Common Java Coding Mistakes to Avoid in 2022
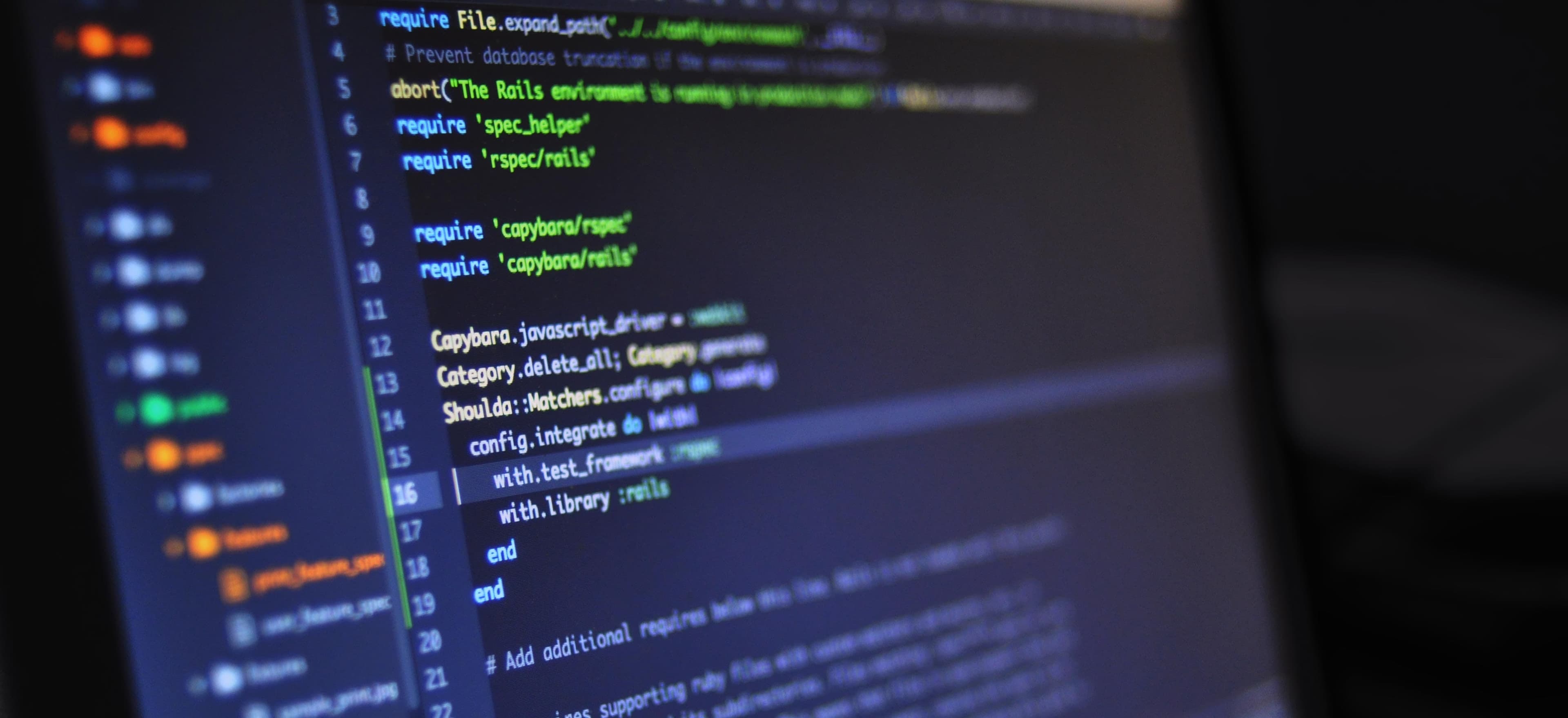
- Published on
Common Java Coding Mistakes to Avoid in 2022
Java is one of the most widely used programming languages, known for its simplicity, reliability, and portability. However, like any programming language, it comes with its own set of challenges. As developers, we all make mistakes, but recognizing and understanding them can significantly improve our coding skills. In this blog post, we will discuss some common Java coding mistakes to avoid—particularly those that are frequently encountered by programmers in 2022.
1. Ignoring Java's NullPointerException
The Issue
One of the most common exceptions in Java is the NullPointerException
. It occurs when your code attempts to use an object reference that has not been initialized. Ignoring this can lead to runtime errors that are difficult to debug.
Solution
Always check for null before using an object. Implement defensive programming practices. Here’s an example:
String name = null;
if (name != null) {
System.out.println(name.length()); // Safe
} else {
System.out.println("Name cannot be null");
}
Why It Matters
By implementing null checks, you can enhance the reliability of your code and prevent unexpected crashes. For more on handling null values in Java, check out the Java documentation on NullPointerException.
2. Overuse of Static Methods
The Issue
While static methods are useful, overusing them can lead to a lack of flexibility and testability in your code. It increases coupling and can be an anti-pattern in object-oriented design.
Solution
Use static methods judiciously. Keep them for utility functions or methods that do not require instance state. Consider using dependency injection (DI) for scenarios where you need to maintain state and behavior.
public class Utility {
private Utility() {} // Private constructor
public static int add(int a, int b) {
return a + b; // Utility function, can be static
}
}
Why It Matters
Relying too much on static methods can hinder your application's scalability. For guidance on good design practices, visit the SOLID principles.
3. Improper Exception Handling
The Issue
Java provides powerful mechanisms for exception handling, but improper usage can lead to swallowed exceptions, poor error messages, and difficulty in tracing bugs.
Solution
Catch specific exceptions and provide meaningful messages. Avoid blank catch blocks at all costs. Here’s how to do it right:
try {
int[] arr = {1, 2, 3};
System.out.println(arr[3]);
} catch (ArrayIndexOutOfBoundsException e) {
System.err.println("Index out of bounds: " + e.getMessage());
}
Why It Matters
Providing clear error handling helps both you and your fellow developers understand why things went wrong and improves maintainability. For further reading, check out the official Java documentation on exception handling.
4. Inefficient Use of Collections
The Issue
Java provides a rich set of collection classes—ArrayList
, HashMap
, TreeSet
, etc. A frequent mistake is mischoosing the collection type, which can lead to performance issues.
Solution
Choose the right collection based on your needs. For example, if you need fast access by key, consider using HashMap
instead of ArrayList
:
Map<String, Integer> ageMap = new HashMap<>();
ageMap.put("John", 30);
ageMap.put("Jane", 25);
System.out.println("John's age: " + ageMap.get("John")); // Fast access
Why It Matters
Selecting the proper collection can dramatically affect the performance of your application. For optimal collection choices, refer to the Java Collections Framework.
5. Misusing the final
Keyword
The Issue
The final
keyword can be an excellent tool when used correctly, but misuse can cause confusion. For instance, declaring a variable as final
when modifications are expected can lead to errors.
Solution
Use final
for constants or immutable instances. Here's an example:
final int MAX_USERS = 100; // Correct usage
Why It Matters
Using the final
keyword appropriately enhances code readability and helps maintain immutable states. To learn more about immutability in Java, check out Immutable objects.
6. Failing to Leverage Java 8 Features
The Issue
Java 8 introduced several enhancements, such as lambda expressions and streams. Failing to utilize these can lead to verbose, less efficient code.
Solution
Embrace newer features to simplify your code. For example, using streams can lead to cleaner, more readable code:
List<String> names = Arrays.asList("John", "Jane", "Doe");
names.stream()
.filter(name -> name.startsWith("J"))
.forEach(System.out::println);
Why It Matters
Using modern Java features can enhance code quality and developmental speed. For a comprehensive look at Java 8 features, check out the Java 8 documentation.
7. Not Following Naming Conventions
The Issue
Poor naming conventions can make code difficult to read and maintain. Avoid cryptic variable names and ensure clarity in your naming patterns across variables and methods.
Solution
Adhere to Java naming conventions:
- Classes: CamelCase (e.g.,
MyClass
) - Methods: camelCase (e.g.,
myMethod
) - Variables: camelCase (e.g.,
myVariable
)
public class DataProcessor {
public void processData(List<String> dataList) {
// Process data
}
}
Why It Matters
Consistent naming enhances code readability and facilitates collaboration. For more on best coding practices, visit Java's official conventions documentation.
8. Not Writing Unit Tests
The Issue
Failing to write unit tests can result in untested, unreliable code.
Solution
Use frameworks like JUnit to create tests for your methods. Here’s a simple unit test example:
import org.junit.Test;
import static org.junit.Assert.assertEquals;
public class CalculatorTest {
@Test
public void testAdd() {
Calculator calc = new Calculator();
assertEquals(5, calc.add(2, 3));
}
}
Why It Matters
Unit testing ensures that your code behaves as expected, making it easier to identify and fix errors. For more about unit testing in Java, check out the JUnit documentation.
The Closing Argument
Mistakes are a natural part of the coding process, but understanding common pitfalls can help you avoid them and write better Java code. As we move further into 2022, being mindful of these coding errors will not only improve your own skills but also contribute to the development of higher-quality software. Adopting best practices and leveraging modern programming techniques will enhance your effectiveness as a Java developer.
By recognizing and avoiding these common mistakes, you set the foundation for better code, improve your team's collaboration, and ultimately your software's success in the real world. Happy coding!
For further reading and resources, explore more about Java programming at Oracle's Java Tutorials.