Common JWT Pitfalls When Securing Javalin REST APIs
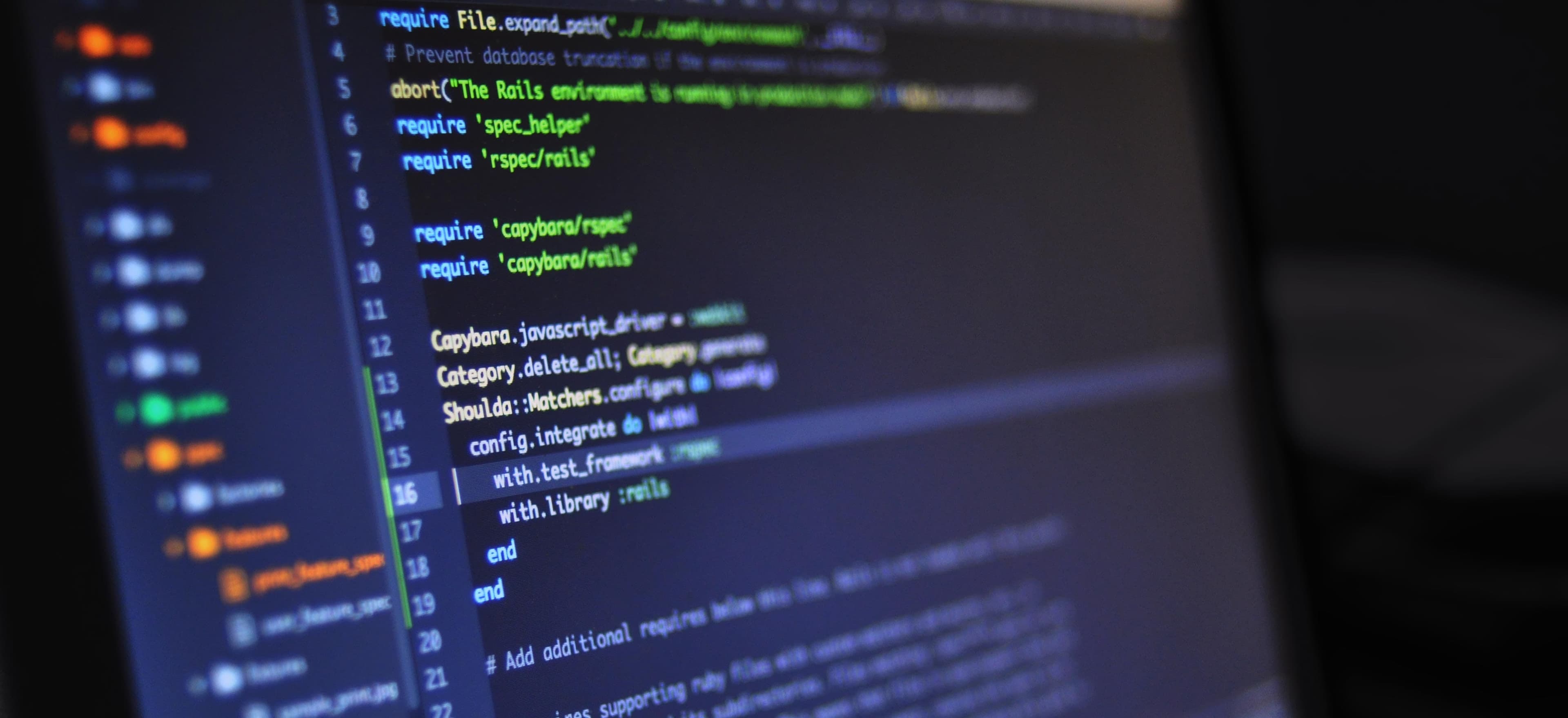
- Published on
Common JWT Pitfalls When Securing Javalin REST APIs
In the realm of API security, JSON Web Tokens (JWT) have emerged as a popular choice for authentication mechanisms. REST APIs, coupled with robust frameworks like Javalin, can leverage JWT for secure data exchanges. However, implementing JWT is not without its challenges. In this blog post, we'll explore some of the common pitfalls when securing Javalin REST APIs using JWT, while offering practical solutions and code examples.
What is JWT?
JSON Web Tokens (JWT) are compact, URL-safe tokens used for securely transmitting information between parties. The information is encoded in a JSON object, which can be verified and trusted using a digital signature. Tokens are used extensively in authentication processes, where a server issues a token to clients after validating their credentials.
For a comprehensive guide on JWT, visit jwt.io.
Common Pitfalls in JWT Implementation
1. Not Using HTTPS
Pitfall: One of the most fundamental mistakes is neglecting secure communications by not utilizing HTTPS. Tokens are often sent as part of the HTTP headers, and transmitting them over unencrypted connections can lead to interception by malicious actors.
Solution: Always enforce HTTPS in your API using a valid SSL certificate.
2. Weak Signing Algorithms
Pitfall: Some developers fall into the trap of using weak or none algorithms for signing tokens. Not specifying a secure algorithm can lead to vulnerabilities.
Solution: Always use strong algorithms, like RS256.
Here is an example of how to generate a JWT using RS256 in Java:
import io.jsonwebtoken.Jwts;
import io.jsonwebtoken.SignatureAlgorithm;
public String createJWT(String subject) {
return Jwts.builder()
.setSubject(subject)
.setIssuedAt(new Date())
.setExpiration(new Date(System.currentTimeMillis() + 864_000_000)) // 10 days
.signWith(SignatureAlgorithm.RS256, privateKey) // Ensure you're using a strong signing algorithm
.compact();
}
3. Storing Secrets Securely
Pitfall: Hardcoding secret keys in your source code can easily expose them to unauthorized access.
Solution: Use environment variables or a secrets management tool to store keys securely.
// Example of retrieving a secret key from environment variables
String secretKey = System.getenv("JWT_SECRET");
4. Token Expiration Mismanagement
Pitfall: Not implementing token expiration or using overly long expiration times can leave your API vulnerable.
Solution: Implement short-lived access tokens with refresh tokens.
In the JWT creation example above, we set the expiration to 10 days. For better security, consider generating a refresh token:
public String createRefreshToken(String subject) {
return Jwts.builder()
.setSubject(subject)
.setIssuedAt(new Date())
.setExpiration(new Date(System.currentTimeMillis() + 604_800_000)) // 7 days
.signWith(SignatureAlgorithm.RS256, privateKey)
.compact();
}
5. Inadequate Claims Usage
Pitfall: Developers might overlook the importance of claims. Claims contain metadata about the token, and inadequate claims can lead to trust issues.
Solution: Always include relevant claims in your JWT. For example, include user roles, token issuer, and audience.
public String createJWTWithClaims(String subject, String role) {
return Jwts.builder()
.setSubject(subject)
.claim("role", role)
.setIssuer("example.com")
.setAudience("app-users")
.setIssuedAt(new Date())
.setExpiration(new Date(System.currentTimeMillis() + 864_000_000))
.signWith(SignatureAlgorithm.RS256, privateKey)
.compact();
}
Building on this, you can assess user permissions on each request based on their role.
6. Not Validating Tokens Properly
Pitfall: Failing to validate JWTs properly during API calls may lead to unauthorized access. This includes validating the signature, claims, and expiration date.
Solution: Always ensure complete validation of incoming tokens.
import io.jsonwebtoken.Claims;
import io.jsonwebtoken.Jws;
import io.jsonwebtoken.Jwts;
public Claims validateJWT(String token) {
return Jwts.parser()
.setSigningKey(publicKey)
.parseClaimsJws(token)
.getBody(); // This will throw an exception if the token is invalid or expired
}
7. Handling Token Revocation
Pitfall: JWT doesn’t have a built-in revocation mechanism which can be dangerous if tokens need to be invalidated before expiration.
Solution: Implement a revocation strategy such as a token blacklist or a database that records active tokens.
8. Relying on Client-Side Validation
Pitfall: Relying too heavily on client-side validation can lead to security holes. Clients may be compromised, making server-side checks essential.
Solution: Always validate JWTs on the server side and enforce checks such as role permissions and more.
9. Ignoring Logout Functionality
Pitfall: Overlooking the requirement to log users out can be problematic. If a user logs out, the token should be invalidated.
Solution: Consider adding token invalidation logic or a blacklist when a user logs out.
10. Excessive Claims
Pitfall: Including an excessive amount of claims can bloat your JWT and cause performance issues.
Solution: Include only essential claims to keep your tokens lean.
To Wrap Things Up
Implementing JWT in your Javalin REST APIs provides a modern approach to secure authentication and authorization. However, it is paramount to navigate common pitfalls effectively.
Remember to always enforce HTTPS, use robust signing algorithms, manage token expiration assiduously, and validate tokens accurately. Possible enhancements include leveraging environment variables for secrets, incorporating claims judiciously, and developing a proper revocation system.
Always keep security as the forefront of your implementation processes. Don't just adopt JWT for the sake of it; understand the best practices and shadow the potential pitfalls to create a resilient system.
For additional resources on JWT implementation, consider reading the official documentation of Javalin.
Happy coding!