Maximizing Results: Common Pitfalls in Performance Experiments
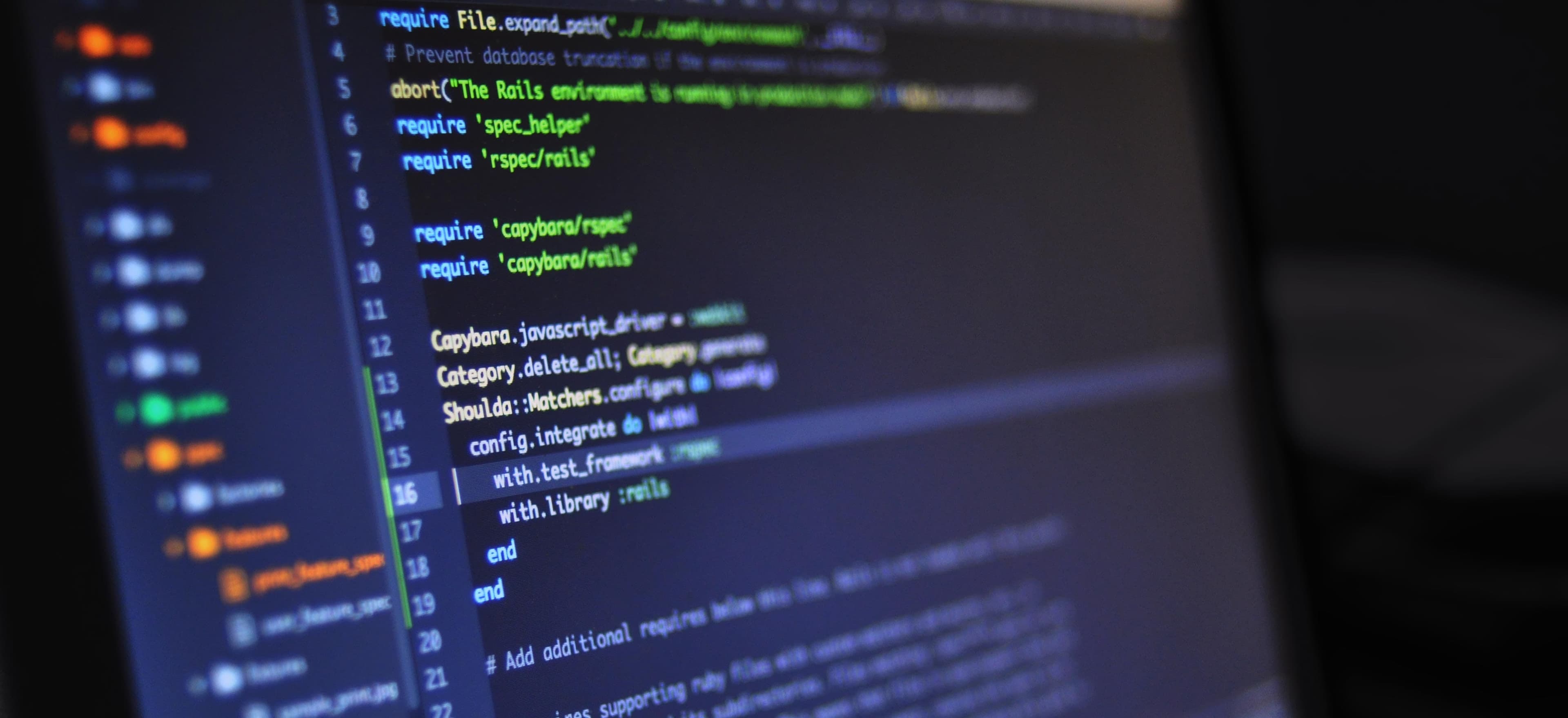
- Published on
Maximizing Results: Common Pitfalls in Performance Experiments
Performance experiments are a crucial aspect of software development, especially in fields like web development, data science, and system architecture. They provide valuable insights into how applications behave under varying loads and conditions. However, pitfalls in conducting these experiments can lead to misleading results and unexpected consequences. In this blog post, we will explore common mistakes in performance testing and how to avoid them, helping you achieve more reliable outcomes in your performance experiments.
Understanding Performance Testing
Before we delve into the pitfalls, let’s clarify what performance testing entails. Performance testing is the process of evaluating the speed, scalability, and stability of a system under a given workload. The main objectives include measuring response times, throughput, and resource utilization.
To learn more about the different types of performance testing, you can check out the Wikipedia page on Software Performance Testing.
Common Pitfalls in Performance Experiments
1. Poorly Defined Objectives
One of the most significant mistakes is failing to establish clear objectives for your performance tests. Without specific goals, it’s nearly impossible to assess whether your system meets the desired performance criteria.
Solution: Before starting any tests, define what you want to measure. Is it response time, maximum concurrent users, or system stability under load? Having well-documented objectives helps you focus your experiments and interpret results accurately.
2. Inconsistent Test Environments
Running tests in inconsistent environments can skew results significantly. Variables such as different hardware, network conditions, or configurations can lead to varying performance metrics.
Solution: Maintain a consistent test environment. Use virtual machines or containers to ensure that all tests are conducted under the same conditions. Tools like Docker and Kubernetes can be invaluable for this purpose, enabling you to replicate environments easily.
# Example of running a Docker container for tests
docker run --rm -p 80:80 my-web-app
3. Ignoring Real-World Conditions
Many developers simulate ideal conditions during performance tests, ignoring the complexities of real-world usage patterns. This misrepresentation can give a false sense of performance.
Solution: Model your tests after actual usage scenarios. Collect data on user behavior, peak usage times, and common actions to create realistic load profiles. Consider using a tool like Apache JMeter for load testing, which simulates multiple users effectively.
// Example of a JMeter test plan configuration in XML format
<TestPlan>
<ThreadGroup>
<numThreads>100</numThreads> <!-- Simulate 100 users -->
<rampTime>60</rampTime> <!-- Ramp up over 60 seconds -->
<loopCount>1</loopCount>
<Sampler>
<HTTPRequest>
<domain>yourdomain.com</domain>
<path>/api/v1/resource</path>
</HTTPRequest>
</Sampler>
</ThreadGroup>
</TestPlan>
4. Not Monitoring Resource Usage
Performance tests without monitoring resource utilization (CPU, memory, disk, network) provide an incomplete picture of system behavior. You may observe improved response times, but behind the scenes, your server may be under duress.
Solution: Use monitoring tools like Prometheus or Grafana to track resource usage during tests. This data can highlight bottlenecks, showing whether the application is experiencing high resource consumption.
5. Lack of Baseline Measurements
Starting performance experiments without baseline measurements is like trying to navigate without a map. If you do not have a reference point, you cannot determine the success or failure of changes made to the system.
Solution: Conduct initial tests to establish baseline measurements before implementing any improvements. Track metrics over time to identify trends or regressions.
// Example of logging baseline metrics
import java.util.logging.*;
public class PerformanceLogger {
private static Logger logger = Logger.getLogger("PerformanceLogger");
public static void log(String message, long executionTime) {
logger.info(message + " took " + executionTime + "ms");
}
}
// Usage
long start = System.currentTimeMillis();
// Some performance-sensitive operation
long end = System.currentTimeMillis();
PerformanceLogger.log("Operation X", end - start);
6. Forgetting About Network Latency
It’s easy to focus solely on server-side performance and lose sight of network latency, which can significantly affect response times. Client-side applications must also be considered.
Solution: Test your application from various geographical locations, especially if you have a global user base. Tools like Blazemeter or LoadRunner allow for testing with simulated user requests from different regions.
7. Not Analyzing Results Thoroughly
Collecting data is just part of performance testing; you must analyze and interpret the results to get meaningful insights. Assuming that you can deduce what went wrong or right without analysis can lead to incorrect conclusions.
Solution: Utilize analytical tools and approaches to dissect the test results. Look for patterns, outliers, and anomalies in the metrics. For instance, if response times consistently exceed the set threshold at a specific load level, investigate further.
8. Failing to Iterate
Performance testing should be an iterative process. If you conduct a single set of tests and implement changes based solely on those results, you might miss additional optimizations or issues that could arise under different conditions.
Solution: Implement continuous performance testing within your development lifecycle. As you make changes, rerun tests to assess their impact. Use CI/CD pipelines to automate these tests, ensuring that performance remains consistently monitored.
9. Ignoring Edge Cases
Focusing solely on average performance metrics can lead to overlooking edge cases that could cause performance failures under certain conditions.
Solution: Identify and test edge cases that could potentially affect performance. This involves analyzing usage patterns and considering abnormal data inputs or sudden spikes in user activity.
// Example of testing an edge case in a web application
// Testing with a high volume of simultaneous requests
for (int i = 0; i < 1000; i++) {
new Thread(() -> {
// Simulate a request to the server
makeHttpRequest("http://yourdomain.com/api/v1/resource");
}).start();
}
10. Neglecting Team Collaboration
A lack of communication among team members during performance testing can lead to fragmented insights and missed optimizations. Developers, testers, and operations teams must work together.
Solution: Foster a culture of collaboration and ensure insights from performance tests are shared across teams. Use tools that facilitate visibility, such as JIRA or Trello, to keep track of performance issues and resolutions.
To Wrap Things Up
Conducting effective performance experiments is crucial for delivering high-quality software. By avoiding these common pitfalls, you can ensure that your testing is reliable, actionable, and representative of actual user experiences.
Incorporate these practices into your performance testing strategy, and you'll be better equipped to maximize results and deliver robust applications that meet user expectations.
For further reading, consider checking out the Performance Testing Checklist for more in-depth details on how to approach your next performance testing initiative.
Remember, performance testing is an ongoing process, and continuous evaluation and iteration are key to maintaining optimal system performance. Happy testing!