Overcoming Common Pitfalls in Tell Don't Ask Refactoring
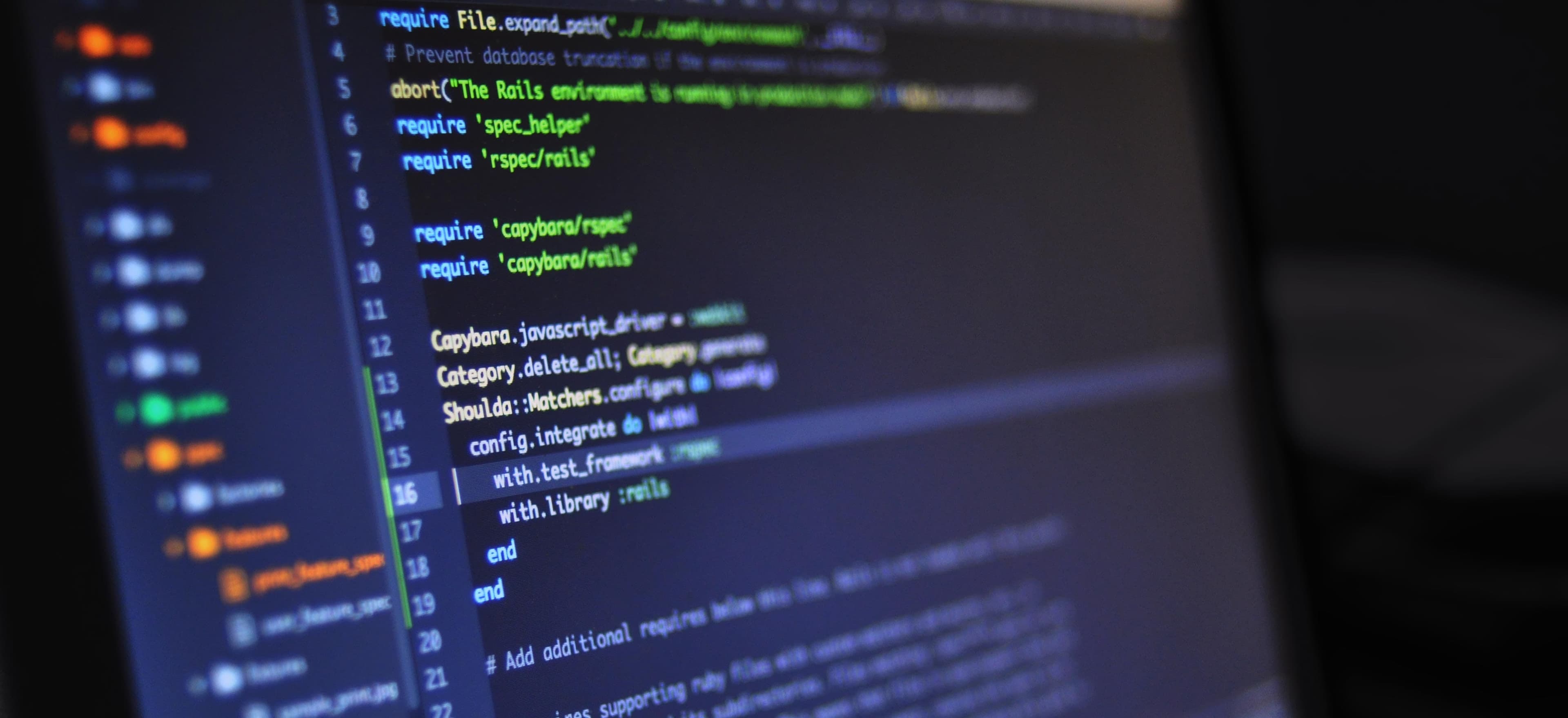
- Published on
Overcoming Common Pitfalls in Tell, Don't Ask Refactoring
Refactoring is a key practice in software development that helps improve code quality, maintainability, and readability. Among the various principles of refactoring, the "Tell, Don't Ask" principle stands as a pivotal concept. This principle encourages developers to command objects to perform tasks rather than querying them for data to make decisions themselves. In this blog post, we will delve into the nuances of "Tell, Don't Ask" refactoring, highlight common pitfalls, and present effective strategies to overcome them.
What is "Tell, Don't Ask"?
At its core, "Tell, Don't Ask" advocates for designing objects that encapsulate behavior, which leads to greater cohesion and enhances encapsulation. When an object provides methods that handle its own state, it can execute responsibilities internally, thereby reducing dependencies and increasing robustness.
Example Without Refactoring
Let's consider a simple example in Java that illustrates the "Ask" approach:
public class Order {
private double amount;
public Order(double amount) {
this.amount = amount;
}
public double getAmount() {
return amount;
}
}
public class OrderProcessor {
public void process(Order order) {
if (order.getAmount() > 100) {
System.out.println("Processing order with high amount.");
} else {
System.out.println("Processing order with low amount.");
}
}
}
In this code, the OrderProcessor
asks the Order
how much it should process, which represents an indirect manner of handling the processing logic. This violates the "Tell, Don't Ask" principle.
Refactored Version
Now, let’s refactor the code to align with this principle:
public class Order {
private double amount;
public Order(double amount) {
this.amount = amount;
}
public void process() {
if (amount > 100) {
System.out.println("Processing order with high amount.");
} else {
System.out.println("Processing order with low amount.");
}
}
}
public class OrderProcessor {
public void process(Order order) {
order.process(); // Telling the order to process itself.
}
}
In this revised version, we moved the responsibility of determining how the order should be processed inside the Order
class. Now, OrderProcessor
simply tells the Order
to process itself.
Common Pitfalls in "Tell, Don't Ask" Refactoring
While working on "Tell, Don't Ask" refactoring, developers often face several challenges. Identifying and overcoming these pitfalls is crucial for successful implementation.
1. Overcommitment to the Principle
One common mistake is rigidly adhering to the principle without considering practicality. It can lead to unnecessarily complicated code structures.
Solution: Embrace flexibility in applying the principle. If adhering to "Tell, Don't Ask" results in highly convoluted objects or excess method calls, consider scaling back. Aim for simplicity while maintaining clarity.
2. Dispersed Logic
When trying to avoid asking, developers may inadvertently spread behavior across multiple classes, resulting in objects that are harder to manage.
Solution: Focus on cohesively grouping related behaviors. For instance, if multiple actions pertain to an object, encapsulate them within that object, preventing the logic from being scattered:
public class Order {
private double amount;
private String status;
public void fulfill() {
// Logic for fulfilling the order
status = "fulfilled";
System.out.println("Order fulfilled.");
}
public void cancel() {
// Logic for canceling the order
status = "canceled";
System.out.println("Order canceled.");
}
public void process() {
// Make decisions based on current state
if (status.equals("pending")) {
fulfill();
}
}
}
3. Ignoring Class Responsibilities
Another challenge is overloading a single class with too many responsibilities, resulting in a violation of the Single Responsibility Principle.
Solution: Carefully evaluate the responsibilities assigned to each class. If a class is managing too many tasks, consider breaking it apart. For example, you might decouple order processing logic from the order itself by introducing dedicated handler classes without violating the "Tell, Don't Ask" principle:
public interface OrderAction {
void execute(Order order);
}
public class FulfillOrder implements OrderAction {
public void execute(Order order) {
// Logic for fulfilling the order
}
}
public class CancelOrder implements OrderAction {
public void execute(Order order) {
// Logic for canceling the order
}
}
public class Order {
private double amount;
private String status;
public void process(OrderAction action) {
action.execute(this);
}
}
This way, you create specialized actions that can be employed through the Order
class, enhancing modularity while still following the "Tell, Don't Ask" principle.
4. Performance Concerns
Some developers worry that focusing too heavily on encapsulation and object calls can lead to performance degradation, primarily when working with large datasets or high-frequency operations.
Solution: Understand the trade-offs. In many cases, the performance impact will be negligible compared to the benefits of maintainability and clarity. However, if performance becomes an issue:
- Profile your code to locate bottlenecks.
- Optimize only those portions that create performance issues, rather than applying global optimizations.
5. Lack of Clear Domain Understanding
Failure to deeply understand the domain can lead to misaligned class designs that incorrectly embody business logic.
Solution: Collaborate closely with domain experts to align your object designs with the true business requirements. By understanding your domain well, you can create more meaningful objects capable of effectively following the "Tell, Don't Ask" principle.
Wrapping Up
The "Tell, Don't Ask" principle is a critical part of crafting clean, maintainable Java applications. By properly understanding its implications and potential pitfalls, developers can create systems that are both robust and easy to manage.
Additional Resources
- Refactoring Guru: Tell, Don't Ask
- Clean Code by Robert C. Martin
- Martin Fowler's Refactoring
By applying the principles discussed in this blog post, you will not only enhance the quality of your refactoring efforts but also ensure that your code remains understandable and agile for future changes. Happy coding!