Mastering Stack Overflow: Common Java Stack Issues Explained
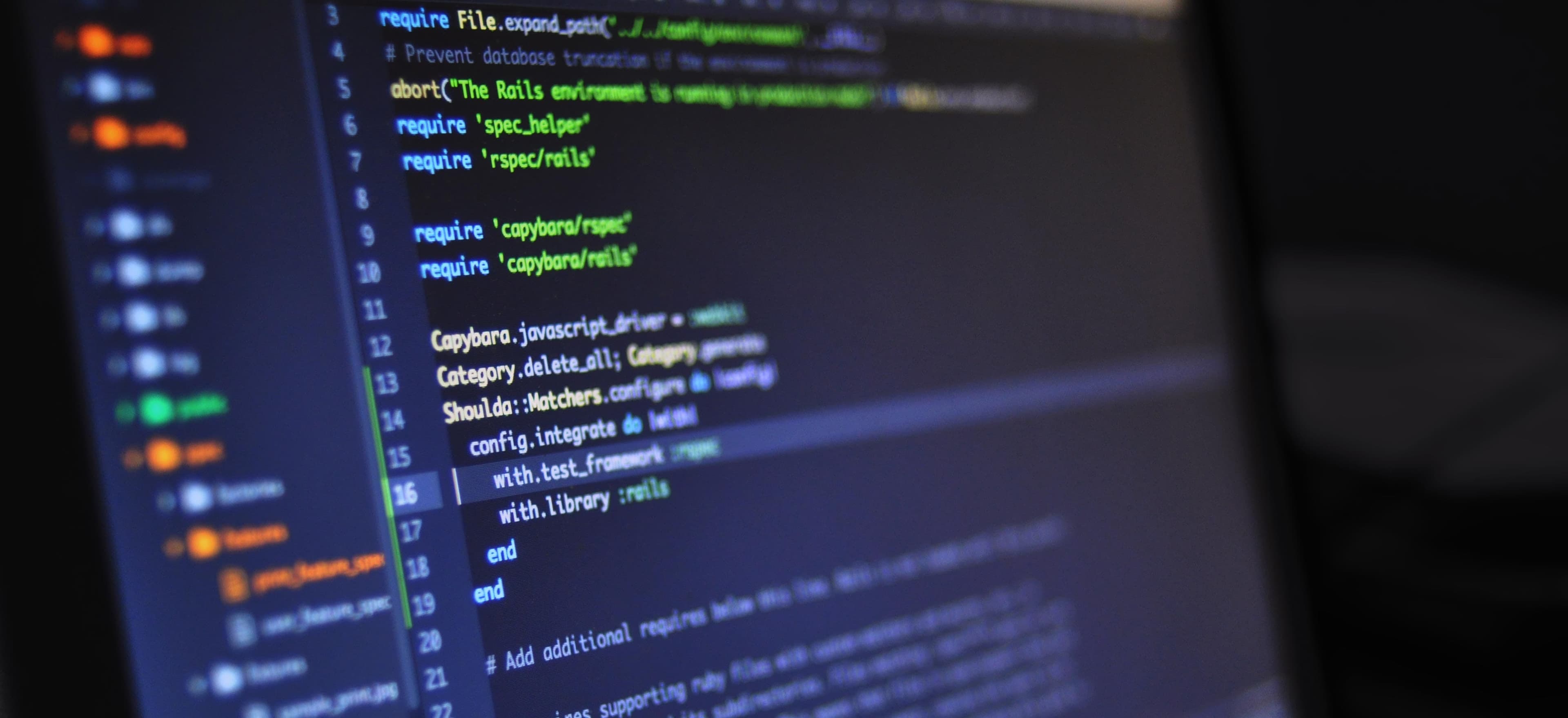
- Published on
Mastering Stack Overflow: Common Java Stack Issues Explained
Java is a powerful and versatile programming language that has gained immense popularity among developers around the globe. While Java offers many features that simplify the programming process, it also introduces complexities that can lead to common issues. One such issue is the dreaded Stack Overflow error. In this blog post, we’ll explore what a Stack Overflow error is in the context of Java, its common causes, and how to debug and resolve these issues.
What is a Stack Overflow?
A Stack Overflow error occurs when a program uses more stack memory than is allocated by the Java Virtual Machine (JVM). The stack memory is a region in RAM where the JVM keeps track of method calls, local variables, and other temporary data. When a method is called, a new frame is added to the stack. If too many frames are added (often due to infinite recursion or excessive method calls), the stack will overflow, leading to a StackOverflowError
.
Why is Stack Memory Important?
Understanding the importance of stack memory is crucial for any Java developer. Stack memory:
- Stores method calls: Each time a method is called, a new frame is pushed onto the stack for execution. If there are too many nested calls, it fills up quickly.
- Contains local variables: Each method's local variables are stored in their respective stack frames. If stack memory is exhausted, the JVM will throw a
StackOverflowError
.
Common Causes of Stack Overflow
1. Infinite Recursion
One of the most common causes of a Stack Overflow error is infinite recursion. This occurs when a method continuously calls itself without a proper base case to terminate the calls. Here’s an example:
public class Demo {
public void recursiveMethod() {
// No termination condition
recursiveMethod();
}
public static void main(String[] args) {
Demo demo = new Demo();
demo.recursiveMethod();
}
}
Why: In the above example, recursiveMethod
calls itself indefinitely. As a result, new stack frames are created without releasing the previous ones, leading to a Stack Overflow.
Solution: Always ensure that recursive methods have an appropriate termination condition to prevent infinite calls. Consider the following adjustment:
public class Demo {
public void recursiveMethod(int count) {
if (count == 0) {
return; // Base condition
}
recursiveMethod(count - 1);
}
public static void main(String[] args) {
Demo demo = new Demo();
demo.recursiveMethod(5);
}
}
2. Deeply Nested Method Calls
Another scenario leading to Stack Overflow is deeply nested method calls. When methods call one another in a chain without returning, it can fill up the stack quickly. For example:
public class DeepNesting {
public void methodA() {
methodB();
}
public void methodB() {
methodC();
}
public void methodC() {
methodD();
}
public void methodD() {
methodA(); // Causes deep nesting
}
public static void main(String[] args) {
DeepNesting demo = new DeepNesting();
demo.methodA();
}
}
Why: In this case, the methods indirectly call each other, creating a cycle of calls. This can lead to a Stack Overflow if not handled properly.
Solution: This structure can be redesigned to break the cycle or limit the depth of calls. Alternatively, consider refactoring the code to use iteration instead of recursion.
3. Flawed Algorithm Design
Sometimes, a poor design of recursion algorithms can lead to unexpected depth, resulting in Stack Overflow errors. A classic example is implementing a Fibonacci sequence using a naive recursive approach:
public class Fibonacci {
public int fibonacci(int n) {
if (n <= 1) return n;
return fibonacci(n - 1) + fibonacci(n - 2); // Poor performance
}
public static void main(String[] args) {
Fibonacci fib = new Fibonacci();
System.out.println(fib.fibonacci(50)); // This will throw StackOverflowError
}
}
Why: The recursion here grows exponentially, causing too many stack frames due to the nature of how Fibonacci is calculated.
Solution: Optimize the algorithm using dynamic programming or memoization to store previously computed results.
import java.util.HashMap;
public class Fibonacci {
private HashMap<Integer, Integer> cache = new HashMap<>();
public int fibonacci(int n) {
if (n <= 1) return n;
if (cache.containsKey(n)) return cache.get(n);
int result = fibonacci(n - 1) + fibonacci(n - 2);
cache.put(n, result);
return result;
}
public static void main(String[] args) {
Fibonacci fib = new Fibonacci();
System.out.println(fib.fibonacci(50)); // Improved performance
}
}
How to Debug Stack Overflow Issues
Debugging stack overflow errors can be challenging. Here are some practical tips:
1. Read the Stack Trace
Always examine the stack trace provided in the error message. It gives insight into the call hierarchy leading to the overflow, helping to pinpoint the offending method.
2. Use Debugger Tools
Leverage IDE debugging tools to step through the code execution. This allows you to observe how method calls unfold and check variable values at runtime.
3. Review Recursive Calls
Carefully analyze any recursive methods. Ensure that they have proper base cases that conditionally exit the recursion.
4. Analyze Method Interactions
If multiple methods interact, chart the call relationships. Tools like UML diagrams can help visualize complex call graphs, making it easier to identify deep nesting problems.
Key Takeaways
Mastering Stack Overflow issues in Java requires a solid understanding of stack memory, recursion, and algorithm design. Common pitfalls include infinite recursion, deep nesting of method calls, and flawed algorithm strategies. By following best practices and utilizing the debugging techniques outlined, developers can effectively address and prevent Stack Overflow errors in their Java applications.
For further reading, you might find these resources helpful:
- Java Stack Overflow Error Documentation
- Understanding Java Call Stack
Understanding these principles will enhance your skills as a developer and enable you to write robust, efficient Java code. Happy coding!