Mastering Java 8 Streams: Grouping by Multiple Fields
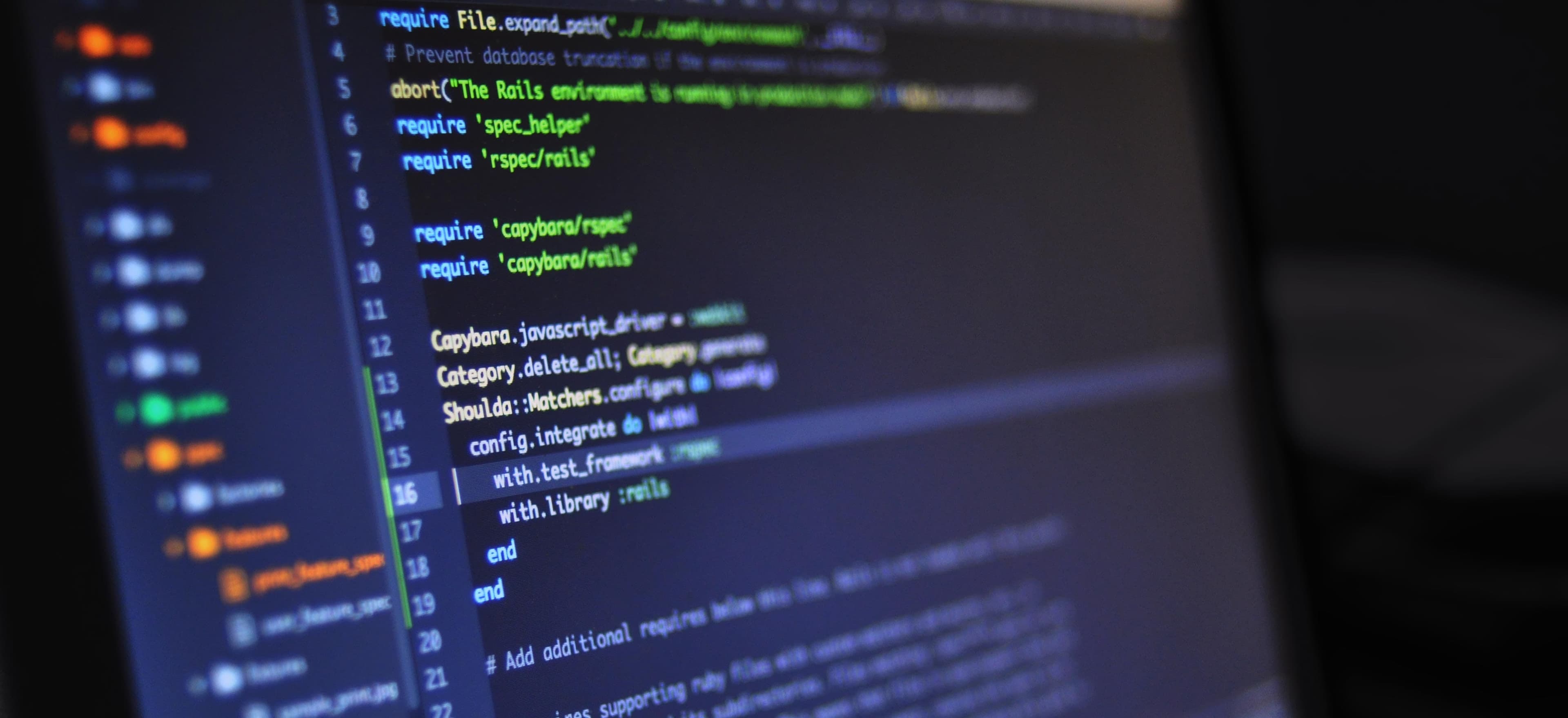
- Published on
Mastering Java 8 Streams: Grouping by Multiple Fields
Java 8 brought a lot of exciting features into the Java ecosystem, most notably the Streams API. This powerful feature allows developers to process collections of objects in a functional manner, making it easier to write clean, concise, and efficient code. In this post, we will explore how to group elements by multiple fields using Java 8 Streams, enabling you to harness the full potential of this approach in your applications.
Understanding Java 8 Streams
Before we dive into grouping by multiple fields, let’s review what Streams are. The Streams API allows for processing sequences of elements through a functional approach. Streams support various operations such as filtering, mapping, and reducing.
A key benefit of using streams is their ability to process data in a lazy fashion, improving performance especially in large datasets.
Sample Data Setup
To illustrate grouping by multiple fields, we will use a data class representing a person. Let's create a simple Person
class.
public class Person {
private String name;
private String city;
private int age;
public Person(String name, String city, int age) {
this.name = name;
this.city = city;
this.age = age;
}
// Getters
public String getName() { return name; }
public String getCity() { return city; }
public int getAge() { return age; }
}
Next, we will create a sample list of Person
objects to work with.
import java.util.Arrays;
import java.util.List;
public class Main {
public static void main(String[] args) {
List<Person> people = Arrays.asList(
new Person("John", "New York", 30),
new Person("Jane", "Los Angeles", 25),
new Person("John", "New York", 25),
new Person("Mike", "San Francisco", 30),
new Person("Jane", "Los Angeles", 25),
new Person("Mike", "New York", 40)
);
// Grouping people will be illustrated here
}
}
Grouping by a Single Field
Before we explore grouping by multiple fields, let's look at grouping by a single field. This sets the stage for a more complex grouping operation.
You can group people by their city, for example:
import java.util.Map;
import java.util.stream.Collectors;
Map<String, List<Person>> peopleByCity = people.stream()
.collect(Collectors.groupingBy(Person::getCity));
peopleByCity.forEach((city, pList) -> {
System.out.println("City: " + city);
pList.forEach(person -> System.out.println(" - " + person.getName()));
});
Code Explanation
- stream(): Converts the list into a stream for processing.
- collect(): Used to accumulate the elements of the stream into a collection.
- groupingBy(): A collector that groups elements by a classifier function.
This code snippet organizes Person
objects by their city. The output would look like this:
City: New York
- John
- John
- Mike
City: Los Angeles
- Jane
- Jane
City: San Francisco
- Mike
Grouping by Multiple Fields
Now, let's take it a step further and group the persons by both city and age. Doing this requires a composite key since one key cannot capture both fields.
Creating a Composite Key
For our purpose, we'll create an inner class, CityAgeKey
, to hold the city and age as keys.
public class CityAgeKey {
private String city;
private int age;
public CityAgeKey(String city, int age) {
this.city = city;
this.age = age;
}
@Override
public String toString() {
return "City: " + city + ", Age: " + age;
}
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (!(o instanceof CityAgeKey)) return false;
CityAgeKey that = (CityAgeKey) o;
return age == that.age && city.equals(that.city);
}
@Override
public int hashCode() {
return Objects.hash(city, age);
}
}
Grouping Logic
Now, we can use the CityAgeKey
in our grouping operation:
Map<CityAgeKey, List<Person>> groupedByCityAndAge = people.stream()
.collect(Collectors.groupingBy(person -> new CityAgeKey(person.getCity(), person.getAge())));
groupedByCityAndAge.forEach((key, pList) -> {
System.out.println(key);
pList.forEach(person -> System.out.println(" - " + person.getName()));
});
Code Explanation
- new CityAgeKey(...): Creates a new composite key for each person's city and age.
- groupingBy: Still groups, but now uses our custom key class.
Output
When you run this code, the output should look like this:
City: New York, Age: 30
- John
City: New York, Age: 25
- John
City: New York, Age: 40
- Mike
City: Los Angeles, Age: 25
- Jane
- Jane
City: San Francisco, Age: 30
- Mike
Further Analysis with Collectors
Now that we have grouped by multiple fields, you might want to analyze this group further. Let's say we wanted to count the number of people in each city-age combination.
Using the counting()
collector, we can achieve this as follows:
Map<CityAgeKey, Long> countByCityAndAge = people.stream()
.collect(Collectors.groupingBy(person -> new CityAgeKey(person.getCity(), person.getAge()), Collectors.counting()));
countByCityAndAge.forEach((key, count) -> {
System.out.println(key + ": " + count);
});
Code Explanation
- Collectors.counting(): Counts the number of elements in each group.
Output
You'll see output similar to:
City: New York, Age: 30: 1
City: New York, Age: 25: 1
City: New York, Age: 40: 1
City: Los Angeles, Age: 25: 2
City: San Francisco, Age: 30: 1
Key Takeaways
Java 8 Streams have significantly simplified data processing, allowing us to write less code while improving readability and maintainability. In this article, we learned how to group data by multiple fields effectively, which is especially useful when working with complex datasets in real-world applications.
The flexibility of Streams and Collectors means that you can customize your grouping operations to suit a variety of needs. Whether you are building a small application or working on a large enterprise solution, mastering streams is an invaluable skill.
For further reading, consider checking out the official Java Documentation for the Streams API.
Happy coding!