Boosting Microservices Performance: Key Load Testing Strategies
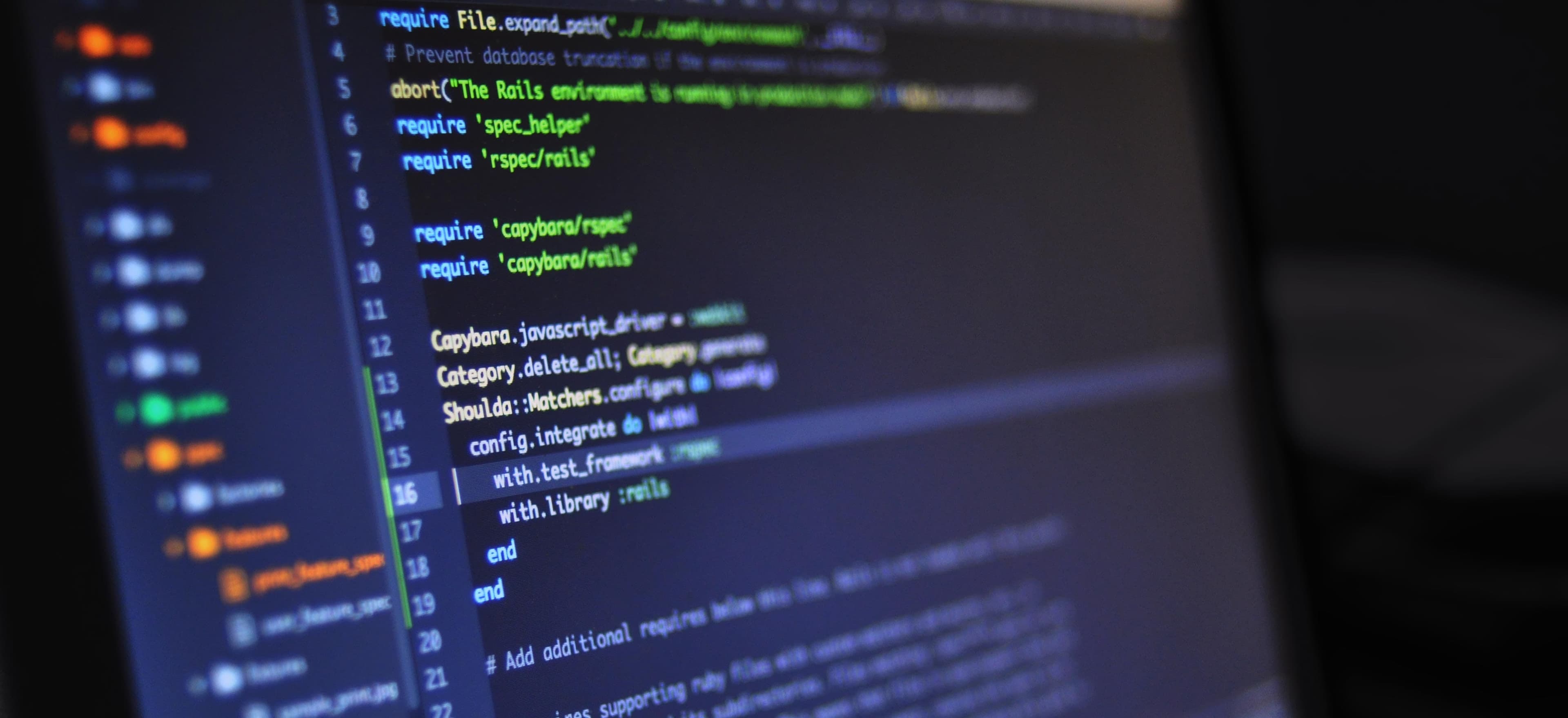
- Published on
Boosting Microservices Performance: Key Load Testing Strategies
In today's rapidly changing technological landscape, microservices architectures have become the gold standard for building scalable applications. They allow developers to deploy services independently, speed up development cycles, and enable teams to focus on their specific areas of expertise. However, with great power comes great responsibility—especially regarding performance. In this blog post, we'll discuss various load testing strategies crucial for enhancing microservices performance.
Understanding Load Testing
Load testing is a crucial step in assessing how systems function under varying degrees of load. It helps identify bottlenecks and ensures that your application can handle the expected load without compromising performance. For microservices architectures, where services are dependent on one another, effective load testing can make all the difference between a seamless user experience and catastrophic failure.
Why Load Testing is Important for Microservices
Microservices bring multiple advantages, but they also introduce complexity. Each service's performance can affect others, making it vital to understand how they interact under load. Some core reasons to implement load testing include:
- Early Detection of Bottlenecks: Identifying performance issues before they impact users.
- Capacity Planning: Understanding how much load your microservices can withstand helps in resource allocation and scaling.
- Improved Reliability: Ensuring performance under various scenarios builds confidence in your application’s reliability.
- Regressions Monitoring: Making sure new changes don’t negatively affect existing performance.
Load Testing Strategies for Microservices
Load testing doesn't have to be monotonous. Adopting varied strategies can yield comprehensive insights into your microservices performance. Here are some key strategies:
1. Unit Testing for Performance
While unit testing is often thought of in terms of functionality, it's also essential for performance. Each microservice can be unit tested to ensure it meets specific performance benchmarks.
Example Code
Here's a simple example of a unit test written using JUnit in Java to evaluate the performance of a hypothetical service method:
import org.junit.jupiter.api.Test;
import static org.junit.jupiter.api.Assertions.assertTrue;
public class MyServiceTest {
@Test
public void testPerformance() {
long startTime = System.currentTimeMillis();
MyService service = new MyService();
service.performOperation();
long endTime = System.currentTimeMillis();
long duration = endTime - startTime;
// Ensure it completes within a specific duration (e.g., 200 ms)
assertTrue(duration < 200, "Service performance exceeds 200ms");
}
}
Why? This code snippet tests whether the method performOperation()
completes within an acceptable timeframe (200 ms), offering confidence in the unit's performance characteristics.
2. Simulating Real User Conditions
To truly understand how your microservices will perform under load, simulations should reflect a real user environment. Tools like Apache JMeter or Gatling can be employed to simulate both normal and peak load conditions.
Example JMeter Setup
You can set up your test by creating a Thread Group and configuring the number of users and ramp-up period. This configuration allows JMeter to simulate 100 users over 10 seconds, steadily increasing load.
Why? By replicating user behavior and interaction patterns, you gather more accurate data on how your services perform under realistic conditions.
3. End-to-End Testing
End-to-end testing focuses on testing the path a user takes from the start to the end of an operation. It helps ensure that all microservices work harmoniously under load.
Code Example
Running a simple end-to-end load test can be accomplished using frameworks like RestAssured:
import io.restassured.RestAssured;
import org.junit.jupiter.api.Test;
public class EndToEndLoadTest {
@Test
public void testLoadDuringUserFlow() {
for (int i = 0; i < 100; i++) {
RestAssured.given()
.header("Content-Type", "application/json")
.body("{\"userId\": 1, \"action\": \"login\"}")
.when()
.post("http://localhost:8080/api/login")
.then()
.statusCode(200);
}
}
}
Why? This approach imitates real user flows and ensures that all system components are functioning well together, thereby identifying weaknesses that are not apparent in isolated tests.
4. Capacity Testing
Capacity testing measures how many users or transactions a microservice can handle at any given time. It is imperative to understand the thresholds of your services to plan scaling effectively.
Approach
- Determine baseline metrics for each microservice.
- Gradually increase the load until performance degradation occurs.
- Document at what point degradation starts to gather critical insights.
Why? This information allows you to establish informed limits and effectively plan for future traffic during peak times.
5. Stress Testing
Stress testing goes beyond capacity testing by determining how much stress your microservices can endure before they fail. This kind of testing is essential for understanding the limits of your application.
How to Perform Stress Testing
- Push the application beyond its limits for an extended period.
- Identify failure points, response times, and unexpected behaviors.
Why? Knowing how your system reacts under extreme conditions helps prepare for unexpected spikes in traffic.
6. Monitoring Performance Metrics
Use monitoring tools such as Prometheus or Grafana to keep track of performance metrics during load tests. Gather data on:
- Response times
- Error rates
- Resource utilization (CPU, memory, and disk I/O)
Why? Monitoring should not be an afterthought but an integral part of the load testing process. It provides insights that can inform architectural changes and optimizations.
Key Considerations When Load Testing Microservices
-
Service Dependencies: Recognize that one microservice's performance may depend on another. Consider simulating these dependencies without actually hitting every service during load testing.
-
Realistic Data Sets: Use realistic data sets that mimic production data to gain more accurate results.
-
Test Automation: Automate load tests to ensure they can be executed frequently and with ease.
-
Frequent Testing: Regularly scheduled load tests can capture performance degradation early, especially as microservices often undergo constant changes.
-
Analyze Results: After each test, don’t just capture data—analyze it to understand performance bottlenecks and areas needing improvement.
The Last Word
Load testing microservices is essential for ensuring performance and reliability in a distributed architecture. By implementing varied strategies—unit testing, end-to-end testing, and performance monitoring—you can effectively prepare your application for real-world challenges.
With the right tools and techniques, you can optimize performance and offer users the seamless experience they expect. Start integrating these load testing strategies today and watch your microservices thrive under pressure.
For further reading on microservices and performance testing, consider exploring:
- Google Cloud’s best practices on microservices
- Martin Fowler’s thoughts on microservices
By adopting and continuously refining the strategies discussed in this post, you're equipping your microservices with the resilience needed to excel in a competitive landscape.