. Read the Documentation
Always start by checking the official Java EE documentation to understand the alternatives and improvements.
2. Use Modern Tools and IDEs
Many modern IDEs such as IntelliJ IDEA or Eclipse can provide alerts to deprecated features and suggest alternatives. This can massively ease the transition from deprecated features.
3. Test Thoroughly
Migrating to new APIs does not only involve changing the code. It is essential to capture the expected behavior through thorough testing.
4. Update Dependencies
Ensure that libraries and dependencies are up to date to include support for Java EE 7 features, and remove dependencies on deprecated APIs.
Wrapping Up
Navigating deprecated features in Java EE 7 may seem daunting, but it is a crucial part of maintaining a robust, secure, and modern application. By understanding the reasons for deprecation and significantly adopting updated methods, developers can ensure that their Java applications remain efficient and manageable.
Stay informed, update your knowledge repository, and continue to adapt to the evolving Java landscape. For more insights into Java EE, consider checking out Java Brains for additional resources and tutorials. Happy coding!