Harnessing Static Nested Classes in Java: Common Pitfalls
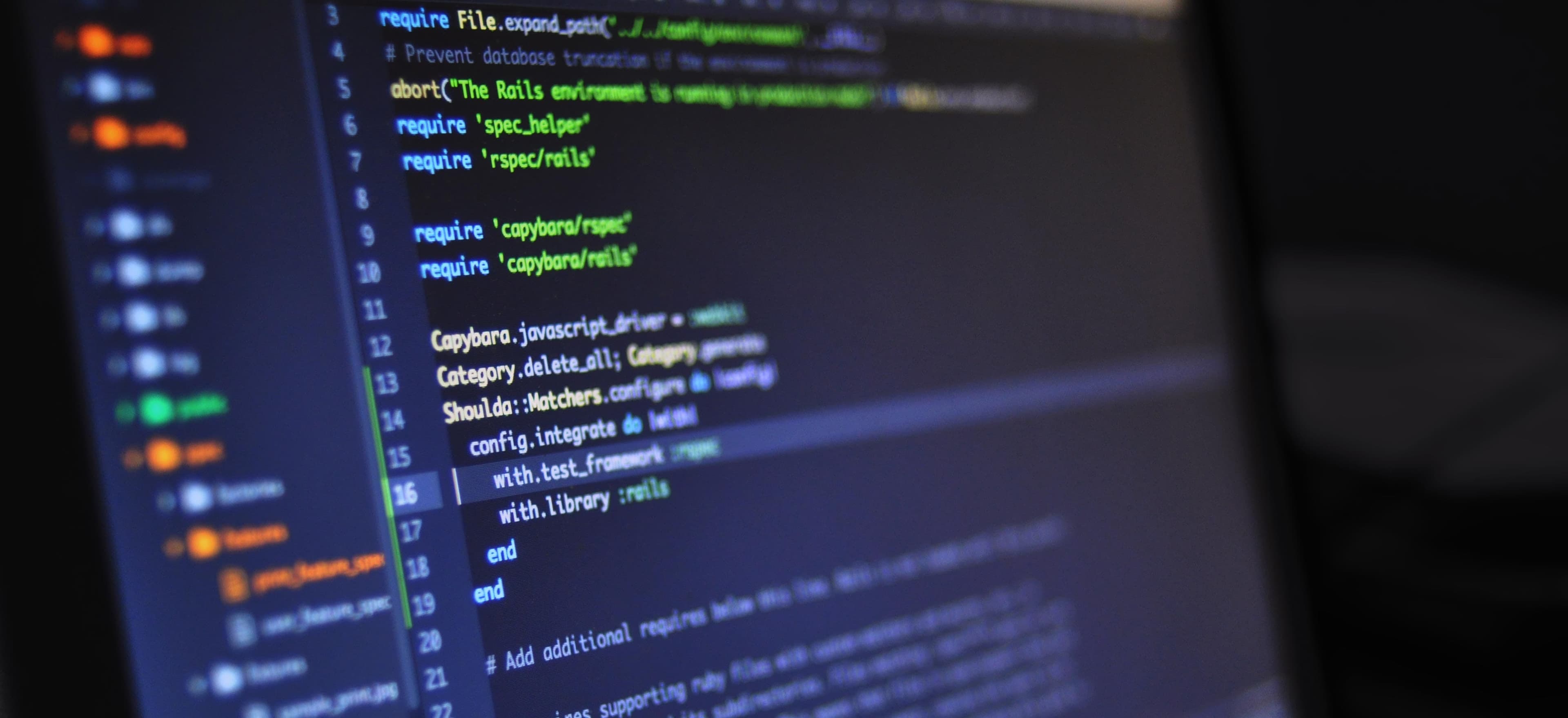
- Published on
Harnessing Static Nested Classes in Java: Common Pitfalls
Java is a powerful programming language that empowers developers to create robust applications with various object-oriented programming techniques. One such technique is the utilization of static nested classes. While static nested classes can greatly enhance the structure and readability of your code, they can also introduce pitfalls if not properly understood.
In this blog post, we will delve into what static nested classes are, their advantages, and the common mistakes developers make. We will also provide clear code examples and commentary to highlight critical points that enhance your understanding of their usage.
What are Static Nested Classes?
A static nested class is a nested class that can be accessed without needing to instantiate its enclosing class. This makes it distinct from the other types of nested classes, which require an instance of the enclosing class.
Key Features of Static Nested Classes
-
Independent of Instance Variables: Static nested classes do not have access to instance variables or methods of the outer class unless you explicitly use an object of the outer class.
-
Enhanced Readability: By grouping related classes together, static nested classes can lead to a cleaner and more understandable code structure.
-
Static Context: They can have static fields and methods of their own.
Basic Syntax of Static Nested Class
Here is a simple example to showcase the syntax of static nested classes in Java:
class OuterClass {
static int outerStaticVariable = 10;
static class StaticNestedClass {
void display() {
System.out.println("Outer static variable: " + outerStaticVariable);
}
}
}
Explanation of the Code
-
OuterClass: Contains a static variable named
outerStaticVariable
. -
StaticNestedClass: This nested class is declared static and has a method
display
that prints the value ofouterStaticVariable
. Notice that it directly references the outer static variable without needing an outer class instance.
Advantages of Using Static Nested Classes
1. Logical Grouping of Classes
Static nested classes allow you to logically group classes that will only be used in one place. By doing this, you enhance encapsulation and reduce the chance of naming conflicts. Grouping related classes makes it easy to maintain them.
2. Performance Optimizations
Since a static nested class does not have a reference to its enclosing class, it can be more efficient in terms of memory and performance when you don’t need to rely on the instance context of the outer class.
3. Simplified Code Structure
Static nested classes can simplify code by separating concerns. For example, if you are designing a data structure, separating the data and algorithms into different classes can be beneficial.
Common Pitfalls and How to Avoid Them
Despite their benefits, developers regularly fall into pitfalls when using static nested classes. Here, we'll discuss the most common mistakes and best practices.
Pitfall 1: Overcomplicating Design
One common mistake is overusing static nested classes for trivial purposes. If a class has no real association with the outer class, it might be better off in its own file.
Recommendation: Use static nested classes when you have a clear relationship with the outer class. If not, consider creating a separate class.
Example:
class Outer {
// A trivial nested class
static class UnrelatedNested {
// This class does not really need to be nested
}
}
Pitfall 2: Confusion with Instance and Static Context
Another common error comes from confusion surrounding the use of instance and static context. Developers sometimes attempt to access instance variables of the outer class directly within the static nested class, which results in a compile-time error.
Recommendation: Always remember that static nested classes cannot directly access non-static outer class members.
Code Example:
class OuterClass {
int outerVar = 5;
static class StaticNestedClass {
void show() {
// Un-commenting the following line will cause a compile-time error
// System.out.println(outerVar); // Error: Cannot make a static reference to the non-static field outerVar
}
}
}
Pitfall 3: Improper Use of Static Context
Using static members in a nested class without understanding their relationship with the outer class can lead to unintended consequences. For instance, if your nested static class has states that depend on the outer class' instance variables, you will encounter issues.
Recommendation: Be cautious with static context. Use it sparingly and ensure it aligns appropriately with your design philosophy.
Example of Proper Use:
class Outer {
private static String outerStatic = "Hello";
static class Nested {
static void nestedMethod() {
// Accessing outer class static member
System.out.println(outerStatic);
}
}
}
Here, Nested
properly utilizes the outer class's static member.
Pitfall 4: Not Leveraging Proper Access Modifiers
It is crucial to consider access modifiers. If a nested class is not meant to be accessed outside of the outer class, it should be private. Exposing static nested classes unnecessarily can lead to misuse and increased coupling.
Recommendation: Always set appropriate access levels depending on your design needs.
Summary of Common Pitfalls:
- Avoid overcomplicating your design with trivial nested classes.
- Maintain a clear understanding of instance variables vs. static context.
- Use nested classes when it makes sense from a design standpoint.
- Set proper access modifiers to preserve encapsulation.
To Wrap Things Up
Static nested classes are a powerful feature in Java that, when used correctly, can greatly enhance the maintainability, readability, and performance of your code. Being mindful of the common pitfalls discussed in this article will help you leverage nested classes effectively.
If you're looking to deepen your understanding further, consider checking out these resources:
By cultivating a proper understanding, you'll not only avoid potential issues, but you'll also empower your programming skills with the knowledge to use static nested classes effectively. Happy coding!
Checkout our other articles