How Java Optionals Can Reduce NullPointerExceptions
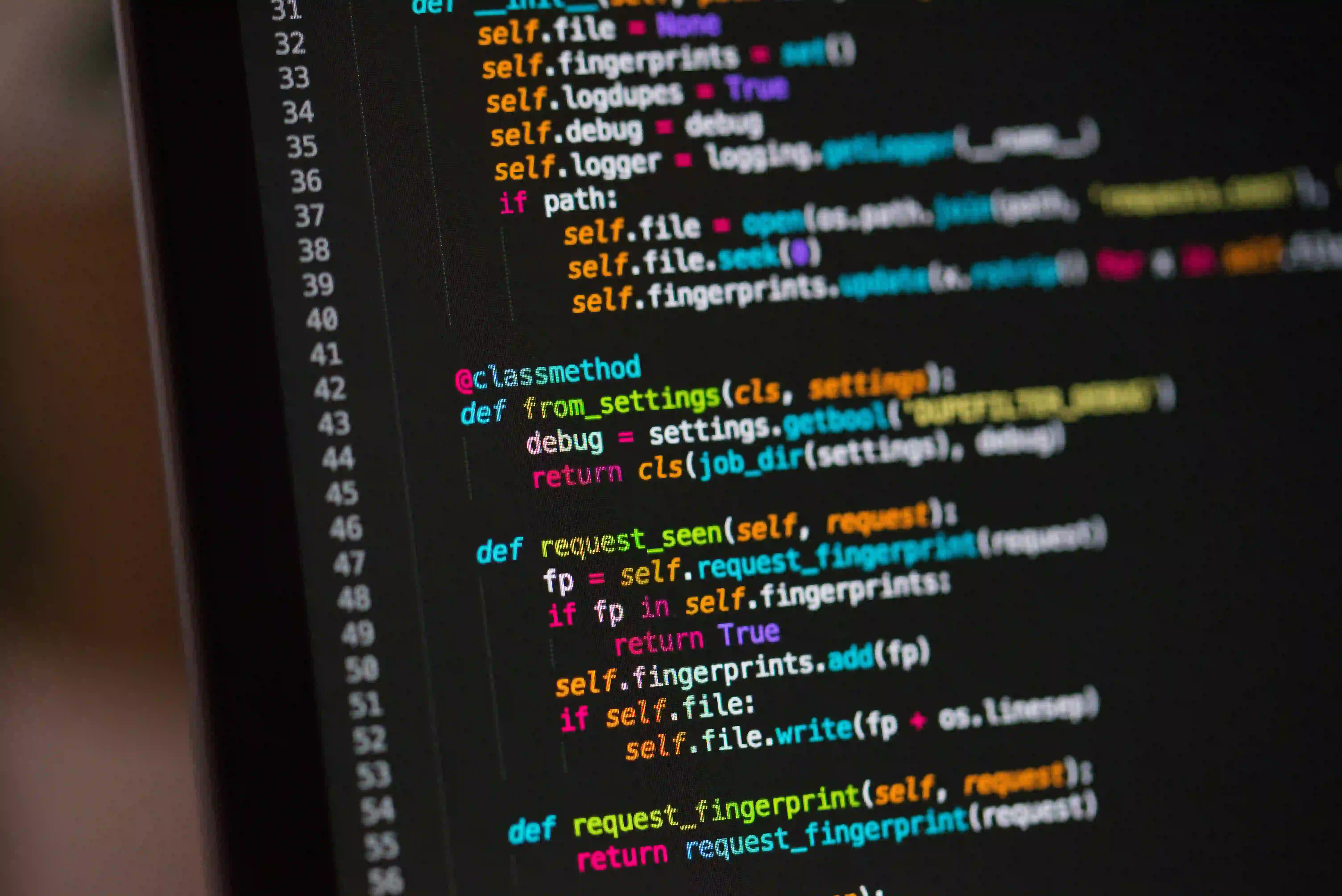
How Java Optionals Can Reduce NullPointerExceptions
Null Pointer Exceptions (NPE) are one of the most common issues in Java programming. They often lead to runtime failures and are notoriously difficult to debug. Fortunately, Java introduced the Optional
class in Java 8, which provides a way to express the presence or absence of a value without resorting to null. In this blog post, we will delve into Optionals
, their advantages, and how they can significantly reduce NPEs in your Java applications.
Understanding NullPointerExceptions
Before we dive into Optionals
, it's crucial to understand the bane that is the NullPointerException.
public class Example {
private String example;
public void printLength() {
// This will throw NullPointerException if 'example' is null
System.out.println(example.length());
}
}
In this code snippet, calling printLength()
without first ensuring that example
is set will result in a NullPointerException, crashing the program unexpectedly. Such scenarios are common when dealing with data that may or may not be present.
What is Optional?
The Optional<T>
class is a container object which may or may not contain a non-null value. It's part of the java.util
package and is designed to prevent NPEs by explicitly indicating whether a value is available.
Basic Example of Optional
Here's a simple demonstration of using Optional
:
import java.util.Optional;
public class UserProfile {
private String name;
private Optional<String> email;
public UserProfile(String name, String email) {
this.name = name;
this.email = Optional.ofNullable(email);
}
public Optional<String> getEmail() {
return email;
}
public static void main(String[] args) {
UserProfile user1 = new UserProfile("John", "john@example.com");
UserProfile user2 = new UserProfile("Jane", null);
System.out.println(user1.getEmail().orElse("No email provided"));
System.out.println(user2.getEmail().orElse("No email provided"));
}
}
Code Commentary
-
Building Optional: In the constructor,
Optional.ofNullable(email)
wraps the email string. If it is null, an empty Optional is produced, avoiding a NullPointerException. -
Retrieving Values: The
orElse
method provides a default value if the Optional is empty, which in this example is "No email provided". This approach not only avoids NPEs but also provides clarity on the result of an absent value.
Advantages of Using Optional
1. Clearer Code & Intent: By using Optional
, you make your code’s intent explicit. Developers can see that absence of a value is an expected condition, rather than a potential bug.
2. Avoiding Boilerplate: Traditional methods involving nullable attributes often lead to lots of null checks, making code verbose and harder to maintain. Optionals
consolidate this logic into simple calls.
3. Method Chaining: The API facilitates method chaining and allows for functional programming techniques to handle optional values gracefully.
Common Optional Methods
Here’s a rundown of some commonly used methods in the Optional
class along with examples:
-
empty(): Returns an empty Optional.
☕snippet.javaOptional<String> emptyOptional = Optional.empty();
-
of(T value): Returns an Optional with a non-null value.
☕snippet.javaOptional<String> nonEmpty = Optional.of("Hello");
-
ofNullable(T value): Returns an Optional that may be empty, depending on the input.
☕snippet.javaOptional<String> userInput = Optional.ofNullable(getUserInput());
-
isPresent(): Checks whether a value is present.
☕snippet.javaif (userInput.isPresent()) { System.out.println("Input: " + userInput.get()); }
-
ifPresent(): Executes a Consumer if a value is present.
☕snippet.javauserInput.ifPresent(input -> System.out.println("User Input: " + input));
-
orElse(): Returns the value if present, otherwise returns a default value.
☕snippet.javaString defaultValue = userInput.orElse("Default Value");
-
map(): Transforms the value if present.
☕snippet.javaString upperCaseInput = userInput.map(String::toUpperCase).orElse("DEFAULT");
When Not to Use Optional
While Optional
is a powerful feature, it is not a silver bullet. There are scenarios where using Optional
may not be suitable:
-
Avoiding Optional in Fields: Do not use
Optional
as a field type. It generally leads to more complexity and overhead. -
Performance Critical Situations: Since
Optionals
introduce additional overhead due to object allocation, consider traditional null checks in performance-sensitive areas. -
Serialization: If working with libraries or frameworks that serialize your objects, using Optionals can lead to unexpected behavior since they are not inherently serializable.
The Bottom Line
Optional
is a game-changer in the Java ecosystem. It provides a robust way to handle optional values without the fear of NullPointerExceptions. By using Optional
, you're not just avoiding NPEs; you're also enhancing the clarity, maintainability, and robustness of your code.
To further enhance your Java skills, consider diving into Java's Stream API, which integrates well with Optional
. Additionally, explore Effective Java by Joshua Bloch for best practices on using Java features effectively.
By adopting Optionals
, you'll make your Java code safer and cleaner, preparing yourself for challenges in larger software systems. Embrace this feature and say goodbye to NPEs!