Resolving Deadlocks in Log4j: A Step-by-Step Guide
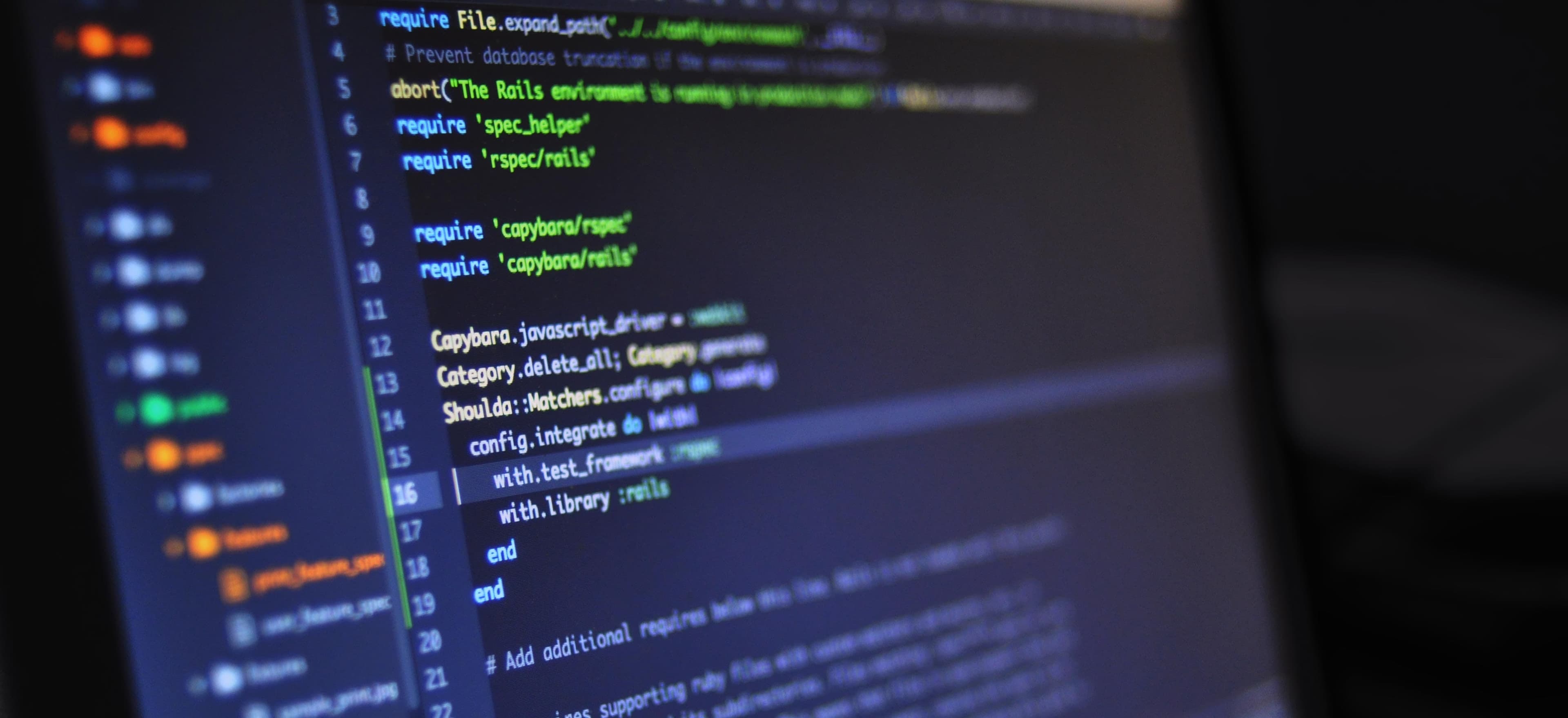
- Published on
Resolving Deadlocks in Log4j: A Step-by-Step Guide
Deadlocks can be a developer's worst nightmare, particularly in multi-threaded applications where multiple threads are vying for resources. In logging frameworks like Log4j, such issues can lead to sporadic application hangs and complicate debugging. This blog post aims to provide a comprehensive guide on understanding and resolving deadlocks specifically within the Log4j framework, while optimizing for Search Engine Optimization (SEO) to enhance discoverability.
Understanding Deadlocks
What is a Deadlock?
A deadlock occurs when two or more threads are blocked forever, each waiting for the other to release a resource. In simpler terms, it’s a standoff where the involved threads cannot progress further.
For example, imagine two threads that hold a lock on separate resources. If Thread A locks Resource 1 and waits for Resource 2, while Thread B locks Resource 2 and waits for Resource 1, neither can proceed—thus, a deadlock.
Deadlocks in Logging
Logging systems like Log4j are often multi-threaded, handling concurrent logging requests from various parts of an application. A deadlock in Log4j can hinder logging operations, making it difficult to evaluate application behavior during critical moments.
Identifying Deadlocks in Log4j
Before jumping into potential solutions, it’s essential first to diagnose whether your Log4j setup is experiencing deadlocks. You can identify deadlocks using tools like:
- Java VisualVM: A powerful tool that provides real-time monitoring and troubleshooting capabilities.
- JConsole: A management console for monitoring Java applications, which allows you to view thread activity and deadlocks.
- Thread Dumps: These can be generated manually or by invoking a JMX command. A thread dump provides crucial insight into the state of all threads.
Here’s how you can initiate a thread dump via the command line:
jstack <pid>
Where <pid>
is the Process ID of your Java application.
Step-by-Step Approach to Resolving Deadlocks
Step 1: Analyze Your Thread Dumps
Upon generating a thread dump, analyze it for deadlocks. Look for lines indicating “Found one Java-level deadlock” followed by the threads involved and the resources they are waiting for.
Example Analysis:
Found one Java-level deadlock:
"Thread-1":
waiting to lock <0x00007f8c8c004358> (a java.lang.Object),
which is held by "Thread-2"
"Thread-2":
waiting to lock <0x00007f8c8c004368> (a java.lang.Object),
which is held by "Thread-1"
In this example, Thread-1 is waiting for a lock held by Thread-2 and vice versa. This indicates a classic deadlock situation.
Step 2: Review Your Logger Configuration
The configuration of your Log4j can significantly influence the occurrence of deadlocks. Check for inappropriate logger configurations that may cause multiple threads to compete for the same resources.
<Configuration status="WARN">
<Appenders>
<Console name="Console" target="SYSTEM_OUT">
<PatternLayout pattern="%d{HH:mm:ss} %-5p %c{1} - %m%n"/>
</Console>
</Appenders>
<Loggers>
<Root level="info">
<AppenderRef ref="Console"/>
</Root>
</Loggers>
</Configuration>
Step 3: Introduce Locking Mechanisms
If you find that certain operations lead to deadlocks, consider introducing more granular locking. The synchronized keyword in Java can help control access to critical sections.
public class LogHandler {
private final Object lock1 = new Object();
private final Object lock2 = new Object();
public void logAction1() {
synchronized (lock1) {
// Perform action
logAction2(); // Avoid invoking locking methods from here without proper management
}
}
public void logAction2() {
synchronized (lock2) {
// Perform action
}
}
}
Step 4: Implement Timeout Handling
In cases where you're unsure whether an operation will succeed, consider implementing timeouts. This basic strategy helps prevent threads from waiting indefinitely. Here’s how to implement a timeout using java.util.concurrent.locks
.
Lock lock = new ReentrantLock();
boolean gotLock = false;
try {
gotLock = lock.tryLock(1000, TimeUnit.MILLISECONDS);
if (gotLock) {
// Perform logging action
}
} catch (InterruptedException e) {
// Handle the exception
} finally {
if (gotLock) {
lock.unlock();
}
}
Step 5: Utilize Asynchronous Logging
Log4j provides an asynchronous logging feature. This decouples the logging process from the application, reducing contention:
<AsyncLogger name="AsyncLogger" level="info">
<AppenderRef ref="Console"/>
</AsyncLogger>
By using asynchronous logging, you reduce the risk of deadlocks since logging operations do not block the main application threads.
Step 6: Upgrade Your Dependencies
Sometimes deadlocks are mitigated by bug fixes in more recent versions of your logging library. Always ensure you are using the latest stable version of Log4j to leverage improvements. You can check for the latest release on Apache Log4j's official website.
The Bottom Line
Deadlocks in Log4j can be an insidious problem that manifests in unpredictable ways. By understanding thread behavior, analyzing configurations, and implementing the above-mentioned strategies, you can significantly reduce the likelihood of encountering deadlocks in your applications.
Always remember, when it comes to troubleshooting, methodical analysis is your best friend. A clear understanding of thread interactions, combined with proper Lock management, logging practices, and an occasional code review, will go a long way in optimizing your logging system.
For deeper insights on managing concurrency in Java, consider exploring Concurrency in Practice. Keep your logs clean and your application robust!
By following this step-by-step guide, you’re equipped to manage and resolve deadlocks effectively in Log4j. Happy Coding!
Checkout our other articles