Unlocking JDK 11: Simplifying String Manipulation
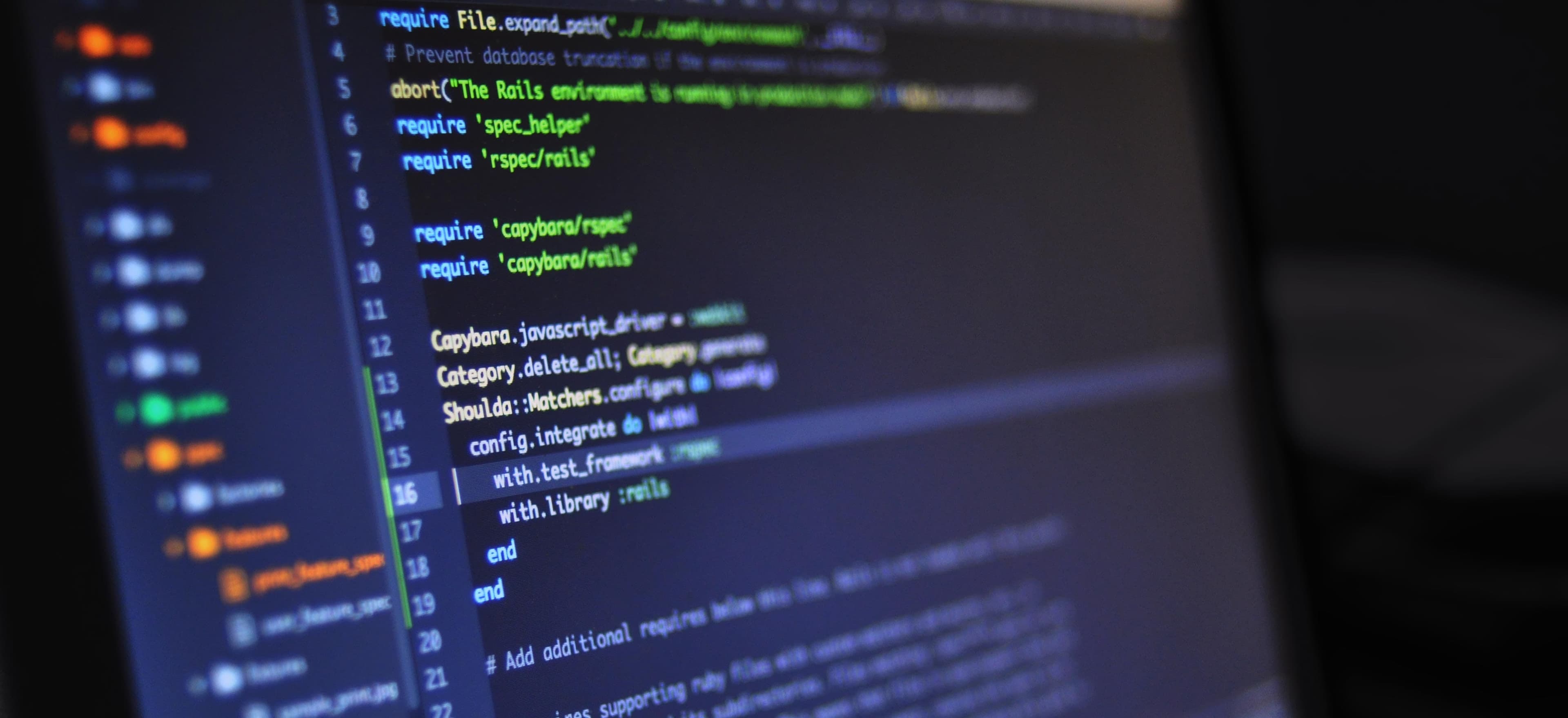
- Published on
Unlocking JDK 11: Simplifying String Manipulation
Java Development Kit (JDK) 11 brought with it several enhancements, especially in the arena of String manipulation—an essential aspect of programming in Java. As developers continue to emphasize the importance of writing clean, efficient, and maintainable code, understanding the new string manipulation features can promote better practices. In this blog post, we will explore the new functionalities introduced in JDK 11 and how they simplify string handling tasks.
Why String Manipulation Matters
Strings are one of the most commonly used data types in programming. They allow us to handle text data and perform operations such as searching, replacing, concatenating, and formatting. In Java, immutable strings are part of platform security and memory efficiency, but they often require additional boilerplate code for basic operations. The new features in JDK 11 address some of these shortcomings, making string manipulation more intuitive and efficient.
New Features in JDK 11 for String Manipulation
1. isBlank() Method
One of the standout features introduced in JDK 11 is the isBlank()
method. This method checks if a string is empty or contains only whitespace characters. This is particularly useful for validating user inputs or parsing data.
Example:
String input = " ";
if (input.isBlank()) {
System.out.println("Input is blank");
} else {
System.out.println("Input contains text");
}
Why?: In previous versions of Java, we had to use the combination of trim()
and length()
methods, which makes the code less readable. Now, with isBlank()
, checking for blank strings is clearer and more concise.
2. lines() Method
Another significant addition is the lines()
method, which returns a stream of lines extracted from a string, splitting it where lines break. This functionality enhances readability and allows for more powerful processing of multi-line strings.
Example:
String multiLineString = "Hello\nWorld\nJava";
multiLineString.lines().forEach(System.out::println);
Why?: This method is particularly handy when dealing with text data that contains line breaks, allowing developers to easily handle each line individually without needing complex parsing.
3. strip(), stripLeading(), and stripTrailing() Methods
JDK 11 introduces the strip()
, stripLeading()
, and stripTrailing()
methods as more Unicode-aware alternatives to trim()
. These methods remove whitespace from both ends, or just the leading or trailing spaces, respectively.
Example:
String paddedString = " Java 11 ";
String strippedString = paddedString.strip();
String leadingStripped = paddedString.stripLeading();
String trailingStripped = paddedString.stripTrailing();
System.out.println("Original: '" + paddedString + "'");
System.out.println("Stripped: '" + strippedString + "'");
System.out.println("Leading Stripped: '" + leadingStripped + "'");
System.out.println("Trailing Stripped: '" + trailingStripped + "'");
Why?: When dealing with internationalization, spaces may vary in representation, and these new methods are built to handle those variations better than the traditional trim()
.
4. repeat(int count) Method
The repeat(int count)
method enables the repetition of a string a specified number of times. This is particularly useful for formatting outputs, such as creating headers or patterns.
Example:
String repeatString = "Java ";
String repeated = repeatString.repeat(3);
System.out.println(repeated); // Output: Java Java Java
Why?: Previously, developers had to use loops to achieve this functionality, making the code cumbersome. This method achieves a cleaner solution with an expressive call.
5. Formatted String (String.format)
While String.format()
has been around for a while, it saw performance improvements and offers better options in JDK 11. This method is critical for creating output strings, allowing variables to be formatted into strings easily.
Example:
String name = "John";
int age = 30;
String formattedString = String.format("My name is %s and I am %d years old.", name, age);
System.out.println(formattedString);
Why?: Using String.format()
provides a clear separation between string content and variable data, enhancing the readability and maintainability of the code.
Practical Application
Understanding these string manipulation methods can significantly affect productivity and code cleanliness. Below is a practical example illustrating the combined use of these methods in a user input scenario.
Example Application: User Input Validation
import java.util.Scanner;
public class UserInputValidation {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.print("Enter your name: ");
String name = scanner.nextLine();
if(name.isBlank()) {
System.out.println("Name cannot be blank!");
} else {
System.out.println("Welcome, " + name.strip() + "!");
}
System.out.print("Enter your biography (use \\n for new line): ");
String bio = scanner.nextLine();
bio.lines().forEach(line -> System.out.println("Bio line: " + line.strip()));
scanner.close();
}
}
Why Combined Methods Matter
By combining these functionalities, we ensure that our application is user-friendly and resilient. This not only improves user experience but also reduces the chances of errors caused by unexpected user input.
Final Thoughts
Java JDK 11 has significantly enhanced string manipulation with several new features that simplify common tasks. By adopting these methods—isBlank()
, lines()
, strip()
, repeat()
, and formatted strings—developers can write cleaner, more efficient code. The ability to manipulate strings effectively not only boosts productivity but also leads to better software design.
For further exploration, visit the official JDK documentation to dive deeper into the capabilities of String handling in Java.
As you navigate through Java’s growing landscape, consider these enhancements and think about how they can improve your everyday coding tasks. Whether you are a newbie or an experienced developer, embracing these changes will undoubtedly lead to more powerful Java applications. Happy coding!