Scaling Challenges in Distributed Deep Learning with Caffe
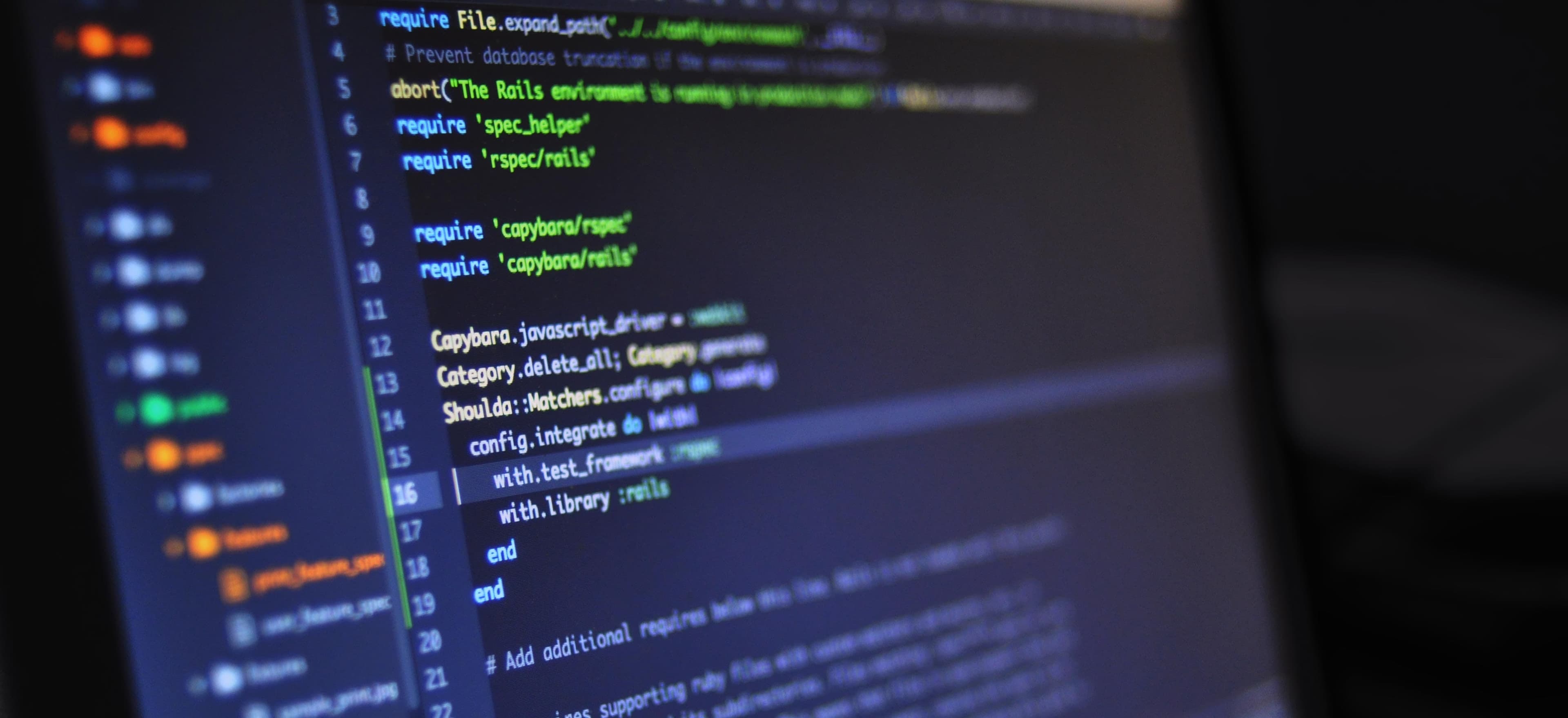
- Published on
Scaling Challenges in Distributed Deep Learning with Caffe
Deep learning has transformed various fields, from computer vision to natural language processing. As its applications expand, so does the demand for scalable solutions. One framework that has made a significant impact in this space is Caffe, an open-source deep learning framework favored for its speed and modularity. However, distributed deep learning poses numerous challenges, particularly in terms of scalability.
In this blog post, we will explore the scaling challenges inherent in distributed deep learning using Caffe, discuss effective strategies to overcome these difficulties, and provide practical code snippets to illustrate our points.
Understanding Distributed Deep Learning
Before diving into the challenges, it's crucial to define what distributed deep learning is. Distributed deep learning refers to the practice of training neural networks across multiple machines or nodes. This approach is essential for handling large datasets and complex models that would be impossible to manage on a single machine.
The Advantages of Using Caffe
Caffe is known for several advantages:
- Performance: Caffe's architecture allows for high speed and efficiency.
- Flexibility: It supports various layers and allows users to easily define custom layers.
- Modularity: The model can be decomposed into simpler, reusable components.
However, while Caffe has many strengths, scaling with it comes with specific challenges.
Scaling Challenges
1. Data Distribution
One of the most significant challenges in distributed deep learning is data distribution. Efficiently splitting the dataset across nodes while ensuring that each model can learn effectively is crucial.
Key Considerations
- Data Sharding: The dataset must be divided correctly to prevent overlap and ensure unique data is utilized by each node.
Example Code Snippet: Data Sharding
def shard_data(data, num_shards):
"""Splits the data into num_shards parts."""
shard_size = len(data) // num_shards
return [data[i*shard_size:(i+1)*shard_size] for i in range(num_shards)]
Commentary: This function splits a dataset into equal parts, ensuring that each worker node gets a unique subset. Efficient data sharding improves training speed and minimizes redundancy, thus enhancing model convergence.
2. Synchronization
In a distributed environment, synchronization is vital. Updating model weights across different nodes can result in inconsistencies if not carefully managed.
Key Considerations
- Asynchronous vs. Synchronous Updates: Choosing between these methods affects convergence speed and model performance.
Example Code Snippet: Synchronous Update
def synchronous_update(global_weights, local_weights, learning_rate):
"""Updates the global model weights synchronously."""
updated_weights = {}
for key in global_weights.keys():
updated_weights[key] = global_weights[key] + (learning_rate * local_weights[key])
return updated_weights
Commentary: This update function modifies the global model weights by incorporating local updates based on a defined learning rate. Synchronous updates provide consistency but may introduce delays, especially with a large number of nodes.
3. Communication Overhead
The communication between various nodes can become a bottleneck. Each model iteration may require synchronization of weights, which involves passing data over the network.
Key Considerations
- Network Latency: Reducing the time taken in communication is crucial for performance.
- Efficient Communication Protocols: Choosing the right protocol can enhance throughput.
Example Code Snippet: Using MPI for Communication
from mpi4py import MPI
def broadcast_weights(weights):
"""Broadcasts model weights using MPI."""
comm = MPI.COMM_WORLD
comm.Bcast(weights, root=0)
return weights
Commentary: This function leverages the Message Passing Interface (MPI) to broadcast model weights from one node to all others. Utilizing efficient communication frameworks can mitigate the overhead significantly.
4. Fault Tolerance
In a distributed training setup, nodes can fail due to hardware issues, network problems, or other unexpected circumstances. Lack of fault tolerance can lead to lost progress and wasted resources.
Key Considerations
- Checkpointing: Regularly saving model states is critical to ensure that progress is not lost.
Example Code Snippet: Checkpointing
import pickle
def save_checkpoint(model, filename):
"""Saves the current state of the model."""
with open(filename, 'wb') as f:
pickle.dump(model.state_dict(), f)
Commentary: This simple function saves a model’s state to a file. Checkpointing is essential in long-running processes typical of deep learning training, as it allows the process to resume in case of failure.
Strategies for Effective Scaling
Having identified the challenges, we can discuss strategies to tackle them.
Use of Efficient Data Loaders
Utilize efficient data loaders to ensure that the data is preloaded into memory and ready for use at all times. Libraries such as Dataloader in PyTorch can help manage this effectively.
Dynamic Load Balancing
Employing dynamic load balancing strategies can help distribute workload evenly across all nodes, reducing idle time and improving resource utilization.
Hyperparameter Tuning
Conduct comprehensive hyperparameter tuning across multiple nodes. Adjusting parameters such as batch size and learning rate can yield significant performance improvements.
Monitoring Systems
Implement robust monitoring systems to track node performance. Tools like Prometheus can help visualize resource consumption, allowing for quicker adjustments to be made.
To Wrap Things Up
Distributed deep learning is essential for tackling modern problems with large datasets and complex models. While Caffe presents unique challenges related to data distribution, synchronization, communication overhead, and fault tolerance, understanding these problems equips developers with the tools needed to address them.
By employing efficient data management strategies, optimizing communication protocols, and maintaining checkpoints, it is possible to scale deep learning processes effectively. Caffe, with its speed and flexibility, remains a powerful choice for deep learning applications, provided users are prepared to navigate its challenges.
For further reading on distributed systems and deep learning, explore TensorFlow’s Distributed Training Guide and Caffe Documentation.
By continuously evolving our understanding and application of distributed deep learning, we can shape the future of machine learning research and applications. Happy coding!