Troubleshooting Common Issues with Event Publish Feeds
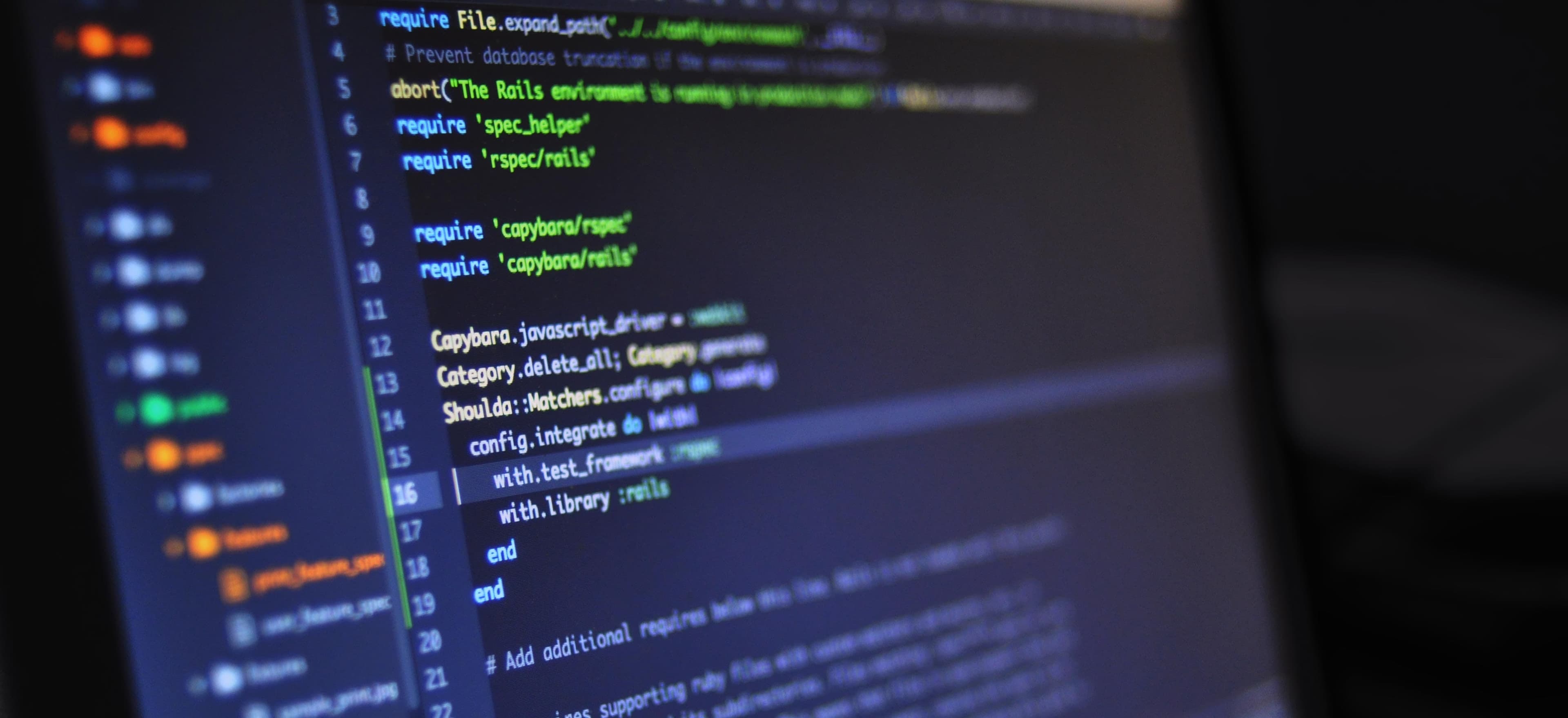
- Published on
Troubleshooting Common Issues with Event Publish Feeds in Java
Event publish feeds play a crucial role in many Java applications, especially those involving real-time data streaming and integration. Understanding how to troubleshoot common issues can significantly improve the reliability and performance of your systems. In this blog post, we will explore some frequent problems you may encounter when working with event publish feeds and provide solutions to overcome these challenges.
Overview of Event Publish Feeds
Before diving into troubleshooting, it's essential to understand what event publish feeds are. An event publisher is responsible for sending out messages or events to subscribers. The subscribers can be other applications, services, or components that need to react to these events. This communication model is essential in systems involving microservices, message queues, and event-driven architecture.
Key Components of an Event Publish Feed
- Publisher: The originator of the event.
- Event: The data or message sent by the publisher.
- Subscriber: The recipient of the event.
- Middleware: Often used to ensure reliable messaging.
Understanding these components will help you better diagnose issues when they arise.
Common Issues with Event Publish Feeds
1. Event Loss
Problem: One of the most critical issues is event loss. This occurs when messages sent by the publisher fail to reach the subscribers.
Possible Causes:
- Network failures.
- Subscriber applications not being available.
- Misconfiguration of message brokers.
Solution: Implementing message acknowledgment and retries can mitigate this issue. Below is an example of how to ensure reliable message delivery using a Java message queue.
import javax.jms.*;
public void sendMessage(Session session, Destination destination, String messageText) {
try {
MessageProducer producer = session.createProducer(destination);
TextMessage message = session.createTextMessage(messageText);
// Setting message expiration
message.setExpiration(System.currentTimeMillis() + 60000); // 1 minute expiry
producer.send(message);
System.out.println("Message sent: " + messageText);
// Close the producer to free resources
producer.close();
} catch (JMSException e) {
e.printStackTrace();
}
}
Why This Works: By setting an expiration time, you can ensure that messages do not linger indefinitely, thus improving performance. Using a try-catch block for JMS exceptions provides better insight into runtime issues.
2. High Latency
Problem: Sometimes the event processing might experience significant delays, leading to latency.
Possible Causes:
- Overloaded systems or resources.
- Inefficient event handling logic.
- Slow network connections.
Solution: Monitor system performance and implement asynchronous processing when necessary. Here's how you can use Java's CompletableFuture
to improve responsiveness.
import java.util.concurrent.CompletableFuture;
public CompletableFuture<Void> processEventAsync(String event) {
return CompletableFuture.runAsync(() -> {
// Simulate event processing
try {
Thread.sleep(2000); // simulating time-consuming task
System.out.println("Processed event: " + event);
} catch (InterruptedException e) {
e.printStackTrace();
}
});
}
Why This Works: Non-blocking I/O operations can help prevent the main thread from being held up. By using CompletableFuture
, you allow multiple events to be processed concurrently.
3. Serialization Issues
Problem: Serialization issues can interrupt the flow of your event feed, especially when sending complex objects.
Possible Causes:
- Lack of proper serialization mechanisms.
- Class version mismatch between the publisher and subscriber.
Solution: Always ensure that your objects implement the Serializable
interface properly and consider using a serialization framework like Jackson or Gson for JSON-based data interchange.
import com.fasterxml.jackson.core.JsonProcessingException;
import com.fasterxml.jackson.databind.ObjectMapper;
public String serializeEvent(Event event) {
ObjectMapper objectMapper = new ObjectMapper();
try {
return objectMapper.writeValueAsString(event);
} catch (JsonProcessingException e) {
e.printStackTrace();
return null;
}
}
Why This Works: JSON serialization allows for easier data interchange across different platforms. Using a well-tested library minimizes compatibility issues.
4. Configuration Issues
Problem: Misconfiguration can lead to a host of issues, from connectivity problems to incorrect topic/subscriptions.
Possible Causes:
- Incorrect broker URLs.
- Missing authentication credentials.
- Topic names mistyped.
Solution: Review your configuration settings regularly. Use environment variables or a dedicated configuration management service, like Spring Cloud Config, to manage your settings.
# application.properties
spring.kafka.bootstrap-servers=localhost:9092
spring.kafka.consumer.group-id=myGroupId
security.protocol=SASL_PLAINTEXT
Why This Works: Externalizing configuration ensures that you can change settings without modifying the codebase. This practice promotes a clean architecture and aids in troubleshooting.
Performance Monitoring
In addition to the above troubleshooting strategies, implementing performance monitoring tools can provide invaluable insights into your event feed operations. Consider integrating:
- Prometheus for metrics collection.
- Grafana for visualizing performance data.
For more information on setting up these tools, check out Prometheus Documentation and Grafana Documentation.
The Last Word
Troubleshooting event publish feed issues in Java applications requires understanding both the underlying architecture and the potential pitfalls that can arise. By following the strategies discussed in this blog, you can build a more reliable and responsive system.
Stay proactive with your monitoring and regularly check your configurations to preemptively address possible issues. As always, testing thoroughly in your development environment will help you discover and fix issues before they affect production.
By implementing effective practices in error management, performance monitoring, and configuration management, your application will be well-equipped to handle even the most stubborn event publishing challenges. Happy coding!
This blog post aimed not just to present the issues but also to furnish you with practical solutions and code snippets that you can apply in your projects. Keep experimenting and enhancing your Java event publishing skills!
Checkout our other articles