Boosting Performance: PrimeFaces Chart Caching Issues
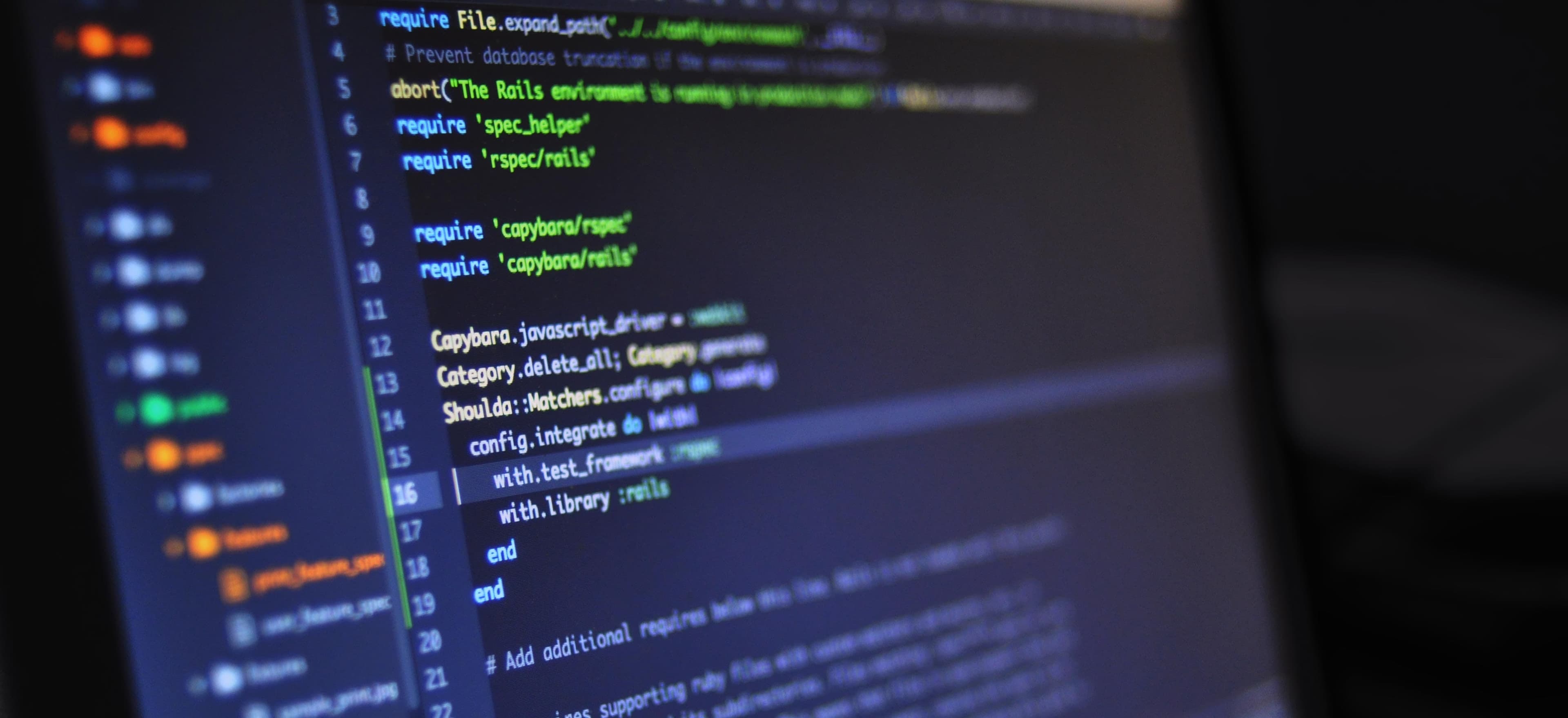
- Published on
Boosting Performance: PrimeFaces Chart Caching Issues
When developing web applications with rich user interfaces, performance is a crucial factor in ensuring a seamless user experience. PrimeFaces, a popular UI framework for JavaServer Faces (JSF), provides powerful components for data visualization, including charting capabilities. However, as developers incorporate charting elements into their applications, they may encounter caching issues that can significantly impact performance. In this article, we will explore these caching challenges and offer strategies for efficient management.
Understanding PrimeFaces Charts
PrimeFaces charts leverage the capabilities of JavaScript libraries like Chart.js and Highcharts to deliver interactive visual representations of data. They can efficiently render dynamic data in a user-friendly format. However, like many rendering libraries, managing how and when charts are updated or re-rendered can be tricky.
When a user interacts with your application—updating data, filtering results, or just navigating—the last thing you want is outdated or stale data being presented. This is where caching comes into play.
Caching in PrimeFaces Charts
Caching can significantly enhance performance by reducing loading times and server calls. However, it can also introduce issues when data changes. By default, browsers cache resources to minimize the number of requests made to the server. If the chart data changes, and the browser serves the cached version, users may see incorrect or incomplete data.
Example Scenario
Consider an example where a user is viewing a sales chart. The chart is initially loaded displaying data from the previous month. After a refresh, the backend updates sales data, but the cached chart might show the previous figures instead. The result? Confusion and a frustrating user experience.
Code Snippet: Chart Implementation
Here is a basic implementation of a PrimeFaces chart, showing how easy it is to get started:
<h:form>
<p:chart type="bar" model="#{chartView.barModel}" />
</h:form>
In this example, chartView
is a managed bean containing the data model for our bar chart. However, this does not factor in how to manage cached data effectively.
Strategies for Managing Cache
1. Disable Browser Caching
If freshness is critical for your data, you can instruct the browser not to cache the chart. This can be done using caching headers set on your backend. For instance:
HttpServletResponse response = (HttpServletResponse) FacesContext.getCurrentInstance().getExternalContext().getResponse();
response.setHeader("Cache-Control", "no-cache, no-store, must-revalidate"); // HTTP 1.1
response.setHeader("Pragma", "no-cache"); // HTTP 1.0
response.setDateHeader("Expires", 0); // Proxies
By setting these headers, you ensure that the browser requests a fresh version every time. However, this may result in lower performance since every request will be made to the server, negating some benefits of caching.
2. Use Versioning or Unique Identifiers
A more sophisticated approach involves using versioning to force the browser to refresh the cache when data updates. This can be achieved by appending a unique query string to the chart's source URL.
public String getChartDataUrl() {
String uniqueId = String.valueOf(System.currentTimeMillis());
return "chartData?timestamp=" + uniqueId;
}
In the view, bind the chart source URL to this method:
<p:chart type="bar" widgetVar="barChart"
data="#{chartView.getChartDataUrl()}" />
Each time the page is rendered, the chart's URL will vary, thereby bypassing the cache without completely disabling it.
3. Polling for Updates
In dynamic environments, consider using polling to refresh your charts at defined intervals. By using JavaScript, you can set a timer to refresh the chart component every few seconds/minutes.
Here’s how that can look:
setInterval(function() {
PF('barChart').refresh();
}, 30000); // Refresh every 30 seconds
This strategy ensures that users have up-to-date information without requiring a complete page reload while striking a balance between performance and data accuracy.
4. WebSocket Integration
For real-time applications, WebSockets may provide the best solution. By establishing a persistent connection between the client and server, charts can be updated instantly with no need for polling:
Implementing WebSocket support can be tricky but significantly enhances the user experience when real-time data updates are crucial.
Example with Java EE WebSockets:
@ServerEndpoint("/chartupdates")
public class ChartUpdateEndpoint {
@OnMessage
public void onMessage(String message, Session session) {
// Broadcast the message to all sessions
}
}
To Wrap Things Up
Optimizing the performance of PrimeFaces charts through effective cache management can significantly enhance user experiences in web applications. Through careful strategies—such as disabling caching for critical updates, using unique identifiers, polling for updates, or incorporating WebSockets—developers can ensure users see the most accurate and timely data available.
For more information on PrimeFaces, you can explore their official documentation. Additionally, for detailed guidelines on JSF caching and optimization techniques, you can refer to this insightful Java EE caching tutorial.
By implementing these strategies, you can improve the responsiveness of your applications, reduce user confusion with stale data, and harness the full power of interactive charts in your Java applications. Happy coding!