Mastering Java EE: Common CDI Bean Scope Mistakes
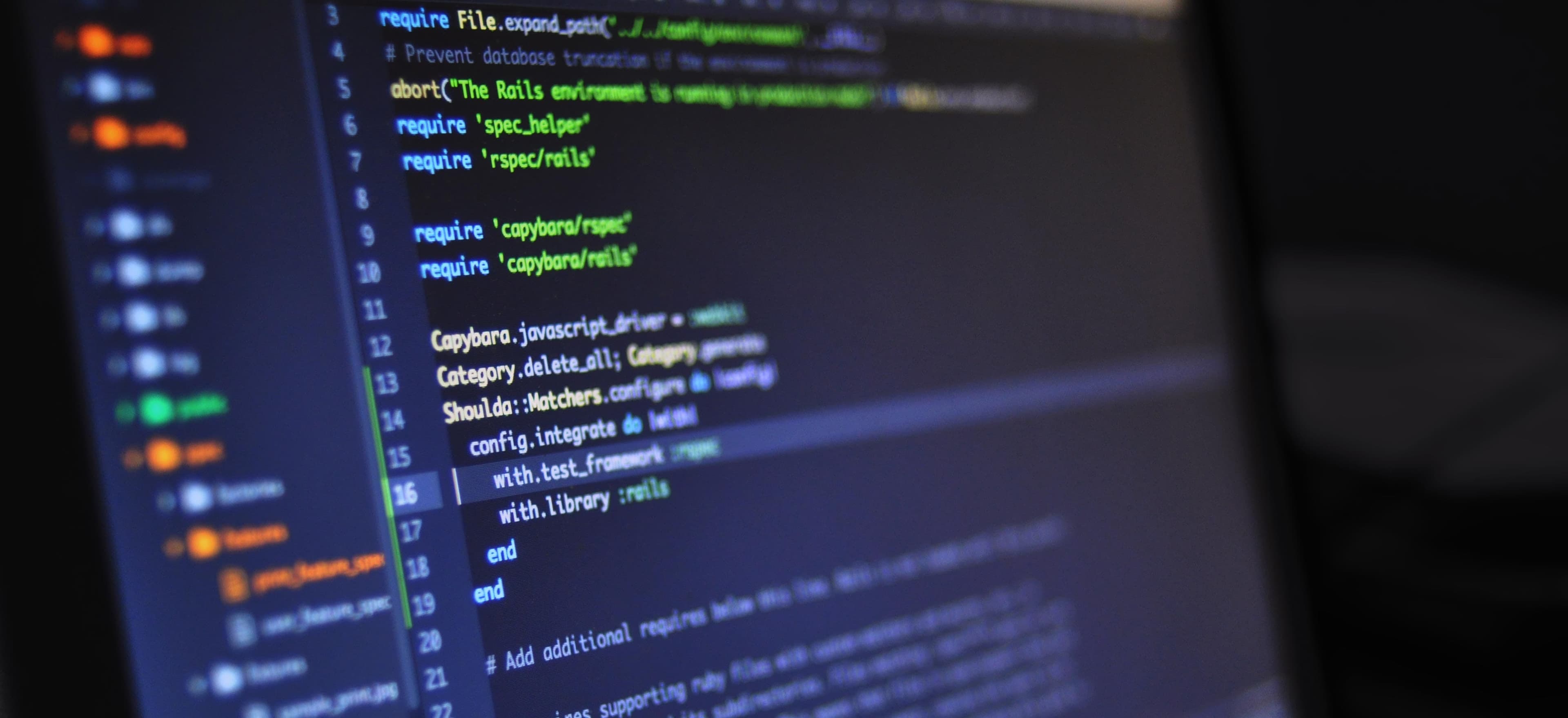
- Published on
Mastering Java EE: Common CDI Bean Scope Mistakes
Java EE, now known as Jakarta EE, remains a powerful framework for building robust, enterprise-level applications. One of its core features is Context and Dependency Injection (CDI), which provides a way to manage the lifecycle of components and their dependencies. However, as developers dive into CDI, they often encounter pitfalls related to bean scope management. This blog post will dive deep into these common mistakes, helping you to master Java EE more effectively.
Understanding CDI Bean Scopes
Before we explore the common mistakes, we should first outline the different scopes that CDI offers:
- REQUEST: A new bean is created for each HTTP request.
- SESSION: A bean instance is created for each HTTP session and shared across all requests within that session.
- APPLICATION: A single instance is created for the entire application lifecycle, available to all users.
- CONVERSATION: This scope is used for managing user interaction across multiple requests while being isolated from other interactions.
- Dependent: The default scope, these beans exist as long as the bean that injects them.
Properly understanding these scopes can help avoid many common mistakes developers make.
Common CDI Bean Scope Mistakes
1. Ignoring Scope Implications
One of the first mistakes developers make is not understanding the implications of the CDI bean scope they select. For example, using the APPLICATION
scope in a multi-user environment can lead to unintended side effects.
Example:
@ApplicationScoped
public class UserService {
private List<User> users = new ArrayList<>();
public void addUser(User user) {
users.add(user);
}
}
Why is this a problem?
If UserService is APPLICATION
scoped, all users share the same instance and the list of users. As a result, one user's data can be visible to others. This could lead to serious security issues or data corruption.
Solution:
Use the appropriate scope per use case. For user-specific operations, consider using SESSION
or REQUEST
scope.
2. Misusing CONVERSATION
Scope
Another common error is the misuse of CONVERSATION
scope, which can cause beans to live longer than intended, often leading to memory leaks or stale data.
Example:
@ConversationScoped
public class ShoppingCart {
private List<Item> items = new ArrayList<>();
public void addItem(Item item) {
items.add(item);
}
}
Why is this a problem?
If you fail to explicitly end the conversation after use, the ShoppingCart
bean will remain in memory, consuming resources unnecessarily.
Solution:
Always manage the conversation lifecycle correctly. Use the CDI @Conversation
object to end the conversation:
@Inject
private Conversation conversation;
public void startConversation() {
conversation.begin();
}
public void endConversation() {
if (conversation.isTransient()) {
return;
}
conversation.end();
}
3. Not Considering Thread Safety
CDI beans are singleton by default in a CDI context, but this doesn’t make them automatically thread-safe. Another common mistake is using APPLICATION
or SESSION
scoped beans without ensuring proper synchronization.
Example:
@ApplicationScoped
public class Counter {
private int count = 0;
public void increment() {
count++;
}
public int getCount() {
return count;
}
}
Why is this a problem?
In a multi-threaded environment, if multiple threads access the Counter
bean simultaneously, one thread may overwrite the changes made by another, leading to unpredictable behavior.
Solution:
Consider using synchronized
methods or Java's AtomicInteger
to maintain thread safety:
@ApplicationScoped
public class Counter {
private AtomicInteger count = new AtomicInteger(0);
public void increment() {
count.incrementAndGet();
}
public int getCount() {
return count.get();
}
}
4. Overusing Dependent
Scope
While Dependent
scope can be useful, overusing it can lead to confusion about the lifecycle of your beans.
Example:
public class ItemProcessor {
@Inject
private Cart cart; // Default Dependent scope
public void processItem(Item item) {
cart.add(item);
}
}
Why is this a problem?
Using Dependent
scope on beans that may be heavy or resource-intensive leads to frequent instantiation, increasing memory pressure and potentially causing performance issues.
Solution:
Only use Dependent
scope for lightweight beans. It might be more efficient to use other scopes like REQUEST
or SESSION
depending on the use case.
5. Assuming Scope Behavior is the Same Across All Contexts
Different Java EE servers may implement CDI differently and can vary in how they handle bean scopes. For instance, using a @SessionScoped
bean in an application server that does not isolate sessions correctly can lead to unexpected behavior.
Solution:
Always check how your application server manages scopes and ensure you perform adequate testing under real-world conditions.
Closing Remarks
Mastering bean scopes in CDI is essential for effective Java EE application development. Mismanagement can lead to data integrity issues, resource inefficiencies, and unpredictable behavior in your applications. By avoiding these common scope mistakes, you can create scalable, maintainable, and robust enterprise applications.
For more reading on Jakarta EE and CDI, check out the Jakarta EE documentation and Java EE tutorial for in-depth insights.
With careful attention to how you manage bean scopes, you’ll be well on your way to mastering Java EE. Happy coding!