Boosting Java Performance: Mastering the Execution Pipeline
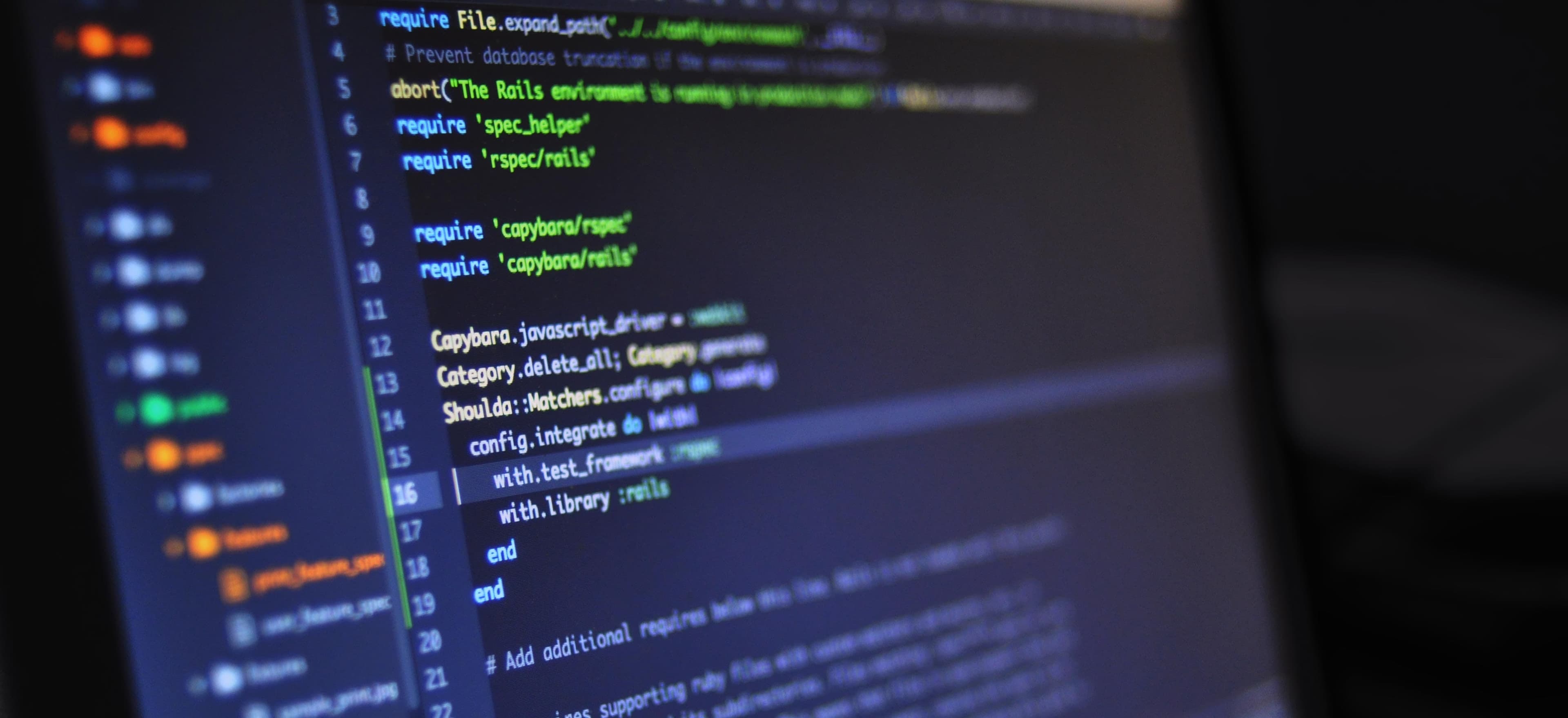
- Published on
Boosting Java Performance: Mastering the Execution Pipeline
Java has long been a favorite among developers due to its portability, security, and robust performance capabilities. However, as applications grow in complexity, the need for performance optimization becomes more pressing than ever. In this blog post, we will explore the Java Execution Pipeline and how mastering it can significantly enhance the performance of your Java applications.
Understanding the Java Execution Pipeline
The execution pipeline in Java is a series of processes through which your Java code passes before it produces output. This includes writing code, compiling it, executing it on the Java Virtual Machine (JVM), and ultimately delivering the result.
- Source Code: This is the human-readable Java code that you write.
- Compilation: The Java compiler translates your code into bytecode (class files), which is an intermediate representation for the JVM.
- Execution: Finally, the JVM interprets or compiles the bytecode into native machine code for execution.
Recognizing these stages helps in pinpointing potential bottlenecks. To optimize performance, we must delve into techniques that enhance each of these stages.
1. Writing Efficient Code
Your journey to performance starts with writing efficient Java code. Here’s a simple code snippet that highlights the use of nested loops, which can be resource-intensive:
public void findDuplicates(int[] arr) {
for (int i = 0; i < arr.length; i++) {
for (int j = i + 1; j < arr.length; j++) {
if (arr[i] == arr[j]) {
System.out.println("Duplicate found: " + arr[i]);
}
}
}
}
Commentary:
- Inefficiency: This nested loop structure results in O(n^2) complexity, which may lead to significant delays with larger datasets.
- Optimization: You can improve performance by leveraging data structures like
HashSet
to track duplicates.
import java.util.HashSet;
public void findDuplicatesOptimized(int[] arr) {
HashSet<Integer> set = new HashSet<>();
for (int num : arr) {
if (set.contains(num)) {
System.out.println("Duplicate found: " + num);
} else {
set.add(num);
}
}
}
Using a HashSet
reduces the complexity to O(n), as both insertions and lookups occur in constant time.
2. Code Compilation
Java uses Just-In-Time (JIT) compilation, allowing performance improvements at runtime. However, developers can take additional steps:
- Modify the Compiler Settings: Tuning the JVM’s JIT parameters (like garbage collection settings) can lead to performance improvements.
Here’s how you can specify these settings:
java -Xms512m -Xmx1024m -XX:+UseG1GC -jar YourApplication.jar
- Profile Your Code: Tools like Java Mission Control can help identify slow-running methods by checking method invocation times, allowing you to optimize hotspots effectively.
3. Efficient Execution with JVM
Understanding JVM memory management is crucial. The garbage collector (GC) works to free up memory but can also introduce latency if not managed properly.
Garbage Collection Optimization
Consider adjusting the GC algorithm based on your application type:
- For response-time sensitive applications: Use
G1GC
, which balances throughput and pause time.
java -XX:+UseG1GC -jar YourApplication.jar
- For throughput sensitive applications: Use
ParallelGC
, optimizing overall performance and minimizing overhead.
Memory tuning is just one part of the pipeline. Understanding JVM flags and their influence can shape execution behavior dramatically.
JVM Tuning Sample
Java provides several options that can be leveraged to tune the performance. Here’s an example configuration to enhance operational efficiency:
java -Xms512m -Xmx2g -XX:PermSize=128m -XX:MaxPermSize=512m -XX:+UseConcMarkSweepGC -jar YourApplication.jar
Commentary:
- Memory Size: The
-Xms
and-Xmx
flags control the initial and maximum memory allocation. - GC Strategy: Selecting an appropriate GC based on your requirements can reduce pause times.
4. Leveraging Java 8 Streams
Java 8 introduced the Streams API, which allows for functional-style operations on collections. They can vastly improve performance by enabling parallel processing.
Example: Summing Numbers Efficiently
Here’s a typical example of summing numbers:
int sum = 0;
for (int num : numbers) {
sum += num;
}
This can also be done efficiently using streams:
int sum = Arrays.stream(numbers).parallel().sum();
Commentary:
- Parallel Processing: By using
parallel()
, you instruct the JVM to utilize multiple cores, leading to better performance on larger datasets. - Less Boilerplate: The Stream API leads to more concise, readable code.
Additional Techniques
-
Use Proper Data Structures: Choosing the right data structure can lead to significant performance gains. For example, using
ArrayList
for frequent read operations versusLinkedList
for frequent insertions and deletions. -
Avoid Unnecessary Object Creation: Minimizing object creation conserves memory and reduces the workload for the garbage collector.
-
Immutable Programming: Treating objects as immutable can help in multi-threaded environments by avoiding concurrency issues and reducing synchronization overhead.
Key Takeaways
In conclusion, mastering the Java execution pipeline is crucial for developers looking to optimize their applications. From writing more efficient code to effectively managing the JVM execution and garbage collection, every small change can lead to significant performance improvements.
Key Takeaways:
- Optimize your code for efficiency.
- Tune the compiler and JVM settings for better performance.
- Leverage Java 8 features like Streams for improved operations.
- Choose appropriate data structures wisely.
For further reading on Java performance tuning techniques, check out Oracle's official documentation.
Immerse yourself in profiling tools and JVM options to harness the full power of Java. With continuous practice and understanding of the execution pipeline, you can significantly boost the performance of your Java applications. Happy coding!