Tackling Memory Leaks: Frequency Vs. Severity Explained
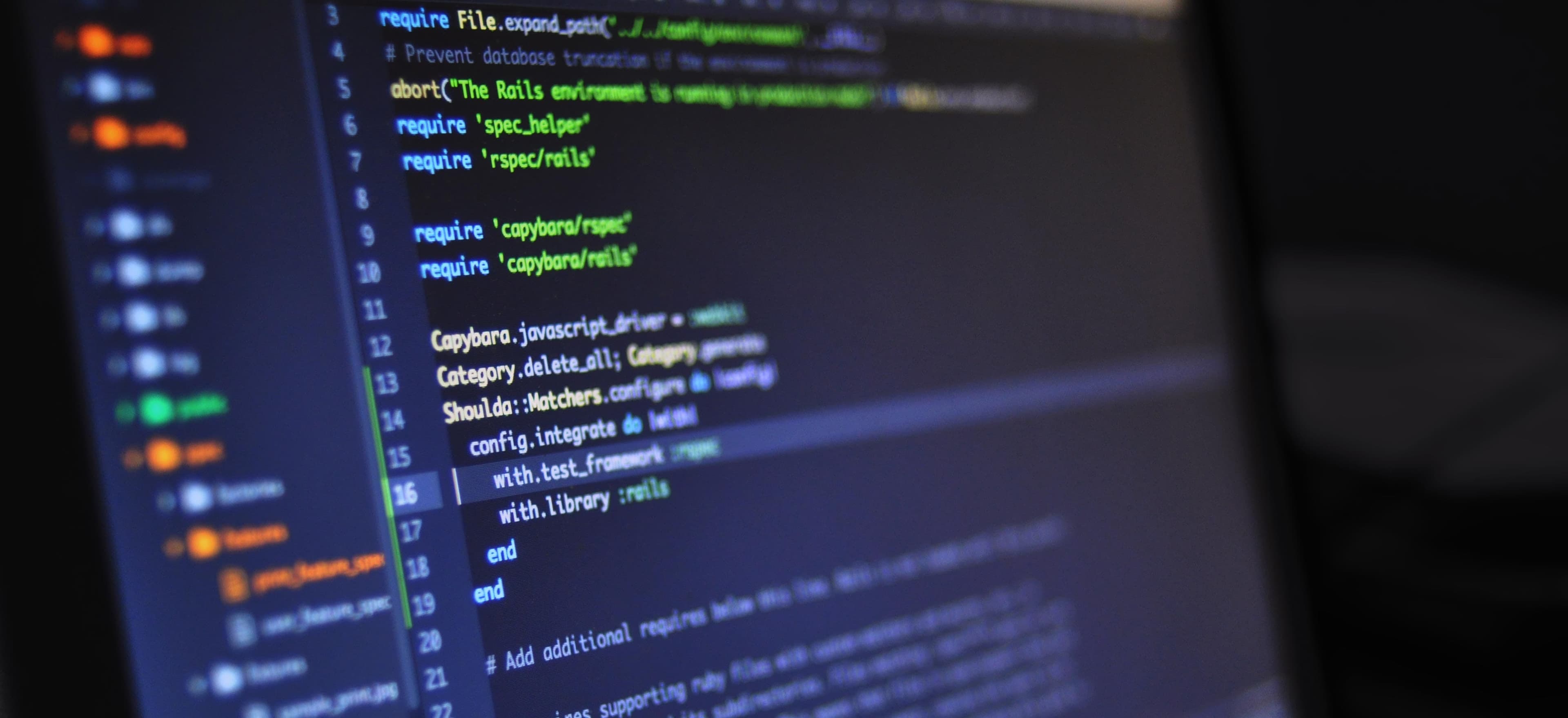
- Published on
Tackling Memory Leaks in Java: Frequency vs. Severity Explained
Memory management is a crucial aspect of Java programming, especially in large-scale applications. While Java's garbage collector provides a level of abstraction for memory management, developers must remain vigilant to avoid memory leaks. In this post, we will dive deep into the concepts of memory leaks, focusing specifically on their frequency and severity, and provide strategies to tackle these issues effectively.
Understanding Memory Leaks
A memory leak occurs when an application unintentionally retains references to objects that are no longer needed. Consequently, this prevents the garbage collector from reclaiming memory, leading to increased memory usage over time. Memory leaks can be categorized primarily by their frequency and severity, two crucial factors that determine the impact of the leaks on application performance.
Frequency of Memory Leaks
Frequency refers to how often a memory leak occurs within the application’s lifecycle. Understanding this concept can help developers diagnose problems and implement timely fixes.
Types of Frequency-Based Leaks
-
Continuous Leaks: These leaks occur consistently during application runtime and gradually consume memory. For instance, if a method continuously adds objects to a list without clearing it afterward.
-
Intermittent Leaks: These are sporadic leaks that may not become apparent until certain conditions are met. They often depend on user actions or specific application workflows.
Conceptually, frequency can act as a guideline for prioritizing leak fixes. Continuous leaks will escalate in severity more quickly than intermittent ones.
Severity of Memory Leaks
Severity measures the impact of memory leaks on application performance and resource management. A leak's severity can vary based on the size of the leaked objects, the frequency of leaking, and the overall architecture.
Types of Severity-Based Leaks
-
Low Severity: In cases where a small object or a handful of objects are leaked, the impact on performance may be negligible initially. These leaks can manifest as minor slowdowns but may not necessitate immediate action.
-
High Severity: When large objects or a significant number of objects are leaked, the application may experience severe performance degradation, crashes, or excessive garbage collection pauses. An example could be caching too many database connections without releasing them.
By evaluating both frequency and severity, developers can focus on fixing the most critical memory leaks first.
Common Sources of Memory Leaks in Java
Awareness of common sources can aid developers in avoiding memory leaks. Here are some frequent culprits:
-
Static Collections: Holding references in static fields can lead to memory leaks if objects are added but never removed.
public class MemoryLeakExample { private static List<Object> cachedObjects = new ArrayList<>(); public static void cacheObject(Object obj) { cachedObjects.add(obj); } }
In this example, cached objects never get cleared, leading to continuous growth in memory usage.
-
Listeners and Callbacks: Not removing listeners when they are no longer needed is a common mistake. This keeps the referenced objects alive longer than necessary.
public class EventManager { private List<EventListener> listeners = new ArrayList<>(); public void registerListener(EventListener listener) { listeners.add(listener); } // Consider adding a method to remove listeners }
-
Inner Classes: Non-static inner classes implicitly hold a reference to their enclosing instance. This can prevent the enclosing object from being garbage collected.
public class Outer { class Inner { void doSomething() { // Some operations } } }
In the example above, the
Inner
instance keeps a reference to anOuter
object, which can result in memory leaks ifOuter
is no longer needed.
Techniques for Monitoring Memory Leaks
To effectively manage memory leaks, developers must implement strategies to identify them. Here are some techniques:
-
Profiling Tools: Utilize tools like VisualVM or Eclipse Memory Analyzer (MAT). These can analyze heap dumps and identify memory leaks.
-
Garbage Collection Logs: Enable garbage collection logging to track memory allocation and collection events.
java -Xlog:gc* -jar YourApp.jar
This allows you to observe how the garbage collector behaves and helps identify memory retention patterns.
- Unit Testing: Write tests targeting edge cases that could lead to memory leaks, ensuring that object references are cleared when no longer needed.
Fixing Memory Leaks Effectively
When you identify a memory leak, swift action is vital. Here are steps developers can take:
-
Analyze the Leak: Use a memory profiler to pinpoint the source of the leak by examining the reference chains.
-
Refactor Code: Implement changes in code designs, such as utilizing weak references, removing unnecessary static fields, or using event unregistration patterns.
// Using WeakReference for caching class Cache { private Map<String, WeakReference<Object>> cache = new HashMap<>(); public void put(String key, Object value) { cache.put(key, new WeakReference<>(value)); } }
-
Implement Best Practices: Maintain best practices in coding to lower the risk of leaks. This includes using frameworks that manage resource lifecycles or adopting design patterns that reduce unnecessary references.
-
Run Regular Load Tests: Simulate high-load scenarios to observe how the application behaves, which may surface memory leaks that occur under load.
The Last Word: Prioritizing Frequency and Severity
In conclusion, tackling memory leaks in Java is a nuanced endeavor that involves understanding both frequency and severity. By being proactive and implementing comprehensive monitoring strategies, developers can mitigate risks and ensure the smooth performance of their applications. The understanding of common sources, detection techniques, and refactoring principles can empower Java developers to create robust, memory-efficient applications.
For further reading on memory management in Java, explore the Java Documentation or the Java Performance Tuning Guide. Understanding and tackling memory leaks will not only enhance performance but provide a better user experience overall.
Checkout our other articles