Overcoming Common Scalability Pitfalls in Akka
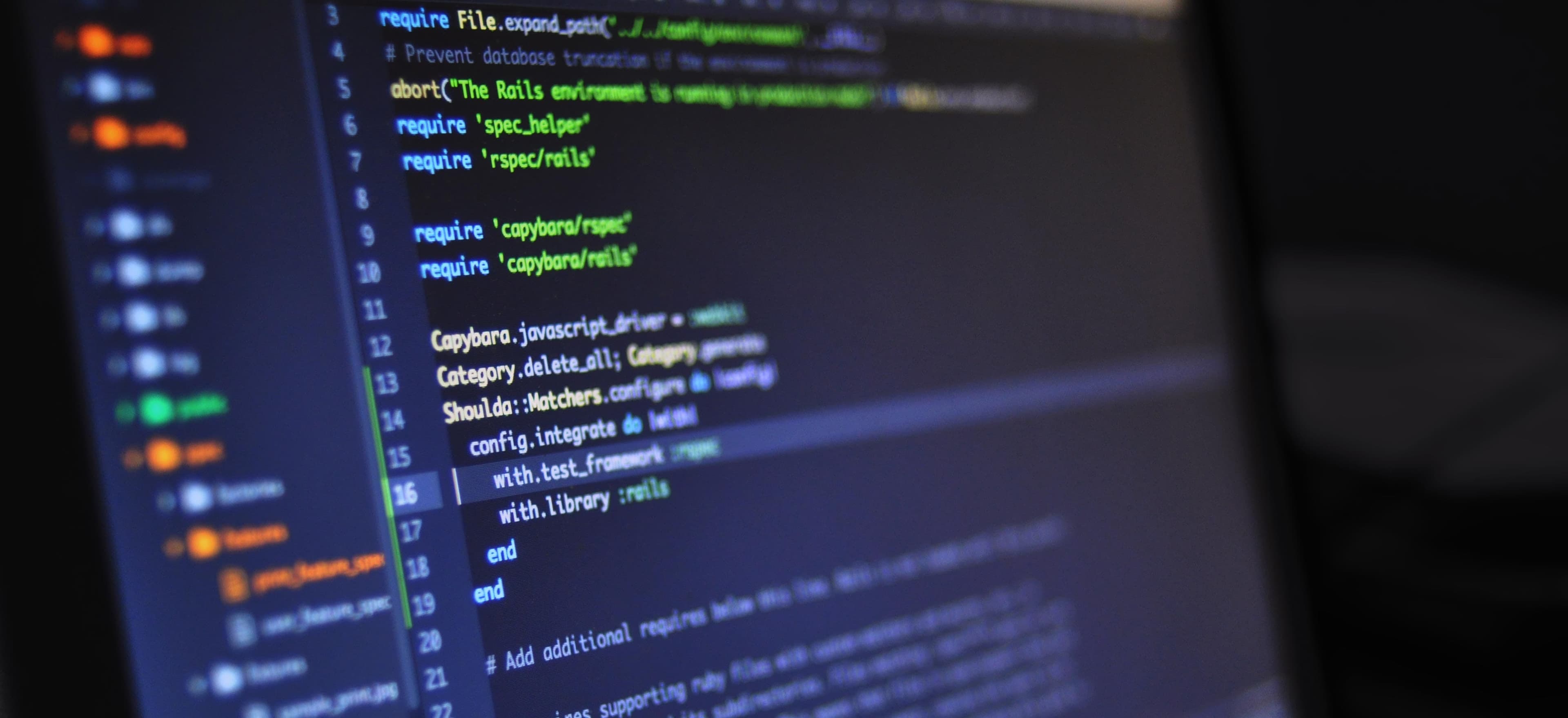
- Published on
Overcoming Common Scalability Pitfalls in Akka
When it comes to building resilient and scalable applications, Akka offers an acclaimed toolkit in the realm of distributed systems. As a framework centered around the Actor Model, Akka provides a means to efficiently manage concurrency and state while promoting a responsive application architecture. However, like any powerful tool, it is crucial to understand and navigate common scalability pitfalls when using Akka. This blog post will outline these pitfalls, while also providing best practices and design patterns to help developers overcome them.
Understanding Scalability in Akka
Before diving into the common pitfalls, it’s essential to grasp what scalability entails in the context of Akka. Scalability is the capability of a system to handle growth, whether in terms of increasing user sessions, data volume, or request handling. Akka achieves this through its actor-based paradigm, enabling separate components to function independently and communicate asynchronously.
Why Akka?
Akka provides several key features that enhance scalability:
- Actor Model: Encapsulates state and behavior, allowing for cleaner designs.
- Fault Tolerance: Built-in supervision strategies manage actor lifecycles.
- Elasticity: Can scale horizontally across distributed nodes.
These features make it easier to design scalable systems, but pitfalls still lurk beneath the surface.
Common Scalability Pitfalls in Akka
1. Misusing Actor Lifecycle
One frequent mistake developers make is neglecting the actor lifecycle management. Actors should be short-lived and designed for a specific task. Using actors as stateful singletons can lead to bottlenecks.
Example:
Here is a typical misuse where an actor handles a persisting job continuously:
class LongRunningActor extends Actor {
def receive = {
case JobRequest(data) =>
performLongRunningTask(data)
}
def performLongRunningTask(data: String): Unit = {
// Simulating long work
Thread.sleep(10000) // Long delay
sender() ! JobResult("Task completed")
}
}
Commentary:
In this code snippet, performLongRunningTask
simulates a time-consuming operation. While processing, this actor becomes unresponsive, resulting in blocked threads. Instead, consider using a pool of shorter-lived actors that can process tasks concurrently.
2. Overusing Actor Communication
Each time actors communicate, it may seem natural to send messages for all interactions. However, excessive communication can diminish performance, particularly if actors exist on different nodes.
Example:
class OrchestratorActor extends Actor {
val worker = context.actorOf(Props[WorkerActor], "worker")
def receive = {
case Orchestrate =>
worker ! WorkRequest("Do something heavy")
worker ! WorkRequest("Do something else")
}
}
Commentary:
This snippet sends multiple work requests to a worker actor without any batching or conditional logic. Instead, consider sending one aggregated message:
class OrchestratorActor extends Actor {
val worker = context.actorOf(Props[WorkerActor], "worker")
def receive = {
case Orchestrate =>
val workRequests = List("Do something heavy", "Do something else")
worker ! WorkRequests(workRequests) // Batching requests
}
}
3. Failing to Monitor Performance
As your application scales, monitoring performance inevitably becomes critical. Failing to implement monitoring tools can result in overlooking bottlenecks or actor failures.
Solution:
Integrate Akka's built-in metrics tools or third-party solutions such as Kamon for monitoring:
import kamon.Kamon
Kamon.start()
// Example of tracking an actor's processing time
class MonitoringActor extends Actor {
def receive = {
case msg =>
val timer = Kamon.timer("actor.processingTime")
timer.start()
// Handle the message
timer.stop()
}
}
Commentary:
In this code, the Kamon library is used to track processing time. Such metrics can help identify slow points in the application and facilitate scaling decisions.
4. Ignoring Backpressure
In high-throughput systems, it’s essential to manage the flow of messages so that the system does not get overwhelmed. Ignoring backpressure can lead to crashes due to unhandled messages piling up.
Solution:
Incorporate backpressure mechanisms, such as:
- Buffers: Allow actors to store messages temporarily.
- Rate Limiting: Slow down the rate of incoming messages.
class RateLimitedActor extends Actor {
override def preStart(): Unit = {
context.setReceiveTimeout(30.seconds) // Rate limiting example
}
def receive = {
case msg => ProcessMessage(msg)
}
def ProcessMessage(msg: String): Unit = {
// Process logic here
}
}
Commentary:
Setting a receive timeout prevents the actor from accumulating too many messages and allows for better management of message flow.
5. Inadequate Resource Management
Finally, developers often ignore the provisioning of adequate resources, such as memory, threads, and CPUs. Underestimating these factors can significantly hinder performance and scalability.
Solution:
Utilize Akka's dispatchers effectively to manage threading:
# application.conf
my-dispatcher {
type = "Dispatcher"
executor = "fork-join-executor"
fork-join-executor {
parallelism-min = 2
parallelism-factor = 2.0
}
}
Commentary:
By defining a custom dispatcher, you ensure that actors have the right resources and parallelism to execute tasks efficiently.
Lessons Learned
While Akka provides a robust framework for building scalable applications, missteps can lead to performance issues. By understanding and addressing the pitfalls discussed above—such as misusing actor lifecycles, overusing actor communication, failing to monitor performance, ignoring backpressure, and inadequate resource management—you can create a more resilient and scalable system.
Adopting best practices, such as monitoring with Kamon or integrating backpressure mechanisms, is vital in overcoming these challenges. Make sure to thoroughly test your Akka implementations and apply the strategies outlined in this blog post to truly harness the power of Akka for scalable software solutions.
For further reading on Akka scalability best practices, consider the official Akka documentation here and explore community discussions on common pitfalls on forums like Stack Overflow.
Happy coding, and may your Akka applications thrive!