Common Pitfalls in Android App UML Design
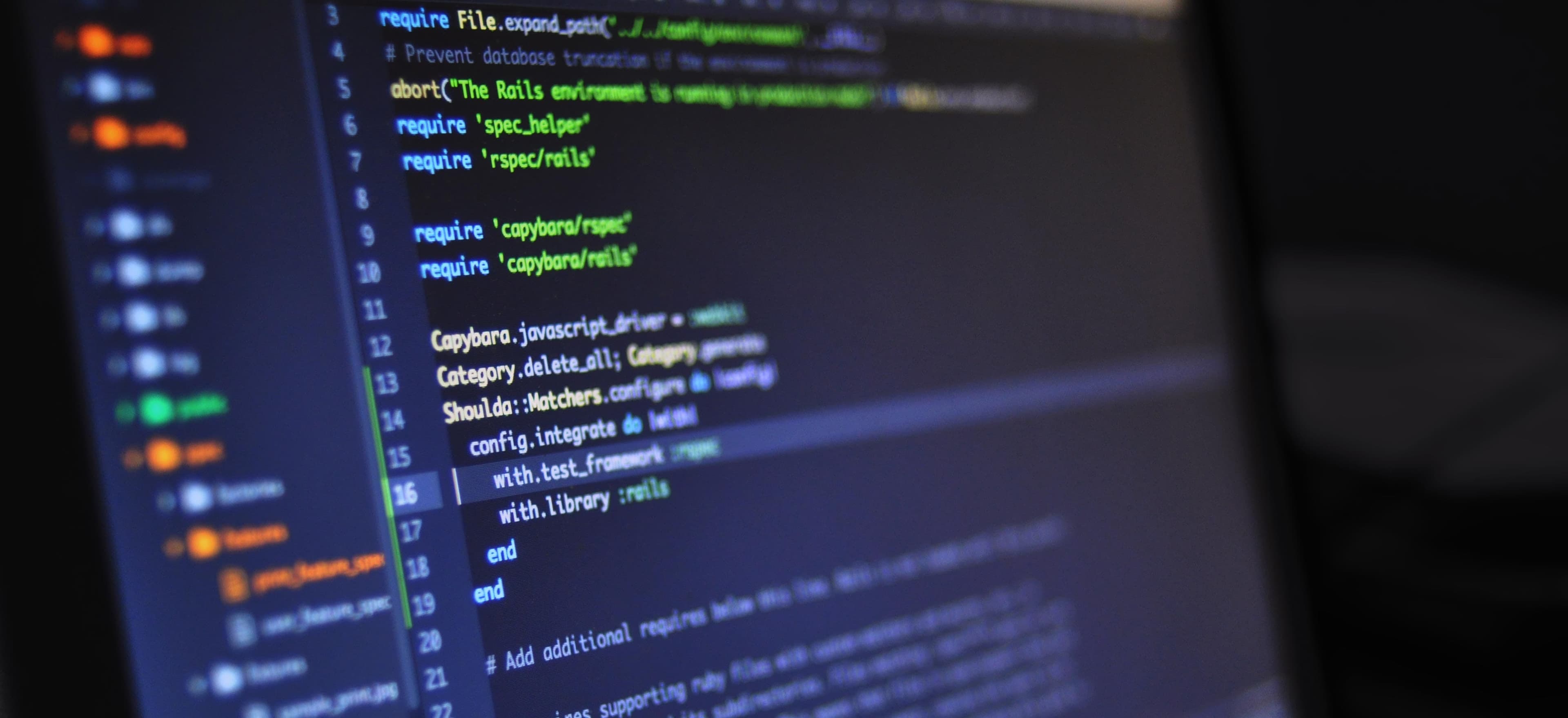
- Published on
Common Pitfalls in Android App UML Design
Unified Modeling Language (UML) is an essential part of the software engineering process. It helps to represent and design the structure and behavior of an application graphically. When it comes to Android app development, UML can streamline the design process, enhance communication among team members, and pave the way for easier implementation. However, there are common pitfalls developers may encounter when designing UML for Android applications. In this post, we will examine these pitfalls and how to avoid them to yield a more effective UML design.
Understanding UML in Android Development
Before diving into the pitfalls, it is crucial to understand how UML fits into Android app development. UML comprises various diagrams that depict different perspectives of a system. Some of the most relevant UML diagrams for Android development include:
- Class Diagrams: Represent the classes and their relationships.
- Sequence Diagrams: Illustrate how objects interact in a particular use case over time.
- Activity Diagrams: Present workflows and possible states of a system.
For an in-depth introduction to UML, the official UML specification is an excellent resource to familiarize yourself with the various diagram types.
The Pitfalls
1. Overcomplicating the Class Diagram
Common Mistake: Developers may attempt to model every detail of their classes, leading to overly complex class diagrams. This can confuse stakeholders and hinder effective communication.
How to Avoid: Focus on the core elements of your application. Begin with high-level classes and gradually add complexity. For example, use abstract classes and interfaces to represent common behavior without cluttering the diagram:
abstract class Animal {
abstract void sound();
}
class Dog extends Animal {
void sound() {
System.out.println("Bark");
}
}
class Cat extends Animal {
void sound() {
System.out.println("Meow");
}
}
In this example, instead of representing each pet class with all their properties, we’ve created a clean abstract structure that simplifies our class diagram.
2. Neglecting Relationships
Common Mistake: Failing to clearly illustrate relationships between classes can lead to ambiguity. This confusion can impact the development process and result in integration issues.
How to Avoid: Utilize UML association, aggregation, and composition to clarify relationships. For example, if you have an Order
class that relates to a Product
class, make sure to represent the relationship clearly:
Order ------<>---- Product
In this representation:
Order
composesProduct
, indicating ownership.
A clear design fosters better understanding among developers, making it easier to implement changes in the future.
3. Ignoring UI Interactions
Common Mistake: Developers often focus on the backend logic and neglect the user interface (UI) interactions. This narrow focus can create disconnects between design and implementation.
How to Avoid: Incorporate Activity Diagrams alongside class diagrams to visualize user interactions. This allows stakeholders to see how different components communicate, enhancing the overall comprehension of the system.
Consider the following activity diagram for a login process:
Start --> Enter Credentials --> Validate Credentials
--> [Valid] --> Access Home Screen
--> [Invalid] --> Show Error Message --> Retry or Exit
This diagram succinctly captures the user experience interaction, ensuring that the development team aligns with the intended design.
4. Underestimating the Importance of Documentation
Common Mistake: Developers might create UML diagrams but fail to document the design decisions behind them. This can hinder knowledge transfer and lead to assumptions that may not be correct.
How to Avoid: Supplement UML diagrams with detailed documentation. Record the rationale behind specific design choices, component interactions, and the overall structure of your application. This will become invaluable for onboarding new team members or revisiting the project after some time.
5. Neglecting Version Control
Common Mistake: Treating UML diagrams as static documents is a mistake many developers make. This can lead to outdated representations that do not accurately reflect the current state of the codebase.
How to Avoid: Use version control systems like Git to maintain UML diagrams alongside your codebase. Commit updates whenever significant changes are made to the application. This creates a historical trail that developers can refer back to when needed.
6. Not Iterating on the Design
Common Mistake: Some developers may believe that UML design is a one-and-done process. This mindset can result in overly rigid designs that do not adapt as requirements evolve.
How to Avoid: Treat UML design as a living document. Continuously review and update your diagrams as the project progresses. Agile methodologies emphasize iterative development, and your UML diagrams should reflect that.
Best Practices for Effective UML Design in Android
1. Use Tools for UML Creation
There are various tools available for drawing UML diagrams, such as Lucidchart, Visual Paradigm, and StarUML. Automated tools can help streamline the process, making it easier to make adjustments as your design evolves.
2. Collaborate with Stakeholders
Include all relevant stakeholders in the UML design process. Regular reviews can help identify inconsistencies and align everyone with the application vision. This collaboration leads to higher-quality designs and more cohesive development.
3. Simplify Where Possible
Aim for simplicity in your diagrams. Focus on essential classes, methods, and interactions. Less complexity means less room for misunderstanding.
4. Educate Your Team
Ensure that all team members comprehend the purpose of UML diagrams. Provide introductory resources or training sessions to improve familiarity with UML concepts. Well-informed team members foster better communication and collaboration.
The Closing Argument
Practicing effective UML design in Android app development can yield significant benefits, but it does come with its challenges. By avoiding common pitfalls such as overcomplicating diagrams, neglecting relationships, and underestimating documentation, you will be on your way to creating robust software architectures that are less prone to miscommunication and errors.
Adopting a collaborative, iterative approach will not only enhance the UML design process but also allow for a more flexible and resilient application architecture. Ultimately, prioritizing clarity and simplicity in your UML diagrams will lead to a more successful Android app development effort.
For further reading on UML and its impact on programming, check out the UML Distilled by Martin Fowler.
This concludes our exploration of common pitfalls in Android app UML design. Remember, UML is a tool; how you use it defines its effectiveness. Happy designing!